Mastering Apache Camel: Common Pitfalls to Avoid
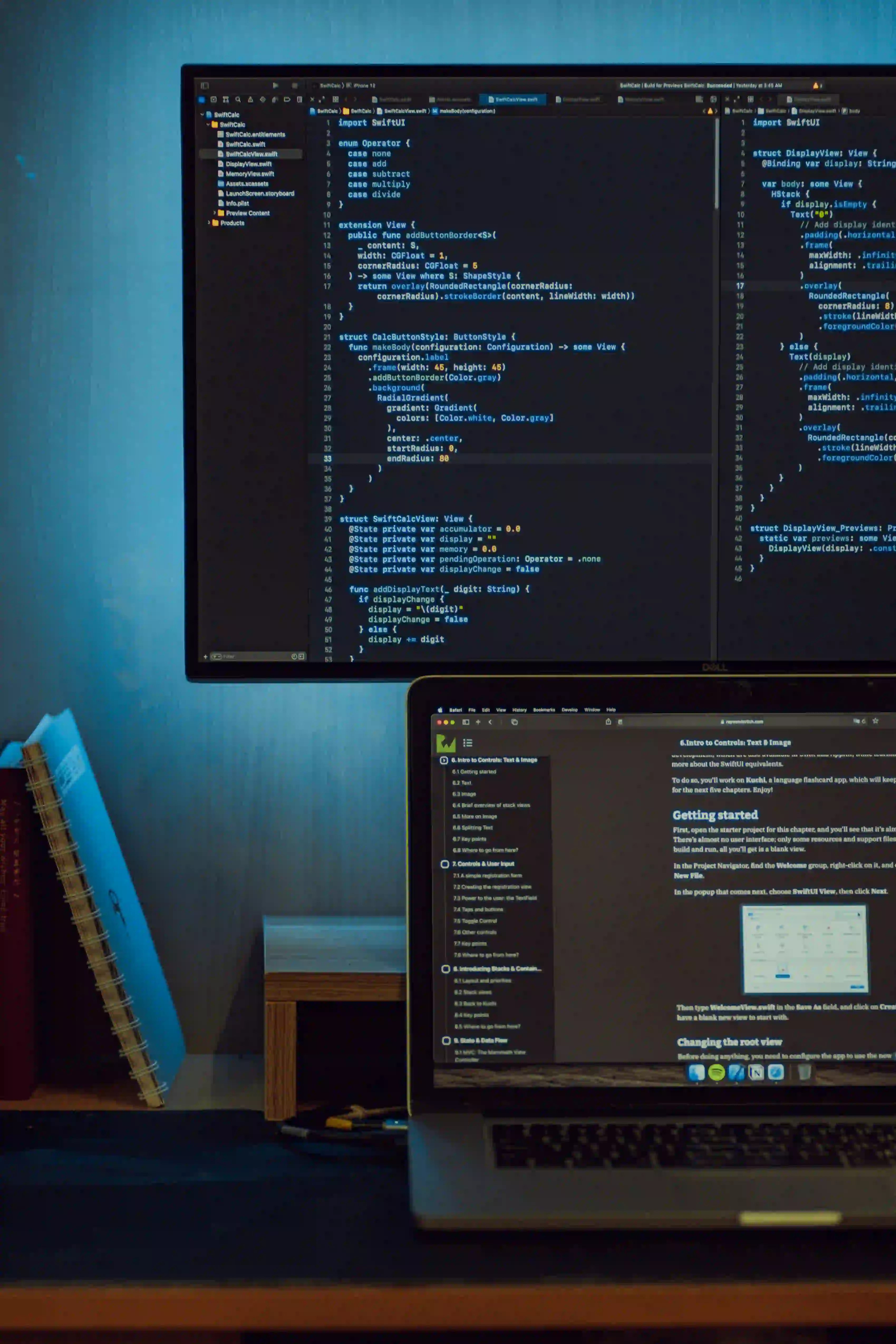
Mastering Apache Camel: Common Pitfalls to Avoid
In the world of integration patterns and enterprise application integration (EAI), Apache Camel is a powerful framework that allows developers to easily manage message routing, transformation, and more. While Camel boasts a flexible API and rich support for various protocols, many developers encounter common issues that can impact their efficiency. This blog post aims to identify these pitfalls and provide guidance on how to avoid them, helping you master Apache Camel.
Understanding Apache Camel
Apache Camel is an open-source integration framework that provides a standardized way to define message routing and mediation rules using a simple domain-specific language (DSL). With a wide array of components and protocols, Camel helps developers integrate various systems seamlessly.
Why Use Apache Camel?
- Versatility: Supports numerous protocols (HTTP, FTP, JMS, etc.) and data formats (XML, JSON, etc.)
- Simplicity: Easy to learn with a concise DSL for defining routes
- Flexibility: Component-based architecture allows easy extension and customization
- Community: Robust community support and documentation available
Still, like any tool, Apache Camel has its challenges, especially for those new to the framework. Let's dive into some common pitfalls that developers should avoid when working with Apache Camel.
