Why Beginners Struggle with Akka Actors Explained
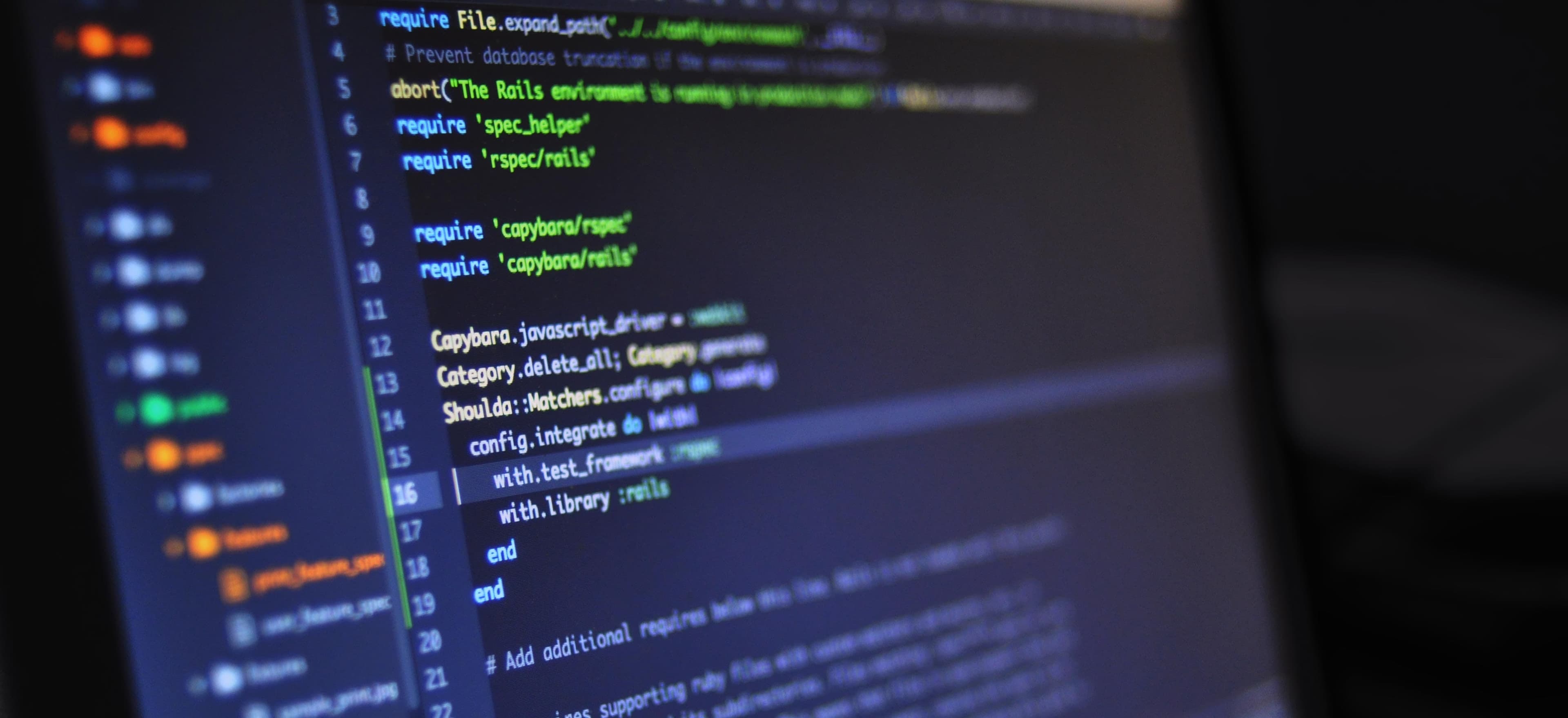
- Published on
Why Beginners Struggle with Akka Actors Explained
When embarking on the journey of learning Akka, a powerful toolkit for building concurrent, distributed, and fault-tolerant applications on the JVM, many beginners find themselves wrestling with the actors model. In this article, we'll explore the core aspects of Akka actors and address common hurdles that new developers face. Through an engaging blend of theory and practical code examples, we aim to demystify the actor model and help streamline your learning experience.
Understanding Akka Actors
At its heart, Akka implements the actor model, which helps manage state and behavior in a concurrent environment. Each actor is an independent unit that can receive messages, process them, and send messages to other actors, all while encapsulating its state. This decoupling greatly simplifies the challenges typically faced in multi-threaded programming.
Why Beginners Struggle
Beginners often struggle with Akka actors for several reasons:
-
Conceptual Shift: Transitioning from traditional object-oriented programming to the actor model requires a mental shift. Developers must learn to think in terms of message passing instead of method calling.
-
Asynchronous Nature: Akka is inherently asynchronous, making it challenging for newcomers used to synchronous programming models. Messages sent between actors do not guarantee immediate processing, leading to potential confusion.
