Mastering Hamcrest: Checking for Null Variables Simplified
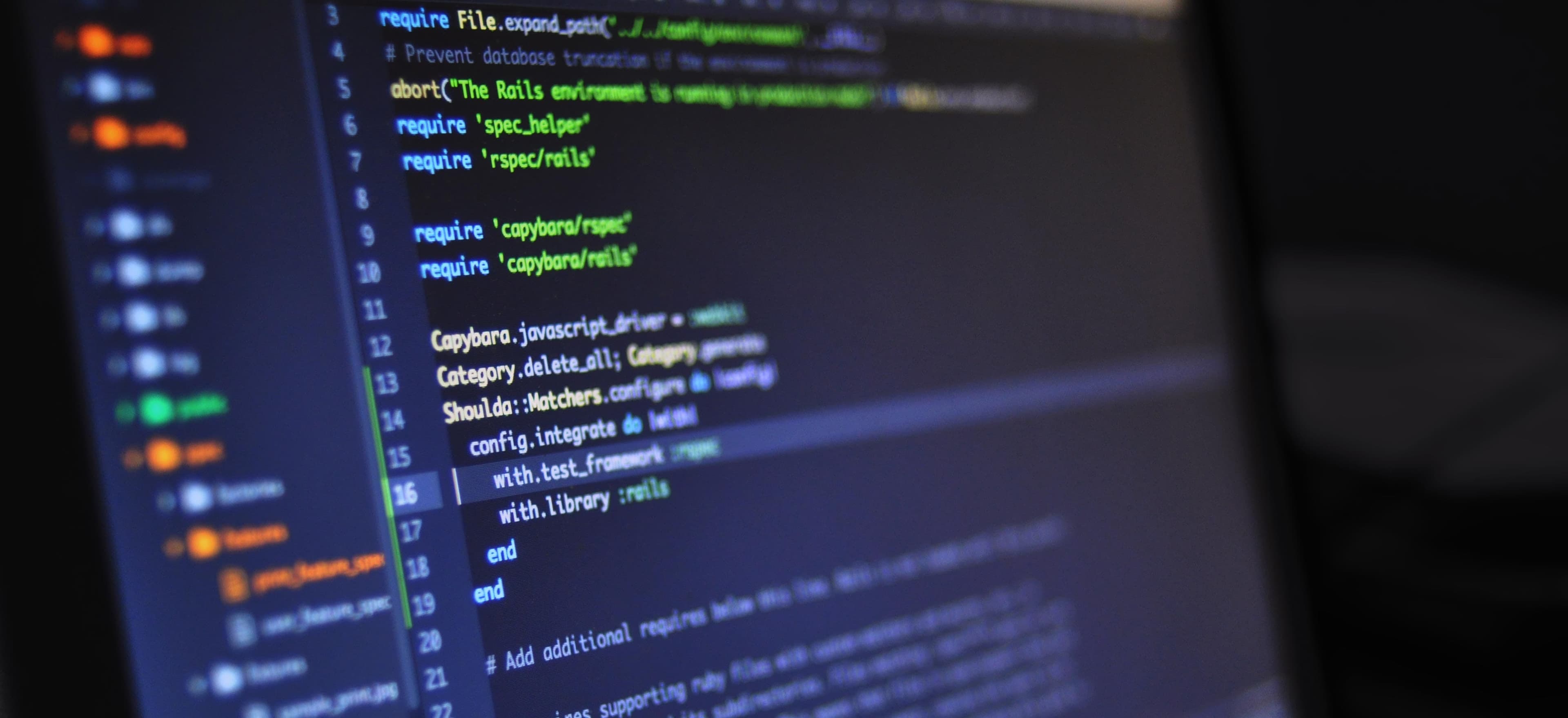
- Published on
Mastering Hamcrest: Checking for Null Variables Simplified
When developing in Java, especially in a world where unit testing is paramount, one essential library you should become familiar with is Hamcrest. While many know it for its expressive matchers, fewer understand its capabilities in simplifying the assertion of null variables. In this blog post, we will explore how to effectively use Hamcrest to check for null variables, simplifying your unit tests while making your code more readable and maintainable.
What is Hamcrest?
Hamcrest is a library designed for writing matcher objects allowing for declarative tests. It provides a framework that collaborates well with JUnit and enables us to write assertions in a more human-readable format. This helps to make test code easier to maintain, especially compared to traditional assertions.
