Common Clustering Pitfalls in Quartz Scheduler for JEE
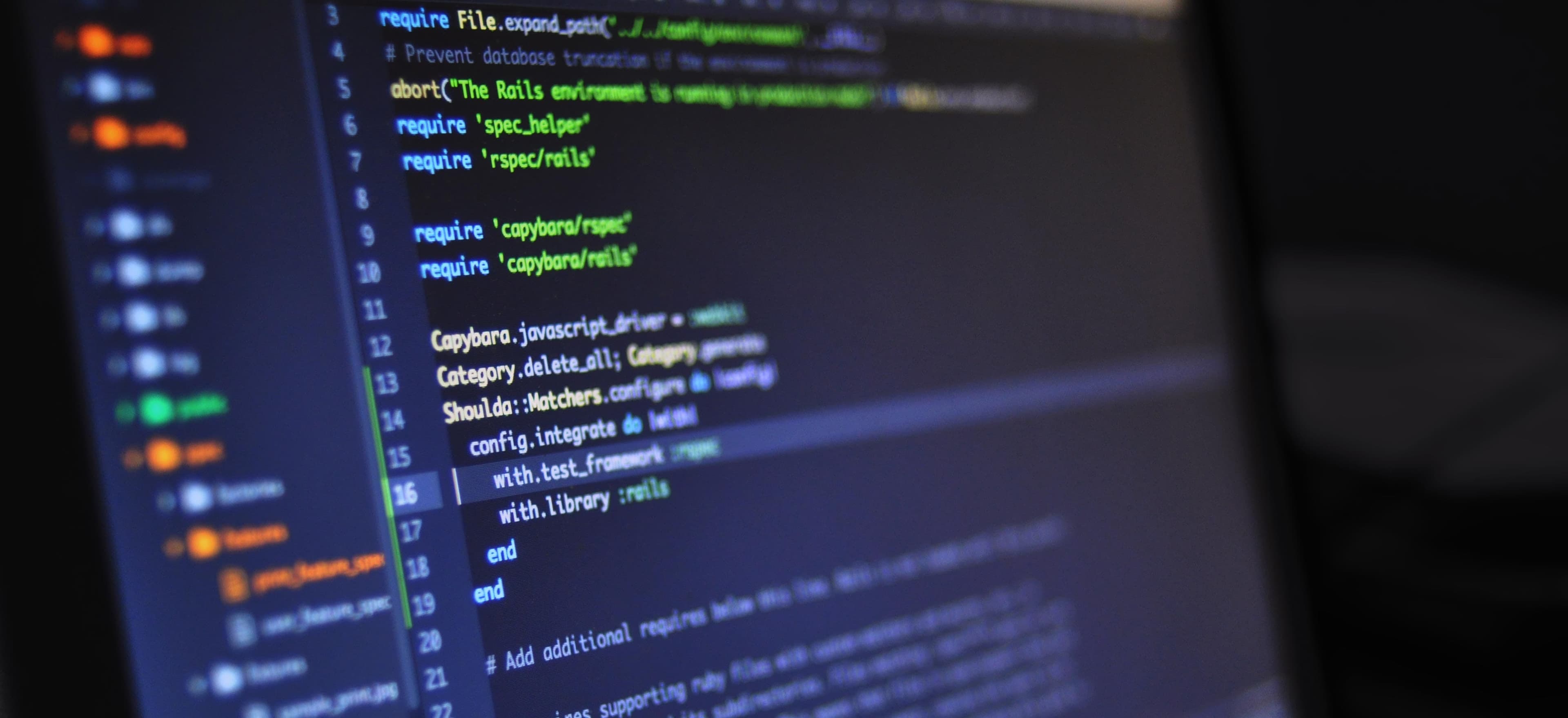
- Published on
Common Clustering Pitfalls in Quartz Scheduler for JEE
Quartz Scheduler is one of the most widely used job scheduling libraries for Java. It provides a simple and elegant way to run scheduled tasks in applications. However, when implementing Quartz in a clustered environment, developers may run into several pitfalls that can hinder application performance or reliability. This post will discuss these common issues and how to overcome them to ensure a robust and efficient job scheduling system.
Understanding Quartz Clustering
Before diving into the pitfalls, it's essential to understand the key concepts of Quartz Scheduler's clustering feature. Clustering in Quartz allows multiple instances of an application to work together to execute jobs in a distributed fashion. This helps improve fault tolerance and load balancing.
In a clustered setup, all the nodes share a common database where they store job details. The Quartz Scheduler handles job execution, ensuring that each job is executed only once across the cluster.
