Solving Common Issues with Multi-Level Fetching in Hibernate
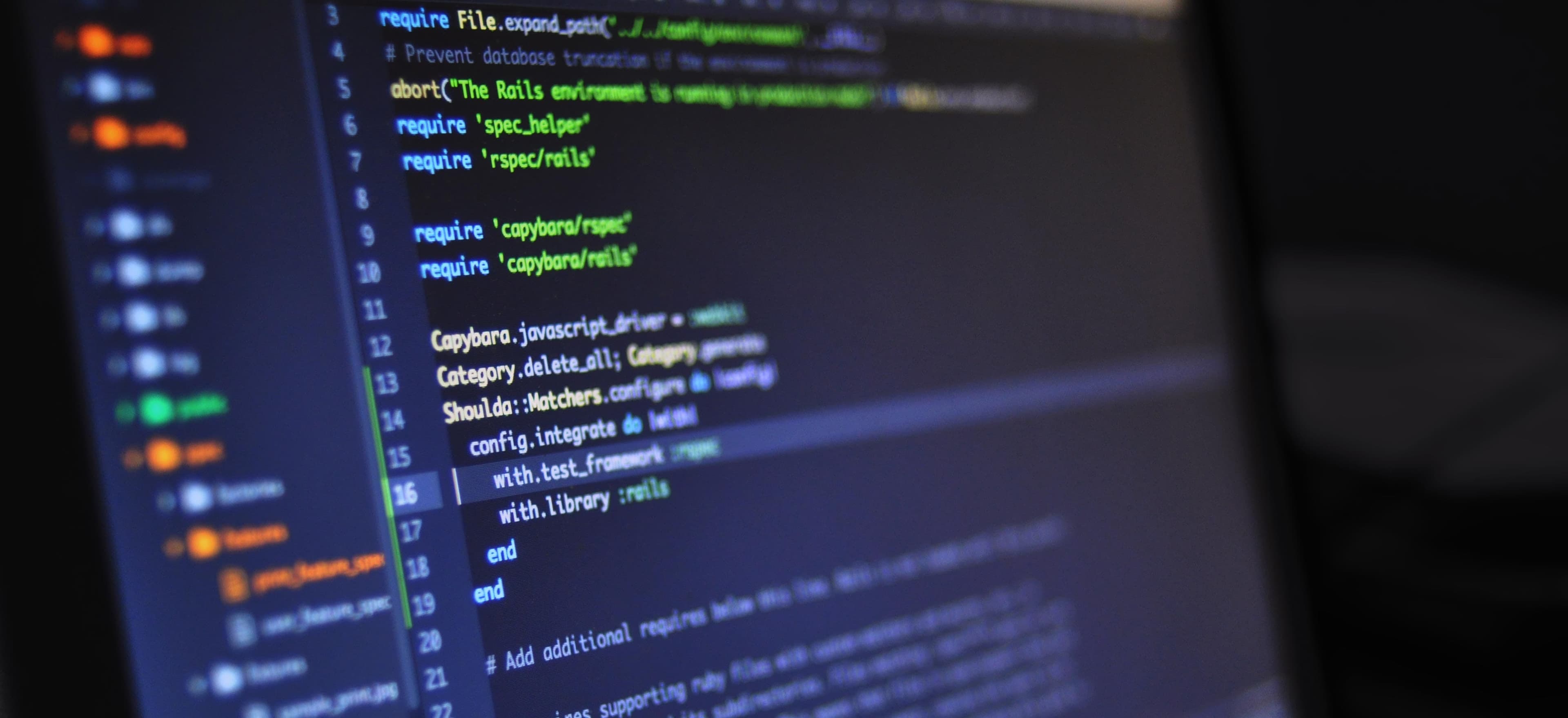
- Published on
Solving Common Issues with Multi-Level Fetching in Hibernate
Hibernate, as an Object-Relational Mapping (ORM) framework, provides developers with tools that simplify data access in Java applications. One of the powerful features of Hibernate is its ability to fetch data across multiple levels of associations. However, multi-level fetching can also lead to issues such as N+1 selects problem or unnecessary data loading, which can significantly decrease application performance.
In this blog post, we'll explore how to solve common problems associated with multi-level fetching in Hibernate and provide practical code snippets to guide you along the way.
