Common Apache Spark Cluster Deployment Pitfalls and Fixes
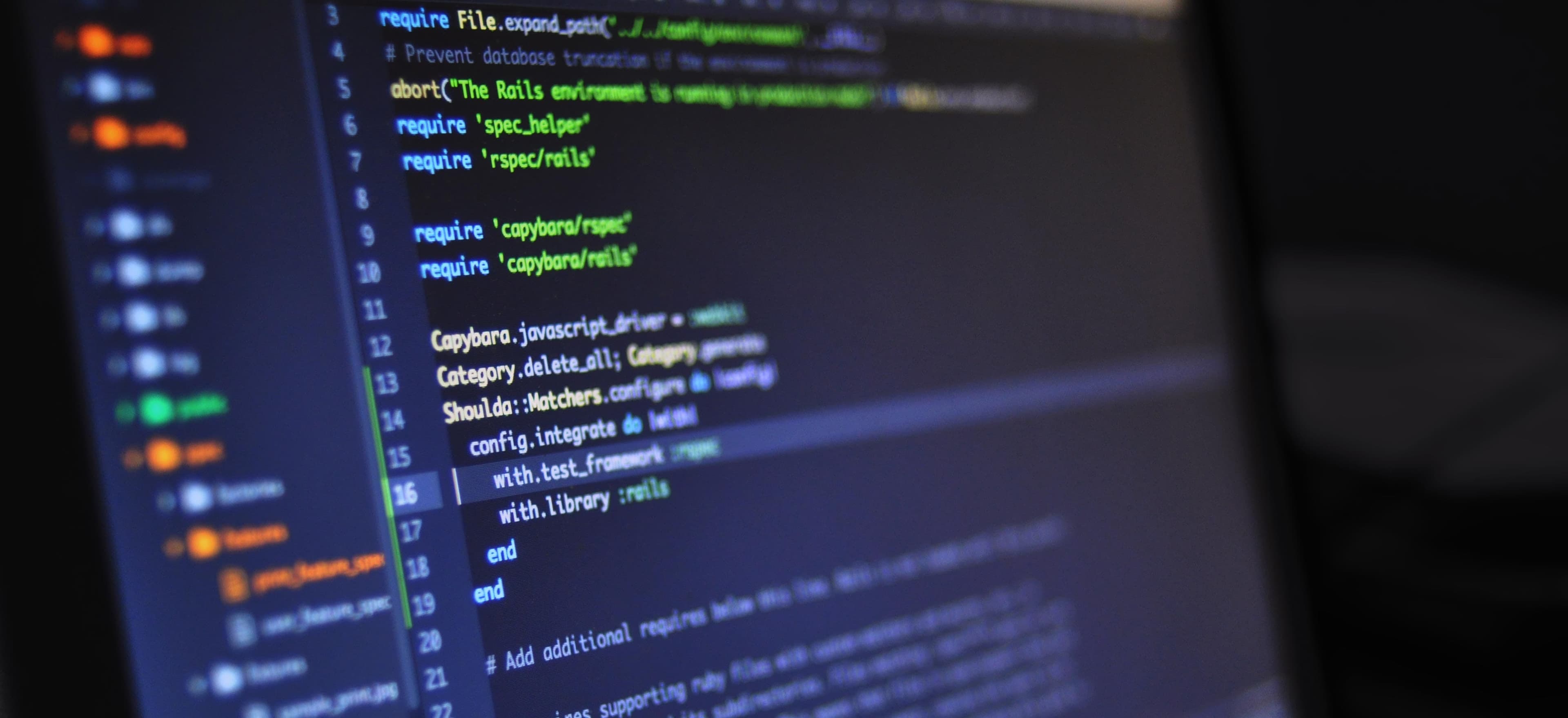
- Published on
Common Apache Spark Cluster Deployment Pitfalls and Fixes
Apache Spark is a powerful open-source engine for big data processing. It offers high-level APIs in various programming languages and has built-in modules for SQL, streaming, machine learning, and graph processing. However, deploying Spark clusters can present some challenges. In this blog post, we will discuss some common pitfalls when deploying Apache Spark clusters and their corresponding fixes.
Understanding the Spark Ecosystem
Before diving into the common pitfalls, it’s important to have a good understanding of the Spark ecosystem. Apache Spark primarily consists of the following components:
- Spark Core: The fundamental component providing basic functionality.
- Spark SQL: For querying structured data.
- MLlib: For machine learning applications.
- GraphX: For graph processing.
- Spark Streaming: For processing real-time data.
With this understanding, let’s explore the common deployment issues.
Pitfall 1: Incorrect Cluster Sizing
The Issue
One of the most frequent mistakes made when deploying a Spark cluster is misjudging the size of the cluster. Whether it’s an under-provisioned cluster that struggles to handle workloads or an over-provisioned one leading to wasted resources, getting this step right is crucial.
The Fix
To avoid this pitfall, start with a thorough understanding of the workload requirements. Conduct performance benchmarks and analyze historical data to determine the right amount of CPU cores, memory, and storage required.