By utilizing Hamcrest matchers, you can construct more complex assertions that can make your unit tests convey meaning more effectively. For example, instead of writing an assertion like this:
assertTrue(myObject == null);
You can use Hamcrest to create a more descriptive test:
assertThat(myObject, is(nullValue()));
This shift not only offers a better reading experience but also clarifies the intent of your assertions.
Setting Up Hamcrest
To get started with Hamcrest, you need to ensure that you have the library included in your project. If you're using Maven, you can easily add it as a dependency by including the following in your pom.xml
:
<dependency>
<groupId>org.hamcrest</groupId>
<artifactId>hamcrest-core</artifactId>
<version>2.2</version>
<scope>test</scope>
</dependency>
If you are using Gradle, add this to your build.gradle
:
testImplementation 'org.hamcrest:hamcrest-core:2.2'
After adding this dependency, you can start writing tests leveraging Hamcrest's capabilities.
Why Use Hamcrest for Null Checks?
When it comes to checking for null variables, clarity is key. The Hamcrest library provides matchers like nullValue()
and notNullValue()
that enhance the expressiveness of your tests. Here's why using Hamcrest for such checks is beneficial:
- Readability: Hamcrest helps convey intent. Other developers can quickly understand that you're checking if a variable is
null
or not. - Chaining: Hamcrest allows for chaining matchers, enabling you to create complex conditionals with ease.
- Customization: You can create custom matchers if you have specific needs that built-in matchers do not fulfill.
Basic Usage of Hamcrest for Null Checks
Understanding how to check for null variables using Hamcrest can significantly improve the clarity of your unit tests. Consider the following examples:
Example 1: Checking for Null Variable
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.nullValue;
@Test
public void testObjectIsNull() {
Object myObject = null; // Simulating a null object
assertThat(myObject, is(nullValue()));
}
In this test, the assertion clearly states that myObject
should be null. The is(nullValue())
matcher offers a natural way to express that expectation.
Example 2: Checking for Non-Null Variable
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.notNullValue;
@Test
public void testObjectIsNotNull() {
Object myObject = new Object(); // Simulating a non-null object
assertThat(myObject, is(notNullValue()));
}
Similarly, when you want to confirm that an object is not null, you can use is(notNullValue())
. This format promotes readability and enhances the expressiveness of your tests.
Combining Multiple Assertions
With Hamcrest, you can combine multiple conditions using logical operators, which can be useful when you need to check multiple variables at once.
Example 3: Combining Null and Non-Null Checks
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.*;
@Test
public void testMultipleObjects() {
Object nullObject = null;
Object notNullObject = new Object();
assertThat(nullObject, is(nullValue()));
assertThat(notNullObject, is(notNullValue()));
}
This test effectively checks both conditions within the same method while maintaining clarity.
Custom Matchers for Advanced Checks
While Hamcrest comes with many built-in matchers, sometimes you might need more specificity in your checks. In such cases, creating custom matchers can be useful.
Example 4: Custom Null Matcher
Here's how you can implement a simple custom matcher:
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.hamcrest.TypeSafeMatcher;
public class IsNullOrEmptyString extends TypeSafeMatcher<String> {
@Override
public void describeTo(Description description) {
description.appendText("a null or empty string");
}
@Override
protected boolean matchesSafely(String item) {
return item == null || item.isEmpty();
}
}
You can use it in your test like this:
@Test
public void testStringIsNullOrEmpty() {
String myString = ""; // Simulating an empty string
assertThat(myString, is(new IsNullOrEmptyString()));
}
This custom matcher checks if a string is either null or empty, thus enhancing the expressiveness of the test.
Final Thoughts
Using Hamcrest for null checks improves the quality of unit tests by enhancing their readability and expressiveness. With intuitive syntax and powerful matchers, Hamcrest can make your assertions succinct and meaningful.
To delve deeper into Hamcrest and explore more about its features, consider visiting the Hamcrest documentation for comprehensive examples and tutorials.
In the end, as you master Hamcrest, you will find that it simplifies numerous aspects of testing, allowing you to focus on the logic of your applications rather than the intricacies of your testing frameworks. By utilizing the clear and powerful assertions provided by Hamcrest, you help guarantee that your Java applications remain robust and reliable in the face of change. Happy testing!