Challenges of Implementing Immutable Embedded Builders
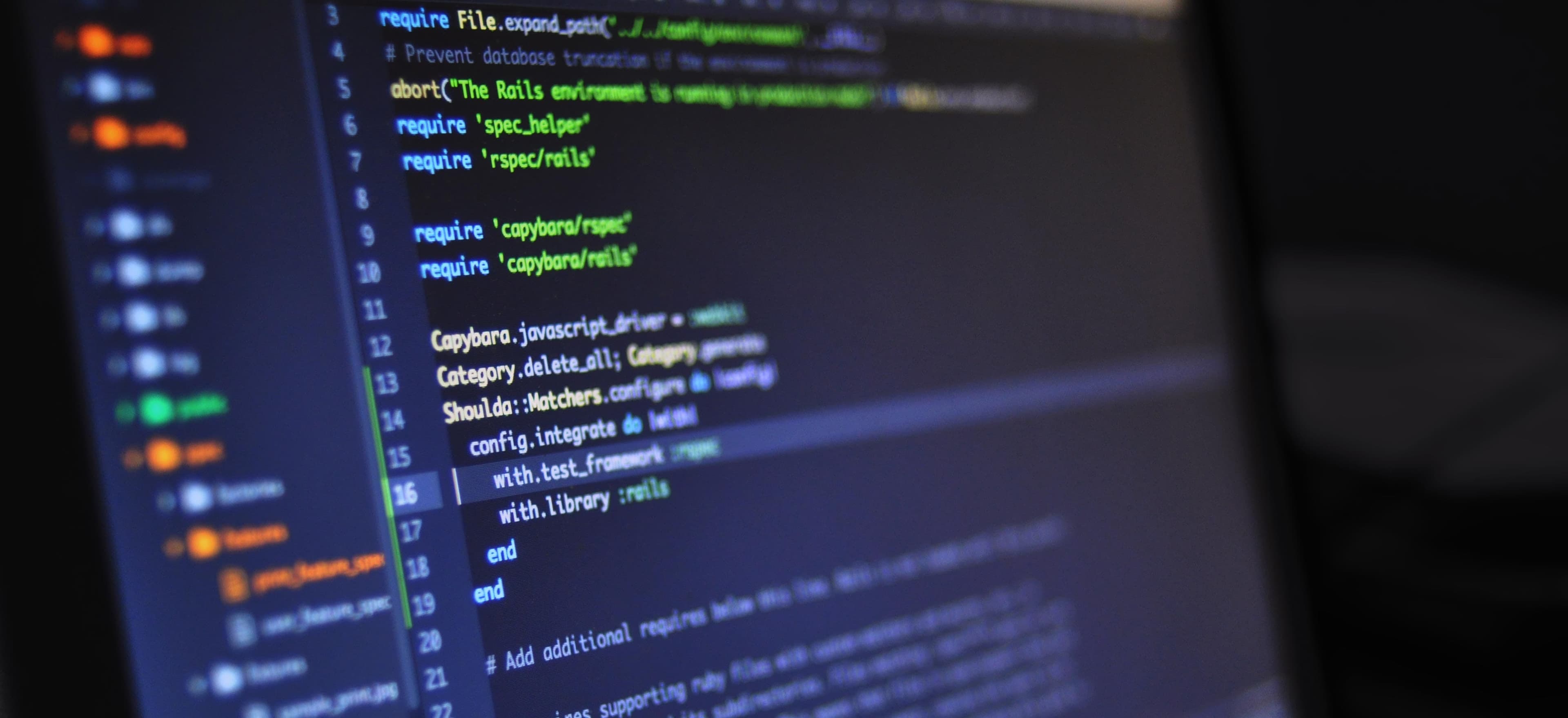
- Published on
Challenges of Implementing Immutable Embedded Builders in Java
Immutable embedded builders are a design paradigm that harnesses immutability to create flexible and easily maintainable object creation patterns in Java. Although this design pattern offers several benefits, such as enhanced thread safety and easier reasoning about code, there are also significant challenges. In this blog post, we'll dive into these challenges, explore their implications, and offer solutions to mitigate them.
What Are Immutable Embedded Builders?
Before we delve into the challenges, let's address what we mean by "immutable embedded builders." An immutable builder is an abstract design pattern where the builder itself does not change state after its creation. This contrasts with traditional builders, which allow for mutable state modifications.
