Efficiently Replace Elements in a List with Java 8 Streams
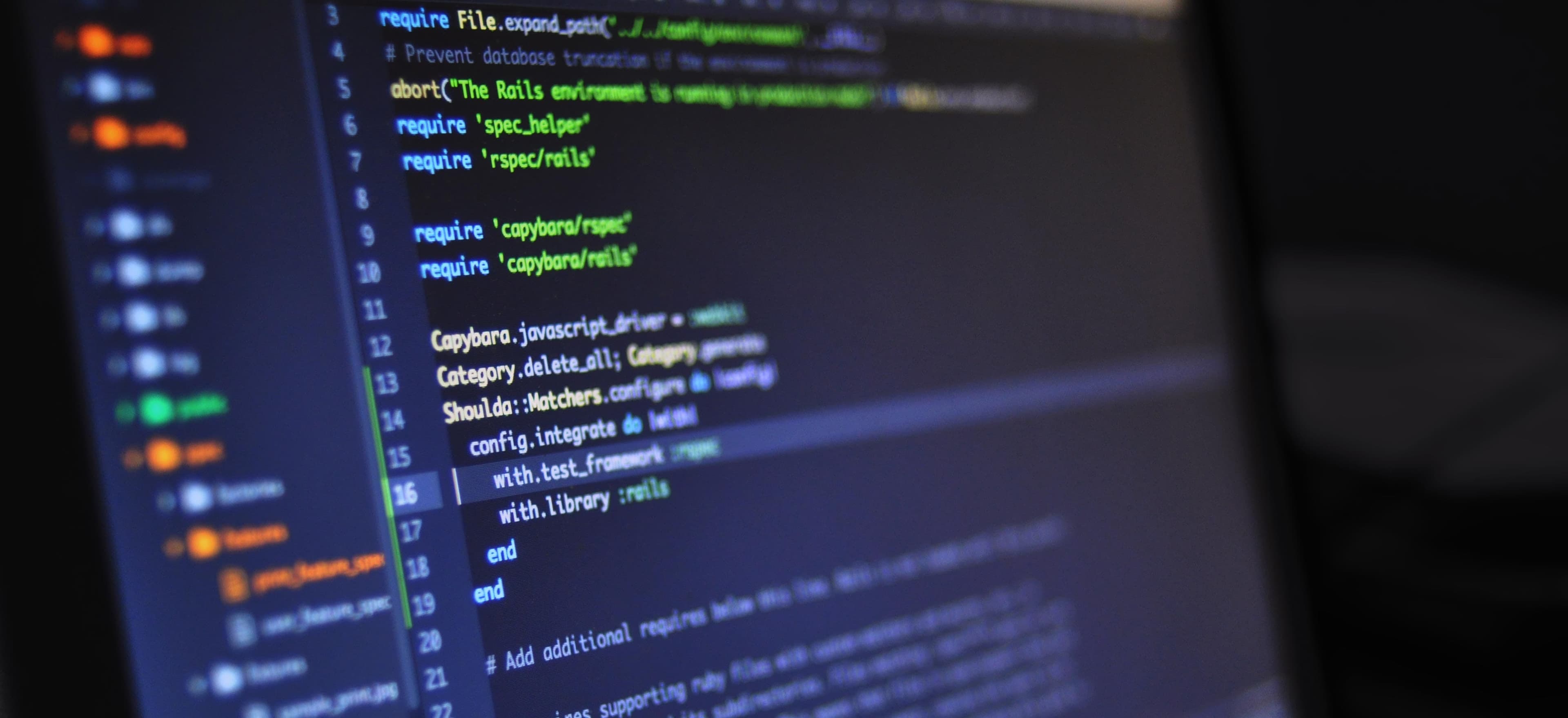
- Published on
Efficiently Replace Elements in a List with Java 8 Streams
Java 8 introduced the Stream API, which revolutionized how we handle collections. With Streams, you can perform operations like filtering, mapping, and reducing with ease and elegance. This blog post focuses on one specific operation: replacing elements in a list using Java 8 Streams.
Understanding the Use Case
Before diving into the code, let's clarify why you might want to replace elements in a list. Suppose you have a list of customer status values, and you want to update any status marked as "inactive" to "active". This scenario is quite common in applications where state management is essential.
Setting Up Your Environment
To work with Java 8 Streams, ensure you have Java 8 or higher installed. You can check your Java version by running:
java -version
If you need to set up a new Java project, consider using an IDE like IntelliJ IDEA or Eclipse, which simplifies the development process.
