Mastering Null Values in ArrayList: A Guide to AddAll
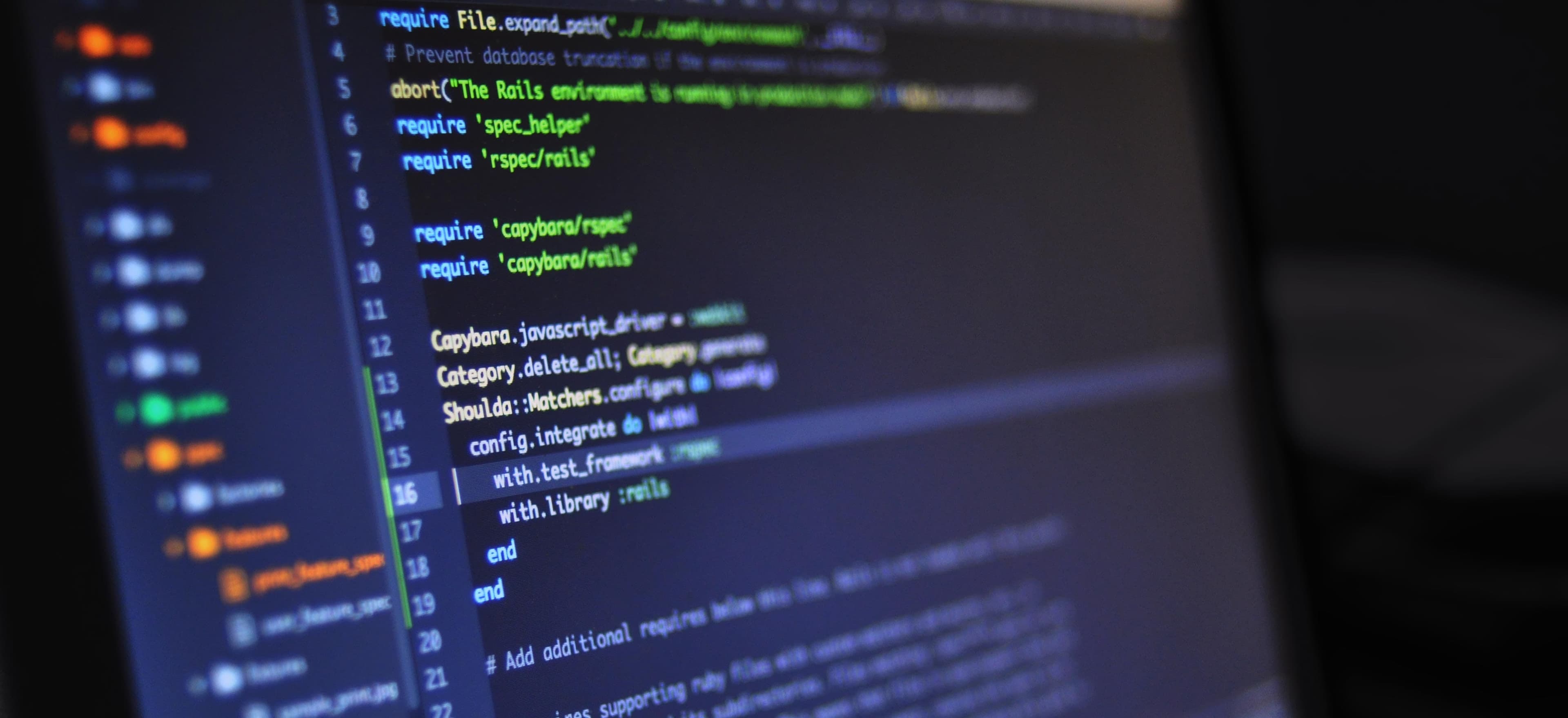
- Published on
Mastering Null Values in ArrayList: A Guide to addAll
Java's ArrayList
is one of the most widely used data structures due to its flexibility and dynamic sizing properties. However, handling null
values within an ArrayList
can be a source of confusion and error for many developers. This post aims to clarify how to efficiently use addAll()
with null
elements in ArrayList
.
