Common Java Coding Mistakes and How to Avoid Them
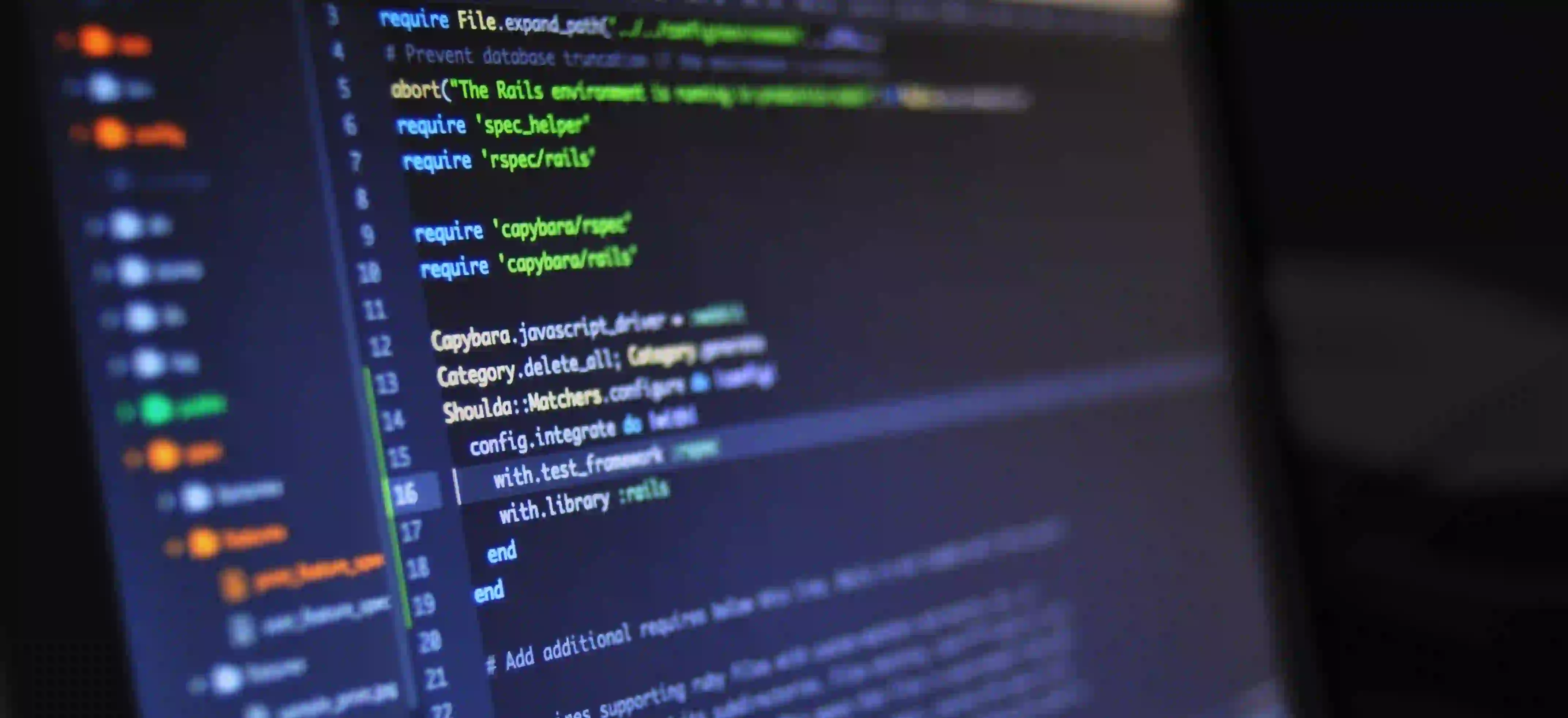
Common Java Coding Mistakes and How to Avoid Them
Java is a powerful programming language that is widely used for building a variety of applications. However, even seasoned developers can fall prey to common coding mistakes. In this blog post, we will explore some of these pitfalls and offer practical advice on how to avoid them.
1. Not Using Proper Naming Conventions
Why It Matters
Proper naming conventions improve code readability and maintainability. When developers give meaningful names to variables, methods, and classes, it makes the code easier to understand for both the original author and others who may work on it in the future.
