Dealing with Issues in @Autowired Optional Dependencies
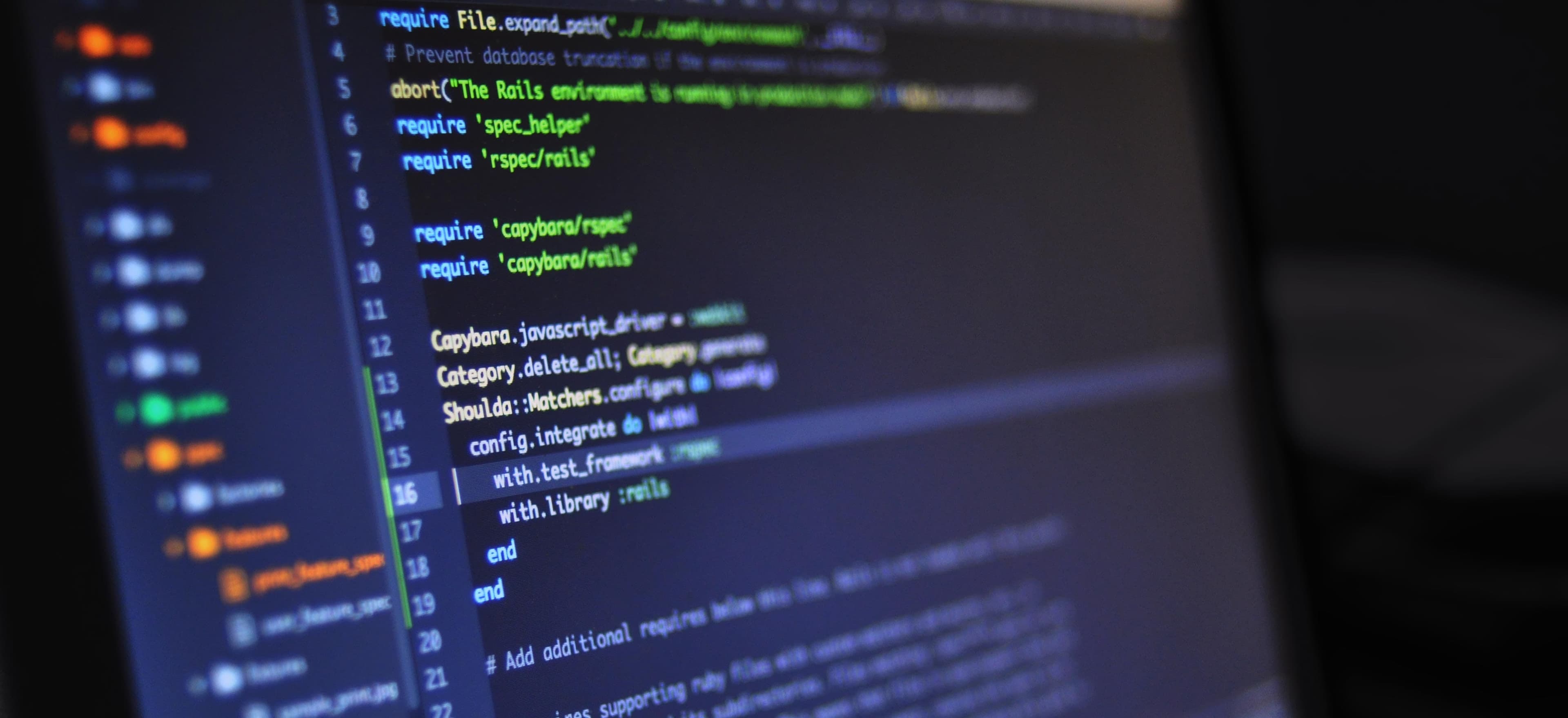
- Published on
Dealing with Issues in @Autowired Optional Dependencies in Spring Framework
When developing applications using the Spring Framework, dependency injection plays a pivotal role. One of the most powerful features Spring provides is the @Autowired
annotation to wire dependencies. However, there can be complexities when dealing with optional dependencies. In this blog post, we will explore how to handle optional dependencies correctly, the challenges you might encounter, and best practices.
What is @Autowired?
The @Autowired
annotation is used in Spring to automatically inject dependent beans. This feature reduces boilerplate code and enhances configuration management. Normally, when you annotate a field, constructor, or setter method with @Autowired
, Spring attempts to inject the corresponding bean. If there is no eligible bean found, a NoSuchBeanDefinitionException
is thrown.