We'll delve into:
- Understanding how
ArrayList
handlesnull
values. - Exploring the
addAll()
method. - Illustrating practical examples.
- Best practices to manage
null
values.
Let's start by understanding ArrayList
and its capability to handle null
values.
What is an ArrayList?
An ArrayList
is part of Java's Collection Framework and implements the List
interface. It allows us to store elements dynamically, meaning you can add or remove elements without worrying about the underlying array size:
ArrayList<String> strings = new ArrayList<>();
strings.add("Hello");
strings.add("World");
Handling Null Values in ArrayList
Java's ArrayList
can hold null
values just like any other object type. This can be useful for denoting absence, placeholders, or for representing optional values.
Here is a simple illustration:
ArrayList<String> strings = new ArrayList<>();
strings.add(null); // Adding a null value
strings.add("Java");
strings.add(null); // Adding another null
You can retrieve null
from the ArrayList
without any issue:
System.out.println(strings.get(0)); // Outputs: null
Understanding the addAll()
Method
The addAll(Collection<? extends E> c)
method is used to add all the elements from a specified collection to the ArrayList
. This might seem simple, but it becomes notably interesting when the collection being added contains null
values.
Syntax of addAll Method
The syntax for addAll()
looks like this:
boolean addAll(Collection<? extends E> c)
Here is what you need to know:
- It returns
true
if theArrayList
was modified as a result of the call. - It throws a
NullPointerException
if the specified collection isnull
.
Example of Using addAll With Null Values
Let’s look at a practical example that illustrates how addAll()
can handle collections with null
values.
import java.util.ArrayList;
import java.util.Arrays;
public class NullInArrayList {
public static void main(String[] args) {
ArrayList<String> firstList = new ArrayList<>(Arrays.asList("Apple", null, "Banana"));
ArrayList<String> secondList = new ArrayList<>(Arrays.asList("Cherry", null, "Date"));
// Merging two ArrayLists using addAll
firstList.addAll(secondList);
System.out.println("Combined List: " + firstList);
}
}
Output
Combined List: [Apple, null, Banana, Cherry, null, Date]
Why Use addAll?
- Efficiency: The
addAll()
method is more efficient than adding elements one by one in a loop. - Clarity: It improves code readability and reduces boilerplate code.
Handling Null Pointer Exception
It’s critical to remember that passing a null
collection to the addAll()
method will result in a NullPointerException
. For example:
ArrayList<String> list = new ArrayList<>();
list.add("Test");
list.addAll(null); // Throws NullPointerException
To prevent this, always ensure your collection is not null
before calling addAll()
. A simple conditional check can handle this:
if (collectionToAdd != null) {
list.addAll(collectionToAdd);
}
Best Practices for Managing Nulls in ArrayLists
When working with null
values in ArrayLists
, here are some best practices to keep in mind:
-
Use Optional: Consider using
Optional
instead ofnull
. This forces you to handle the absence of a value explicitly and reduces errors.Optional<String> value = Optional.ofNullable(getValue());
You can learn more about
Optional
in the official Java documentation. -
Clean Up Nulls: If your logic requires the removal of
null
values, you can filter the list using streams:ArrayList<String> cleanedList = firstList.stream() .filter(Objects::nonNull) .collect(Collectors.toCollection(ArrayList::new));
-
Be Consistent: Avoid mixing
null
values with actual data when possible. A clear approach enhances code maintainability. -
Document Assumptions: Always document the expected behavior in your code concerning
null
values. This helps other developers understand the code better, reducing bugs and misunderstandings.
Final Considerations
Handling null
values in Java's ArrayList
is essential for efficient data management. Understanding how to use the addAll()
method correctly allows you to work seamlessly with collections, while best practices ensure your code remains clean and easy to maintain.
If you want to deepen your knowledge about collections in Java, consider reading the Java Collections Framework Guide. By mastering these concepts, you pave the way to becoming a more effective Java developer.
Happy coding!