Unlocking JVM Performance: Key Tricks You Need to Know
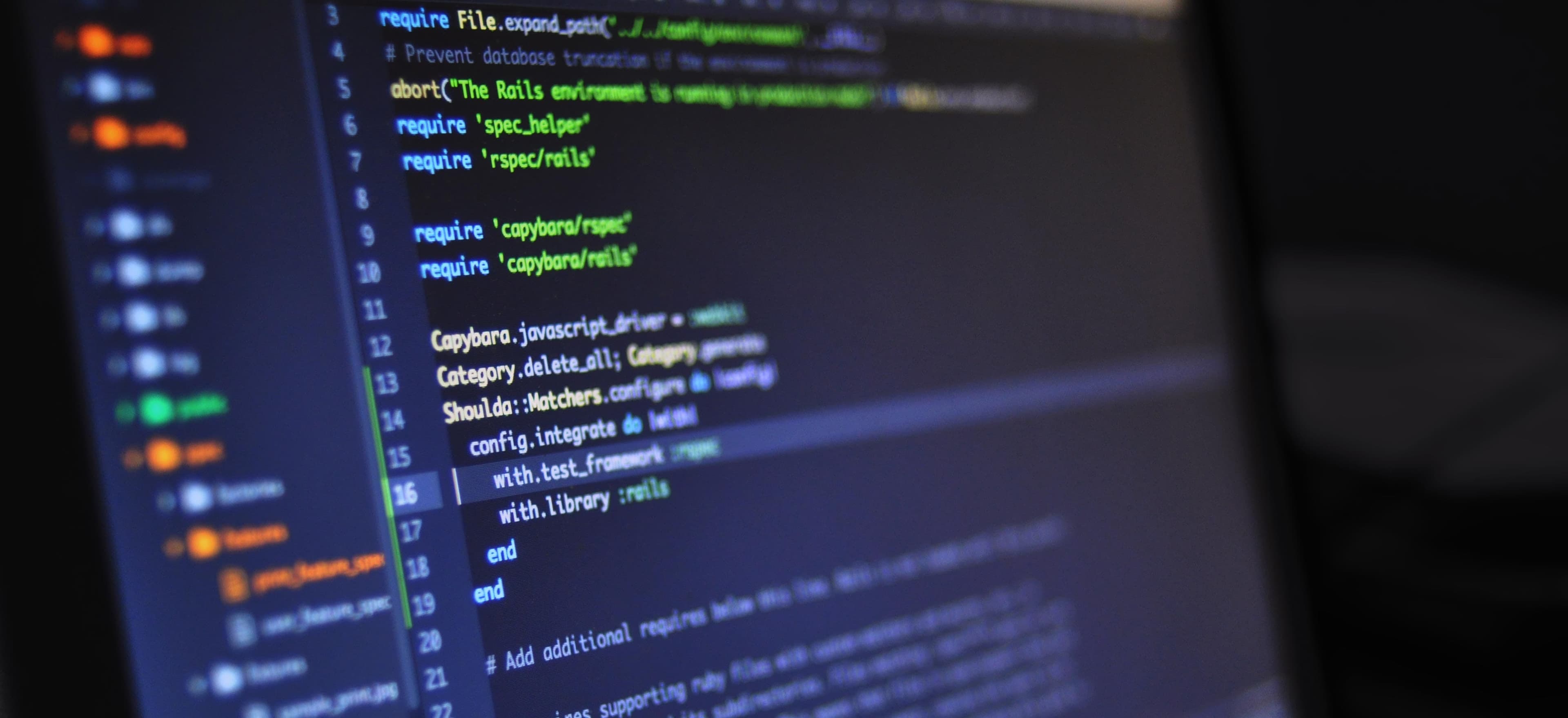
- Published on
Unlocking JVM Performance: Key Tricks You Need to Know
When it comes to optimizing JVM performance, developers often throw around buzzwords without comprehension. However, getting the most out of your Java Virtual Machine (JVM) can dramatically affect your application's performance. In this blog post, we will explore practical tricks to fine-tune your JVM settings, optimize garbage collection, and improve runtime performance.
Understanding Java and the JVM
Before we dive into the tricks, let's quickly understand what the JVM is. The JVM is an abstract computing machine that enables Java bytecode to be executed across platforms. It provides key features like garbage collection, memory management, and thread synchronization while keeping the underlying hardware abstracted.
Importance of Performance Tuning
Performance tuning is essential for developing applications that are not only functional but also efficient. Poorly performing applications can lead to longer response times, higher resource consumption, and ultimately, a negative user experience. To prevent this, developers need to focus on optimizing JVM performance.
Key Tricks to Improve JVM Performance
Let’s break down essential tricks that allow you to maximize your JVM performance.
1. Optimize Garbage Collection (GC)
Garbage collection is one of the most crucial aspects of JVM performance. An inefficient GC can cause application pauses that affect responsiveness.
