Java Patterns: Aligning Code with the Stars of Software Design
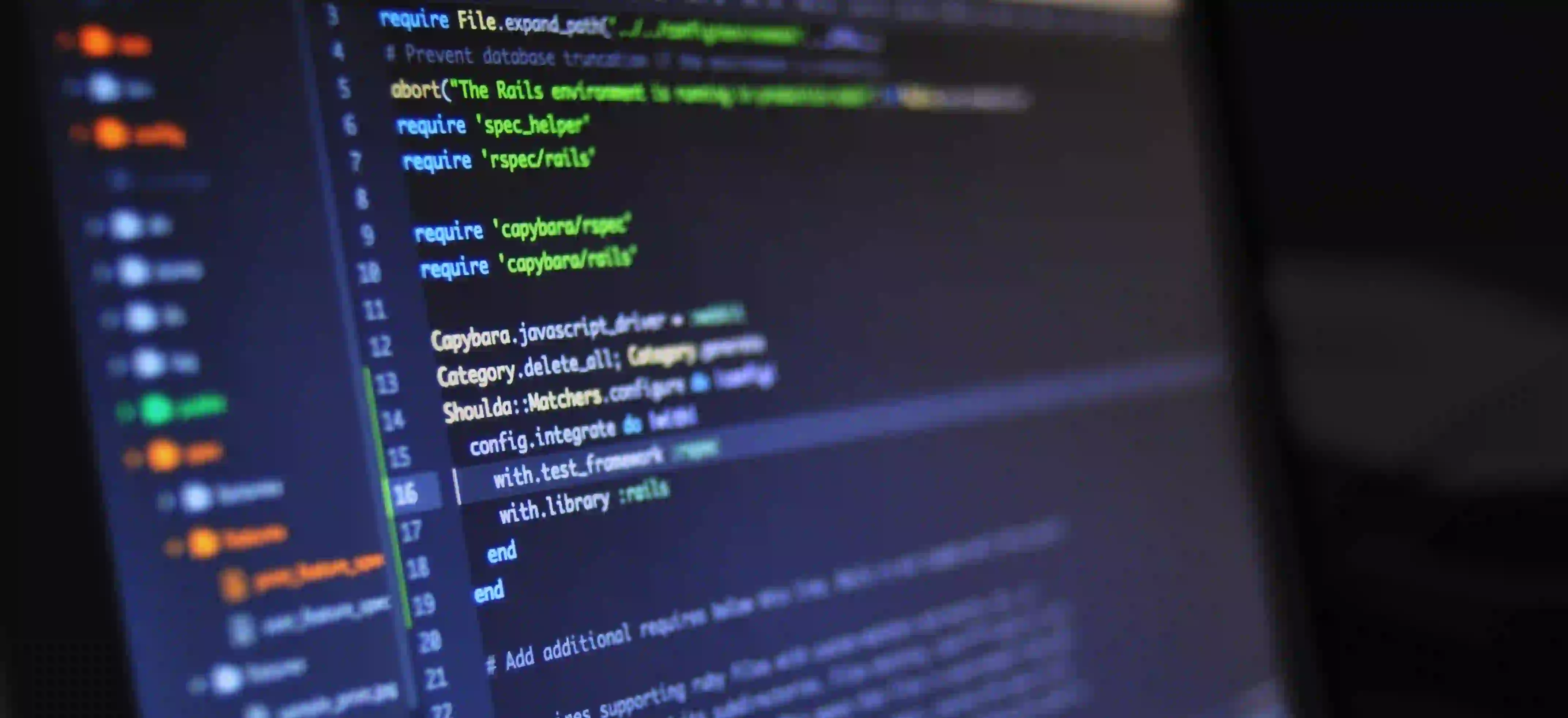
Java Patterns: Aligning Code with the Stars of Software Design
In any programming language, design patterns serve as blueprints for solving common problems. They allow developers to write cleaner, more efficient, and maintainable code. In Java, these patterns are not just tools; they are a philosophy influencing how we approach software development. In this post, we will explore some essential Java design patterns, connecting them metaphorically to the stars in the sky, just as the article "Astrologie: Entdecke die zeitlose Weisheit der Sterne" delivers timeless wisdom from the cosmos (source: https://auraglyphs.com/post/astrologie-entdecke-die-zeitlose-weisheit-der-sterne).
Understanding Design Patterns
Before we dive into specific patterns, it’s important to grasp what design patterns are. They can be seen as generalized solutions to recurring problems in software design. By employing these patterns, developers can avoid reinventing the wheel and instead build upon proven strategies.
