Mastering Error Handling in Java: Avoiding Common Pitfalls
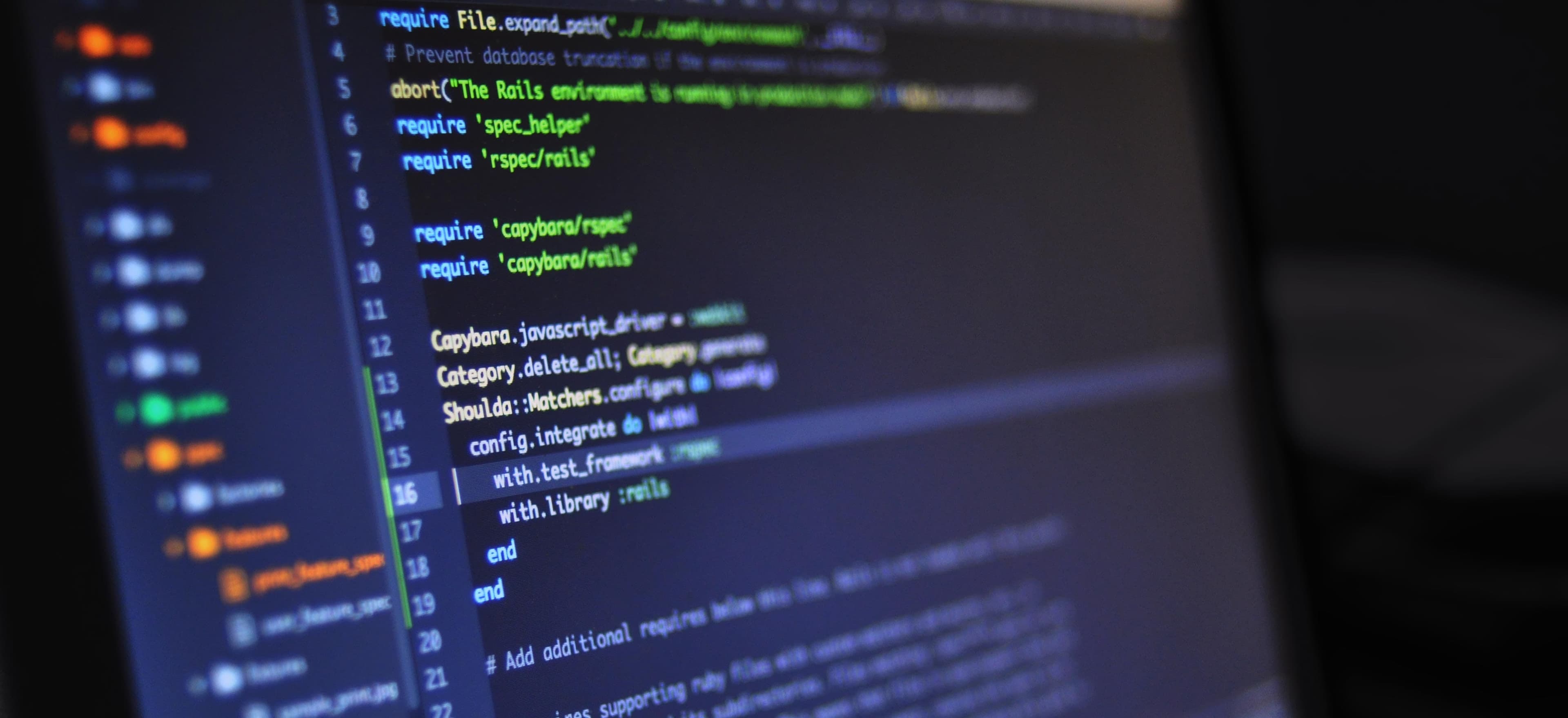
- Published on
Mastering Error Handling in Java: Avoiding Common Pitfalls
Error handling is an essential aspect of programming that can often be overlooked, especially when starting out with new languages. In Java, proper error handling not only ensures the robustness of your applications but also enhances user experience and debuggability. This article aims to take a deep dive into Java's error handling mechanisms, common pitfalls developers face, and how to navigate them effectively, paralleling some of the challenges faced in other languages, such as Rust, as outlined in the article "Navigating Rust's Error Handling: Common Pitfalls to Avoid" (tech-snags.com/articles/navigating-rust-error-handling-pitfalls).
Understanding Exceptions in Java
In Java, exceptions are categorized into two major types: checked exceptions and unchecked exceptions.
-
Checked Exceptions: These are exceptions that the compiler forces you to handle. This means that if you call a method that throws a checked exception, you must either catch it using a try-catch block or declare it in your method’s
throws
clause. -
Unchecked Exceptions: These include
RuntimeException
and its subclasses, such asNullPointerException
orArrayIndexOutOfBoundsException
. The compiler does not require you to catch these exceptions.
