Mastering Database Joins in Java 14: A Step-by-Step Guide
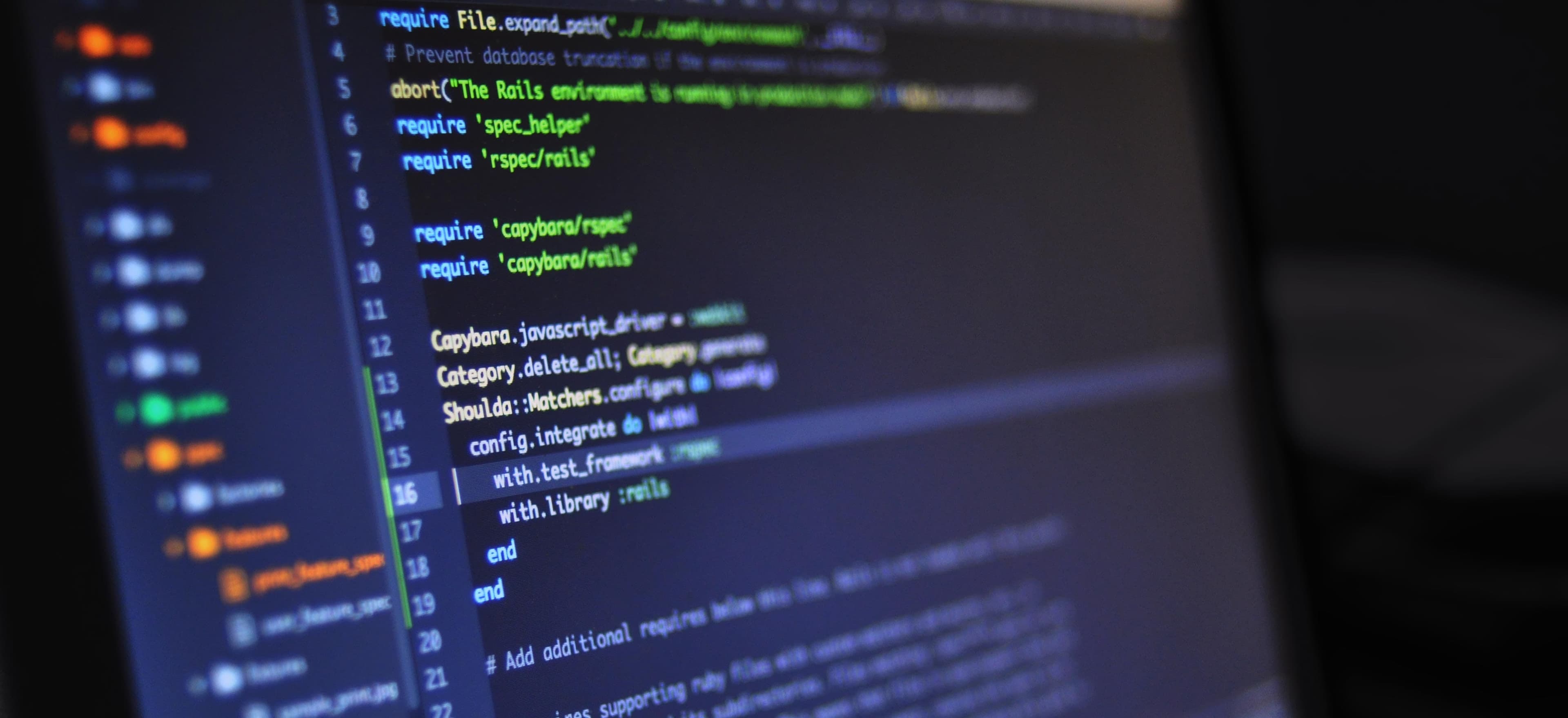
- Published on
Mastering Database Joins in Java 14: A Step-by-Step Guide
Database joins are fundamental concepts in relational database systems. They allow you to combine records from multiple tables based on a related column. In Java, mastering these joins is crucial for efficient data retrieval and manipulation. In this blog post, we will explore various types of joins, their usage within Java applications, and provide practical code examples to illustrate these concepts clearly.
