Boost Your Database Performance in Just 5 Easy Steps!
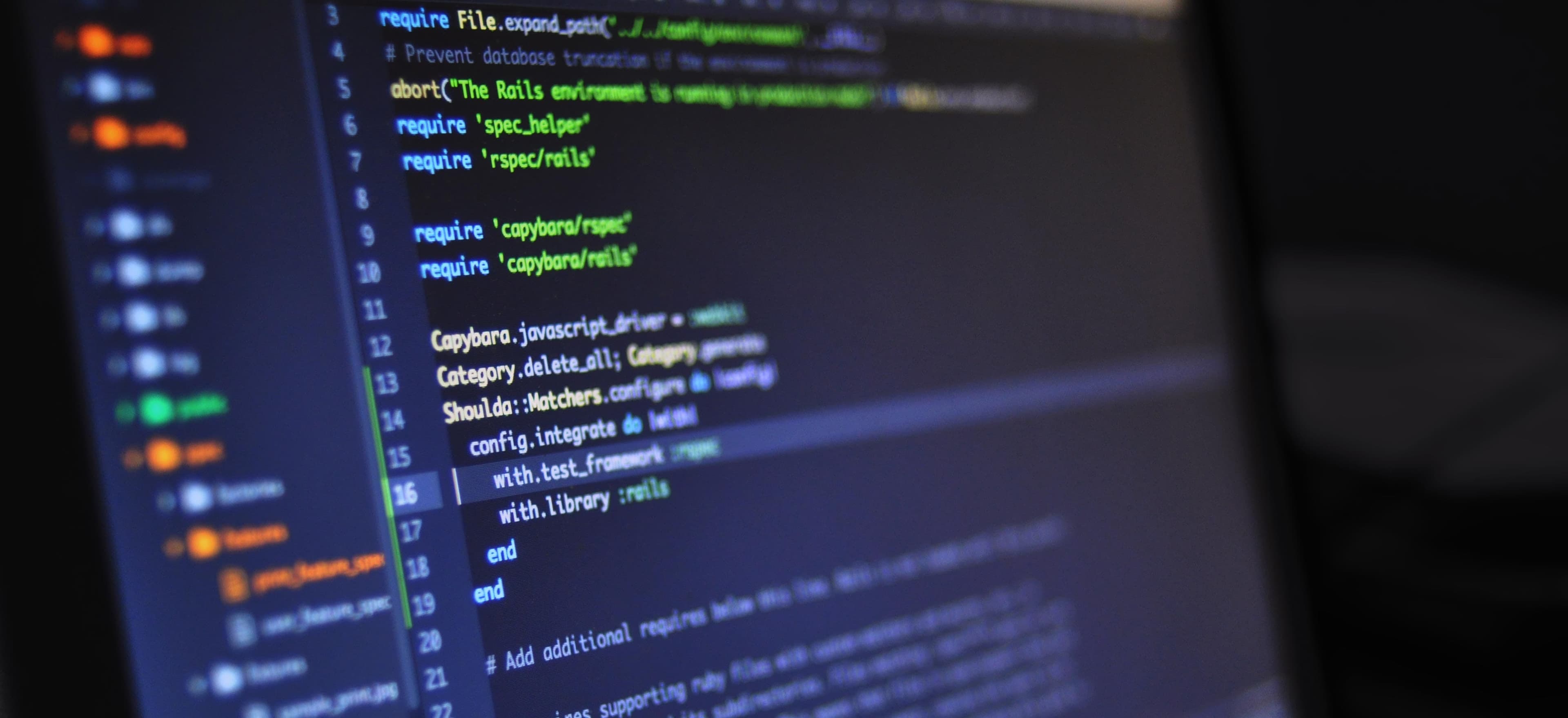
- Published on
Boost Your Database Performance in Just 5 Easy Steps!
In the world of software development, database performance is paramount. A slow database can cripple an application, frustrate users, and ultimately drag down your business. However, optimizing your database performance doesn't need to be a daunting task. In this blog post, we will explore five key strategies that can dramatically enhance your database’s performance, particularly focusing on those using Java applications.
