How to Efficiently Check if an Array is Empty or Null
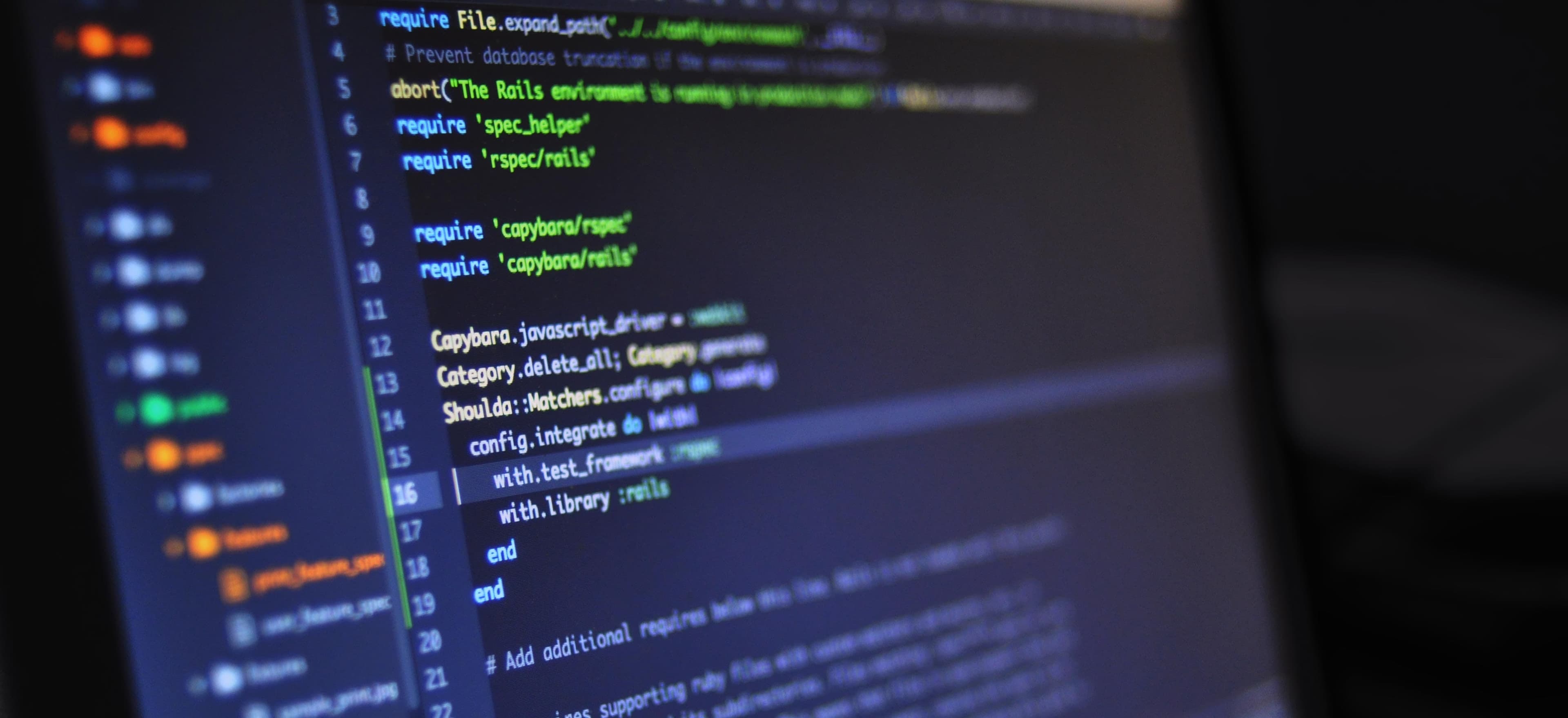
- Published on
How to Efficiently Check if an Array is Empty or Null in Java
In Java, arrays are fundamental data structures that hold fixed-size collections of elements of the same type. They can be an excellent tool for various programming tasks, but before you dive into manipulating an array, it's crucial to ensure that it is neither null nor empty. In this blog post, we'll explore efficient ways to check if an array is empty or null, understand why these checks are important, and provide code examples with commentary.
Why Check for Null or Empty Arrays?
Before heading into the implementation, it’s essential to discuss why checking for null or empty arrays is crucial:
- Avoiding NullPointerExceptions: Attempting to access or iterate through a null array will throw a
NullPointerException
, leading to unexpected application crashes. - Logic Errors: An empty array is still a valid data structure. If you treat it as null, the logic of your code could become skewed.
- Performance: Pre-checking can save unnecessary processing time, especially in complex algorithms.
Understanding these aspects will ground you in best programming practices.
How to Check for Null or Empty Arrays
Method 1: Using Basic Conditional Statements
The simplest way to check if an array is null or empty is by employing basic conditional statements. Here’s how you can do it:
public boolean isArrayEmptyOrNull(Object[] array) {
return array == null || array.length == 0;
}
Explanation:
array == null
: This checks whether the array reference itself is null.array.length == 0
: This checks whether the array has any elements. An empty array has a length of zero.
Method 2: Using Optional Class
If you're using Java 8 or later, the Optional
class can provide a more elegant approach:
import java.util.Optional;
public boolean isArrayEmptyOrNull(Object[] array) {
return Optional.ofNullable(array)
.map(arr -> arr.length > 0)
.orElse(false);
}
Explanation:
Optional.ofNullable(array)
: This creates anOptional
object. If the array is null, it returns an emptyOptional
.map(arr -> arr.length > 0)
: If the array isn't null, it checks the length.orElse(false)
: Returns false if the array is null or empty.
Method 3: Leveraging Java Streams
Another modern way to check for empty arrays involves Java Streams. Here's how you can do it:
import java.util.Arrays;
public boolean isArrayEmptyOrNull(Object[] array) {
return array == null || Arrays.stream(array).findAny().isEmpty();
}
Explanation:
Arrays.stream(array)
: Converts the array into a stream.findAny()
: Returns anOptional
describing any element of the stream, if any are present.isEmpty()
: Checks if the stream contains any elements. Using this method could be beneficial if you want to extend the functionality later on, as streams offer powerful processing capabilities.
Method 4: Using Apache Commons Lang
If you are working on a project where you are already using external libraries, Apache Commons Lang provides utility methods that can make this check even simpler:
import org.apache.commons.lang3.ArrayUtils;
public boolean isArrayEmptyOrNull(Object[] array) {
return ArrayUtils.isEmpty(array);
}
Explanation:
ArrayUtils.isEmpty(array)
: This utility method checks whether the array is null or has a length of zero, consolidating the checks into a single method.
Performance Considerations
The time complexity for checking if an array is null or empty is O(1). This means that no matter how large your array is, the operations require a constant time regardless of the size. However, using streams can introduce some overhead, making it marginally less optimal than a straightforward conditional check or using library methods.
Be mindful of the following guidelines:
- Favor simplicity and readability in most cases, particularly for common checks like this.
- Use more complex approaches only if you need additional functionality.
Summary
In this post, we explored various methods to efficiently check if an array is empty or null in Java. Here’s a quick recap:
- Basic Conditionals: Simple and effective for quick checks.
- Optional Class: A modern approach that adds some elegance.
- Java Streams: Offers powerful capabilities if you plan to extend functionality later.
- Apache Commons Lang: A handy utility when working with external libraries.
Each method has its strengths, and your choice should depend on the context and requirements of your project.
For further reading, you might find these resources useful:
By following best practices and employing efficient checking methods, you’ll create code that is both robust and clean. Happy coding!
Checkout our other articles