Mastering Java Error Handling: Avoiding Common Mistakes
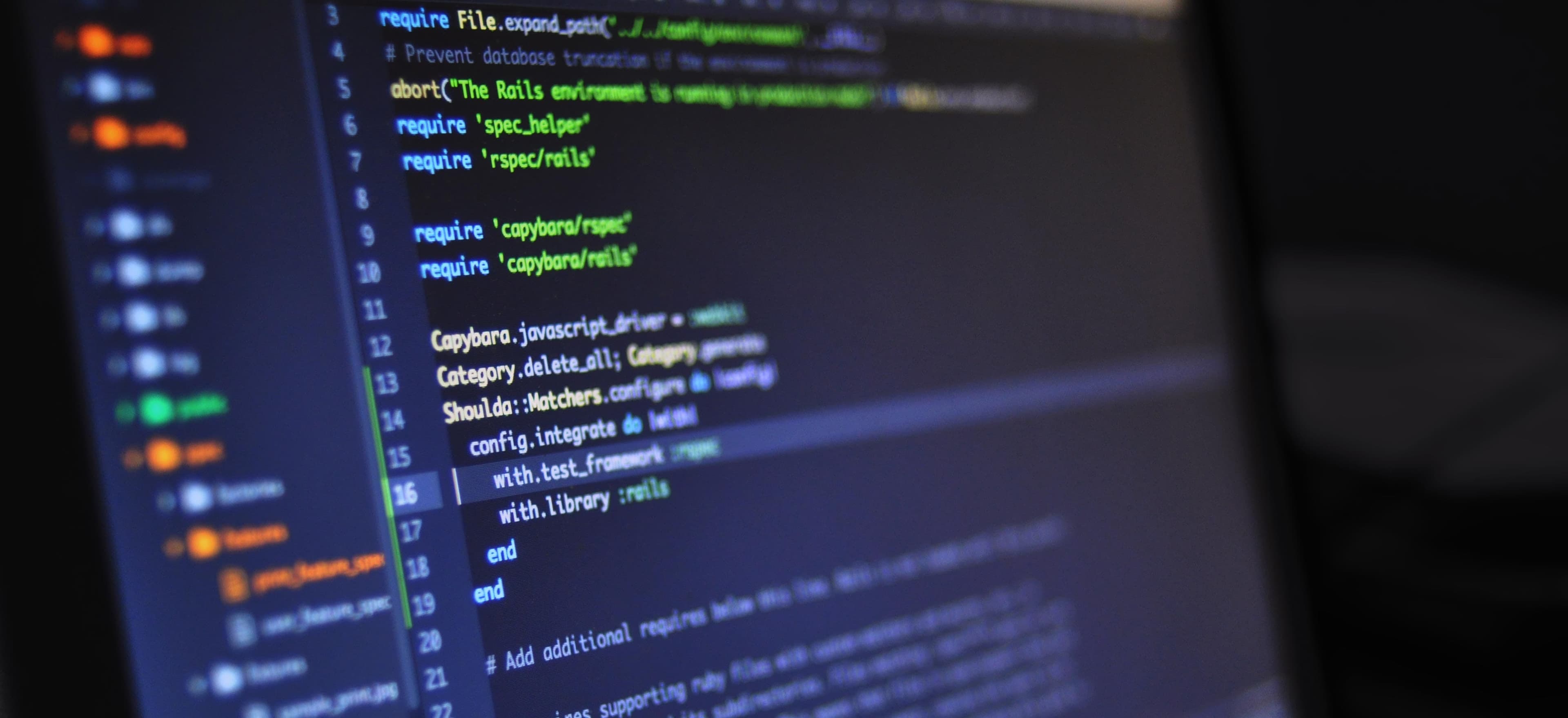
- Published on
Mastering Java Error Handling: Avoiding Common Mistakes
Error handling is an essential aspect of programming that can significantly impact the robustness and reliability of your Java applications. Just as managing errors effectively in Rust can often be a source of complexity, the same applies to Java, albeit through a different approach. Delving into this topic not only equips you with the tools necessary for seamless development but also ensures that your projects remain sustainable and maintainable.
In this article, we will explore common pitfalls in Java error handling, illustrate examples with code snippets, and discuss best practices to ensure that you master this critical aspect of Java programming. We'll also draw some parallels with Rust’s error handling as discussed in the article Navigating Rust's Error Handling: Common Pitfalls to Avoid.
Understanding Exceptions in Java
In Java, exceptions are special conditions that disrupt the normal flow of the program. Errors that occur during runtime, such as invalid input or file errors, are typically represented as exceptions. Here's a brief overview of the categories of exceptions in Java:
-
Checked Exceptions: These exceptions are checked at compile-time. For example, attempting to read from a file that does not exist can throw a
FileNotFoundException
. The programmer must handle these exceptions explicitly. -
Unchecked Exceptions: These exceptions are not checked at compile-time. They typically include programming errors such as
NullPointerException
orArithmeticException
. -
Errors: These are severe conditions that a reasonable application should not catch. For example,
OutOfMemoryError
is a type of error.