Basic Setup
Here’s a simplified example of configuring Quartz for clustering in your Java EE application:
<property name="org.quartz.scheduler.instanceName" value="MyClusteredScheduler" />
<property name="org.quartz.scheduler.instanceId" value="AUTO" />
<property name="org.quartz.jobStore.class" value="org.quartz.impl.jdbcjobstore.JobStoreTX" />
<property name="org.quartz.jobStore.isClustered" value="true" />
<property name="org.quartz.jobStore.dataSource" value="myDS" />
<property name="org.quartz.dataSource.myDS.driver" value="com.mysql.jdbc.Driver" />
<property name="org.quartz.dataSource.myDS.URL" value="jdbc:mysql://localhost:3306/quartz" />
<property name="org.quartz.dataSource.myDS.user" value="root" />
<property name="org.quartz.dataSource.myDS.password" value="password" />
<property name="org.quartz.dataSource.myDS.maxConnections" value="5" />
Common Pitfalls
Now, let's discuss some common pitfalls that developers encounter while configuring Quartz in a clustered environment.
1. Inadequate Database Configuration
Why It Matters: In a clustered setup, multiple Quartz instances will connect to the same database. If the database is not tuned appropriately, it can lead to performance bottlenecks.
Solution: Ensure the database can handle multiple connections concurrently. Increasing the connection pool size and optimizing queries related to Quartz can lead to improved performance.
<property name="org.quartz.dataSource.myDS.maxConnections" value="10" />
2. Job Misconfigurations
Why It Matters: Jobs that are not configured correctly can cause failures in job execution across clusters. This can include not setting the correct job store properties or misnaming jobs.
Solution: Always ensure job details such as name, group, and triggers are appropriately defined. Here’s a sample job creation:
JobDetail jobDetail = JobBuilder.newJob(MyJob.class)
.withIdentity("myJob", "group1")
.build();
Trigger trigger = TriggerBuilder.newTrigger()
.withIdentity("myTrigger", "group1")
.startNow()
.withSchedule(SimpleScheduleBuilder.simpleSchedule()
.withIntervalInSeconds(10)
.repeatForever())
.build();
Scheduler scheduler = StdSchedulerFactory.getDefaultScheduler();
scheduler.scheduleJob(jobDetail, trigger);
This ensures that Quartz correctly identifies and executes the job.
3. Ignoring Job Misfires
Why It Matters: Job misfires occur when a job cannot start at the scheduled time. This can be the result of numerous factors, including system overload or downtime.
Solution: Implement a robust misfire strategy for your triggers. For example:
Trigger trigger = TriggerBuilder.newTrigger()
.withIdentity("myTrigger", "group1")
.startNow()
.withSchedule(SimpleScheduleBuilder.simpleSchedule()
.withIntervalInSeconds(10)
.repeatForever()
.withMisfireHandlingInstructionNextWithRemainingCount())
.build();
Incorporating misfire instructions allows you to configure how Quartz should handle missed executions.
4. Poor Error Handling
Why It Matters: Each job may encounter exceptions or errors that could cause job execution to fail. If not handled properly, this can lead to jobs not executing as expected.
Solution: Use the JobListener
and TriggerListener
to monitor and handle job executions effectively.
public class MyJobListener implements JobListener {
@Override
public String getName() {
return "MyJobListener";
}
@Override
public void jobToBeExecuted(JobExecutionContext context) {
// Logic before job execution
}
@Override
public void jobExecutionVetoed(JobExecutionContext context) {
// Logic if job execution is vetoed
}
@Override
public void jobWasExecuted(JobExecutionContext context, JobExecutionException jobException) {
// Logic after job execution
if (jobException != null) {
System.err.println("Job failed: " + jobException.getMessage());
}
}
}
This will help maintain an effective logging strategy and ensure that jobs are retried or escalated as necessary.
5. Neglecting Cluster Instance Communication
Why It Matters: In a clustered environment, instances must communicate efficiently. Failing to configure clustering parameters can lead to poor load balancing.
Solution: Ensure that all Quartz instances share the same configuration and database settings. Regular health checks between nodes can help identify communication issues.
Final Considerations
Quartz Scheduler is a powerful tool for managing background jobs in Java EE applications. However, when deploying it in a clustered environment, developers must be mindful of various pitfalls that can affect performance and reliability.
By following the recommendations outlined above, you can avoid common mistakes and ensure your scheduling system is robust and effective. For a deeper dive into Quartz, check out the official documentation.
If you have encountered any pitfalls while using Quartz in your applications, please feel free to share your experiences and solutions in the comments below.
Checkout our other articles