Table of Contents
- Understanding Joins
- Types of Joins
- Inner Join
- Left Join
- Right Join
- Full Join
- Setting Up Java with JDBC
- Writing Join Queries
- Practical Examples
- Conclusion
1. Understanding Joins
At its core, a join is an operation that combines rows from two or more tables based on a related column. The power of joins allows for a more flexible and comprehensive retrieval of data, enabling applications to present a more complete picture of the database.
When you design an application that interacts with a relational database, familiarity with joins can reduce the complexity of your SQL queries and improve performance.
2. Types of Joins
There are several types of joins, each serving a unique purpose:
Inner Join
An inner join returns only the rows that have matching values in both tables. It's the most common type of join.
SELECT A.*, B.*
FROM employees A
JOIN departments B ON A.department_id = B.id;
Here, A
is an alias for the employees
table and B
for departments
. This query fetches all employee details along with their corresponding department details.
Left Join
A left join (or left outer join) returns all records from the left table and the matched records from the right table. If there is no match, the result is NULL
on the side of the right table.
SELECT A.*, B.*
FROM employees A
LEFT JOIN departments B ON A.department_id = B.id;
This retrieves all employees and their corresponding department information; those without a department will still be included, but with NULL
values for the department fields.
Right Join
Conversely, a right join (or right outer join) returns all records from the right table and the matched records from the left table.
SELECT A.*, B.*
FROM employees A
RIGHT JOIN departments B ON A.department_id = B.id;
This will fetch all departments, including those without assigned employees.
Full Join
A full join (or full outer join) combines the results of both left and right joins. It retrieves all records from both tables, filling in NULL
where there are no matches.
SELECT A.*, B.*
FROM employees A
FULL JOIN departments B ON A.department_id = B.id;
3. Setting Up Java with JDBC
To run join operations in Java, you typically use JDBC (Java Database Connectivity). This allows Java applications to connect to a database and execute SQL queries.
First, ensure you have the necessary JDBC driver for your database in your project’s classpath. For instance, if you're using MySQL, download the appropriate driver from MySQL JDBC Connector.
4. Writing Join Queries
Here is a simple method to execute a join query using JDBC in Java:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class DatabaseJoinExample {
private static final String URL = "jdbc:mysql://localhost:3306/your_database";
private static final String USER = "your_username";
private static final String PASSWORD = "your_password";
public static void main(String[] args) {
try (Connection connection = DriverManager.getConnection(URL, USER, PASSWORD);
PreparedStatement statement = connection.prepareStatement(
"SELECT A.*, B.* FROM employees A JOIN departments B ON A.department_id = B.id")) {
ResultSet results = statement.executeQuery();
while (results.next()) {
System.out.println("Employee: " + results.getString("A.name") +
", Department: " + results.getString("B.name"));
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
5. Practical Examples
Example 1: Inner Join
Let's modify the above example to demonstrate an inner join specifically:
String joinQuery = "SELECT A.name AS employee_name, B.name AS department_name " +
"FROM employees A " +
"INNER JOIN departments B ON A.department_id = B.id";
Here are some key takeaways:
- Aliases:
A
andB
make your SQL more readable. - Specific Columns: Selecting specific columns rather than
*
can enhance performance by returning only the necessary data.
Example 2: Left Join
You can also implement a left join to include employees without departments:
String leftJoinQuery = "SELECT A.name AS employee_name, B.name AS department_name " +
"FROM employees A " +
"LEFT JOIN departments B ON A.department_id = B.id";
The method remains consistent, but the resulting data will differ.
In Conclusion, Here is What Matters
Understanding and mastering database joins in Java is essential for developing efficient data-driven applications. From inner and outer joins to practical implementations through JDBC, you now possess a strong foundation to leverage this knowledge in your projects.
For further reading on JDBC, you might check the official Java JDBC documentation. Understanding joins can significantly enhance your database handling skills, ensuring your applications perform efficiently while delivering comprehensive data views.
Feel free to experiment with different join types in your applications, and watch how they shape the data you retrieve. Happy coding!