Example of Checked vs Unchecked Exceptions
public class ExceptionExample {
public static void checkedExceptionExample() throws Exception {
throw new Exception("This is a checked exception");
}
public static void uncheckedExceptionExample() {
throw new RuntimeException("This is an unchecked exception");
}
public static void main(String[] args) {
try {
checkedExceptionExample();
} catch (Exception e) {
System.out.println("Caught: " + e.getMessage());
}
try {
uncheckedExceptionExample();
} catch (RuntimeException e) {
System.out.println("Caught: " + e.getMessage());
}
}
}
Why It Matters
Understanding the difference between checked and unchecked exceptions helps in designing robust applications. Checked exceptions prompt the developer to anticipate potential failures and handle them, while unchecked exceptions can often indicate programming errors that should not be handled at runtime.
Common Pitfalls in Error Handling
-
Swallowing Exceptions: One of the most frequent mistakes developers make is catching an exception but doing nothing with it—commonly referred to as ‘swallowing’ the exception. This deprives you of crucial debugging information and makes it harder for you to diagnose issues in production.
try { // some code that may throw an exception } catch (Exception e) { // Swallowed exception }
Why It's Problematic: Swallowing exceptions can lead to silent failures where errors go unreported, causing confusion for users and other developers who may not understand the context.
-
Overly Broad Exception Handling: Another pitfall is catching all exceptions indiscriminately. For instance, catching
Exception
or evenThrowable
can lead to losing context about what went wrong and may obscure programming errors.try { // some code } catch (Exception e) { // Not ideal as this captures all exceptions, even those we might not want to handle here }
Best Practice: Catch specific exceptions and handle them accordingly, as this maintains clarity and focus on the actual issues that may arise.
-
Failing to Use
finally
: Thefinally
block is an essential part of the try-catch mechanism that can be used for cleanup operations, such as closing file streams or releasing resources. Omitting it can lead to resource leaks that degrade performance over time.public static void readFile(String path) { FileInputStream fis = null; try { fis = new FileInputStream(path); // Read the file... } catch (FileNotFoundException e) { System.out.println("File not found: " + e.getMessage()); } finally { if (fis != null) { try { fis.close(); } catch (IOException e) { System.out.println("Failed to close file: " + e.getMessage()); } } } }
Why Use
finally
: This ensures that critical cleanup code is executed regardless of whether an exception occurred, preventing resource leaks.
Best Practices for Error Handling in Java
To enhance your error handling practices, consider the following additional tips:
Use Custom Exceptions
Creating custom exception classes can help encapsulate specific error types unique to your domain, making your code clearer and better organized.
public class UserNotFoundException extends Exception {
public UserNotFoundException(String userId) {
super("User not found: " + userId);
}
}
Use Case: If you have a specific operation related to user management, using a UserNotFoundException
conveys more information about the context of the error than a generic exception would.
Log Exceptions
Implementing logging frameworks can provide valuable context when an error occurs, allowing you to diagnose issues more effectively. Logging platforms like SLF4J, Log4J, or Java's java.util.logging
offer versatile options for logging your exceptions.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ExceptionLogger {
private static final Logger logger = LoggerFactory.getLogger(ExceptionLogger.class);
public static void handleException(Exception e) {
logger.error("An error occurred: ", e);
}
}
Benefit: By logging exceptions, you can track issues over time, identify recurring problems, and improve your application's resilience.
Rethrow Exceptions
Sometimes, you want to catch an exception, perform some actions (like logging), and then rethrow it. This can help maintain the application continuity while alerting users of issues.
public void performOperation() {
try {
// code that might throw an exception
} catch (SpecificException e) {
// Handle and log the specific exception
throw new CustomRuntimeException("Operation failed", e);
}
}
Final Thoughts
Mastering error handling in Java is a nuanced yet essential skill for every developer. By avoiding common pitfalls, such as swallowing exceptions or using overly broad exception handling, you not only enhance the reliability of your software but also provide better maintainability and user experience.
While error handling in Java takes a different approach than languages like Rust, where methods of error handling can sometimes lead to complex pitfalls as discussed in "Navigating Rust's Error Handling: Common Pitfalls to Avoid" (tech-snags.com/articles/navigating-rust-error-handling-pitfalls), understanding Java’s model equips you with the tools needed to navigate the landscape of unexpected behaviors and failures effectively.
In summary, remembering the best practices such as using custom exceptions, implementing logging, effectively using try-catch-finally, and rethrowing exceptions can vastly improve your error handling approach. With these guidelines, you are well on your way to building robust, error-resilient Java applications.
For more resources on handling errors in various programming languages and understanding best practices, consider exploring Java’s documentation on Exception Handling or diving deeper into specific challenge areas in programming.
Checkout our other articles