The Three Categories of Design Patterns
- Creational Patterns: Deal with object creation mechanisms.
- Structural Patterns: Concerned with object composition.
- Behavioral Patterns: Focus on communication between objects.
Let’s explore some key patterns from each category and see how they align with the principles of effective software design.
Creational Patterns
Singleton Pattern
The Singleton pattern ensures that a class has only one instance while providing a global access point to that instance. This is particularly useful when you need to control access to some shared resource, like a configuration object or a connection pool.
Here’s how the Singleton pattern is implemented in Java:
public class Singleton {
private static Singleton instance;
private Singleton() {
// Constructor is private to prevent instantiation
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton(); // Lazy initialization
}
return instance;
}
}
Why Use the Singleton Pattern?
Using the Singleton pattern allows for controlled access to a particular resource, ensuring that there’s only one instance to manage. Lazy initialization also helps in conserving system resources.
Factory Method Pattern
The Factory Method pattern defines an interface for creating objects but allows subclasses to alter the type of objects that will be created. This is particularly useful when the exact type of the object may not be known until runtime.
Here’s an example:
abstract class Product {
public abstract void create();
}
class ConcreteProductA extends Product {
@Override
public void create() {
System.out.println("ConcreteProductA created.");
}
}
class ConcreteProductB extends Product {
@Override
public void create() {
System.out.println("ConcreteProductB created.");
}
}
abstract class Creator {
public abstract Product factoryMethod();
}
class ConcreteCreatorA extends Creator {
@Override
public Product factoryMethod() {
return new ConcreteProductA();
}
}
class ConcreteCreatorB extends Creator {
@Override
public Product factoryMethod() {
return new ConcreteProductB();
}
}
Why Use the Factory Method Pattern?
The Factory Method pattern allows for greater flexibility in code creation. The code can produce different products dynamically based on the specific context during runtime.
Structural Patterns
Adapter Pattern
The Adapter pattern allows the interface of an existing class to be used as another interface. This is incredibly useful for integrating disparate systems.
Here’s an example:
// Existing interface
interface OldSystem {
void specificRequest();
}
class OldSystemImpl implements OldSystem {
public void specificRequest() {
System.out.println("Specific request from the old system.");
}
}
// New target interface
interface NewSystem {
void request();
}
// Adapter class
class Adapter implements NewSystem {
private OldSystem oldSystem;
public Adapter(OldSystem oldSystem) {
this.oldSystem = oldSystem;
}
@Override
public void request() {
oldSystem.specificRequest(); // Adapt to new interface
}
}
Why Use the Adapter Pattern?
By using the Adapter pattern, you can integrate new components with old ones without modifying their code. This contributes to cleaner, modular architecture, just like celestial bodies maintain their order without affecting each other's paths.
Behavioral Patterns
Observer Pattern
The Observer pattern defines a one-to-many dependency between objects. When one object changes state, all its dependents are notified and updated automatically.
Here's an example of this pattern:
import java.util.ArrayList;
import java.util.List;
interface Observer {
void update(String message);
}
class ConcreteObserver implements Observer {
private String name;
public ConcreteObserver(String name) {
this.name = name;
}
@Override
public void update(String message) {
System.out.println(name + " received: " + message);
}
}
class Subject {
private List<Observer> observers = new ArrayList<>();
public void attach(Observer observer) {
observers.add(observer);
}
public void notifyObservers(String message) {
for (Observer observer : observers) {
observer.update(message);
}
}
}
Why Use the Observer Pattern?
This pattern promotes loose coupling, as the subject does not need to know anything about the observers. It allows the maintainability and scalability of systems, ensuring that they still align with the greater design objectives.
Wrapping Up
Utilizing Java design patterns not only helps in developing high-quality applications but also enables developers to unlock creativity in their solutions. By aligning our coding practices with these patterns, we can navigate the complexities of software design more effectively.
Just as astrologers study the stars to glean insights into life’s mysteries, Java developers must familiarize themselves with design patterns to achieve excellence in software engineering. By embracing the patterns we discussed, developers can ensure their code remains as steadfast and reliable as the constellations above.
For further insights into timeless philosophies beyond programming, check out "Astrologie: Entdecke die zeitlose Weisheit der Sterne" (auraglyphs.com/post/astrologie-entdecke-die-zeitlose-weisheit-der-sterne).
By understanding and implementing these patterns, we can code solutions that not only meet present requirements but also withstand the tests of time, much like the stars that have guided civilizations for millennia.