Mastering JUnit 5 for Clear Executable Specifications
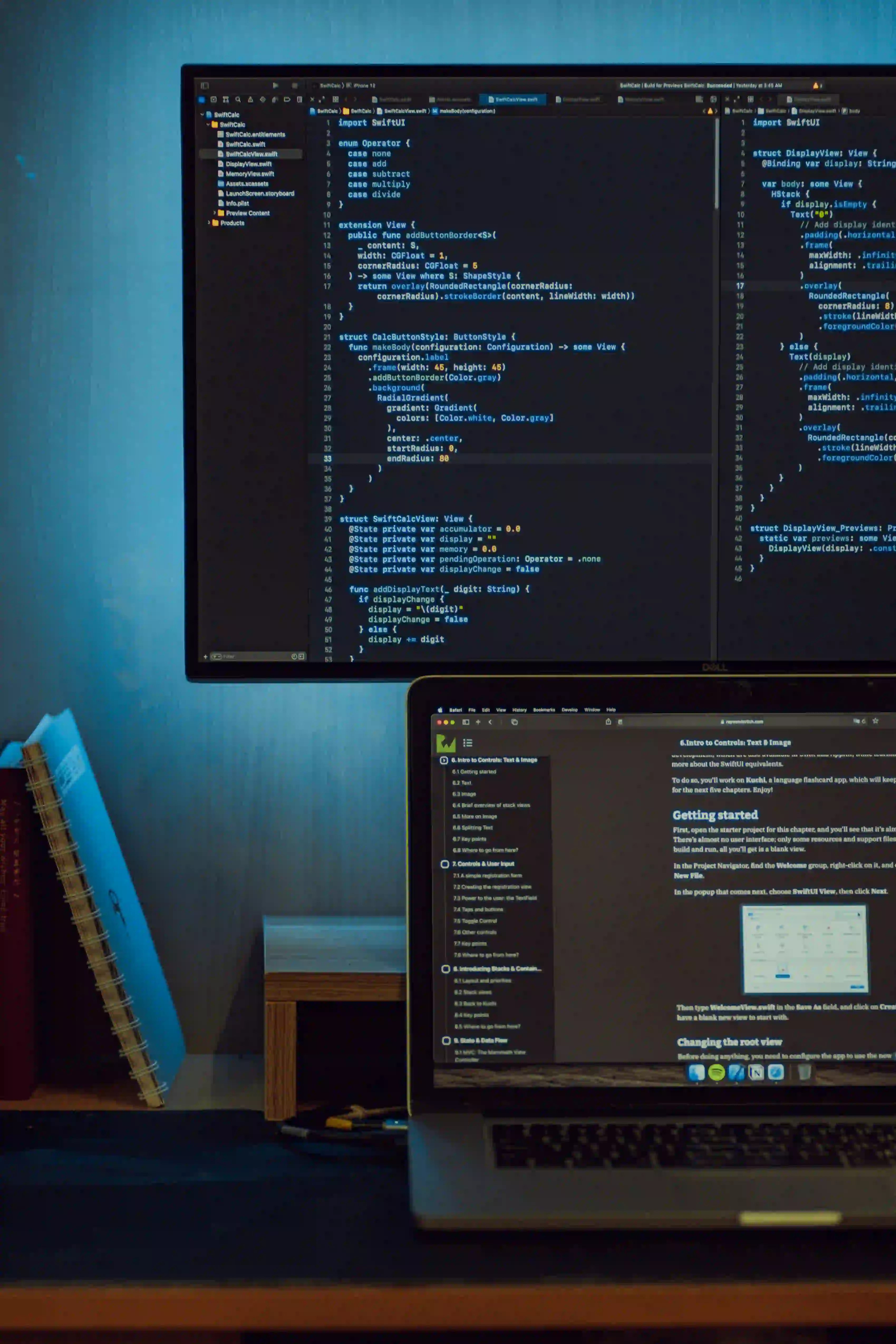
Mastering JUnit 5 for Clear Executable Specifications
When it comes to Java unit testing, JUnit remains one of the most popular frameworks. With the introduction of JUnit 5, developers gained access to a plethora of new features that enhance testing capabilities and improve the clarity of executable specifications. This blog post will guide you through mastering JUnit 5, helping you write tests that are not only effective but also easy to understand.
What is JUnit 5?
JUnit 5 is a major upgrade over its predecessor, JUnit 4. It introduced a modular architecture, allowing for better extensibility and integration with various tools. Unlike JUnit 4, JUnit 5 is composed of three main components:
- JUnit Platform: Provides a foundation for launching testing frameworks on the JVM.