# Example: Using Apache Spark built-in metrics to assess resource usage
./bin/spark-submit --class org.apache.spark.examples.SparkPi --master <master-url> \
--conf spark.executor.memory=4g --conf spark.executor.cores=2 \
examples/jars/spark-examples_2.12-3.1.1.jar 1000
By monitoring Spark's web UI, you can observe memory consumption patterns and adjust configurations accordingly. The spark.executor.memory
and spark.executor.cores
settings can greatly impact performance.
Pitfall 2: Ignoring Data Locality
The Issue
Data locality refers to the proximity of data to the compute resources that process it. Ignoring data locality can lead to performance issues due to increased data transfer times.
The Fix
Optimize your data storage so that it aligns with how your Spark jobs are executed. Utilizing distributed filesystems like HDFS can help leverage data locality better.
# PySpark example for reading from HDFS
from pyspark.sql import SparkSession
spark = SparkSession.builder \
.appName("Data Locality Example") \
.getOrCreate()
# Loading data that is stored in HDFS
df = spark.read.csv("hdfs://<namenode>:<port>/path/to/data.csv")
By organizing your data in HDFS correctly, Spark can perform data-aware scheduling, leading to reduced latency and improved throughputs.
Pitfall 3: Resource Configuration Missteps
The Issue
Inadequate configuration of resources such as memory, CPU, and storage can severely impact Spark’s execution performance.
The Fix
Leverage Spark configuration parameters to effectively allocate resources.
# Configuration settings in spark-defaults.conf
spark.driver.memory 4g
spark.executor.memory 4g
spark.memory.fraction 0.75
spark.executor.instances 3
Understanding these configurations helps ensure that your jobs run efficiently. The spark.memory.fraction
parameter is critical, as it dictates how much of the executor memory is devoted to storage rather than computation tasks.
Pitfall 4: Data Serialization Issues
The Issue
Data serialization plays a key role in data transfer among the Spark nodes. If your serialization mechanism is poorly chosen, it can lead to performance bottlenecks.
The Fix
Choose the appropriate serialization format based on your use case. For most scenarios, using Kryo serialization is preferred due to its efficiency.
# Enable Kryo serialization in spark-defaults.conf
spark.serializer org.apache.spark.serializer.KryoSerializer
spark.kryo.registrator com.example.KryoRegistrator
By registering custom classes using Kryo, you can further optimize serialization performance.
Pitfall 5: Neglecting Task Parallelism
The Issue
Not utilizing task parallelism effectively may lead to resource underutilization. Ignoring the optimal number of tasks can slow down job execution.
The Fix
To fix this, ensure that your Spark jobs are designed to take advantage of available parallelism. Setting the number of partitions correctly can lead to better performance.
# Adjusting the number of partitions in PySpark
rdd = spark.textFile("hdfs://<namenode>:<port>/path/to/data.txt")
rdd = rdd.repartition(10) # Sets number of partitions to 10
result = rdd.count()
The repartition
function allows you to increase the number of partitions, distributing your workload more evenly across the cluster.
Pitfall 6: Poorly Managed Dependencies
The Issue
Apache Spark jobs often rely on external libraries. If these dependencies are poorly managed, they can lead to issues such as version conflicts and runtime errors.
The Fix
Utilize a build management tool such as Maven or SBT to manage your dependencies efficiently.
<!-- Example in Maven pom.xml -->
<dependency>
<groupId>org.apache.spark</groupId>
<artifactId>spark-sql_2.12</artifactId>
<version>3.1.1</version>
</dependency>
By specifying versions and scopes, you can maintain a stable environment and avoid inconsistencies across different deployments.
Pitfall 7: Lack of Monitoring and Logging
The Issue
Monitoring and logging are critical yet often overlooked aspects of Spark deployment. Without proper visibility, it's hard to troubleshoot problems in real time.
The Fix
Promote the use of monitoring tools such as Ganglia, Spark's built-in UI, or third-party services like Datadog for real-time performance monitoring.
# Access Spark monitoring UI
http://<spark-master-url>:8080/
Utilizing effective logging can also provide insights into job executions and performance. Ensure that you’ve configured the logging level appropriately.
# Log4j properties in log4j.properties
log4j.logger.org.apache.spark=INFO
log4j.logger.org.apache.hadoop=ERROR
By keeping track of logs, you can preemptively identify issues before they escalate.
Final Considerations
Deploying an Apache Spark cluster can seem daunting, but being aware of common pitfalls can lead to a smoother and more efficient deployment process. Ensure that you size your cluster correctly, pay attention to data locality, configure resources appropriately, choose the right serialization method, utilize task parallelism, manage dependencies judiciously, and implement robust monitoring practices.
By addressing these issues directly, you can create a more resilient Apache Spark environment capable of handling big data workloads efficiently. For further information on optimizing your Spark deployment, you can explore the official Apache Spark documentation.
Embrace the power of Spark, tackle these common pitfalls, and unlock efficient data processing for your organization. Happy coding!
Checkout our other articles