Mastering the Delegate Pattern: Common Pitfalls to Avoid
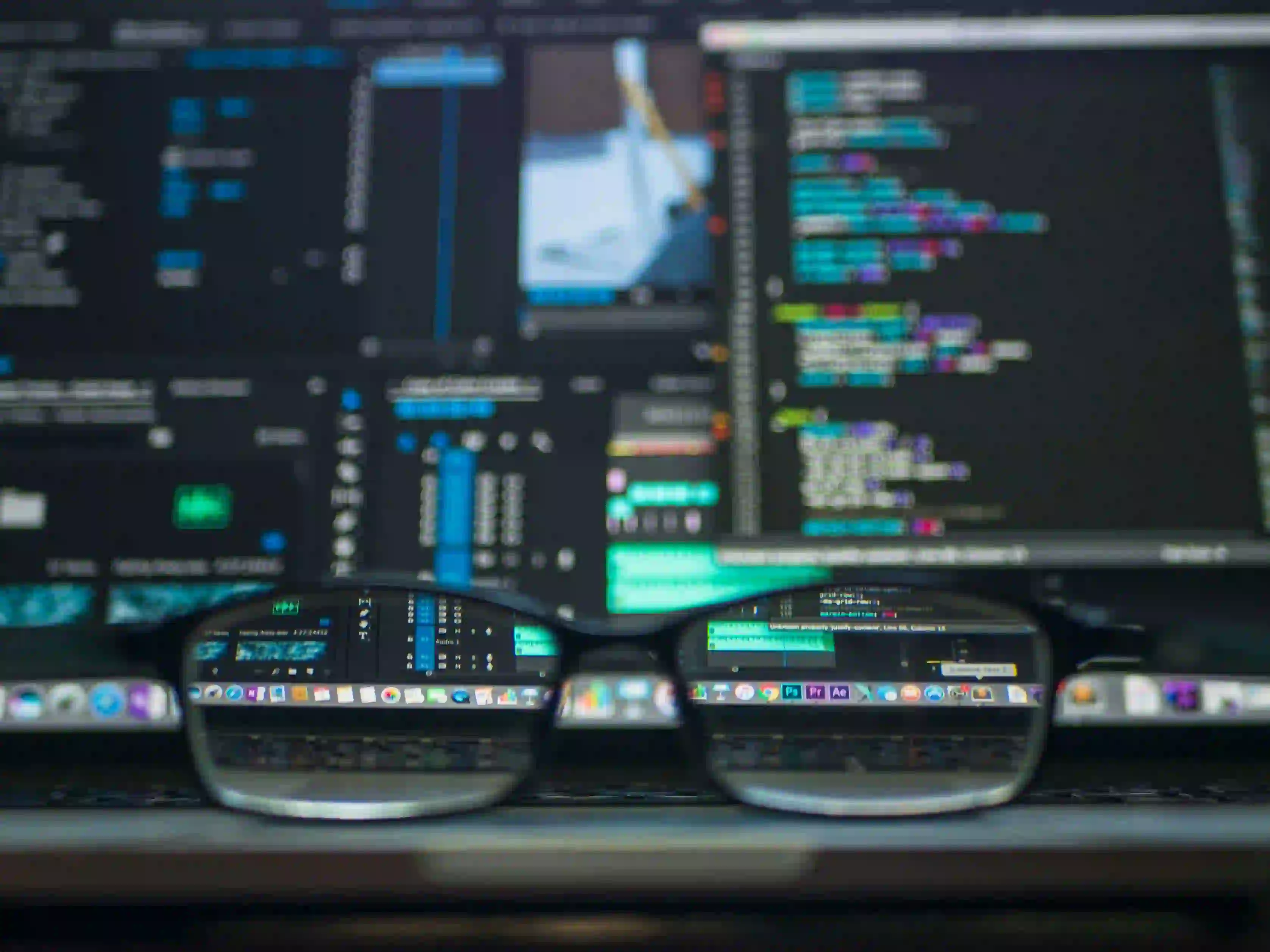
Mastering the Delegate Pattern: Common Pitfalls to Avoid
The delegate pattern is a versatile design pattern widely used in Java programming. Not only does it reduce complexity in class definitions, but it also promotes reusability and flexibility in your code. However, even seasoned developers can stumble upon common pitfalls when implementing this pattern. In this blog post, we will explore the delegate pattern, how to implement it correctly, and the common mistakes you should avoid.
