Why Your Bcrypt Salt Length Could Compromise Security
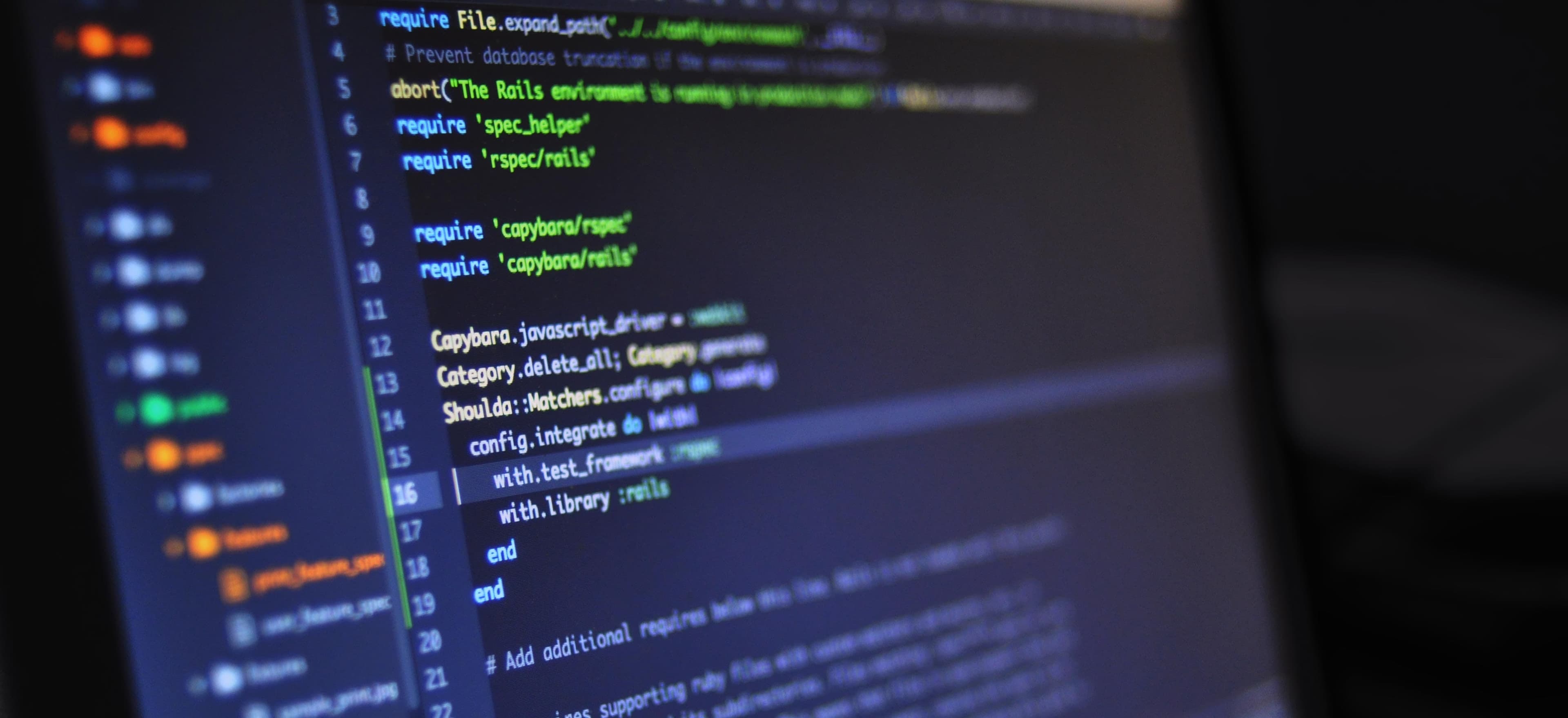
- Published on
Why Your Bcrypt Salt Length Could Compromise Security
In the realm of web application development, security is non-negotiable. One of the most essential components is password hashing, where Bcrypt has emerged as an industry standard. However, many developers overlook the nuances of Bcrypt, particularly when it comes to salt length. This post will discuss the importance of salt in password hashing, the role of Bcrypt, and why your choice of salt length could matter significantly.
Understanding Password Hashing
Before diving into Bcrypt and salt length, let’s briefly explore what password hashing is. Password hashing is the process of transforming a plain-text password into a scrambled format using a mathematical algorithm. This makes it nearly impossible to retrieve the original password, even if the hashed password is compromised.
