Overcoming Challenges in Legacy Integration Testing with Spock
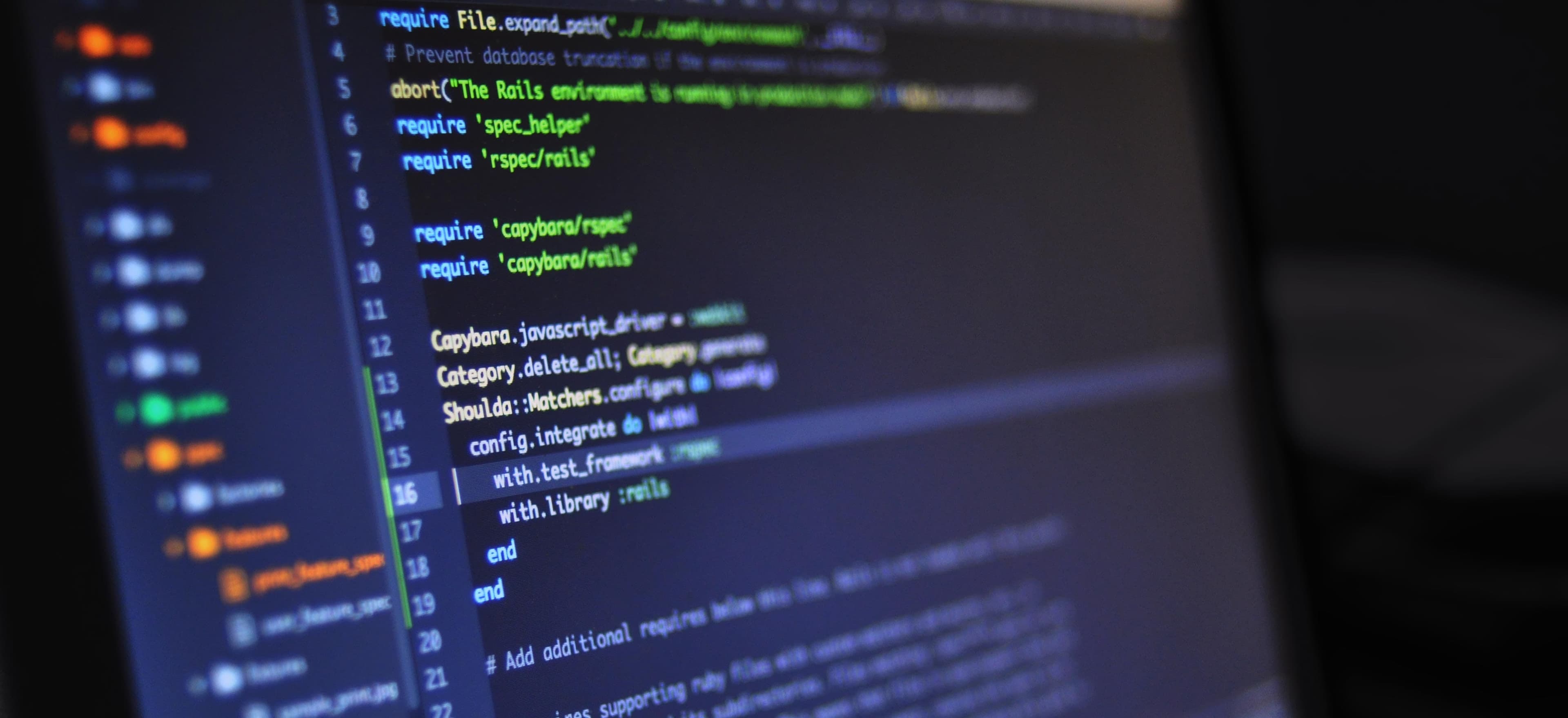
- Published on
Overcoming Challenges in Legacy Integration Testing with Spock
In the ever-evolving landscape of software development, legacy systems often pose significant challenges, especially when it comes to integration testing. Legacy codebases may not have been designed with testing in mind, leading to complications down the road as developers strive to maintain and enhance existing applications. Fortunately, tools like Spock Framework provide innovative solutions that can help simplify and streamline the testing process.
In this blog post, we will explore the various challenges associated with legacy integration testing and demonstrate how Spock can be leveraged to overcome these hurdles effectively.
Understanding Legacy Integration Testing
Legacy systems are software applications that are outdated but still in use. These systems often feature:
- Antiquated programming languages
- Lack of documentation
- A mix of old and newer code
- Dependencies that are difficult to manage
Challenges Faced in Legacy Integration Testing
-
Complexity of Code: Legacy systems are often intricate, with tightly coupled components that make it hard to determine the interaction points during testing.