What is the Delegate Pattern?
In its essence, the delegate pattern allows an object to delegate some of its responsibilities to another object. This pattern separates concerns between classes, making the system more modular and easier to maintain.
Advantages of the Delegate Pattern
- Encapsulation: The delegate encapsulates specific behaviors that can be changed without altering the main object.
- Flexibility: You can switch out delegates as needed, leading to a more adaptive codebase.
- Reusability: Delegates can be reused across different classes, promoting code reuse.
Implementing the Delegate Pattern in Java
Let's start by implementing a simple example of the delegate pattern. Imagine we have a Printer
class that is responsible for printing documents. We can delegate the actual printing operation to a Document
class.
Step 1: Define the Delegate
First, we define the Document
class that will handle the printing logic.
public class Document {
private String content;
public Document(String content) {
this.content = content;
}
public void print() {
// Simulate printing
System.out.println("Printing Document: " + content);
}
}
Step 2: Create the Delegate Owner
Next, we create a Printer
class that will delegate the print action to a Document
instance.
public class Printer {
private Document document;
public void setDocument(Document document) {
this.document = document;
}
public void printDocument() {
if (document != null) {
document.print(); // Delegates the print task to Document
} else {
System.out.println("No document to print.");
}
}
}
Step 3: Utilize the Delegate
To see the delegate pattern in action, we can create instances of Document
and Printer
and invoke the printing operation.
public class DelegatePatternExample {
public static void main(String[] args) {
Document doc1 = new Document("Hello, world!");
Printer printer = new Printer();
printer.setDocument(doc1);
printer.printDocument();
}
}
Why Does This Work?
The Printer
doesn't need to know the details of how the document is printed. It simply delegates the task to the Document
class. This encapsulation of behavior increases the separation of concerns and adheres to the Single Responsibility Principle.
Common Pitfalls to Avoid
-
Breaking Encapsulation
One of the biggest mistakes is exposing the delegate too much. If the delegate object is exposed publicly or has too many direct accesses, it undermines the encapsulation principle. Always aim to keep the delegate private or protected.
Tip: Use interfaces where possible to abstract away the implementations.
-
Tight Coupling
Strong dependencies can nullify the benefits of the delegate pattern. Make sure your delegate does not become too dependent on any specific class. Use interfaces to decouple the system.
☕snippet.javapublic interface PrintStrategy { void print(); } public class Document implements PrintStrategy { private String content; public Document(String content) { this.content = content; } @Override public void print() { System.out.println("Printing Document: " + content); } }
-
Overloading Responsibilities
Delegates should maintain a specific responsibility. When a delegate class takes on too much functionality, it becomes harder to manage and defeats the purpose of delegation.
Example: Avoid creating a
Document
class that handles file storage, rendering, and other functionalities at once. -
Not Validating State
Delegating a task without checking the state of the delegate can lead to unexpected issues. Always validate that the delegate is in a suitable state to perform the task.
☕snippet.javapublic void printDocument() { if (document == null) { throw new IllegalStateException("Document has not been set."); } document.print(); }
-
Ignoring Exception Management
When delegating tasks, remember exceptions may arise. Handling exceptions in the delegate is crucial to avoid crashes or unintended behaviors.
☕snippet.javapublic void printDocument() { try { if (document != null) { document.print(); } else { throw new IllegalArgumentException("No document to print."); } } catch (Exception e) { System.err.println("Printing error: " + e.getMessage()); } }
When to Use the Delegate Pattern
The delegate pattern is particularly useful in scenarios such as:
- Event Handling: When events occur, you can delegate the logic to specific handlers.
- Task Delegation: For tasks that can be broken down into smaller units, where each unit can be a delegate to handle a specific subtask.
- State Management: To manage various states or modes effectively, allowing delegates to handle behavior based on state.
Closing Remarks
Mastering the delegate pattern can enhance your Java programming capability and foster better software design practices. It's crucial to avoid common pitfalls such as tight coupling, breaking encapsulation, and overloading responsibilities to truly benefit from this design approach.
By implementing the delegate pattern with care, you can create code that is not only robust but also easier to read and maintain. Remember always to encapsulate behaviors, validate states, and manage exceptions, ensuring a seamless delegation process.
For further reading, consider checking out The Object-Oriented Design Principles for more design patterns and best practices to implement in your projects. Happy coding!