Overcoming Common Challenges in Netty WebSocket Servers
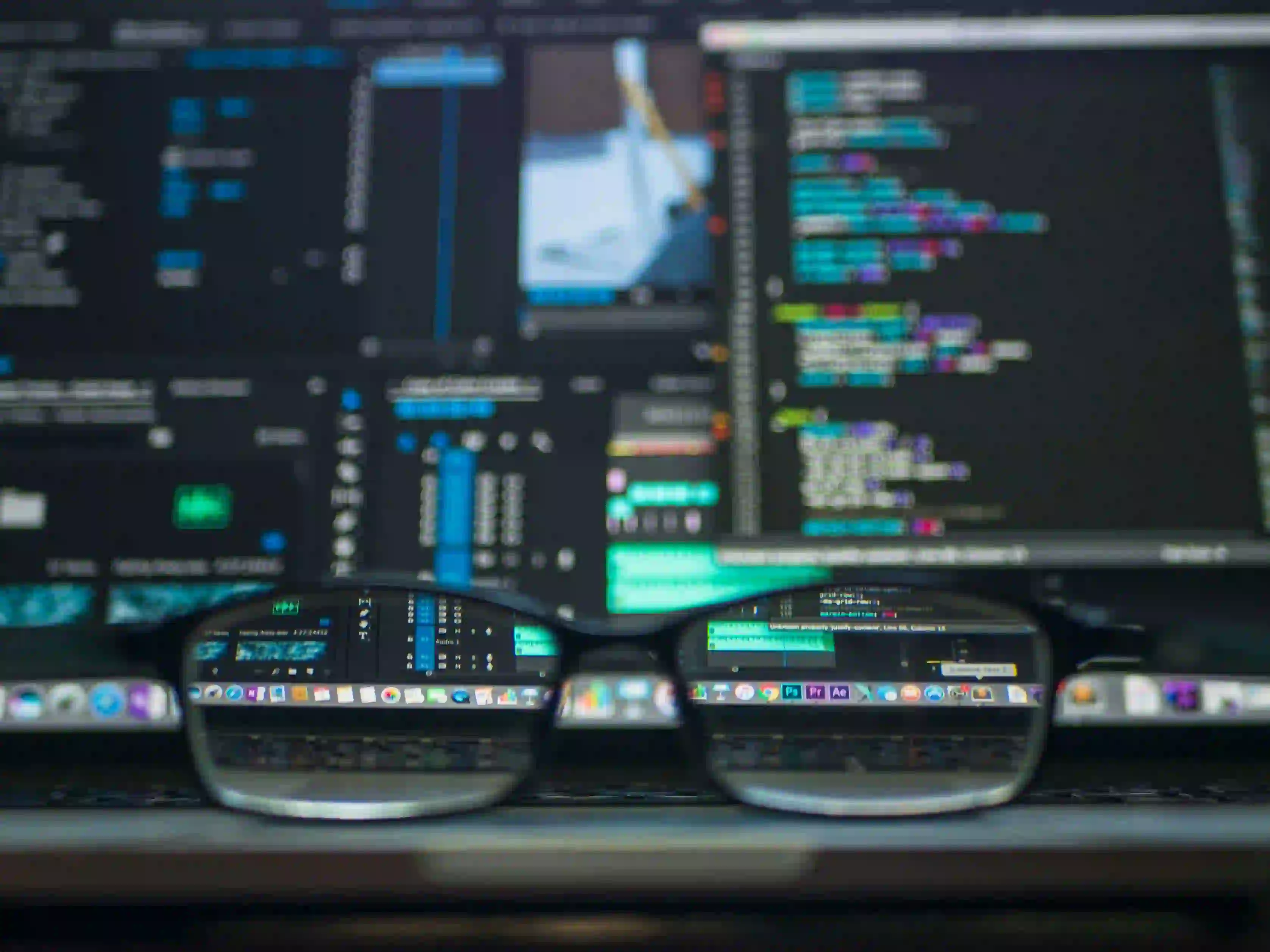
Overcoming Common Challenges in Netty WebSocket Servers
WebSocket has gained immense popularity as a protocol that provides full-duplex communication channels over a single TCP connection. When building robust WebSocket servers in Java, the Netty framework comes to the forefront with its ability to handle concurrent connections efficiently. However, developing a WebSocket server using Netty does not come without its challenges. This blog post delves into some common hurdles and offers practical solutions to overcome them.
Table of Contents
- Understanding WebSocket and Netty
- Common Challenges in Developing WebSocket Servers
- Best Practices for Developing Netty WebSocket Servers
- Conclusion
Understanding WebSocket and Netty
WebSocket is an advanced communication protocol that enables interactive communication sessions between a client (usually a browser) and a server. This protocol is particularly useful for applications that require constant updates, such as chat applications or real-time notifications.