- JUnit Jupiter: Introduces new programming models and extension points.
- JUnit Vintage: Allows older, JUnit 3 and 4 tests to run on the JUnit 5 platform.
In this post, we will focus primarily on JUnit Jupiter, where most exciting features are found.
Why Use JUnit 5?
JUnit 5 enhances the testing experience in multiple ways:
- Annotations: New, intuitive annotations like
@Test
,@BeforeEach
, and@AfterEach
make writing tests straightforward. - Parameterization: JUnit 5 makes it easy to create parameterized tests using
@ParameterizedTest
. - Conditionally Executable Tests: With
@EnabledIf
and@DisabledIf
, you can control test execution based on specific conditions. - Improved Assertions: The
Assertions
class now provides more expressive and flexible methods.
Overall, these features lead to clearer and more maintainable test code.
Getting Started with JUnit 5
First, you’ll need to set up your project. If you're using Maven, add the following dependencies to your pom.xml
:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.9.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.9.0</version>
<scope>test</scope>
</dependency>
For Gradle users, add the following lines to your build.gradle
:
testImplementation 'org.junit.jupiter:junit-jupiter-api:5.9.0'
testRuntimeOnly 'org.junit.jupiter:junit-jupiter-engine:5.9.0'
Creating Your First Test
Let’s create a simple test class to understand the basic structure. Consider a method that adds two numbers together.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Now, let’s create a JUnit 5 test for this method:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
void testAdd() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result, "The add method should correctly add two numbers.");
}
}
Breaking Down the Test
- Test Annotation: The
@Test
annotation indicates that the method is a test case. - Assertions: The
assertEquals
statement checks if the actual result matches the expected value, enhancing readability. - Failure Message: The message in the assertion provides context, making debugging easier.
Advanced Features of JUnit 5
Parameterized Tests
Parameterized tests allow you to run the same test with different parameters, increasing test coverage efficiently.
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.Arguments;
import org.junit.jupiter.params.provider.MethodSource;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
static Stream<Arguments> additionProvider() {
return Stream.of(
Arguments.of(1, 2, 3),
Arguments.of(2, 3, 5),
Arguments.of(5, 5, 10)
);
}
@ParameterizedTest
@MethodSource("additionProvider")
void testAdd(int a, int b, int expected) {
Calculator calculator = new Calculator();
assertEquals(expected, calculator.add(a, b), "The add method should add the numbers.");
}
}
Why Use Parameterized Tests?
Parameterized tests are a great way to ensure that your methods handle various inputs, clarifying executable specifications without duplicating code. This contributes to maintaining a DRY (Don't Repeat Yourself) codebase.
Conditional Test Execution
JUnit 5 provides flexible conditions under which tests are executed.
import org.junit.jupiter.api.condition.EnabledIfSystemProperty;
public class SystemPropertyTest {
@Test
@EnabledIfSystemProperty(named = "env", matches = "prod")
void testOnlyRunsInProd() {
// Test code that should only run in production.
}
}
Why Conditional Execution?
This feature is invaluable for integration tests or tests that depend on external systems. Conditional execution allows controlling test scenarios based on the environment, ensuring tests only run when applicable.
Using Extensions
JUnit 5 introduces an extension model that allows you to add functionality to your tests via annotations.
Example of a Custom Extension
import org.junit.jupiter.api.extension.AfterEachCallback;
import org.junit.jupiter.api.extension.ExtensionContext;
public class ResetDatabaseExtension implements AfterEachCallback {
@Override
public void afterEach(ExtensionContext context) {
// Logic to reset the database after each test
}
}
Now, you can apply the extension to your tests:
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
@ExtendWith(ResetDatabaseExtension.class)
public class DatabaseTest {
@Test
void testDatabaseFunctionality() {
// Test code here
}
}
Why Use Extensions?
Extensions allow for reusability and modularity in test code. They help encapsulate cross-cutting concerns such as resource management, providing cleaner and more maintainable test classes.
Lessons Learned
Mastering JUnit 5 opens the door to writing effective, clear, and executable specifications in your tests. By utilizing its advanced features like parameterized tests, conditional test execution, and extensions, you can achieve higher coverage and greater clarity in your unit tests.
For further reading on JUnit 5 capabilities, consider visiting the official JUnit 5 user guide or the JUnit 5 GitHub repository.
Integrate JUnit 5 into your development workflow, and watch the quality of your code improve alongside the maintainability of your test cases.
Happy Testing!