Mastering Java 8 Streams: Common Pitfalls to Avoid
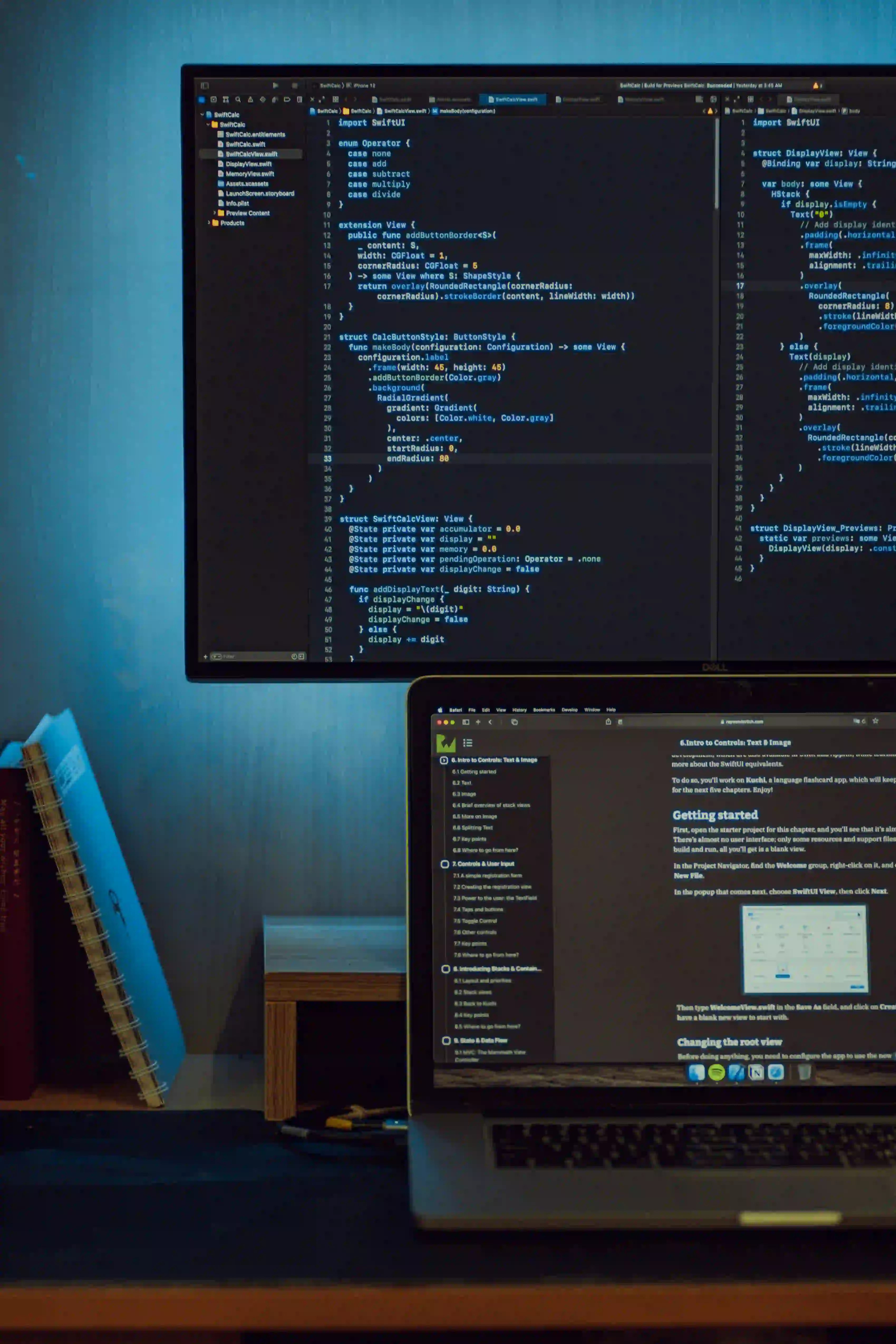
Mastering Java 8 Streams: Common Pitfalls to Avoid
Java 8 introduced a powerful new abstraction for working with collections: Streams. This approach revolutionized how developers manipulate data, making operations more readable and expressive. Although Streams can significantly improve code quality and efficiency, they also come with their pitfalls. In this blog post, we'll explore common mistakes developers make when using Java 8 Streams and how to avoid them.
