Java Solutions for Handling Short Content Display Issues
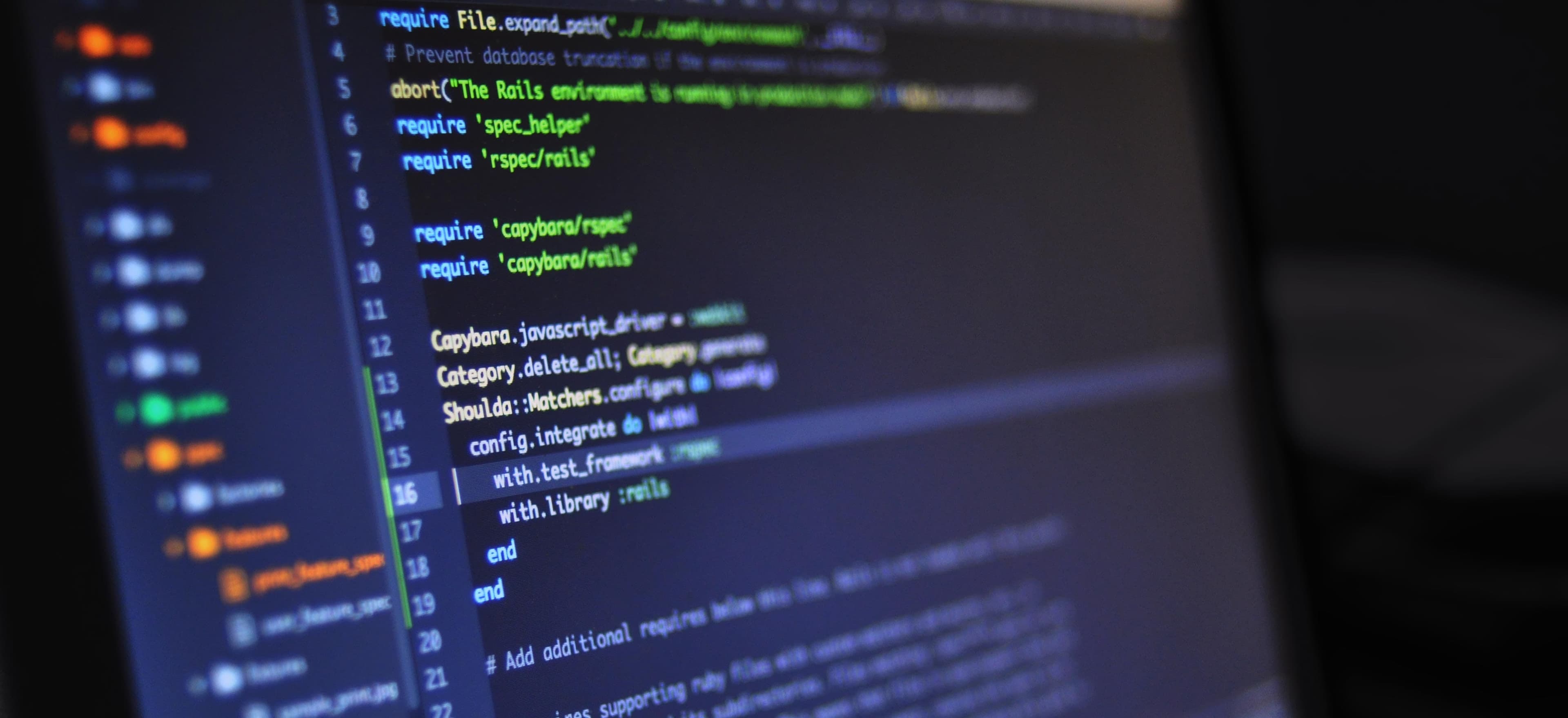
- Published on
Java Solutions for Handling Short Content Display Issues
In today’s fast-paced digital environment, the way we display content on our webpages can significantly impact user experience and engagement. Issues like short content not displaying appropriately can frustrate both users and developers alike. This article delves into Java-based solutions for handling such display issues, ensuring your webpage maintains an elegant and user-friendly appearance.
