Overcoming Common Mistakes in Builder Design Pattern
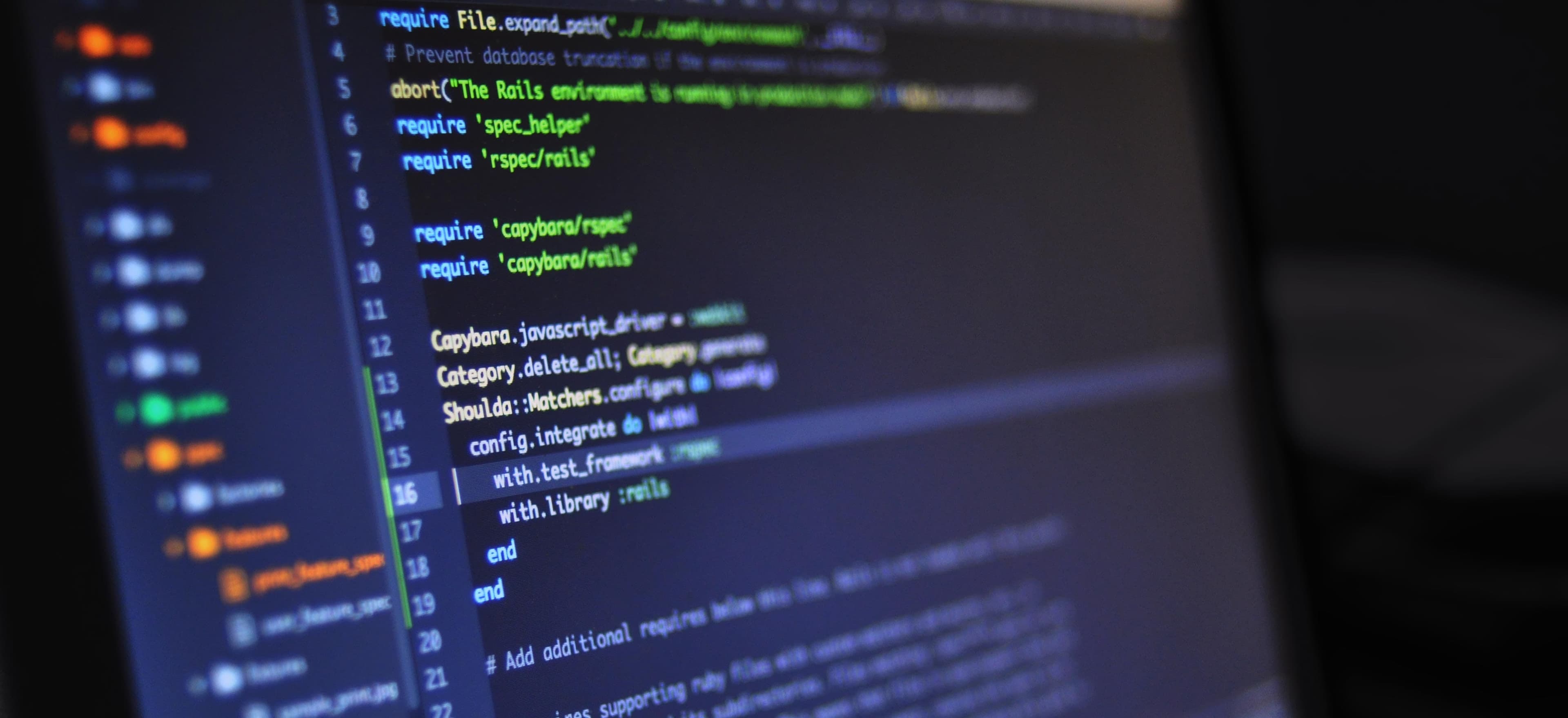
- Published on
Overcoming Common Mistakes in Builder Design Pattern
The Builder Design Pattern is a crucial architectural pattern that simplifies the construction of complex objects. It allows for a step-by-step approach to assembling parts. However, even seasoned developers make mistakes when implementing this pattern. In this blog post, we will explore common pitfalls and how to overcome them to ensure efficient and effective builder implementation in Java.
What is the Builder Pattern?
The Builder Pattern is part of the Gang of Four (GoF) Design Patterns and is used to tackle the issue of constructing complex objects. It provides a way to create objects with numerous parts without creating an over-engineered constructor. It achieves this goal by separating the construction of a complex object from its representation.