Understanding Java Streams
Before delving into the pitfalls, let's outline what a Stream is. A Stream is a sequence of data that can be processed in a functional style. It allows operations such as filtering, mapping, and reducing through an intuitive API.
Example Code Snippet
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Using Stream to filter names beginning with 'A'
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
In the example above, we convert the List
of names into a Stream to filter entries beginning with the letter "A". The results are collected back into a List. This approach enhances readability and conciseness.
Common Pitfalls
1. Not Understanding Lazy vs. Eager Evaluation
One common mistake is not distinguishing between lazy and eager evaluation. Streams are lazily evaluated, meaning that operations do not occur until a terminal operation is invoked.
Example Code Snippet
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// This line does not initiate any operations
Stream<String> nameStream = names.stream().filter(name -> name.length() > 3);
// Terminal operation
List<String> longNames = nameStream.collect(Collectors.toList());
In this case, the filter operation is not performed until we call collect()
. Understanding this concept helps in debugging performance issues. If you expect an operation to execute immediately, you might face unexpected behavior.
2. Modifying the Source Collection
Streams do not operate on the source directly. Attempting to modify a source collection while processing can lead to ConcurrentModificationException
.
Example Code Snippet
List<String> names = new ArrayList<>(Arrays.asList("Alice", "Bob", "Charlie", "David"));
// This will throw ConcurrentModificationException
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(name -> names.remove(name));
To solve this problem, make a copy of the original list or use removeIf()
instead:
List<String> namesToRemove = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
names.removeAll(namesToRemove);
3. Using Stateful Lambda Expressions
Stateful Lambdas can lead to unpredictable behavior and performance issues, especially when they modify shared data. This occurs when each invocation of the lambda relies on mutable data from its outer scope.
Example Code Snippet
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
Set<String> nameSet = new HashSet<>();
// Risky stateful operation
names.stream()
.filter(nameSet::add)
.forEach(System.out::println);
Use stateless lambdas whenever possible. Instead, consider filtering by using elements that do not depend on outer variables.
4. Confusion Between Terminal and Intermediate Operations
Another pitfall arises from misunderstanding the difference between terminal and intermediate operations. Intermediate operations (such as filter
or map
) return a new Stream, while terminal operations (like collect
, forEach
) produce a result or side-effect.
This understanding is crucial, as forgetting to use a terminal operation means your Stream pipeline will not run.
Example Code Snippet
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Forgetting terminal operation
Stream<String> longNameStream = names.stream().filter(name -> name.length() > 3);
// This does not execute anything
// Correct usage with a terminal operation
List<String> longNames = longNameStream.collect(Collectors.toList());
5. Overusing Streams for Simple Operations
Not every situation requires the use of Streams. For simple operations, looping through conventional structures can be more readable and expressive. Using Streams could introduce unnecessary complexity, particularly for developers not familiar with functional programming concepts.
Example Code Snippet
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Simple for loop instead of Stream
for (String name : names) {
if (name.length() > 3) {
System.out.println(name);
}
}
Choose the right tool for the job. Use Streams when operations are complex or when working with larger data sets.
6. Not Handling Null Safely
A common oversight with Streams is failing to handle null values. If a Stream contains nulls and you attempt to execute operations like map
, you will encounter a NullPointerException
.
Example Code Snippet
List<String> names = Arrays.asList("Alice", null, "Charlie", "David");
// Will throw NullPointerException
names.stream()
.map(String::toUpperCase)
.forEach(System.out::println);
To avoid these issues, you can filter out nulls before processing:
names.stream()
.filter(Objects::nonNull)
.map(String::toUpperCase)
.forEach(System.out::println);
Final Considerations
Java 8 Streams offer powerful tools to manipulate collections seamlessly. However, to leverage their full potential, it’s essential to be aware of common pitfalls. Understanding the distinctions between lazy and eager evaluation, avoiding modifications of source collections during processing, utilizing stateless lambdas, and recognizing when to use simple loops instead of Streams can make your Java applications more robust and maintainable.
For a deep dive into Java 8 Streams, consider checking out the Oracle Java Tutorials and the Java Platform SE 8 Documentation. Experiment with Streams in your next project and enjoy the elegance they bring to your code!
If you found this article helpful, feel free to share it with your colleagues or on social media. Happy coding!