Creating a Sample List
Let’s create a simple list of customer statuses as our starting point. Here’s how you can initialize a list in Java:
import java.util.Arrays;
import java.util.List;
public class CustomerStatusUpdater {
public static void main(String[] args) {
List<String> statuses = Arrays.asList("active", "inactive", "pending", "inactive", "active");
System.out.println("Original statuses: " + statuses);
}
}
Explanation
- The
Arrays.asList
method is used to create a fixed-size list. - The
main
method serves as our entry point for testing the code.
Replacing Elements using Streams
You can replace elements in the list by streaming through it, applying a transformation, and collecting the results. Here’s how to do it:
import java.util.List;
import java.util.stream.Collectors;
public class CustomerStatusUpdater {
public static void main(String[] args) {
List<String> statuses = Arrays.asList("active", "inactive", "pending", "inactive", "active");
List<String> updatedStatuses = statuses.stream()
.map(status -> "inactive".equals(status) ? "active" : status)
.collect(Collectors.toList());
System.out.println("Updated statuses: " + updatedStatuses);
}
}
Explanation
- Stream Creation:
statuses.stream()
converts the list into a stream, allowing for functional-style operations. - Mapping: The
map
function applies a transformation where "inactive" is replaced with "active". This uses a ternary conditional operator for brevity. - Collecting Results: Finally,
collect(Collectors.toList())
gathers the transformed elements into a new list.
Why Use Streams?
Using streams makes your code more readable and maintainable. Instead of using loops and conditionals, you express your intent more directly. Additionally, streams can be parallelized easily in larger datasets, potentially improving performance.
Handling More Complex Scenarios
Suppose we want to replace multiple statuses at once, such as converting "inactive" to "active" and "pending" to "under review". Here’s how you could accomplish this:
import java.util.List;
import java.util.stream.Collectors;
public class CustomerStatusUpdater {
public static void main(String[] args) {
List<String> statuses = Arrays.asList("active", "inactive", "pending", "inactive", "active");
List<String> updatedStatuses = statuses.stream()
.map(status -> {
if ("inactive".equals(status)) {
return "active";
} else if ("pending".equals(status)) {
return "under review";
}
return status;
})
.collect(Collectors.toList());
System.out.println("Updated statuses: " + updatedStatuses);
}
}
Explanation
- Conditional Logic: The
map
function now contains a broader conditional structure to handle multiple replacements cleanly. This illustrates the flexibility of streams in dealing with more complex requirements.
Performance Considerations
While streams provide a high level of convenience and readability, performance can be a consideration, especially with larger datasets. The overhead of creating streams and collecting results may not be ideal in all scenarios.
Benchmarking Streams vs. Traditional Loops
If you are curious about the performance implications, you can set up a simple benchmark against a traditional for
loop:
import java.util.List;
import java.util.stream.Collectors;
public class CustomerStatusUpdater {
public static void main(String[] args) {
List<String> statuses = //... (assume this is initialized with many elements)
// Using streams
long startTime = System.nanoTime();
List<String> streamResult = statuses.stream()
.map(status -> "inactive".equals(status) ? "active" : status)
.collect(Collectors.toList());
long durationStream = System.nanoTime() - startTime;
// Using a traditional loop
startTime = System.nanoTime();
List<String> loopResult = new ArrayList<>();
for (String status : statuses) {
if ("inactive".equals(status)) {
loopResult.add("active");
} else {
loopResult.add(status);
}
}
long durationLoop = System.nanoTime() - startTime;
System.out.println("Stream Duration: " + durationStream + " ns");
System.out.println("Loop Duration: " + durationLoop + " ns");
}
}
The Last Word of Performance Testing
Ideally, you should validate the performance of both approaches in the context of your specific application to make informed decisions based on the size of your datasets and the complexity of your transformations.
The Last Word
Using Java 8 Streams for replacing elements in a list can lead to simpler and more readable code. You can efficiently handle single or multiple replacements while maintaining a functional programming style. As indicated throughout this blog post, evaluate the performance of discrete operations in the context of larger applications.
If you're eager to explore more about Java Streams and functional programming, check out Java 8 Official Documentation.
By continuously experimenting and applying this knowledge, you will become proficient in Java Stream operations, enabling you to write cleaner and more maintainable code in your applications.
Checkout our other articles