Maximize Your Efficiency: Switch to Groovy for Unit Testing
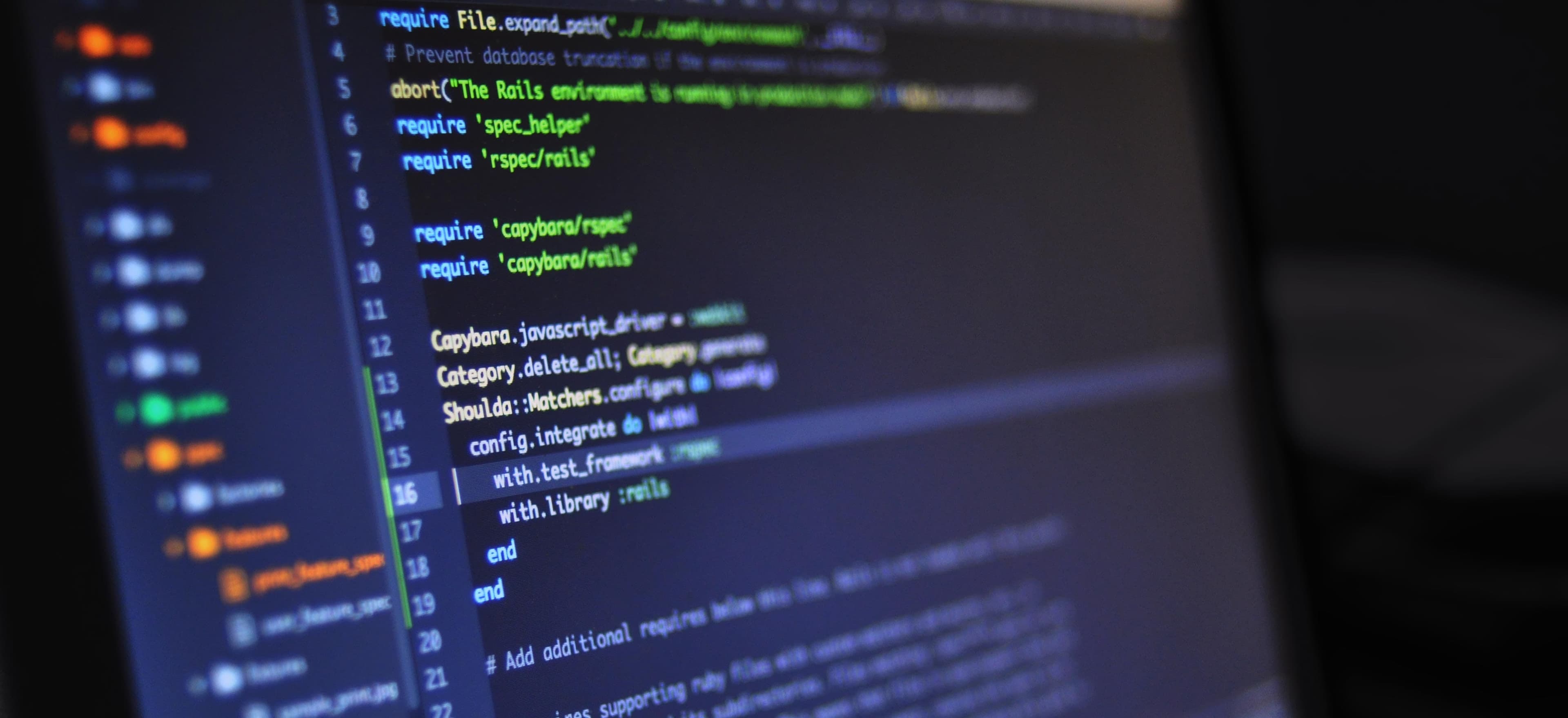
- Published on
Maximize Your Efficiency: Switch to Groovy for Unit Testing
Unit testing is a critical aspect of software development that ensures the functionality of individual components. While Java has been the foundation for creating robust unit tests using frameworks like JUnit, developers often overlook Groovy as a powerful alternative. In this blog post, we'll explore why switching to Groovy for unit testing can significantly enhance your productivity and make testing more enjoyable.
Why Groovy?
1. Dynamic Language Benefits
Groovy is a dynamic language built on the Java platform. This means that while it draws upon the solidity of Java, it introduces elements that can streamline coding and testing. One of Groovy's standout features is its concise syntax, which helps reduce boilerplate code.