Embedded builder refers to a pattern where a builder for a nested or complex object is encapsulated within another class. This allows you to construct objects with complex structures seamlessly.
Why Choose Immutable Builders?
Here are some compelling reasons for opting for immutability:
- Thread Safety: Immutable objects are inherently thread-safe since their state cannot change after construction.
- Easy to Debug: Since state doesn't change, tracking the source of bugs becomes more straightforward.
- Composability: Immutable builders allow for easier composition, enabling complex object creation without side effects.
The Challenges of Implementing Immutable Embedded Builders
While the advantages are clear, implementing immutable embedded builders presents its unique set of challenges. Let's explore them in detail.
1. Increased Complexity in Code Structure
Immutable embedded builders may lead to complex class hierarchies. Designing a builder for a nested object within the parent object could lead to nested builders that complicate the code.
public class User {
private final String username;
private final Profile profile;
private User(UserBuilder builder) {
this.username = builder.username;
this.profile = builder.profile;
}
public static UserBuilder builder() {
return new UserBuilder();
}
public static class UserBuilder {
private String username;
private Profile profile;
public UserBuilder username(String username) {
this.username = username;
return this;
}
public UserBuilder profile(Profile profile) {
this.profile = profile;
return this;
}
public User build() {
return new User(this);
}
}
}
public class Profile {
private final String bio;
private Profile(ProfileBuilder builder) {
this.bio = builder.bio;
}
public static ProfileBuilder builder() {
return new ProfileBuilder();
}
public static class ProfileBuilder {
private String bio;
public ProfileBuilder bio(String bio) {
this.bio = bio;
return this;
}
public Profile build() {
return new Profile(this);
}
}
}
Commentary on Code Example
In the above code snippet, we see a User
class and its corresponding UserBuilder
. The Profile
class is similarly structured with its own builder, ProfileBuilder
. While this design provides a certain level of clarity, managing nested immutable builders can become unwieldy, especially for large applications.
2. Duplicated Code
In scenarios where multiple builders have similar attributes, repeating similar code across builders can lead to bloated and redundant codebases. This duplication reduces maintainability.
Solution to Code Duplication
To prevent redundancy, you can use a base builder class or an interface that encapsulates common builder logic.
public abstract class BaseBuilder<T> {
protected String commonField;
public BaseBuilder<T> commonField(String commonField) {
this.commonField = commonField;
return this;
}
}
3. Difficulty in Validation
Valuating the input during construction can be tricky. Immutable builders encapsulate creation logic, but imposing validation rules throughout various builders could complicate development.
Example of Validation
You can tackle validation separately, either in a static factory method or within the builder's build
method.
public User build() {
if (username == null || username.isEmpty()) {
throw new IllegalArgumentException("Username cannot be null or empty");
}
return new User(this);
}
4. Learning Curve for New Developers
Developers who are new to the immutable design paradigm may find it challenging to understand and adapt to the structure of immutable builders. The concept itself can introduce a steep learning curve.
Mitigating the Learning Curve
- Documentation: Comprehensive documentation keeps your team on the same page.
- Training: Conduct workshops or tutorials focused on immutable programming concepts.
5. Performance Concerns
Creating multiple immutable objects can lead to performance issues due to memory allocation. Frequent allocations can result in increased garbage collection overhead, especially with large object hierarchies.
Performance Considerations
- Object Pooling: Implementing object pooling for frequently used objects can mitigate performance concerns.
- Value Objects: Instead of creating new instances, consider using value objects when appropriate.
6. Balancing Immutability with Flexibility
Another challenge is the trade-off between having all attributes as immutable or allowing some level of mutability for convenience. Finding the right balance can sometimes be subjective.
A Balanced Approach
You can adopt a hybrid approach where certain attributes are mutable, but others remain immutable. This approach requires careful consideration and often leads to more complex builder logic.
Wrapping Up
Implementing immutable embedded builders in Java can be rewarding, providing several benefits such as improved thread safety and easier code management. However, the challenges presented—like increased complexity, code duplication, and validation difficulties—require careful consideration.
By adopting good design practices, thorough documentation, and validation techniques, many of these challenges can be effectively mitigated. As you strive to write cleaner, more maintainable code, understanding these nuances will be key to successfully implementing immutable embedded builders.
For more information regarding Java immutability and builders, you can refer to the following resources:
- Effective Java by Joshua Bloch
- Java Design Patterns
Embrace the challenge and happy coding in your Java journey!