Unlocking Hidden Features of Spring PropertyPlaceholderConfigurer
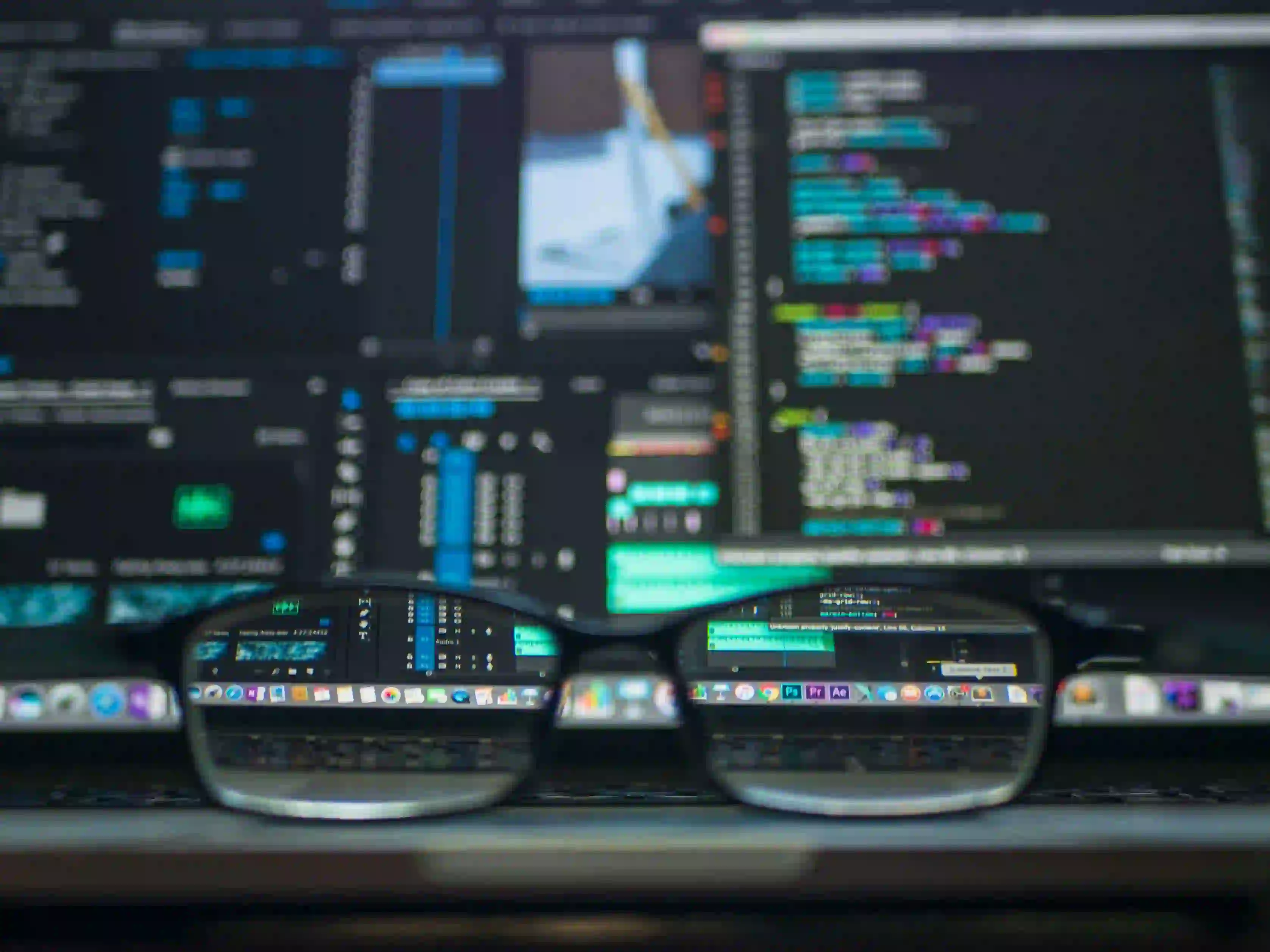
Unlocking Hidden Features of Spring PropertyPlaceholderConfigurer
The Spring Framework is a powerful and flexible tool in Java development, primarily used for building enterprise applications. One of the lesser-known but essential features within Spring is PropertyPlaceholderConfigurer
, which provides a way to externalize configuration properties and manage them effectively in your application.
In this article, we will discuss what the PropertyPlaceholderConfigurer
is, why it's significant, and explore its hidden features while providing best practices and code snippets to illustrate our points.
What is Spring PropertyPlaceholderConfigurer?
