Common Mockito Pitfalls: Avoiding Testing Mistakes
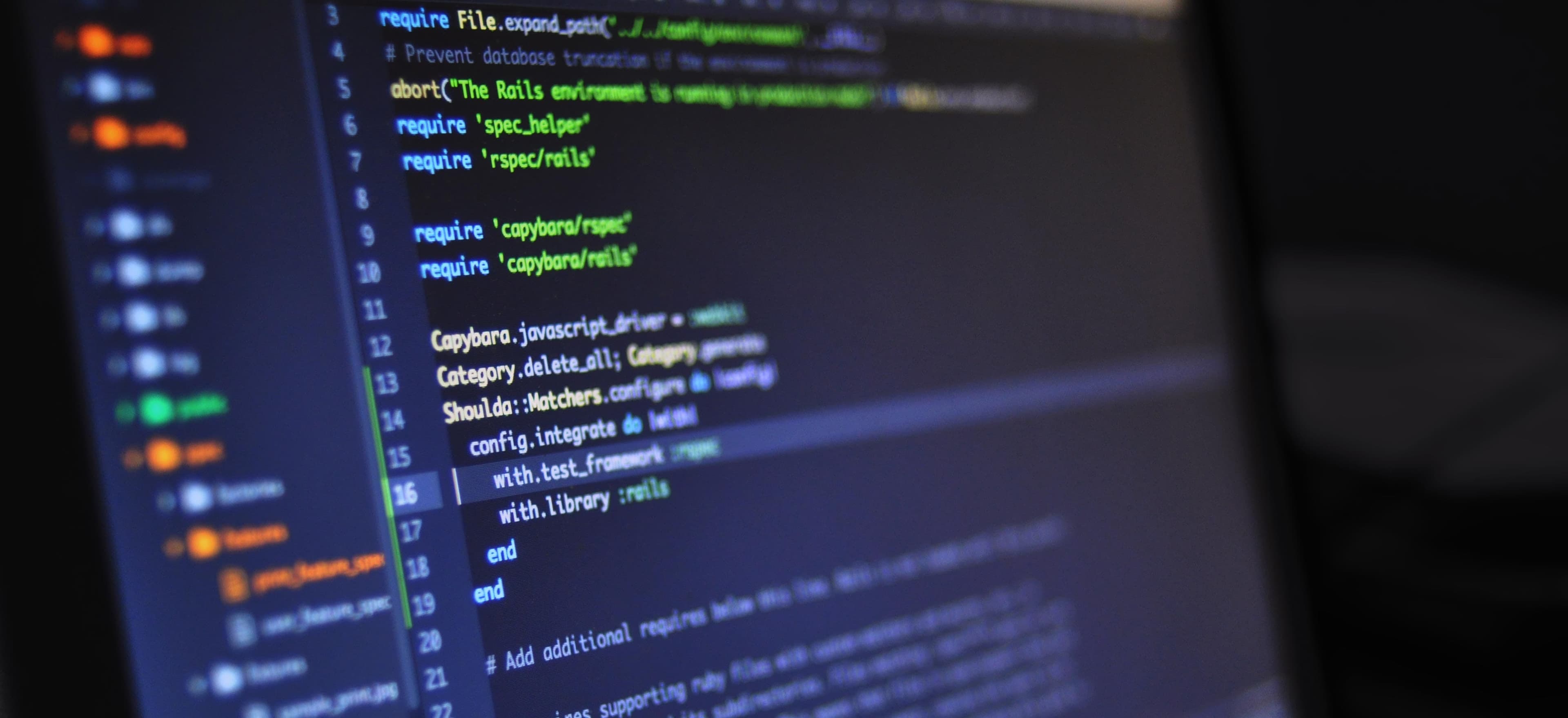
- Published on
Common Mockito Pitfalls: Avoiding Testing Mistakes
Mockito has emerged as a pivotal tool in the realm of Java testing, particularly for unit tests. It enables developers to adopt a more modular and clean approach to testing by simply mocking dependencies. However, despite its advantages, developers sometimes stumble upon common pitfalls that can lead to ineffective testing and maintainability issues. In this post, we will delve into these pitfalls, illustrate code snippets to demonstrate the issues, and provide best practices on overcoming them.