-
Lack of Documentation: Without adequate documentation, understanding the intended behavior of legacy code becomes extremely challenging.
-
Inconsistent Environments: Legacy systems may run on outdated hardware or within peculiar configurations that are difficult to replicate in testing environments.
-
Slow Feedback Loops: The process of running tests on legacy systems can be painstakingly slow, leading to delayed response times during development.
-
Incompatibility with Modern Tools: Legacy code may not be compatible with modern testing tools and frameworks, necessitating custom solutions.
The Benefits of Using Spock Framework
Spock is a testing and specification framework for Java and Groovy applications. Here’s why Spock shines when it comes to legacy system testing:
-
Groovy-Based Syntax: Spock’s expressive syntax emphasizes readability, making it easier to write and understand tests.
-
Data Driven Testing: Spock promotes data-driven testing through parameterized tests.
-
Mocking and Stubbing: Built-in mocking and stubbing capabilities simplify interaction with legacy code dependencies.
-
Strong Integration: Spock integrates seamlessly with existing Spring applications, simplifying the testing of enterprise applications.
Setting Up Spock for Integration Testing
Before we dive into specific examples, let’s briefly cover how to set up Spock in a Java project. You can add Spock to your project by including it as a dependency in your build.gradle
file:
dependencies {
testImplementation 'org.spockframework:spock-core:2.0-groovy-3.0'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
Ensure that you have the appropriate versions compatible with your project setup.
Example: Testing a Legacy Service
Let's say we have a legacy service that interacts with a database. We'll demonstrate how to write a Spock test for such a service.
The Legacy Service
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User findUserById(String userId) {
return userRepository.findById(userId);
}
}
Here, UserService
leverages a UserRepository
that interacts directly with the database to retrieve user information. The goal is to write an integration test for the findUserById
method without actually hitting the database.
Spock Test for UserService
import spock.lang.Specification
import spock.lang.Unroll
class UserServiceSpec extends Specification {
def userRepository = Mock(UserRepository)
def userService = new UserService(userRepository)
@Unroll
def "should return user for id #userId"() {
given: "A mock user is returned by the repository"
userRepository.findById(userId) >> Optional.of(new User(userId: userId, name: "John Doe"))
when: "The user service is called"
def result = userService.findUserById(userId)
then: "A user should be returned"
result.userId == userId
where:
userId << ["1", "2", "3"]
}
}
Commentary on Spock Test
-
Mocking with Mock(): Here we create a mock for the
UserRepository
. This allows us to isolate our test and avoid making actual database calls. -
Using
@Unroll
: The annotation lets us run the same test case multiple times with different input values. This can help us cover various input scenarios efficiently. -
Data-driven testing via
where
: By using thewhere
block, we create parameterized tests for multiple user IDs, ensuring our service behaves consistently across a range of conditions.
Overcoming Specific Challenges
-
Handling Complexity: When legacy code is complex, mocking allows you to effectively create a controlled environment. Instead of testing the intricate code base directly, just test the interactions.
-
Mapping Out Code Paths: Use Spock's descriptive language to document code behavior within your tests. A well-commented test serves as living documentation, improving your understanding of the legacy system.
-
Environmental Consistency: Spock enables you to use TestContainers for integration with external systems like databases and messaging systems. This can help simulate production environments more accurately.
-
Fast Feedback: By isolating tests through mocking, you can speed up feedback loops. Instead of waiting on slow database responses, you receive immediate outcomes from your assertions.
-
Adoption of Modern Practices: Even in legacy scenarios, Spock helps you apply modern test practices, leading to cleaner, more maintainable, and readable code.
To Wrap Things Up
The challenges of legacy integration testing can indeed be formidable. However, with powerful tools like the Spock Framework, you can approach these hurdles with confidence. By leveraging Spock’s expressive syntax and robust features, you not only streamline your testing processes but also elevate the quality of your codebase.
As your team continues to develop and refine legacy systems, embrace modern testing practices that enhance maintainability. With Spock, integrating legacy applications does not need to be a daunting task. Take advantage of this powerful framework to create reliable tests, and you’ll find your legacy integration testing process can evolve into a more manageable, effective journey.
For further insights and advanced use cases, you can explore the Spock User Guide and start transforming how you approach legacy integration testing today!
Checkout our other articles