PropertyPlaceholderConfigurer
is a Spring utility that enables the loading of property files into your Spring application context. This allows developers to externalize configuration, which means you can change the configuration without modifying your source code.
Why Use PropertyPlaceholderConfigurer
?
- Separation of Configuration: It decouples configuration from your code, enabling you to swap out configuration files based on different environments (development, testing, production).
- Enhanced Readability: It makes managing your application more straightforward. You can quickly understand the configuration using a properties file rather than sifting through Java code.
- Dynamic Configuration: You can change properties without the need to restart the application.
Here, we will unlock some hidden features of the PropertyPlaceholderConfigurer
that can greatly enhance your application's configuration management.
Setting Up PropertyPlaceholderConfigurer
To use PropertyPlaceholderConfigurer
, you must first configure it in your Spring application context.
<context:property-placeholder location="classpath:application.properties"/>
In the above snippet, context:property-placeholder
loads the application.properties
file from the classpath.
Example property file
Consider a sample application.properties
file:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=db_user
spring.datasource.password=db_password
To use these properties in your Spring beans, you might configure it like this:
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="url" value="${spring.datasource.url}"/>
<property name="username" value="${spring.datasource.username}"/>
<property name="password" value="${spring.datasource.password}"/>
</bean>
In the dataSource
bean above, the ${...}
syntax is used to reference the properties defined in the properties file.
Hidden Features of Spring PropertyPlaceholderConfigurer
1. Support for Multiple Property Files
One of the hidden features of PropertyPlaceholderConfigurer
is the ability to load multiple property files. This can be useful when you have a common properties file for all environments and an environment-specific one.
<context:property-placeholder>
<property name="locations">
<list>
<value>classpath:common.properties</value>
<value>classpath:dev.properties</value>
</list>
</property>
</context:property-placeholder>
In this case, if there are overlapping properties, the last file loaded will take precedence, allowing you to easily override settings for specific environments.
2. Property Overrides in XML
Sometimes, modifying a property file may not be convenient when doing quick changes. You can override properties directly in your XML configuration using the property
element.
<context:property-placeholder>
<property name="spring.datasource.password" value="new_secure_password"/>
</context:property-placeholder>
This method can be particularly helpful in CI/CD pipelines where specific values might change dynamically, making it easier to manage sensitive information without changing the property file.
3. System Properties
You can inject system properties directly into your beans using ${systemPropertyName}
syntax. This allows you to use various settings from the environment when initializing beans.
<bean id="myBean" class="com.example.MyBean">
<property name="someProperty" value="${system.property.name}"/>
</bean>
You can provide system properties as JVM arguments when starting your application, like this:
java -Dsystem.property.name=myValue -jar myapp.jar
4. Fallback Properties with Default Values
If you wish to provide defaults for your properties, you can specify them directly in your XML, which will act as fallbacks if the property is not found.
<context:property-placeholder location="classpath:application.properties" ignore-unresolvable="true" />
If a property cannot be resolved, it can default to null
, which your application can handle gracefully.
5. Profiles Support
In addition to loading properties based on environment, Spring also supports profiles that can help in selectively activating specific properties.
Create two property files, application-dev.properties
and application-prod.properties
. You can specify the active profile in your XML configuration as follows:
<beans profile="dev">
<context:property-placeholder location="classpath:application-dev.properties"/>
</beans>
<beans profile="prod">
<context:property-placeholder location="classpath:application-prod.properties"/>
</beans>
To activate a profile, you can pass the spring.profiles.active
argument when starting your application:
java -Dspring.profiles.active=dev -jar myapp.jar
Best Practices When Using PropertyPlaceholderConfigurer
- Keep Configuration Simple: Avoid overly complex configurations; fewer properties make it easier to maintain.
- Use Clear Naming Conventions: Use meaningful property names, which will aid in readability and understanding.
- Secure Sensitive Data: Avoid placing sensitive data in properties files. Consider offering encryption or using a vault service (such as Spring Cloud Config).
- Documenting Your Properties: Comment on properties in the property files to maintain clarity for future developers or for revisiting your own code.
Key Takeaways
The PropertyPlaceholderConfigurer
in Spring offers numerous benefits and hidden capabilities that streamline configuration management in Java applications. Through our exploration, we have seen various ways to load multiple property files, inject system properties, provide default values, and take advantage of profiles.
By following best practices and these techniques, you can maintain a clean, scalable, and secure configuration for your Spring applications.
For more in-depth Spring tutorials and guides, feel free to explore the official Spring documentation.
Example Repository
To see these features in action, I recommend visiting this GitHub repository where a sample Spring Boot project demonstrates how to effectively use PropertyPlaceholderConfigurer
.
Happy coding!