Mastering Advanced Java: Top Resources You Must Know
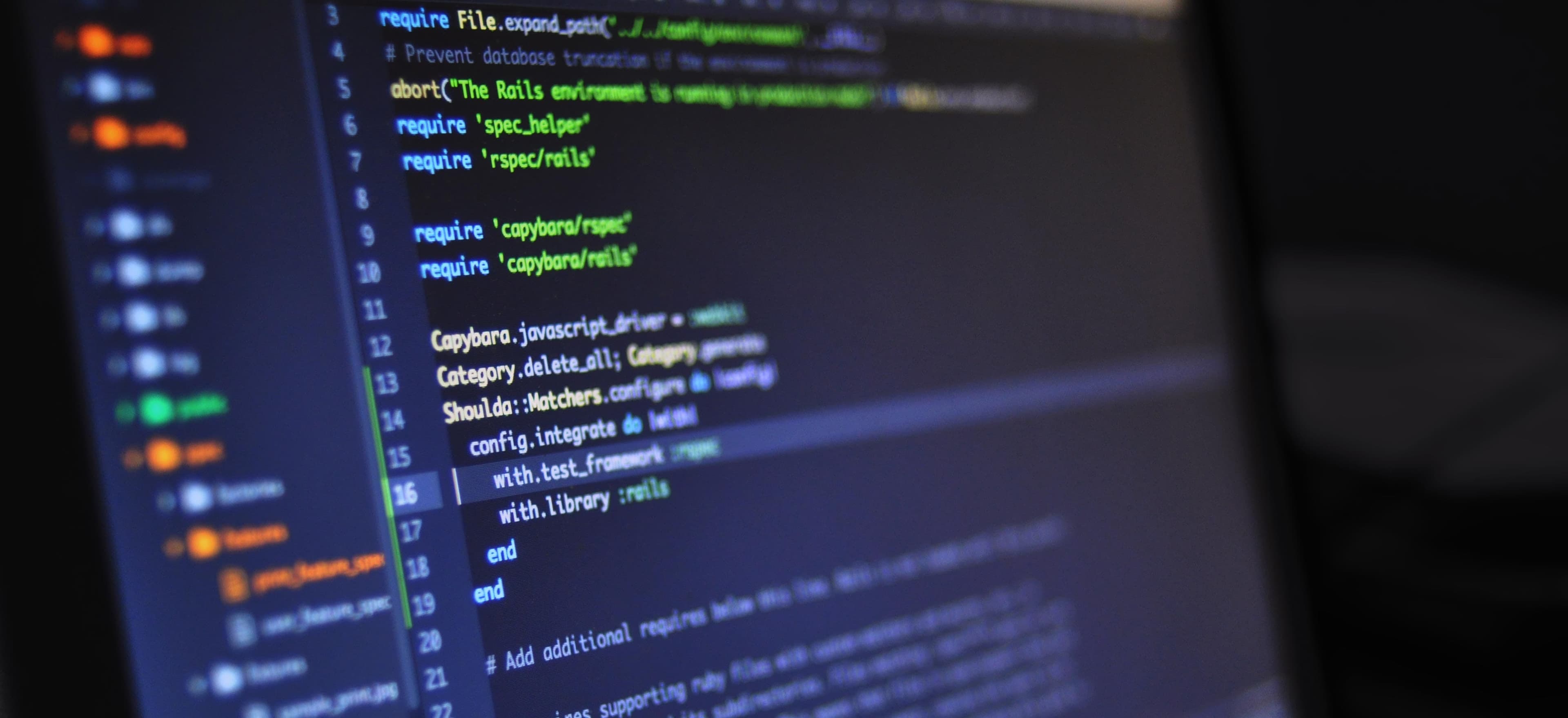
- Published on
Mastering Advanced Java: Top Resources You Must Know
Java has been a leading programming language for decades, making its way into enterprise applications, mobile apps, and even server-side technology. As you transition from a beginner to an advanced Java developer, having the right resources can significantly impact your learning curve and mastery of complex topics. This blog post will cover the top resources, tools, and frameworks that every advanced Java developer should be aware of, while providing code snippets to illustrate key points.
Why Master Advanced Java?
Before diving into resources, let's briefly discuss the importance of mastering advanced Java. While basics like syntax and data structures are crucial, advanced knowledge equips developers with tools to tackle complex problems and enhance efficiency. Mastering advanced concepts such as multi-threading, concurrency, and design patterns can lead to better performance, maintainability, and scalability in applications.