Netty, on the other hand, is an asynchronous event-driven networking framework that simplifies network programming in Java. It’s lightweight, fast, and highly customizable, making it an excellent choice for building WebSocket servers.
For more information on WebSocket, you can check out the MDN Web Docs.
Common Challenges in Developing WebSocket Servers
Challenge 1: Handling Concurrent Connections
One of the main challenges with WebSocket servers is managing multiple client connections concurrently. Each connection represents a unique session that must be maintained independently.
Solution: Netty provides an event-driven architecture that can efficiently handle numerous concurrent connections. Using the ChannelPipeline, you can create a handler stack that processes events as they occur.
Here’s an example of how you can set up a basic Netty WebSocket server:
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class WebSocketServer {
private final int port;
public WebSocketServer(int port) {
this.port = port;
}
public void start() throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
// Add your channel handlers here
}
});
ChannelFuture f = b.bind(port).sync();
f.channel().closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
new WebSocketServer(8080).start();
}
}
In this code:
- EventLoopGroup: Used to handle the concurrency. The boss group accepts incoming connections, while the worker group processes the actual connections.
- ServerBootstrap: This is the starting point to set up your server. You can configure it with channel types and handlers.
Challenge 2: Managing Message Framing
WebSocket communication involves the fragmentation of messages. This fragmentation can cause issues if not managed properly, as clients may send large messages that need to be processed in parts.
Solution: Netty’s WebSocketFrame
offers various types of frames, such as TextFrame, BinaryFrame, etc. Using these frames allows you to manage message sizes easily.
import io.netty.handler.codec.http.websocketx.TextWebSocketFrame;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
public class TextWebSocketFrameHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
// Handling incoming message
if (msg instanceof TextWebSocketFrame) {
String request = ((TextWebSocketFrame) msg).text();
// Process the message accordingly
ctx.channel().writeAndFlush(new TextWebSocketFrame("Received message: " + request));
}
}
}
In this example:
- The TextWebSocketFrame class allows you to efficiently handle text data sent between the server and the client.
- Each message received can be processed and responded to, ensuring that fragmented messages are dealt with effectively.
Challenge 3: Dealing with Network Latency
Network latency can hinder real-time communication, especially in scenarios where timely data transfer is critical. High latency can lead to delays in message delivery.
Solution: Implementing proper backpressure and flow control mechanisms within your Netty handlers helps mitigate this challenge.
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
try {
// Process the incoming message
} catch (Exception e) {
ctx.close(); // Closing connections for any processing error
}
}
With this code:
- Exceptions during message processing will automatically trigger a channel closure, which can help manage server load during peak times.
- This enhances response times as problematic connections can be quickly identified and handled.
Challenge 4: Security Considerations
In an age where cyber threats are prevalent, securing your WebSocket server is crucial. Vulnerabilities such as Cross-Site WebSocket Hijacking and denial-of-service attacks can arise if proper security measures are not enforced.
Solution: Use HTTPS and enable authentication/authorization mechanisms.
import javax.net.ssl.SSLContext;
import io.netty.handler.ssl.SslContextBuilder;
SSLContext sslContext = SslContextBuilder.forServer(new File(privateKeyPath), new File(certChainPath)).build();
By implementing SSL:
- You encrypt the data transmitted between the client and the server.
- This ensures that unauthorized entities cannot observe or manipulate data in transit.
You can also consider using a token-based authentication system to verify users before allowing them to interact with the WebSocket server.
Best Practices for Developing Netty WebSocket Servers
-
Graceful Shutdowns: Ensure that your server can gracefully shut down connections using hooks to close existing sessions.
-
Load Balancing: Implement load balancing in your architecture to efficiently distribute client connections among multiple server instances.
-
Monitoring: Use tools like JMX (Java Management Extensions) to monitor your server's performance metrics and improve scalability.
-
Testing: Always conduct load testing using tools like Apache JMeter to identify potential bottlenecks.
-
Keep Dependencies Updated: Regularly check for updates to the Netty framework to apply the latest performance and security enhancements.
The Last Word
Developing a Netty WebSocket server involves overcoming common challenges such as managing concurrent connections, message framing, network latency, and security concerns. By implementing best practices and employing the solutions highlighted in this post, you can build a robust WebSocket server that meets the demands of real-time communication.
As WebSocket technology continues to evolve, it’s crucial to stay updated with the latest practices. For more resources and information on network programming and Netty, consider exploring the Netty official documentation.
Happy coding, and may your connections remain open!