Mastering JPA: Common Mapping Errors to Avoid
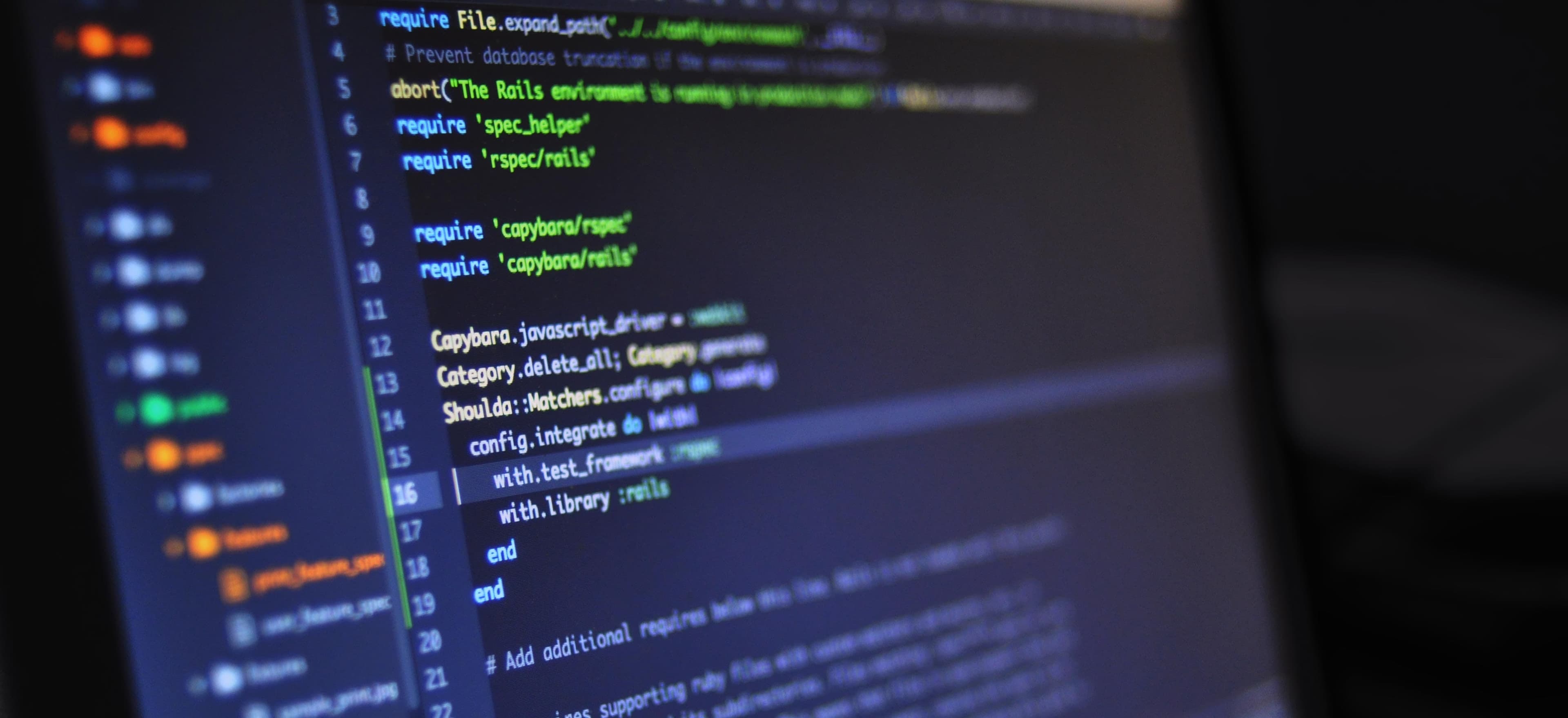
- Published on
Mastering JPA: Common Mapping Errors to Avoid
Java Persistence API (JPA) is an essential technology for Java developers involved in data access and manipulation. It provides a standard for ORM (Object-Relational Mapping) which simplifies database interactions. However, working with JPA is not without its pitfalls. This blog post will dive into common mapping errors developers face and how to avoid them.
Understanding JPA Mapping
JPA allows developers to define how Java objects map to database tables. This mapping involves several annotations that dictate relationships, constraints, and how Java objects should be persisted.