Basic Exception Handling Syntax
In Java, exceptions are handled using try-catch-finally
blocks. Here’s a simple example:
public class ExceptionExample {
public static void main(String[] args) {
try {
int result = divideNumbers(10, 0);
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Error: Cannot divide by zero.");
} finally {
System.out.println("Execution completed.");
}
}
public static int divideNumbers(int a, int b) {
return a / b; // This will throw ArithmeticException when b is zero.
}
}
Why this code matters: The try
block executes the code that may cause an exception, while the catch
block handles the specific exception type. The finally
block runs regardless of an exception being thrown, which is useful for resource cleanup (like closing files or connections).
Common Pitfalls in Java Error Handling
1. Catching Generic Exceptions
One significant mistake that many Java programmers make is catching generic exceptions. For example:
try {
// code that may throw an exception
} catch (Exception e) {
// handle exception
}
Pitfall: By catching Exception
, you may inadvertently catch exceptions that should not be handled at that level, such as OutOfMemoryError
or a StackOverflowError
. This can mask actual issues that need attention.
Best Practice: Always catch the most specific exception classes first. For instance, if you expect an IOException
, catch IOException
rather than Exception
.
2. Ignoring Exception Handling
Developers often overlook the importance of exception handling in certain scenarios. For example:
public void readFromFile(String filename) {
try {
// Assume readFile() may throw FileNotFoundException
readFile(filename);
} catch (FileNotFoundException e) {
// Logging the exception only
e.printStackTrace();
// Ignoring the issue entirely
}
}
Pitfall: Simply logging the exception without taking any additional action can lead to bugs and unexpected application behavior.
Best Practice: Instead of ignoring exceptions, consider rethrowing them or providing an alternative flow. This conveys the importance of the error to the calling methods.
3. Failing to Use Finally Blocks
Another omnipresent mistake arises from not managing cleanup effectively:
public void processFile(String filename) {
FileInputStream fis = null;
try {
fis = new FileInputStream(filename);
// Process the file
} catch (FileNotFoundException e) {
e.printStackTrace();
}
// fis will not be closed if an exception occurs before it's initialized
}
Pitfall: If an exception occurs after opening a resource but before closing it, leaks can occur, compromising system performance.
Best Practice: Always close resources in the finally
block, to guarantee that resources are cleaned up. In modern Java (Java 7 onward), use try-with-resources:
public void processFile(String filename) {
try (FileInputStream fis = new FileInputStream(filename)) {
// Process the file
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
Best Practices in Java Error Handling
- Use Custom Exceptions: When standard exceptions do not convey the specific error nature clearly, create custom exceptions to enhance error representation.
public class ResourceNotAvailableException extends Exception {
public ResourceNotAvailableException(String message) {
super(message);
}
}
Why Custom Exceptions Matter: They enable you to convey more meaningful messages tailored to the situation, assisting troubleshooting efforts.
- Log Exception Details: Use a structured logging framework to record exceptions adequately. This helps in diagnosing issues later on.
import java.util.logging.Level;
import java.util.logging.Logger;
public class Main {
private static final Logger logger = Logger.getLogger(Main.class.getName());
public static void main(String[] args) {
try {
// Some risky operation
} catch (Exception e) {
logger.log(Level.SEVERE, "An error occurred", e);
}
}
}
Importance of Logging: Proper logging can provide context when diagnosing failures, especially in production environments where users may not report issues directly.
- Fail Fast: When encountering an unexpected scenario, throw an exception immediately. This helps to catch misuse early.
public int calculateDiscount(int price) {
if (price < 0) {
throw new IllegalArgumentException("Price cannot be negative");
}
// Apply discount logic
}
Failing Fast Principle: Catching issues up front makes the system more predictable and easier to debug.
In Conclusion, Here is What Matters
In Java, mastering error handling is crucial for creating robust and maintainable applications. The key takeaways include avoiding the pitfalls of generic exception handling, not ignoring exceptions, ensuring proper resource management, and following best practices for logging and exception design.
As seen in the parallel discussion of Rust’s error handling in the article Navigating Rust's Error Handling: Common Pitfalls to Avoid, understanding and effectively managing errors is essential for any developer.
By implementing these best practices, you can elevate your Java programming to new heights, bolstering both the reliability of your applications and your reputation as a knowledgeable developer. Remember, effective error handling isn't just about preventing crashes—it's about creating a better experience for your users and yourself as a developer.
Now go ahead, implement these practices in your projects, and watch your Java applications thrive!
Checkout our other articles