We will also link to a valuable resource titled Display Issues: Fixing Short Content on Your Webpage, which provides additional insights into this topic.
Understanding the Problem
When a webpage contains short content, it may lead to a layout that appears unbalanced or empty. This often results in users questioning the quality of your site. If the content area lacks sufficient height or width, it can make the page feel incomplete.
Short content display issues can often stem from:
- CSS styling errors
- Improper layout management
- Content generation problems
Java can assist in creating the necessary backend logic to address these display issues effectively. Below, we explore several Java solutions using common frameworks and libraries such as Spring Boot and Java Servlets.
Solution 1: Dynamic Content Height Adjustment
One effective approach to mitigating short content display issues is to dynamically adjust the height of content areas based on the content loaded. This involves using Java to calculate the required dimensions and apply them directly on the frontend.
Example: Spring Boot Backend
Here is a simple Spring Boot application that provides content dynamically. We'll use the @Controller
annotation to manage incoming requests:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class ContentController {
@GetMapping("/dynamicContent")
@ResponseBody
public String getDynamicContent() {
return "<div style='min-height: 100px; background-color: lightgray;'>"
+ "This is dynamic content!"
+ "</div>";
}
}
Why Use Dynamic Content Heights?
In the above example, we set a minimum height (min-height
) for the content area. This ensures that even if the content is short, the area won’t collapse. It's a small yet efficient way to maintain a well-structured layout, preventing the "empty box" syndrome that can detract from a user’s experience.
Solution 2: Flexbox for Responsive Layouts
If your content is frequently short, a more visually appealing approach involves using CSS Flexbox in tandem with Java to manage your display properties. Flexbox allows for greater control over alignment, justification, and spacing within your layout.
Example: Java Servlet with Flexbox Styles
Here’s how to implement a basic Flexbox layout in a Java Servlet:
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class FlexboxServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html");
response.getWriter().println("<style>"
+ ".container { display: flex; justify-content: center; align-items: center; min-height: 100vh; }"
+ ".box { padding: 20px; background-color: lightblue; }"
+ "</style>"
+ "<div class='container'>"
+ "<div class='box'>Short Content Here</div>"
+ "</div>");
}
}
Why Choose Flexbox?
Flexbox is beneficial because it inherently adapts to the available space. The min-height
property ensures that your content area stretches to a decent height even when the content itself is minimal. This technique of centering content ensures that your layout remains visually appealing and meets modern aesthetic standards.
Solution 3: Placeholder Content
In some cases, it may be worthwhile to include placeholder content when the main content is too brief. This allows continuity for the user and can help in maintaining interest.
Example: Java Backend with Placeholder Logic
Here is how you might implement this in a Java application:
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class PlaceholderContentController {
@GetMapping("/contentWithPlaceholder")
@ResponseBody
public String getContentWithPlaceholder(@ModelAttribute("content") String content) {
if (content.length() < 50) {
content += " ... [Placeholder content to enrich the experience]";
}
return "<div>" + content + "</div>";
}
}
Why Use Placeholder Text?
In the above example, we first check if the content length is below a specific threshold (in this case, 50 characters). If it is, we append placeholder text to provide context and balance to the user interface. This method keeps user engagement intact even with minimal content.
Final Considerations
Handling short content display issues on your webpage requires a mix of back-end and front-end strategies. By dynamically adjusting content heights, utilizing Flexbox for responsive layouts, and adding placeholder text when necessary, you can create a more engaging and visually appealing experience for your users.
For further details on addressing these issues, refer to the comprehensive insights available in the article Display Issues: Fixing Short Content on Your Webpage.
Adopting these techniques not only enhances the user experience but also keeps your applications robust and future-proof. With evolving design practices and standards, staying updated with modern web design principles is essential. Implement these solutions in your Java applications to ensure that content display is consistent, appealing, and user-friendly.