Choosing the Right Garbage Collector
Java provides several GC algorithms, each with specific advantages. Selecting the right one based on your application needs can significantly enhance performance.
For example, the G1 Garbage Collector is designed for applications with large heaps and comes with predictability in pause times:
-XX:+UseG1GC
Why: Using G1 GC helps minimize the pause time during GC cycles, making it suitable for applications requiring low-latency responses.
You can measure GC performance using the following JVM flags to generate GC logs:
-verbose:gc -Xloggc:gc.log -XX:+PrintGCDetails -XX:+PrintGCTimeStamps
Why: This setup provides insight into GC behavior, allowing for effective tuning based on collected data.
2. Fine-Tuning Heap Memory
The heap is where Java objects are allocated, and managing heap size is crucial for performance.
Setting Heap Size
You can control the size of your heap memory using the -Xms
(initial size) and -Xmx
(maximum size) options:
-Xms512m -Xmx4g
Why: The initial allocation of heap (-Xms
) should ideally match your application's typical workload to minimize resizing during runtime. The maximum heap size (-Xmx
) should fit the server's capacity while leaving room for other operations.
Monitor Heap Utilization
Regularly monitor your heap utilization and adjust accordingly. Use tools like VisualVM or JConsole to ascertain how much memory your application is using.
3. Use the Right Java Version
Java versions matter. Each new version tends to improve performance incrementally and add new features that can help your application run better.
Stay Updated
An application crafted in Java 8 may not perform as well as the same application running on Java 17 due to many behind-the-scenes improvements and optimizations.
Why: Always test your application with the latest Long-Term Support (LTS) version for the best performance.
4. Optimize Thread Usage
Threads are fundamental to concurrent Java applications. However, excessive or insufficient thread count can lead to performance bottlenecks.
Limit the Threads Wisely
Set appropriate thread pool sizes based on your application’s workload and the number of concurrent users:
ExecutorService executorService = Executors.newFixedThreadPool(10);
Why: A fixed thread pool size prevents thread creation overhead and ensures that you do not exhaust system resources.
Monitor CPU Usage
Tools like Java Mission Control can help identify threads that consume excessive CPU time, allowing you to make data-driven decisions about thread optimization.
5. Enable JIT Compilation
The Just-In-Time (JIT) compiler compiles your bytecode into native machine code during runtime, improving performance significantly through optimization.
Enable Tiered Compilation
This feature allows the JVM to use a multi-level compilation strategy for methods, producing optimized code based on usage:
-XX:+TieredCompilation
Why: It leads to faster startup times while continuing to optimize performance over time as the application runs.
6. Monitor and Diagnose with Profilers
Regular profiling can bring to light issues that adversely impact performance.
Use Profilers Like VisualVM or YourKit
You can track CPU, memory usage, and thread activity, which facilitates identifying bottlenecks in your application.
Why: Profiling provides a clearer picture about where optimizations are needed, making it easier to focus your efforts.
7. Optimize I/O Operations
Input/output operations can often be a bottleneck. Optimize file reads/writes and database queries to improve performance.
**Asynchronous I/O}
Consider using asynchronous I/O operations for reading/writing files or querying databases. Using java.nio
can provide better performance than traditional java.io
.
Path path = Paths.get("example.txt");
AsynchronousFileChannel channel = AsynchronousFileChannel.open(path, StandardOpenOption.READ);
Why: This method allows your application to perform other tasks while waiting for the completion of I/O operations, hence improving responsiveness.
8. Avoid Unnecessary Object Creation
Frequent object creation can lead to GC overhead, thus impacting performance.
Use Object Pools
In scenarios where object creation and destruction are high, consider using object pooling to reuse existing objects.
public class ObjectPool {
private final List<MyObject> availableObjects = new ArrayList<>();
public MyObject acquireObject() {
if (availableObjects.isEmpty()) {
return new MyObject(); // create a new object if pool is empty
}
return availableObjects.remove(availableObjects.size() - 1);
}
public void releaseObject(MyObject obj) {
availableObjects.add(obj); // put the object back into the pool
}
}
Why: This reduces the overhead of object creation and takes advantage of memory already allocated, minimizing GC frequency.
Wrapping Up
Optimizing the performance of your Java applications running on the JVM requires a combination of strategies. By focusing on garbage collection, heap management, threading, Java versioning, and I/O operations, you can significantly enhance your application's speed and responsiveness. Monitoring tools and profilers provide invaluable insights that guide your tuning.
For further in-depth knowledge on Java performance tuning, check out the Official Java Documentation on garbage collection or refer to the Oracle Java Performance Tuning Guide. By understanding these concepts and applying them, you not only improve your application's performance but also enhance its usability - a win-win for you as a developer and your users.
Ensure to practice these performance optimizations and monitor your application's behavior in production to keep it running smoothly. Happy coding!
Checkout our other articles