-
Complexity in State Management: Managing state in an actor-based system can be complex. Beginners may be unsure of how to maintain data consistency when multiple actors interact.
-
Error Handling: Akka actors can fail independently, which introduces new patterns for error handling unfamiliar to many developers. Understanding the supervision strategy and fault tolerance is crucial but can be daunting.
Getting Started with Akka Actors
Before diving into practical challenges, let's establish a basic understanding of how Akka actors work. Below is a simple example of how to create and interact with actors.
Example: A Basic Akka Actor
import akka.actor.AbstractActor;
import akka.actor.ActorRef;
import akka.actor.ActorSystem;
import akka.actor.Props;
import akka.actor.ReceiveTimeout;
public class HelloWorld {
public static class Greeter extends AbstractActor {
@Override
public Receive createReceive() {
return receiveBuilder()
.match(String.class, this::onReceiveMessage)
.build();
}
private void onReceiveMessage(String message) {
System.out.println("Hello, " + message + "!");
}
}
public static void main(String[] args) {
final ActorSystem system = ActorSystem.create("HelloSystem");
final ActorRef greeter = system.actorOf(Props.create(Greeter.class), "greeter");
greeter.tell("World", ActorRef.noSender());
system.terminate();
}
}
Breaking Down the Code
-
Creating an Actor Class: The
Greeter
class extendsAbstractActor
, making it an actor. ThecreateReceive()
method specifies how the actor responds to messages. This is where the shift from method calls to message receipt becomes apparent. -
Matching Messages: The
match
method is used to define how the actor will handle different incoming message types. In this case, it handlesString
messages. -
Sending Messages: In the
main
method, we create an actor system and instantiate theGreeter
actor. Usingtell
, we send a message. ThenoSender()
signifies that there is no need to get a reply. This highlights the asynchronous messaging nature of Akka.
Understanding the Actor Lifecycle
Actors do not exist in isolation; they interact within their lifecycle, which typically includes creation, waiting, processing, and termination. Understanding these stages is crucial for beginners to effectively utilize actors.
Common Pitfalls in Actor Communication
A common mistake among beginners is mismanaging actor references, leading to confusion and runtime errors. Here are some tips to mitigate this:
-
Use of ActorRef: Whenever you create an actor, ensure that you store its
ActorRef
properly for communication purposes. -
Avoid Blocking Calls: Never block the main thread while waiting for responses. This is counterproductive in an asynchronous model and can easily lead to performance issues.
Patterns for Managing State
As mentioned earlier, state management within actors requires a different approach. Here's a simple pattern to illustrate state encapsulation in an actor.
Example: Counter Actor
import akka.actor.AbstractActor;
import akka.actor.ActorRef;
import akka.actor.ActorSystem;
import akka.actor.Props;
public class Counter {
public static class CounterActor extends AbstractActor {
private int count = 0;
@Override
public Receive createReceive() {
return receiveBuilder()
.matchEquals("increment", msg -> incrementCount())
.matchEquals("getCount", msg -> getCurrentCount())
.build();
}
private void incrementCount() {
count++;
}
private void getCurrentCount() {
System.out.println("Current Count: " + count);
}
}
public static void main(String[] args) {
final ActorSystem system = ActorSystem.create("CounterSystem");
final ActorRef counter = system.actorOf(Props.create(CounterActor.class), "counter");
counter.tell("increment", ActorRef.noSender());
counter.tell("increment", ActorRef.noSender());
counter.tell("getCount", ActorRef.noSender());
system.terminate();
}
}
Why This Pattern Works
In this example, the CounterActor
encapsulates its state (count
). The actor only modifies the state within the incrementCount
method. It handles two types of messages: one to increment the count and one to retrieve the current count. This clear segregation of concerns simplifies the actor's responsibilities.
Error Handling in Akka
Error handling is a pivotal aspect of building resilient actor systems. Akka embraces the "let it crash" philosophy, encouraging developers to focus on supervision strategies rather than extensive try-catch blocks.
Supervision Strategies
-
Restart: The actor is restarted if it encounters an error, regaining a fresh state.
-
Stop: The actor stops processing and is removed from the system.
-
Resume: The actor continues where it left off but may not apply the last state update, allowing for recovery without complete restart.
Developers often underutilize supervision strategies, leading to complicated error handling. Being deliberate about the behavior of actors in case of failure will significantly improve application stability.
In Conclusion, Here is What Matters
Learning Akka and its actor model can indeed be a challenging journey for beginners. The key challenges often arise from the paradigm shift towards message-driven communication, asynchronous processing, complex state management, and effective error handling.
Understanding these challenges, as well as embracing practical patterns and supervision strategies, can drastically enhance your ability to work with Akka actors. To delve deeper into the actor model, consider checking out the Akka Documentation and exploring community-driven Akka Samples.
As with any new technology, perseverance, and practice will turn confusion into clarity. Take the time to play with examples, engage with the community, and soon you will master the intricacies of Akka actors. Happy coding!
Checkout our other articles