Taming the Beast: Navigating Unintended Legacy Code Chaos
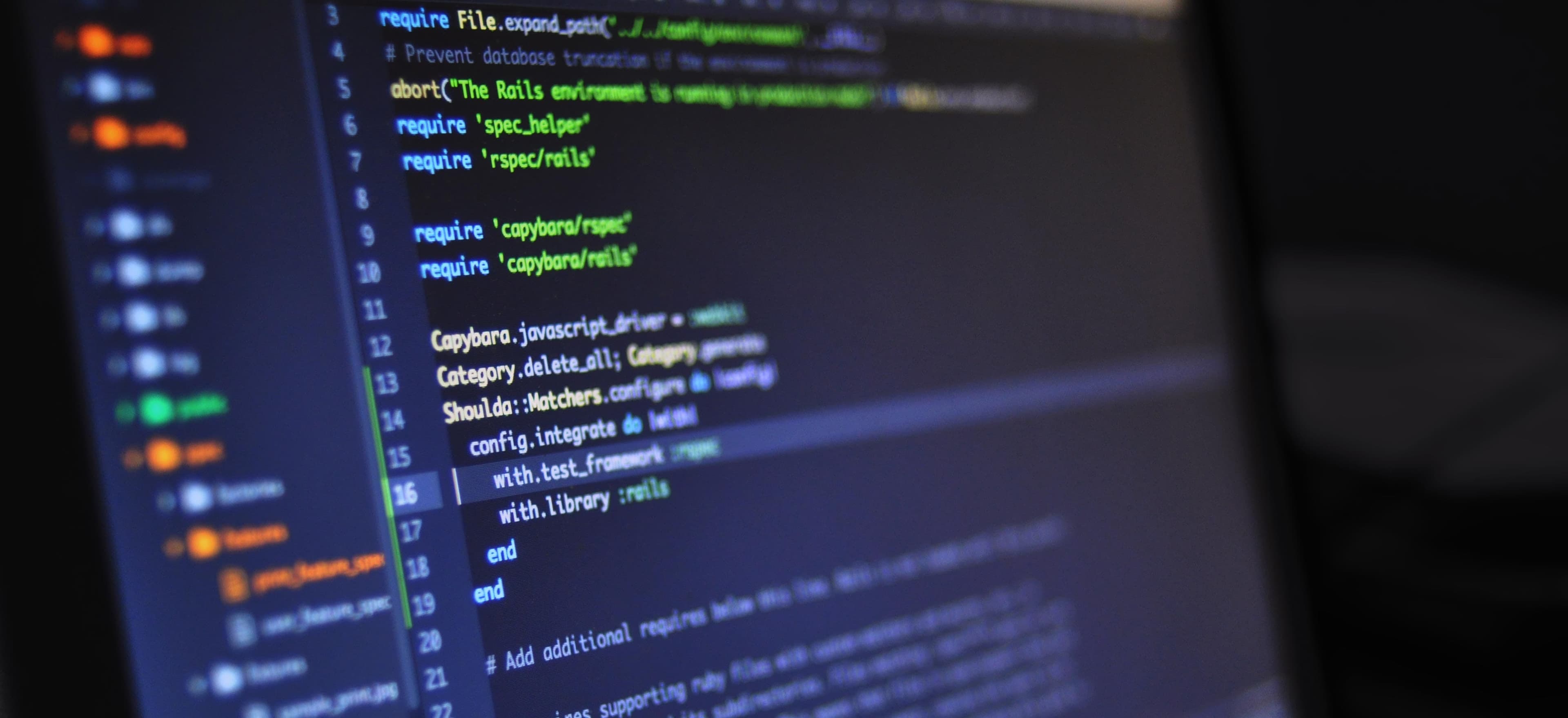
- Published on
Taming the Beast: Navigating Unintended Legacy Code Chaos
Legacy code is the bane of every developer's existence. What once started as a well-intentioned project can quickly devolve into a chaotic series of dependencies, outdated patterns, and infamous bugs. According to a survey by Stack Overflow, around 60% of professional developers spend at least half their time maintaining existing code. Given the substantial impact of legacy code on development efficiency, it's essential to discuss how to navigate and ultimately tame these beasts.
In this post, we will explore what legacy code is, why it often gets a bad rap, and how we can manage it effectively. Moreover, we will dive into practical Java examples, addressing some common pitfalls and how to refactor for a more manageable and maintainable codebase.
What is Legacy Code?
Legacy code refers to any code that is inherited from someone else or from an older version of a system. It can be a program written in an outdated programming language, or, more locally, Java code that does not adhere to current design principles or practices. Such code often lacks proper documentation, tests, and clarity, making it even more challenging.
