Overcoming Latency Issues in Terabyte-Size Java Queues
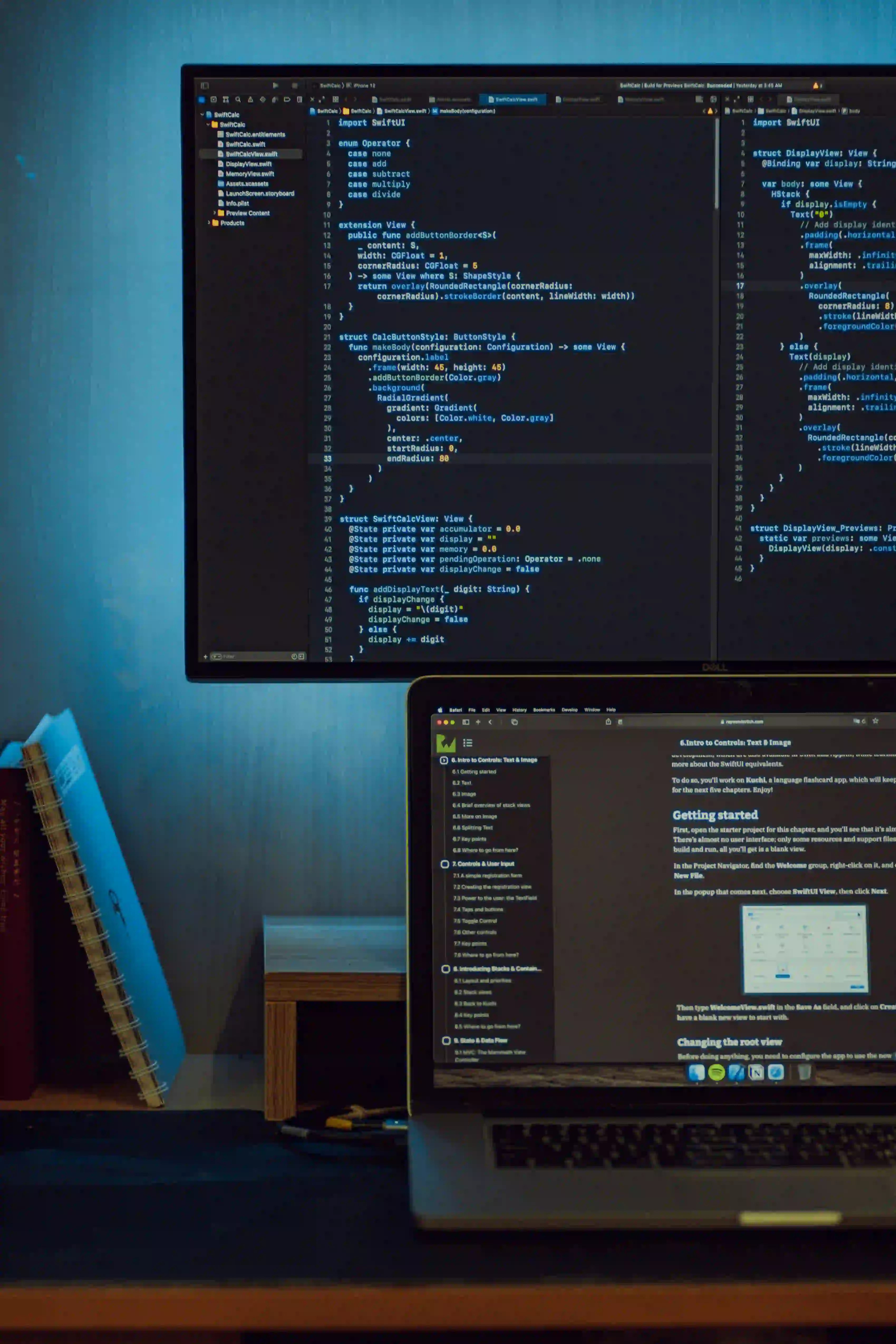
Overcoming Latency Issues in Terabyte-Size Java Queues
As modern applications grow in size and complexity, managing large data sets efficiently becomes critical. One common challenge developers face is latency in message queues, particularly when dealing with terabyte-sized queues in Java applications. Here, we'll explore strategies to mitigate latency issues, ensuring smooth performance and robust application behavior.
Understanding Java Queues
In Java, queues are commonly implemented using data structures like LinkedList
, ArrayDeque
, or concurrent implementations like ConcurrentLinkedQueue
. The choice of implementation heavily influences performance characteristics, especially under high load with large data volumes.
Why Latency Matters
Latency is the time it takes for data to travel from one point to another in a system. High latency in message queues can lead to:
- Reduced user experience: Long wait times degrade application performance.
- Increased system load: Slow consumption of messages may create backlogs.
- Poor scalability: Applications fail to handle growing data volumes efficiently.
Factors Contributing to Latency
- Queue Implementation: The underlying data structure can lead to significant performance differences.