1. Ignoring the Learning Curve
The Problem
Many developers underestimate the learning curve associated with Apache Camel. The extensive range of consumers, processors, and endpoints can be overwhelming.
Solution
Take the time to study the core concepts and components involved in Apache Camel. Start with the Apache Camel Documentation to understand routes, processors, and various DSL options.
Example
from("file:data/inbox?noop=true")
.to("log:incoming-file");
This simple route reads files from a specified directory and logs the incoming files. Familiarizing yourself with similar examples can help you understand the framework better!
2. Overcomplicating Routes
The Problem
Another common mistake is overcomplicating Camel routes. Developers sometimes create complex, convoluted routes that are hard to maintain and debug.
Solution
Keep your routes as simple and modular as possible. Break down larger routes into smaller, reusable components. This not only enhances readability but also improves maintainability.
Example
from("direct:start")
.process(exchange -> {
String input = exchange.getIn().getBody(String.class);
exchange.getIn().setBody(input.toUpperCase());
})
.to("direct:end");
In this route, the transformation is isolated to a single processor. If business logic changes, you can easily adjust this without affecting the entire route.
3. Neglecting Error Handling
The Problem
Forgetting to implement proper error handling is a common shortfall. When encounters errors, your routes might fail silently, leading to lost messages.
Solution
Use Camel's error handling features, such as the errorHandler
and the onException
DSL, to catch and handle exceptions appropriately.
Example
onException(Exception.class)
.handled(true)
.to("log:error-handler")
.to("direct:error-recovery");
In this example, any exception that occurs will be sent to a specified log and an error recovery route. This ensures that you can handle and remedy issues effectively.
4. Not Leveraging Component Configuration
The Problem
Developers often miss the opportunity to configure components effectively. Misconfiguring or overloading the default settings can lead to performance issues.
Solution
Understand the configuration options available for each component you're utilizing. For example, in a JMS route, setting the maxConcurrentConsumers
can drastically improve throughput.
Example
<bean id="jmsConfig" class="org.apache.camel.component.jms.JmsComponent">
<property name="connectionFactory" ref="connectionFactory"/>
<property name="maxConcurrentConsumers" value="10"/>
</bean>
Using the above configuration ensures efficient consumer management in a JMS-based route.
5. Missing Unit Tests
The Problem
Omitting unit tests is a frequent mistake made by developers when working with Camel. This often results in unverified routes and unexpected behaviors.
Solution
Investing time in unit testing improves the reliability of your routes. Camel offers a testing framework that simplifies writing and executing tests.
Example
CamelContext camelContext = new DefaultCamelContext();
camelContext.addRoutes(new MyRouteBuilder());
ProducerTemplate template = camelContext.createProducerTemplate();
template.sendBody("direct:start", "Hello Camel!");
assertMockEndpointsSatisfied();
By adding unit tests using CamelTestSupport, you ensure your routes behave as expected, ultimately saving time and effort in debugging later.
6. Overusing the Java DSL
The Problem
While the Java DSL provides flexibility and power, excessive use may lead to complex and less readable code.
Solution
Consider leveraging alternative DSLs like the Spring XML configuration or the REST DSL when appropriate. This can improve readability and simplify routing logic.
Example
<route>
<from uri="direct:start"/>
<process ref="myProcessor"/>
<to uri="mock:result"/>
</route>
Using XML-based routes highlights the routing configuration, making it easier for team members to understand at a glance.
7. Not Monitoring Camel Routes
The Problem
Many developers overlook the importance of monitoring their Camel routes. Lack of visibility can lead to undetected issues and subsequently lost data.
Solution
Utilize Camel's built-in monitoring features and external tools like Hawtio or Camel K to gain insights into your routes.
Example
With Hawtio, you can visualize your routes, monitor statistics, and quickly spot potential issues. Simply deploy your application with Hawtio enabled, and you can access a dashboard with real-time metrics.
Bringing It All Together
While Apache Camel is an incredibly robust and versatile integration framework, it presents unique challenges. By being aware of the common pitfalls discussed in this article, developers can navigate the complexities of Apache Camel with confidence.
The key takeaways from this discussion include:
- Invest in Knowledge: Understand Camel’s core concepts and components.
- Simplicity Wins: Keep routes clean, simple, and modular.
- Embrace Error Handling: Design proper error handling to manage exceptions effectively.
- Configuration is Key: Leverage the configuration options for optimized performance.
- Test for Success: Regularly implement unit tests to ensure route integrity.
- Select the Right DSL: Choose between Java, XML, or REST DSL as necessary.
- Monitor Your Routes: Implement monitoring solutions to gain insights and prevent issues.
By avoiding these pitfalls, you increase the reliability and efficiency of your integration projects with Apache Camel. Happy coding!
For further reading on integration patterns and best practices with Apache Camel, consider exploring the Apache Camel User Guide and the Camel Component Documentation. These resources will aid you as you continue your journey toward mastering Apache Camel.