A basic understanding of entities and their relationships will help solidify your grasp of JPA mapping. Here’s a simplified entity model:
import javax.persistence.*;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
@OneToMany(mappedBy = "user", cascade = CascadeType.ALL)
private List<Order> orders;
// Getters and Setters
}
In this code snippet, we declare a User
entity mapped to the "users" table. The @OneToMany
annotation establishes a relationship with the Order
entity.
Common Mapping Errors
1. Forgetting the @Id Annotation
Every entity must have a primary key. Failing to annotate a field with @Id
results in an EntityNotFoundException
. This is a fundamental requirement of JPA.
Example:
@Entity
@Table(name = "users")
public class User {
// Forgetting @Id creates issues
private Long id; // Wrong: This field should have @Id annotation
// ...
}
Solution
Always ensure that your primary key is annotated:
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
2. Misconfigured Relationships
Misconfiguring relationships between entities can lead to an array of problems. For example, if the relationship is supposed to be @ManyToOne
, yet you configure it as @OneToMany
, it may confuse the JPA provider leading to unexpected behaviors.
Example:
@Entity
public class Order {
// Incorrectly assuming a ManyToOne relationship
@ManyToMany
private User user; // This should probably be ManyToOne
}
Solution
Understand the cardinality of the relationship and use the appropriate annotations.
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
3. Using Incorrect Fetch Types
The fetch type determines how related entities are retrieved. Setting the fetch type to FetchType.EAGER
by default can significantly hinder performance because it fetches all related entities immediately.
Example:
@OneToMany(fetch = FetchType.EAGER)
private List<Order> orders; // This can lead to performance issues
Solution
Use FetchType.LAZY
where applicable to load related entities on demand:
@OneToMany(fetch = FetchType.LAZY)
private List<Order> orders;
4. Not Handling Bidirectional Relationships Properly
Bidirectional relationships need careful management to avoid cycling and unnecessary complexity. If one side of the relationship is not keeping the other in synchronization, it may lead to issues.
Example:
@Entity
public class User {
@OneToMany(mappedBy = "user")
private List<Order> orders;
}
@Entity
public class Order {
@ManyToOne
private User user; // Not synchronized with User
}
Solution
Ensure both sides of the relationship are synchronized:
public void addOrder(Order order) {
orders.add(order);
order.setUser(this); // Keep both sides updated
}
5. Ignoring Cascade Types
Cascade operations are essential to maintain the lifecycle of related entities. Neglecting the appropriate cascade types can lead to foreign key constraints being violated.
Example:
@OneToMany(mappedBy = "user")
private List<Order> orders; // Forgetting cascade can lead to orphaned entities
Solution
Define cascade types based on your business requirements:
@OneToMany(mappedBy = "user", cascade = CascadeType.ALL)
private List<Order> orders;
6. Not Utilizing Proper Constraints
Failing to use constraints such as @Column(nullable = false)
or @UniqueConstraint
can lead to issues when invalid data tries to persist.
Example:
@Column
private String username; // Should be nullable=false unless specified
Solution
Always define constraints that reflect the business rules:
@Column(nullable = false, unique = true)
private String username; // Username must be unique and not null
Debugging JPA Mapping Issues
When debugging mapping issues:
-
Enable SQL Logging: Configure your application to log SQL statements to understand what queries JPA generates.
spring.jpa.show-sql=true
-
Review Exception Logs: Analyze exception stack traces to identify where an issue might be stemming from.
-
Utilize Entity Graphs: JPA 2.1 introduced entity graphs to customize fetch plans for specific use cases, aiding in troubleshooting relationship issues.
The Bottom Line
Utilizing JPA effectively involves understanding its mapping configurations and adhering to best practices. Mapping errors can lead to catastrophic failures in your application, but they can be sidestepped with the insights provided in this guide.
For more information about advanced JPA configurations, consider checking out the official JPA specification or exploring the vast resources available through Baeldung's JPA tutorials.
Adopting robust practices and paying attention to detail will significantly improve your persistence layer's performance and reliability. Now that you’re aware of the common mapping errors, it’s time to refine your skills and master JPA!
Checkout our other articles