- Garbage Collection: Large queues can exacerbate garbage collection pauses, slowing down applications.
- Thread Contention: High contention for shared resources can increase wait times in multi-threaded environments.
- Network Latency: When working with distributed systems, data transfer times can add significant delays.
Strategies to Overcome Latency
1. Choose the Right Queue Implementation
Selecting the appropriate queue implementation is fundamental. For high-throughput applications, consider using ConcurrentLinkedQueue
or specialized libraries like Disruptor. Here’s a comparison:
- LinkedList: Good for simple use cases but can lead to high garbage collection pauses.
- ArrayDeque: Offers better performance than LinkedList but is limited by memory constraints.
- ConcurrentLinkedQueue: Designed for high concurrency with non-blocking algorithms, minimizing latency.
Example: Using ConcurrentLinkedQueue
import java.util.concurrent.ConcurrentLinkedQueue;
public class MessageQueue {
private ConcurrentLinkedQueue<String> queue = new ConcurrentLinkedQueue<>();
public void enqueue(String message) {
queue.add(message);
}
public String dequeue() {
return queue.poll();
}
}
In this snippet, ConcurrentLinkedQueue
is used to avoid blocking threads when multiple consumers are dequeuing messages.
2. Optimize Garbage Collection
When dealing with large data sets, understanding Java's garbage collection (GC) can be key to reducing latency. Two primary techniques include:
- Tuning GC settings: Use the G1 Garbage Collector, which is designed for large heaps and minimizes pause times.
- Reducing object creation: Employ primitive types where possible to avoid object bloat.
Example: GC Tuning
To enable G1 GC, you can add the following flag when starting your Java application:
-XX:+UseG1GC -XX:MaxGCPauseMillis=200
These settings direct the JVM to prioritize minimizing GC pause times, which can significantly reduce latency during heavy load.
3. Implement Backpressure
Backpressure is a design pattern where consumers signal to producers about their capacity to handle more data. Implementing backpressure helps prevent overwhelming consumers, thus reducing latency.
Example: Simple Backpressure Implementation
public class BackPressureQueue {
private final int capacity;
private final ConcurrentLinkedQueue<String> queue = new ConcurrentLinkedQueue<>();
public BackPressureQueue(int capacity) {
this.capacity = capacity;
}
public synchronized void enqueue(String message) throws InterruptedException {
while(queue.size() >= capacity) {
wait(); // Wait until there is space in the queue.
}
queue.add(message);
notifyAll(); // Notify consumers that a new message is added.
}
public synchronized String dequeue() throws InterruptedException {
while(queue.isEmpty()) {
wait(); // Wait until there is a message to consume.
}
String message = queue.poll();
notifyAll(); // Notify producers that there is space in the queue.
return message;
}
}
In this example, the queue uses synchronization to manage access, ensuring that producers wait when the queue is full and consumers wait when it's empty, thus avoiding spikes in latency.
4. Use Partitioning and Sharding
For extreme scenarios involving terabyte-sized queues, partitioning data can significantly reduce both processing time and latency. By distributing messages across multiple queues, you can process them in parallel.
Example: Partitioning Messages
If you are handling various types of messages, consider dividing them into queues based on their type or priority:
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentLinkedQueue;
public class PartitionedQueue {
private final ConcurrentHashMap<String, ConcurrentLinkedQueue<String>> partitions = new ConcurrentHashMap<>();
public void enqueue(String partitionKey, String message) {
partitions.computeIfAbsent(partitionKey, k -> new ConcurrentLinkedQueue<>()).add(message);
}
public String dequeue(String partitionKey) {
ConcurrentLinkedQueue<String> queue = partitions.get(partitionKey);
return (queue != null) ? queue.poll() : null;
}
}
This approach allows individual partitions to scale independently and improves throughput, alleviating latency from any single queue.
5. Utilize Asynchronous Processing
Asynchronously processing messages can prevent blocking behaviors. Using Java's CompletableFuture
or reactive programming libraries like Project Reactor can make a difference.
Example: CompletableFuture for Async Processing
import java.util.concurrent.CompletableFuture;
public class AsyncProcessing {
public void processMessages(String message) {
CompletableFuture.runAsync(() -> {
// Simulate processing
System.out.println("Processing message: " + message);
// Perform actual processing here
});
}
}
Using CompletableFuture
, we can process messages without holding up the main thread, significantly decreasing perceived latency for users.
In Conclusion, Here is What Matters
Overcoming latency issues in terabyte-size Java queues is challenging but achievable. By selecting appropriate queue implementations, optimizing garbage collection, implementing backpressure, using partitioning, and leveraging asynchronous processing, developers can enhance application performance significantly.
These strategies not only improve throughput but also ensure that applications can scale effectively under high load, providing a better experience for users. For deep dives into message processing frameworks, consider exploring Apache Kafka or RabbitMQ to see how they integrate these principles into their design.
By applying the aforementioned methods, you will gain the confidence to tackle latency issues in even the most demanding applications, ensuring efficient and reliable data processing.