Example Scenario: Building a Pizza
Imagine you want to build a pizza with various toppings, sizes, and styles. Here, a pizza can be constructed using multiple parameters, making its creation complex.
Common Mistakes and Solutions
Mistake 1: Not Defining the Product's Complexity
Problem: One of the most frequent mistakes when using the Builder Pattern is failing to identify the complexities of the product you're building.
Solution: Clearly define the product's attributes and behaviors. This understanding guides the construction process.
Code Example:
public class Pizza {
private final String size;
private final boolean cheese;
private final boolean pepperoni;
private final boolean mushrooms;
private Pizza(Builder builder) {
this.size = builder.size;
this.cheese = builder.cheese;
this.pepperoni = builder.pepperoni;
this.mushrooms = builder.mushrooms;
}
public static class Builder {
private final String size;
private boolean cheese;
private boolean pepperoni;
private boolean mushrooms;
public Builder(String size) {
this.size = size;
}
public Builder cheese() {
this.cheese = true;
return this;
}
public Builder pepperoni() {
this.pepperoni = true;
return this;
}
public Builder mushrooms() {
this.mushrooms = true;
return this;
}
public Pizza build() {
return new Pizza(this);
}
}
@Override
public String toString() {
return "Pizza{" +
"size='" + size + '\'' +
", cheese=" + cheese +
", pepperoni=" + pepperoni +
", mushrooms=" + mushrooms +
'}';
}
}
In this example, the Pizza.Builder
class ensures that required attributes (like size) are defined, avoiding potential runtime errors related to undefined or invalid parameters.
Mistake 2: Ignoring Immutability
Problem: Another common mistake is not ensuring that the built object remains immutable. This can lead to complications when objects might be shared across threads.
Solution: Make your product class immutable. This will enhance thread safety and make it easier to reason about your code.
Code Commentary:
- Use
final
for fields. - Design the constructor to assign values only once via the builder.
- Avoid setter methods.
The Pizza
class shown above achieves immutability by declaring all fields as final
.
Mistake 3: Overcomplicating the Builder
Problem: Developers often introduce too many parameters or methods in the builder, making it cumbersome to use.
Solution: Keep the API clean. Only include the necessary methods that ease the construction process.
Remember: The intention of the builder is to simplify.
Code Example:
Pizza pizza = new Pizza.Builder("Large")
.cheese()
.pepperoni()
.build();
System.out.println(pizza);
As seen here, the fluent API allows for concise and readable object creation.
Mistake 4: Not Using a Fluent Interface
Problem: The builder pattern can lose its effectiveness when a fluent interface isn't employed, resulting in less readable code.
Solution: Implement a fluent interface by returning the Builder
object within its methods. This enhances the readability of the client code.
With our Pizza.Builder
, we return this
in each method, allowing method chaining.
Mistake 5: Skipping Validation
Problem: Sometimes, developers neglect to validate values before building the object, which can lead to unsatisfactory or invalid states.
Solution: Implement validation checks either in the builder or during the construction phase. This helps catch potential issues early.
Code Example:
public Pizza build() {
if (size == null || size.isEmpty()) {
throw new IllegalStateException("Pizza size must be specified");
}
return new Pizza(this);
}
By adding a simple validation check, we ensure clients cannot build pizzas without specifying a size.
Additional Enhancements
While the Builder Pattern can stand alone, it often works well in conjunction with other design patterns. Here are two recommendations:
- Prototype Pattern: This can be used to clone existing objects with minimal modification.
- Factory Pattern: Combine with Factory methods to produce builders selectively based on input parameters.
Resources for Further Learning
- Effective Java by Joshua Bloch – A must-read book for any Java developer, discussing best practices including builder patterns.
- Gang of Four Design Patterns – A comprehensive look at the fundamental design patterns, including the Builder Pattern.
Final Thoughts
Mastering the Builder Design Pattern unlocks the potential for easier maintenance and flexibility in your code. By addressing common mistakes like defining product complexity, ensuring immutability, avoiding overcomplication, using fluent interfaces, and enforcing validation, developers can create robust, reliable classes.
The Builder Pattern not only adds clarity but also adheres to design principles that encourage maintainable code. As you implement your own builders, remember to keep things simple—and don’t hesitate to explore related design patterns for extended architecture design.
Checkout our other articles