Common Issues
Using vague or overly abbreviated names can lead to confusion. For example, naming a variable a
or temp
does not convey its purpose.
Example of Poor Naming:
int a; // What does 'a' represent?
Recommended Approach:
Use descriptive names:
int userAge; // Clearly indicates that this variable stores the age of a user
Best Practice
Follow Java naming conventions:
- Classes: CamelCase (e.g.,
CustomerOrder
) - Variables/Methods: lowerCamelCase (e.g.,
calculateTotal
)
2. Ignoring Exceptions
Why It Matters
Java is known for its robust exception handling capabilities. Ignoring exceptions can lead to program crashes or unexpected behavior.
Common Issues
Failing to catch exceptions or inadequately logging them can make debugging difficult.
Example of Ignoring Exceptions:
public void readFile(String fileName) {
FileReader file = new FileReader(fileName); // What if the file does not exist?
// More code here
}
Recommended Approach:
Always catch exceptions and log them properly:
public void readFile(String fileName) {
try {
FileReader file = new FileReader(fileName);
// More code here
} catch (FileNotFoundException e) {
System.err.println("File not found: " + e.getMessage());
}
}
Best Practice
Use logging frameworks like SLF4J or Log4j to log exceptions and application flow.
3. Not Using final
Keyword
Why It Matters
The final
keyword in Java can be used to declare constants, prevent method overriding, and prevent inheritance. Not using it where appropriate can lead to unintended modifications of variables and methods.
Common Issues
Developers may forget to mark variables as final
, allowing them to be modified mistakenly.
Example of Not Using final
:
int number = 10;
number = 20; // This may not be intended
Recommended Approach:
Declare constants as final
:
final int MAX_USERS = 100; // This value cannot be changed later
Best Practice
Use final
for variables that should not change. This adds clarity to your code.
4. Overusing Static Methods
Why It Matters
Static methods allow you to call functions without creating an instance of a class. However, overusing them can lead to less flexible and more difficult-to-test code.
Common Issues
Developers might use static methods indiscriminately, leading to procedural programming rather than utilizing object-oriented principles.
Example of Overusing Static Methods:
public static void calculateTax() {
// Tax calculation logic
}
Recommended Approach:
Prefer instance methods where appropriate:
public class TaxCalculator {
public double calculateTax(double income) {
// Tax calculation logic
}
}
Best Practice
Differentiate between utility classes (which may have static methods) and business logic classes. Only use static methods when they don’t depend on instance variables.
5. Failing to Leverage Collections Properly
Why It Matters
Java Collections Framework provides a set of classes to handle groups of objects more efficiently. Not leveraging these can lead to unnecessary complexity.
Common Issues
Using arrays where collections may be more appropriate can lead to issues with resizing and type safety.
Example of Poorly Using Arrays:
String[] names = new String[10];
names[0] = "Alice";
// Need to handle resizing manually later if more names come
Recommended Approach:
Use List from the Collections Framework:
List<String> names = new ArrayList<>();
names.add("Alice"); // Dynamic resizing handled automatically
Best Practice
Embrace the Java Collections Framework. It's powerful, flexible, and optimized for performance. Familiarize yourself with different types of collections such as List
, Set
, and Map
.
6. Not Following Code Formatting Standards
Why It Matters
Inconsistent code formatting can reduce readability and complicate collaboration. A well-formatted codebase enhances team productivity.
Common Issues
Not following indentation and brace styles leads to messy code that’s hard to read.
Example of Poor Formatting:
if(condition){
doSomething();
}
Recommended Approach:
Follow a consistent code style:
if (condition) {
doSomething();
}
Best Practice
Use an IDE like IntelliJ IDEA or Eclipse, which allows you to auto-format your code according to defined style guidelines.
The Bottom Line
Avoiding these common Java coding mistakes can significantly enhance the quality of your applications. By adopting best practices like proper naming conventions, effective exception handling, and appropriate use of collections, you can improve code readability, maintainability, and reduce bugs.
Always remember that software development is not just about writing code; it’s about building systems that are clear, efficient, and scalable.
For more in-depth information, take a look at Effective Java by Joshua Bloch, which covers many best practices and principles of Java programming.
Happy coding!