Here's why legacy code can be likened to a beast:
- Riddled with Bugs: Over time, as more features are added, legacy code may begin to develop unexpected behaviors.
- Difficult to Understand: Poorly structured code can be nearly impossible to decipher, especially without clear comments.
- Dependency Hell: Legacy systems often rely on outdated libraries and frameworks which may not be compatible with modern tools.
Understanding these challenges illustrates why taming the beast of legacy code is so important.
Why Tame the Legacy Beast?
Before we explore strategies to manage legacy code, let’s consider why it’s crucial to prioritize this task.
- Maintainability: Easier to understand and modify code means less time spent in bug fixes and feature updates.
- Scalability: Clear, organized code can easily accommodate new features without spiraling into chaos.
- Team Morale: Developers tend to feel more satisfied when working with clean, comprehensible, and efficient code.
In essence, taking the time to manage your code can save more time than you spend on it.
Dealing with Legacy Code: Practical Strategies
Now, let's look at some strategies we can employ to tame legacy code, focusing on Java as our primary programming language.
1. Understand the Code
Before making any adjustments, genuinely comprehend the existing codebase. This involves reading through the code, commenting where necessary, and even constructing diagrams to visualize the system architecture.
Example: Understanding a Legacy Java Class
Consider a Java class representing a customer:
public class Customer {
private String name;
private int id;
private String email;
public Customer(String name, int id, String email) {
this.name = name;
this.id = id;
this.email = email;
}
public String getName() {
return name;
}
public int getId() {
return id;
}
public String getEmail() {
return email;
}
}
While this class appears straightforward, understanding how it interacts within the broader application is vital. You might require additional documentation or contextual understanding of how Customer
objects are created, modified, and stored.
2. Write Tests
One of the most important steps in handling legacy code is to write tests to assist with refactoring. Tests help ensure that functionality remains intact despite changes.
Example: Writing a JUnit Test
Here’s how you can write a simple JUnit test for the Customer
class shown earlier:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class CustomerTest {
@Test
public void testCustomerCreation() {
Customer customer = new Customer("John Doe", 1, "john@example.com");
assertEquals("John Doe", customer.getName());
assertEquals(1, customer.getId());
assertEquals("john@example.com", customer.getEmail());
}
}
This unit test checks the basic functionality of the Customer
class. Writing automated tests helps you ensure the system behaves as expected as you make changes.
3. Refactor Gradually
Refactoring should be incremental. Avoid the temptation to rewrite entire classes or systems from scratch in one go, as this method is fraught with risks. Focus on one aspect of the code at a time, methodically improving it while preserving existing behavior.
Example: Refactoring a Method
Suppose we have an unwieldy method in a class:
public void processOrder(Order order) {
// outdated way of handling orders
if (order.getStatus() == OrderStatus.NEW) {
// process order
} else if (order.getStatus() == OrderStatus.PROCESSED) {
// handle processed orders
}
// many more conditions...
}
Through refactoring, we can make this method more organized:
public void processOrder(Order order) {
switch (order.getStatus()) {
case NEW:
handleNewOrder(order);
break;
case PROCESSED:
handleProcessedOrder(order);
break;
// more cases...
default:
handleUnknownStatus(order);
}
}
The switch statement improves readability and maintainability, making it easier for future developers to add more order statuses without too much hassle.
4. Implement Design Patterns
Leveraging design patterns can often mitigate some of the messiness of legacy code. Patterns such as Adapter, Strategy, and Factory can yield tremendous benefits in making the codebase cleaner and more manageable.
Using the Strategy pattern in our Customer
example above could allow different payment methods to be encapsulated. It clarifies the payment process and keeps our code organized.
Example: Implementing the Strategy Pattern
public interface PaymentStrategy {
void pay(int amount);
}
public class CreditCardPayment implements PaymentStrategy {
public void pay(int amount) {
// Processing credit card payment
}
}
public class PaypalPayment implements PaymentStrategy {
public void pay(int amount) {
// Processing PayPal payment
}
}
In this example, we centralize payment logic that enhances maintainability and focus, making it easier to incorporate new payment options later.
The Bottom Line
Taming legacy code is no small feat, but with a solid strategy in mind, it becomes a feasible endeavor that pays off handsomely. Start by understanding the codebase, writing tests, gradually refactoring, and implementing design patterns.
Legacy systems may not always be easy to work with. However, by applying these principles, you can turn what was once a scary beast into a well-disciplined pet that obeys your commands. Consider diving deeper with resources like Martin Fowler's Refactoring and Java Design Patterns to further expand your knowledge and best practices.
Here's to a future of cleaner, more maintainable Java code—where the beast resides peacefully!