Why Salt Matters
At its core, a salt is a random value that is used as an additional input to the hashing algorithm. Salts are crucial for several reasons:
-
Uniqueness: Salts ensure that two identical passwords do not produce the same hash. This is vital to thwart precomputed attacks like rainbow tables.
-
Enhanced Security: Adding randomness to the hash function increases the computational effort required to crack passwords.
-
Mitigation of Brute Force Attacks: By using salts, even if an attacker obtains a hashed password, they must also manage the salt to attempt to crack it, thus increasing the time needed for brute force attacks.
Bcrypt Overview
Bcrypt is a password-hashing function designed to be slow. Its slowness is intentional, as it helps defend against brute force attacks intended to guess passwords.
Key Features of Bcrypt:
- Uses a salt to protect against rainbow table attacks.
- The hashing algorithm is intentionally slow to reduce the risk of brute-force attacks.
- It allows the computational cost of hashing to be increased over time (by increasing the number of iterations).
Bcrypt Salt Length
Bcrypt generates a salt of a fixed length, which is 128 bits, or 16 bytes. This salt is then used along with the password to create the final hashed password. It's essential to understand how this salt functions in the hashing algorithm.
Here’s an illustrative Java code snippet that demonstrates how to use Bcrypt, including adequately handling the salt and hash:
import org.mindrot.jbcrypt.BCrypt;
public class BcryptExample {
// Method to hash a password
public static String hashPassword(String plainPassword) {
// Generate a salt with a default workload (cost) of 10
String salt = BCrypt.gensalt(10);
// Create the hash using the password and the generated salt
return BCrypt.hashpw(plainPassword, salt);
}
// Method to check if the password matches the hash
public static boolean checkPassword(String plainPassword, String hashedPassword) {
// Check the password against the stored hash
return BCrypt.checkpw(plainPassword, hashedPassword);
}
public static void main(String[] args) {
String myPassword = "securePassword123";
// Hash the password
String hashed = hashPassword(myPassword);
// Verify the password
boolean isMatch = checkPassword(myPassword, hashed);
System.out.println("Does the password match? " + isMatch);
}
}
Commentary on Salt Length in Bcrypt
In the code example above, the salt is automatically generated by Bootstrap, ensuring it meets the necessary length and complexity. However, using a custom salt length or an incorrect implementation can weaken the hash. Let’s break down why salt length is crucial.
-
Shorter Salts are Easier to Predict: If you choose to define your salt lengths and go below Bcrypt's default of 128 bits, you risk making it too similar to common hashes, thus increasing the likelihood of successful brute-force attacks.
-
Cryptographic Strength: Bcrypt employs a secure random number generator to create a salt of 128 bits. A shorter salt may not provide sufficient uniqueness, increasing exposure to attacks.
-
Risk of Collision: With a smaller salt space, there is a higher chance of hash collisions, where different inputs can lead to identical hash outputs, making it easier for an attacker to exploit this.
Recommendations for Secure Usage
-
Always Use Bcrypt's Default Salt: While it might be tempting to roll your own implementations or choose different lengths, it's best to stick with Bcrypt's configuration. The hashing algorithm was developed for strength and effectiveness.
-
Adjust Workload Cost: Although this blog primarily focuses on salt, your security should also depend on the workload cost (i.e., the number of rounds). The higher the number of rounds, the more computation is required, making it difficult for attackers to derive hashes.
-
Stay Updated: Security is an ever-evolving field. Regularly review your implementations, keeping pace with the latest recommendations from the community.
-
Layer Your Security: Relying on password hashing alone is not enough. Integrate additional security layers like rate limiting, account lockout mechanisms, and strong user authentication practices.
The Bottom Line
The security of your application relies heavily on how you manage user passwords. While Bcrypt provides a robust hashing mechanism with built-in salt generation, it is of utmost importance to understand the implications of salt length. Inadequate salt lengths can lead to vulnerabilities that attackers can exploit.
Following best practices in hashing, including using Bcrypt's default settings and maintaining a focus on other aspects of security, will serve to fortify your web applications against the ongoing battle of security threats.
For further reading on password hashing and security best practices, check out OWASP’s Password Storage Cheat Sheet and Bcrypt Documentation.
By understanding and adhering to these principles regarding Bcrypt and salt lengths, you ensure that your applications remain secure, and user data stays protected.