Essential Resources for Advanced Java Development
1. Online Learning Platforms
Several online platforms deliver comprehensive courses in advanced Java programming. Here are some of the best:
- Coursera: Offers courses like "Java Programming and Software Engineering Fundamentals" and specialized courses on Java frameworks.
- Udemy: Provides a variety of in-depth tutorials and projects focused on advanced Java topics, often at a reasonable cost.
- edX: Hosts university-level courses that delve into advanced Java techniques.
These platforms typically offer a mix of video lectures, quizzes, and hands-on projects, effectively blending theoretical knowledge with practical application.
2. Books Worth Reading
Books remain one of the best ways to deepen your understanding. Some recommended books include:
-
Effective Java by Joshua Bloch: A must-read for understanding best practices in Java programming. It comprises several guidelines that will help you write more efficient and understandable Java code.
// Example of a singleton pattern in Effective Java public class Singleton { private static final Singleton INSTANCE = new Singleton(); private Singleton() {} public static Singleton getInstance() { return INSTANCE; } }
This code snippet illustrates the singleton design pattern, which ensures that a class has only one instance and provides a global point of access to it. This is fundamental in many scenarios where a single resource is required.
-
Java Concurrency in Practice by Brian Goetz: This book introduces advanced concurrency concepts and how to effectively utilize threads in Java applications.
3. Java Frameworks and Libraries
If you want to conquer advanced Java, familiarity with mainstream frameworks is vital.
-
Spring Framework: A powerful framework for developing Java applications. It simplifies data access, security, transaction management, and more. The Spring Boot extension can help you quickly create stand-alone applications.
// A simple REST controller using Spring Boot @RestController public class HelloWorldController { @GetMapping("/hello") public String helloWorld() { return "Hello, World!"; } }
This code snippet shows how simple it is to create a RESTful service using Spring Boot. Understanding how to build microservices is paramount in modern Java development.
-
Apache Maven: A project management tool that helps in managing build processes. It is indispensable for handling dependencies in larger projects.
4. Advanced Java Concepts
To truly excel, you need to understand and master advanced Java concepts:
-
Functional Programming in Java: Java 8 introduced features like lambdas and streams that allow for functional programming patterns, which can lead to clearer and more concise code.
// Using a stream to filter a list List<String> names = Arrays.asList("Alice", "Bob", "Charlie"); List<String> filteredNames = names.stream() .filter(name -> name.startsWith("A")) .collect(Collectors.toList());
In this example, the use of streams simplifies the operation of filtering names, showcasing modern Java's capabilities in functional programming.
-
Design Patterns: Familiarity with design patterns such as the Observer, Strategy, and Factory patterns can significantly enhance the way you write Java applications.
// Simple Factory Pattern Example public class ShapeFactory { public static Shape getShape(String shapeType) { if ("circle".equalsIgnoreCase(shapeType)) { return new Circle(); } // Additional shape creation logic... return null; } }
Using the Factory pattern helps in decoupling object creation from the client code, rendering it easier to scale the application later.
5. Community and Forums
Engaging with communities can provide invaluable support and resource sharing. Some of the best places to participate include:
- Stack Overflow: Perfect for asking specific questions and viewing solutions related to Java coding problems.
- Reddit: Subreddits like r/java can be an excellent way to stay updated on the latest trends and discussions within the Java community.
- Java User Groups (JUGs): Join local Java meetups to network and learn from other professionals.
6. Advanced Tools
Tools can elevate your development experience. Here are a few to consider:
- IDE Plugins: Using integrations such as Lombok or CheckStyle within your IDE can lead to cleaner and more efficient code.
- JProfiler: A popular open-source profiler that helps developers find memory leaks and performance bottlenecks within applications.
Putting It All Together
Mastering advanced Java is a journey that encompasses many facets, from technical skills to the use of numerous tools and frameworks. By leveraging a mix of online courses, books, and community engagement, you are setting yourself up for success.
Continuous learning is crucial, especially in a technology as expansive as Java. Make sure to explore and dive into new areas, like microservices architecture, reactive programming, and cloud technologies.
Lessons Learned
The resources outlined in this post will serve as pillars in your quest to master advanced Java development. Embrace the learning process, utilize the tools at your disposal, and never hesitate to ask for help from the community or online forums.
For additional context on Java or related technologies, you can explore:
Happy coding!
Checkout our other articles