Understanding Multi-Level Fetching
Multi-level fetching involves loading not just an entity, but also its related entities. For instance, if you have a Department
entity that has multiple Employee
entities, and each Employee
entity links to a Project
entity, loading a department with its employees and their projects would be considered multi-level fetching.
Consider the following entity relationship:
- Department
- Has many Employees
- Employee
- Has many Projects
Example Entity Classes
@Entity
public class Department {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "department", fetch = FetchType.LAZY)
private List<Employee> employees;
// Getters and Setters
}
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@ManyToOne
@JoinColumn(name = "department_id")
private Department department;
@OneToMany(mappedBy = "employee", fetch = FetchType.LAZY)
private List<Project> projects;
// Getters and Setters
}
@Entity
public class Project {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToOne
@JoinColumn(name = "employee_id")
private Employee employee;
// Getters and Setters
}
In this setup, fetching a Department
will result in retrieval of its employees and their respective projects if we set up the fetching strategy correctly.
Common Issues and Solutions
1. The N+1 Selects Problem
One of the most common pitfalls in multi-level fetching is the N+1 selects problem, where an additional query is fired for each associated entity. This can dramatically slow down your application, especially when dealing with large datasets.
Solution: Use Eager Fetching
To mitigate the N+1 selects problem, consider employing eager fetching when it makes sense. You can use Hibernate's JOIN FETCH
to load associations in a single query.
String hql = "SELECT d FROM Department d JOIN FETCH d.employees e JOIN FETCH e.projects";
TypedQuery<Department> query = entityManager.createQuery(hql, Department.class);
List<Department> departments = query.getResultList();
2. Cartesian Product Issues
When using JOIN FETCH
, you may end up with a Cartesian product if you're not careful, leading to duplicated records in your results. This can create issues with data processing and performance.
Solution: Use Set instead of List
Change the collection type to a Set
in your entities. This will automatically remove duplicates since sets cannot contain duplicate elements.
@OneToMany(mappedBy = "department", fetch = FetchType.LAZY)
private Set<Employee> employees = new HashSet<>();
@OneToMany(mappedBy = "employee", fetch = FetchType.LAZY)
private Set<Project> projects = new HashSet<>();
3. Fetching Too Much Database Data
Fetching more data than necessary can slow down your application. Sometimes you need only a subset of the data in related entities.
Solution: Specify Projections
You can also use projections to fetch only the required fields. Let’s say we only want the name
of the employee and the title
of the project.
String hql = "SELECT new map(e.name as employeeName, p.title as projectTitle) " +
"FROM Department d JOIN d.employees e JOIN e.projects p";
TypedQuery<Map<String, String>> query = entityManager.createQuery(hql, Map.class);
List<Map<String, String>> results = query.getResultList();
4. LazyInitializationException
If you attempt to access a lazily-loaded collection outside of the Hibernate session, you will run into LazyInitializationException
. This often occurs in web applications after executing a query within a transaction's scope, then trying to access the data outside the transaction.
Solution: Open Session in View Pattern
In web applications, employing the "Open Session in View" pattern allows you to keep the session open during the rendering of the view:
<bean id="sessionFactory" class="org.springframework.orm.hibernate5.LocalSessionFactoryBean">
<!-- Configuration settings -->
</bean>
<bean id="openSessionInViewFilter" class="org.springframework.orm.hibernate5.support.OpenSessionInViewFilter" />
5. Caching Strategies
If not managed properly, fetching can overwhelm your application with queries, particularly in a high-traffic environment. Caching can improve performance significantly.
Solution: Utilize Second Level Cache
Hibernate supports second-level caching, which can be configured in hibernate.cfg.xml
:
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property>
The Closing Argument
Multi-level fetching in Hibernate can provide immense benefits, but it can also introduce challenges that affect performance and data integrity. By understanding and addressing issues such as the N+1 selects problem, Cartesian product risks, excessive data fetching, and improper session handling, we can harness the full power of Hibernate without compromising application performance.
For further reading, refer to the following resources:
- Hibernate Documentation on Fetching Strategies
- Spring Framework's
Open Session in View
Now that you are equipped with solutions to common issues in multi-level fetching with Hibernate, you're ready to implement effective data access strategies in your Java applications!
Checkout our other articles