Step 1: Employ Indexing Effectively
Indexes are essential tools when it comes to enhancing the speed of data retrieval operations. Think of an index like a book's table of contents; without it, finding specific information would be time-consuming.
Why Use Indexes?
Indexes allow the database engine to find data without scanning every row in a table. This speed-up can be crucial in high-volume transactions.
Example Code Snippet
// Creating an index in SQL
CREATE INDEX idx_user_email ON users(email);
This SQL command creates an index on the users’ email column, accelerating queries searching for user emails.
Note: However, be cautious—over-indexing can lead to increased storage costs and slower write operations. Balance is key.
Step 2: Optimize Queries
Just as a well-written query can improve performance, poorly structured queries can significantly slow things down. The goal is to write efficient SQL statements that retrieve only the data you need.
Why Optimize Queries?
Inefficient queries can lead to full table scans, which are time-consuming and resource-intensive.
Example Code Snippet
// Instead of using this...
SELECT * FROM orders WHERE user_id = ?;
// Use this:
SELECT id, order_date, total_amount FROM orders WHERE user_id = ?;
By selecting only the necessary columns, we reduce the amount of data processed during the query.
Resource for Further Reading
For a deeper dive into SQL query optimization strategies, check out SQL Performance: Optimizing Query Execution.
Step 3: Connection Pooling
In a multi-threaded application, establishing a new connection to the database for every request can be resource-intensive. Connection pooling mitigates this overhead by reusing connections, thus improving performance.
Why Use Connection Pooling?
It lowers the cost of repeatedly opening and closing connections, which can be time-consuming and bad for performance.
Example Code Snippet
import javax.sql.DataSource;
import org.apache.commons.dbcp.BasicDataSource;
// Setting up a connection pool
BasicDataSource dataSource = new BasicDataSource();
dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/mydb");
dataSource.setUsername("user");
dataSource.setPassword("password");
dataSource.setInitialSize(5);
dataSource.setMaxTotal(10);
This code configures a connection pool using Apache Commons DBCP. You specify the maximum number of connections which can be opened, thus controlling resource use.
Step 4: Utilize Caching
Caching frequently accessed data can greatly speed up application response times. Instead of hitting the database every time for common queries, you can store those results temporarily in memory.
Why Implement Caching?
By keeping data in memory, fetch times significantly reduce, leading to lower latency in user interactions.
Example Code Snippet
import java.util.HashMap;
import java.util.Map;
// Simple cache implementation
public class SimpleCache {
private Map<String, String> cache = new HashMap<>();
public String getValue(String key) {
return cache.get(key);
}
public void putValue(String key, String value) {
cache.put(key, value);
}
}
This simplistic Caching mechanism stores key-value pairs in memory. For a more detailed caching strategy, consider using libraries like Ehcache or Redis for production-grade implementations.
Resource for Further Reading
To see how various caching strategies can improve application performance, visit The Ultimate Guide to Caching.
Step 5: Regular Maintenance
Like any well-tuned machine, databases require regular maintenance. This step involves tasks such as updating statistics, defragmenting tables, and analyzing execution plans.
Why Regular Maintenance?
Preventive measures are crucial for long-term performance. Regular reviews and maintenance can catch performance issues before they escalate.
Example Maintenance SQL
ANALYZE TABLE users;
OPTIMIZE TABLE orders;
In this SQL snippet, ANALYZE
updates the statistics used by the SQL query planner, whereas OPTIMIZE
reorganizes the physical storage of the table.
Closing the Chapter
Boosting database performance does not have to be a complicated endeavor. By implementing indexing effectively, optimizing queries, utilizing connection pooling, applying caching wisely, and scheduling regular maintenance, you can substantially enhance your database’s speed and efficiency.
The strategies outlined in this article are user-friendly and scalable, making them applicable whether you're developing a small business application or a large-scale enterprise software. Get started on these optimizations today and see the transformation in your application's performance!
Additional Resources
- For more details on database performance tuning, refer to Database Performance Tuning.
- Interested in advanced Java practices? Check out Java Concurrency in Practice.
Feel free to share your thoughts and experiences with database performance improvements in the comments below! Happy coding!
Checkout our other articles