Table of Contents
- Understanding Mockito
- Common Pitfalls
- Pitfall 1: Overusing Mocks
- Pitfall 2: Ignoring Verification
- Pitfall 3: Incorrect Argument Matchers
- Pitfall 4: Not Resetting Mocks
- Best Practices
- Conclusion
Understanding Mockito
Mockito is a Java-based framework that allows you to create mock objects for testing. It helps in isolating the behavior of a single unit of code, ensuring that it works as expected without interference from its dependencies. This can lead to faster tests and more focused assertions.
Before we dive into the common pitfalls, let's take a closer look at a basic usage of Mockito.
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class SimpleMockitoExampleTest {
@Test
public void testMockito() {
// Create a mock object
List<String> mockedList = mock(List.class);
// Define behavior for the mock
when(mockedList.get(0)).thenReturn("Mockito");
// Use the mock
String result = mockedList.get(0);
// Verify the result
assertEquals("Mockito", result);
}
}
In this example, we create a mock for a List, define its behavior, and then verify it. However, simple as it seems, it is easy to run into trouble.
Common Pitfalls
As simplistic as Mockito may appear, developers often face challenges that can hinder their testing effectiveness. Let’s explore these pitfalls in detail.
Pitfall 1: Overusing Mocks
Problem
When developers tend to mock everything rather than actual collaborative objects, this can lead to tests that are disconnected from reality. It becomes difficult to reason about code when most dependencies are mocked.
Example
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public String findUser(int id) {
User user = userRepository.findById(id);
return user != null ? user.getName() : "Not Found";
}
}
If in our tests we mock the UserRepository
, we lose the context of the actual implementation and how the UserService
interacts with it.
Solution
Use mocks only when it is necessary. If you have a simple object that is already well-tested, rely on its actual implementation.
Pitfall 2: Ignoring Verification
Problem
Not verifying interactions with mocks can leave gaps in tests. If our code simply calls a mock but we forget to verify the expected interactions, our tests might pass without checking the real behavior.
Example
@Test
void testUserService() {
UserRepository userRepository = mock(UserRepository.class);
UserService userService = new UserService(userRepository);
userService.findUser(1);
// Missing verification
// verify(userRepository).findById(1); // This line should be included for proper verification
}
Solution
Always verify that your mocks are being interacted with as expected. For instance:
verify(userRepository).findById(1);
This line ensures that our service behaves correctly, truly utilizing the repository.
Pitfall 3: Incorrect Argument Matchers
Problem
Using argument matchers incorrectly can lead to ambiguous or misleading tests. Each time you use argument matchers, you must be aware of the context in which you are applying them.
Example
when(myMock.doSomething(anyString())).thenReturn("Hello");
However, if there are other overloaded methods that are similar, but with different parameter expectations, this can lead to confusion.
Solution
Make use of the appropriate matchers or the appropriate overload when expecting method calls. Be explicit in your tests to avoid any overlay.
when(myMock.doSomething("specificValue")).thenReturn("Hello");
Pitfall 4: Not Resetting Mocks
Problem
Failing to reset mocks between tests can lead to stale data and lead to flaky tests. When mocks persist state between test method executions, they can yield incorrect behaviors.
Example
@Test
void testOne() {
// some test logic
}
@Test
void testTwo() {
// might produce unexpected results due to state carried over
}
Solution
Use Mockito.reset()
in a @BeforeEach
or @AfterEach
method to ensure test isolation.
@BeforeEach
void setUp() {
Mockito.reset(myMock);
}
Best Practices
After outlining the pitfalls, let's look at best practices to ensure effective use of Mockito:
-
Limit Mocking: Mock only what you need to for the test. Prefer working with real objects whenever feasible.
-
Verify Interactions: Always verify that the expected interactions with mocks occurred. This helps in maintaining confidence in the tests.
-
Use Argument Matchers Wisely: Be careful while using argument matchers. Make sure they are appropriate for the method signature.
-
Reset Mocks: Reset mocks between tests to avoid side effects and state carryover.
-
Keep Tests Focused: Keep each test focused on a single behavior to avoid complexity.
To Wrap Things Up
Mockito is a powerful framework that simplifies unit testing in Java, yet it's easy to make mistakes that can stall progress. By understanding these common pitfalls and adhering to best practices, developers can maximize the effectiveness of their testing efforts.
For further reading on unit testing in Java, you might explore JUnit 5 Documentation or Mockito Documentation for deeper insights into creating robust test cases.
By avoiding these common pitfalls, you can create more reliable tests that contribute to the overall success of your software development efforts. Happy testing!