The Challenge of Optional Dependencies
Sometimes, a service might not require a dependency to function properly. For example, a service implementation may be optional for certain business logic, and its absence should not cause application failure. This is where optional dependencies come into play.
Example Scenario
Consider an application that sends notifications. We may have a service that sends email notifications and another that sends SMS notifications. If SMS functionality is optional, failing to inject it should not lead to application startup failing.
A Simple Use Case
@Service
public class NotificationService {
private final SmsService smsService;
@Autowired(required = false)
public NotificationService(SmsService smsService) {
this.smsService = smsService;
}
public void sendNotification(String message) {
// Always send email
sendEmail(message);
// Send SMS only if SmsService is available
if (smsService != null) {
smsService.sendSms(message);
}
}
private void sendEmail(String message) {
// Logic to send email
System.out.println("Sending email: " + message);
}
}
Explanation of the Above Code
Here we declare our NotificationService
, which has an optional dependency on SmsService
. By specifying required = false
, we tell Spring not to throw an exception if SmsService
is unavailable. This allows our application to function without it.
Using Optional<T>
for Clarity
Another approach to handling optional dependencies is by using java.util.Optional
. This makes it clear in your code that the dependency might not be present.
Example with Optional
import java.util.Optional;
@Service
public class NotificationService {
private final Optional<SmsService> smsService;
@Autowired
public NotificationService(Optional<SmsService> smsService) {
this.smsService = smsService;
}
public void sendNotification(String message) {
sendEmail(message);
smsService.ifPresent(service -> service.sendSms(message));
}
private void sendEmail(String message) {
System.out.println("Sending email: " + message);
}
}
Key Takeaways
Using Optional<SmsService>
, we express intent clearly and promote better readability. The method ifPresent
succinctly checks if the smsService
is present and calls it, eliminating the need for a null check.
Challenges with @Autowired Optional Dependencies
While using @Autowired
with optional dependencies can simplify your code, it may lead to some challenges:
-
Hidden Dependencies: When dependencies are optional, it might obscure the understanding of the exact components required by your service, complicating maintainability.
-
Testing: It can become tricky to mock optional dependencies during unit testing. Ensure you account for both cases, where the dependency is available or not.
-
Configuration Management: As your application grows, managing optional dependencies may cause configuration clutter, requiring clear documentation.
-
Feature Flags: When dealing with optional features, consider if using feature flags would provide a more dynamic solution. This can enhance functionality without tying it to dependency injection.
Best Practices for Handling Optional Dependencies
Here are some best practices to follow when working with optional dependencies in Spring:
1. Use @Autowired(required=false)
This method is straightforward and commonly used for optional dependencies. However, always remember to handle null checks.
2. Employ Optional<T>
By using Optional
in your method parameters, you improve readability and communicate intent clearly. This pattern adheres to best practices in Java.
3. Documentation
Document your optional dependencies clearly in your code. This is crucial. Code that is inherently complex can benefit significantly from thorough documentation, particularly when dependencies are optional.
4. Favor Constructor Injection
Constructor injection is generally more preferred than field injection. It encourages immutability and easier testing scenarios. Therefore, opt for constructor injection when managing optional dependencies.
5. Keep Your Dependencies Clean
Avoid convoluted dependencies. Ensure your beans remain cohesive and manageable. Regular code reviews can help maintain these practices.
6. Unit Test Extensively
Unit test both scenarios: when the optional dependency exists and when it does not. Doing so will help you capture potential issues before they make it to production.
My Closing Thoughts on the Matter
In the Spring Framework, handling optional dependencies with @Autowired
provides flexibility and promotes cleaner code. However, it also introduces complexities that should be managed effectively. By using tools like Optional<T>
, following best practices, and documenting your code well, you ensure maintainability and readability.
Utilizing optional dependencies expands your design choices, allowing your application to evolve seamlessly over time. Make sure to leverage these tips to improve your Spring applications' robustness and clarity.
For further reading, check out the Spring Documentation and consider exploring design patterns that naturally accommodate optional dependencies.
By implementing these guidelines, you can face challenges with optional dependencies confidently and optimize your Spring applications for success. Happy coding!
Checkout our other articles