Example: Less Boilerplate Code
In Java, a simple unit test may look like this:
import static org.junit.Assert.*;
import org.junit.Test;
public class MyTest {
@Test
public void testAddition() {
int result = 1 + 1;
assertEquals(2, result);
}
}
In Groovy, the equivalent test shrinks down to:
import spock.lang.*
class MyTest extends Specification {
def "test addition"() {
expect:
1 + 1 == 2
}
}
Here, Groovy's syntax allows you to express the same logic more succinctly. This improves readability and maintainability, which are crucial for effective unit testing.
2. Built-in Features
Groovy comes equipped with an array of features that improve unit test writing capabilities. For instance, it allows for closures, which are blocks of code that can be executed later. This means you can define behavior without the need for boilerplate classes or methods.
Example: Utilization of Closures
Using closures, we can create more flexible tests. Instead of hard-coding values, we can delegate logic to functions:
def calculate(def closure) {
closure()
}
assert calculate { 2 + 2 } == 4
This level of abstraction can make tests not only cleaner but also reusable.
Groovy Testing Frameworks
1. Spock Framework
One of the most compelling reasons to adopt Groovy for unit testing is the Spock framework. Spock's DSL (Domain Specific Language) makes writing tests enjoyable while maintaining clarity.
Features of Spock
- Descriptive syntax: Tests can often read almost like plain English.
- Data-driven testing: Easily create tests that run with multiple sets of data.
Example: Data-driven Testing in Spock
Imagine you need to test a method that checks whether a number is even. You can run the same test with different inputs as follows:
import spock.lang.*
class EvenCheckerSpec extends Specification {
def "should determine if a number is even"() {
expect:
isEven(input) == expected
where:
input | expected
2 | true
3 | false
4 | true
5 | false
}
boolean isEven(int number) {
return number % 2 == 0
}
}
In this example, a single test method can validate multiple cases, drastically reducing redundancy.
2. Geb Framework
When considering integration testing alongside unit testing, Geb should be on your radar. Geb combines the power of Groovy with WebDriver, allowing seamless browser interaction in tests.
Example: Simple Geb Test
import geb.spock.GebSpec
class SampleGebTest extends GebSpec {
def "check title of Google"() {
when:
go "http://www.google.com"
then:
title == "Google"
}
}
This is a straightforward test that navigates to Google and checks the title. It's simple yet effective. The use of Geb within the Groovy environment enhances integration testing capabilities.
Advantages of Groovy Testing
-
Rapid Development: You’ll notice an increase in speed, allowing you to focus on your application logic rather than verbose test code.
-
Enhanced Readability: The less-cluttered syntax in Groovy makes tests more accessible to read and understand, even for newcomers.
-
Less Configuration: Groovy tests often require fewer annotations and boilerplate, cutting down on configuration time.
Groovy with Existing Java Libraries
One significant point to consider is that Groovy is fully interoperable with Java. This means you can still utilize existing Java libraries while writing your tests in Groovy.
Example: Using JUnit in Groovy
If you have JUnit tests already, you can easily integrate Groovy like this:
import static org.junit.Assert.assertEquals
import org.junit.Test
class JUnitGroovyTest {
@Test
void testAddition() {
assertEquals(2, 1 + 1)
}
}
You can see that there's no limitation in leveraging Java's robust libraries while enjoying Groovy's convenience.
Transitioning to Groovy: Steps to Consider
Switching to Groovy does require some considerations. It’s essential to establish a development environment conducive to Groovy programming.
1. Gradle Integration
If you're using Gradle, integrating Groovy into your project is seamless. Simply add the following to your build.gradle
:
dependencies {
testImplementation 'org.spockframework:spock-core:2.0-groovy-3.0'
testImplementation 'org.codehaus.groovy:groovy-all:3.0.3'
}
This will ensure that you have the necessary dependencies to start writing tests with Groovy.
2. Training and Resources
While Groovy is easy to pick up, consider utilizing resources such as Groovy Documentation and online courses to familiarize yourself and your team with its nuances.
My Closing Thoughts on the Matter
Switching to Groovy for unit testing may require an adjustment period, but the gains in efficiency, clarity, and joy in writing tests are worth the effort. With frameworks like Spock and Geb, you can streamline your testing processes and improve the overall quality of your software.
Unit tests are a safety net, and Groovy equips you to create that net more effectively. The dynamic capabilities, coupled with seamless Java integration, make it a powerful tool in every developer’s arsenal.
By embracing Groovy, you are not just maximizing your efficiency—you are taking a significant step toward a better development experience.
Further Reading
As you transition into the groovy world of testing, may your test cases become simpler and your debugging sessions shorter. Happy testing!
Checkout our other articles