Understanding Type Erasure in Java Generics: A Deep Dive
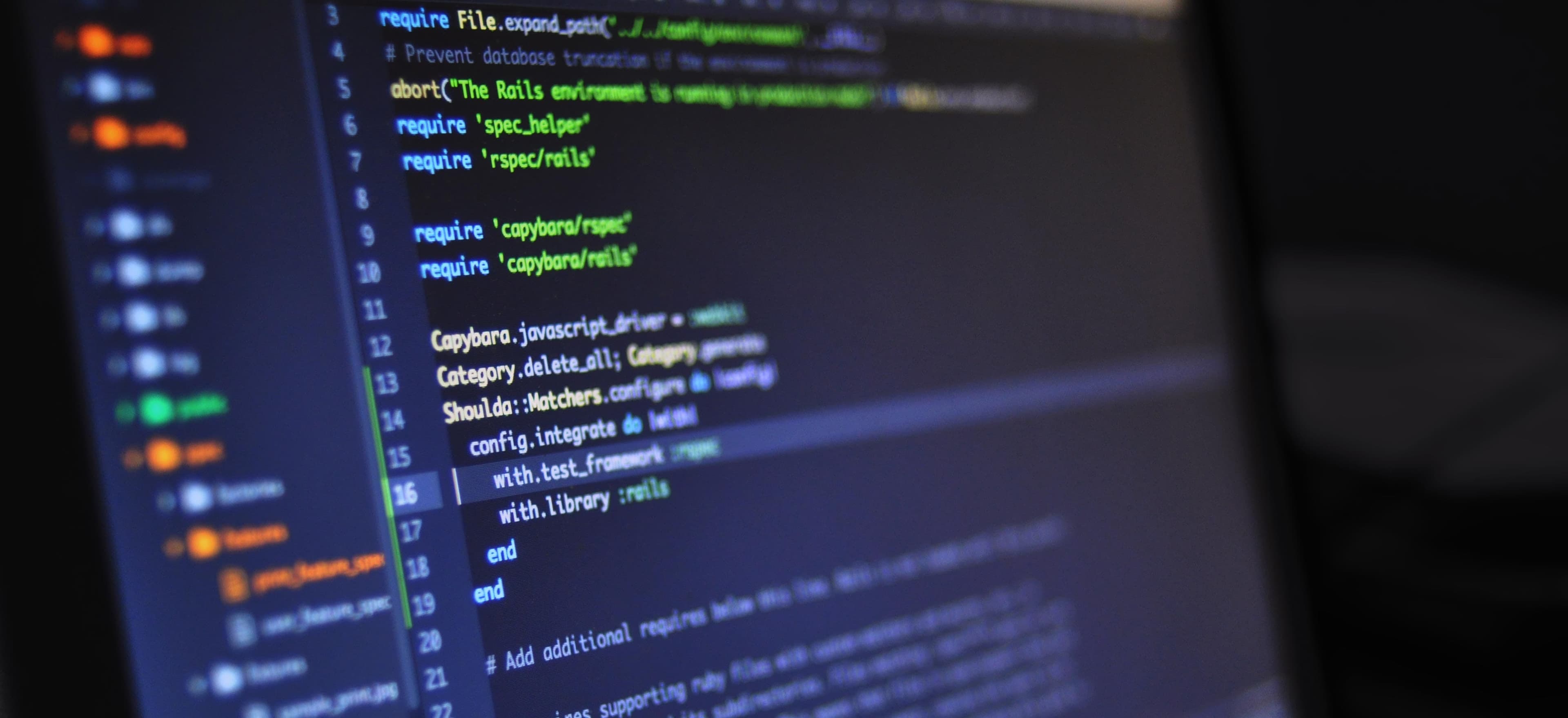
- Published on
Understanding Type Erasure in Java Generics: A Deep Dive
Java Generics was introduced in Java 5 to provide a way to implement generic programming, allowing developers to write code that is more reusable and type-safe. However, one critical aspect of Java Generics is type erasure, which can be somewhat bewildering for newcomers. In this comprehensive guide, we will explore what type erasure is, why it is implemented, and how it affects your Java code. By the end of this post, you should have a solid understanding of this concept.
What Is Type Erasure?
Type erasure is the process by which the Java compiler removes all generics-related type information from your code during compilation. Essentially, it replaces generic types with their bounds or the Object
class when the code is compiled. This ensures compatibility with older versions of Java that did not support generics, allowing for backward compatibility.
Example of Type Erasure in Action
To understand type erasure better, let’s take a look at a simple usage of Java Generics:
class Box<T> {
private T content;
public void setContent(T content) {
this.content = content;
}
public T getContent() {
return content;
}
}
In the above code snippet, we defined a generic class Box
that can hold any type T
. When the Java compiler processes this class, it will apply type erasure, resulting in the following "normal" class that is essentially what the JVM will deal with:
class Box {
private Object content;
public void setContent(Object content) {
this.content = content;
}
public Object getContent() {
return content;
}
}
Here, the type parameter T
has been replaced with Object
. This means that when you use a Box<T>
, any type information associated is effectively erased at runtime.
Why Is Type Erasure Used?
There are a couple of key reasons for the implementation of type erasure:
-
Backward Compatibility: Maintaining compatibility with older versions of Java that did not support generics is crucial. Type erasure allows developers to use generics without breaking existing code.
-
Type Safety: Even with type erasure, generics help to catch type errors during compile-time. Developers get warnings if they attempt to assign incompatible types to a generic. Without generics, such issues would only surface at runtime.
Effects of Type Erasure
Type erasure has several implications when working with Java Generics. Below we will explore some important effects, including the use of generics in collections, casting issues, and restrictions on generic types.
1. Use in Collections
Collections, such as List<T>
, gain a lot from generics. However, because of type erasure, what you might think of as type-safe collections don't retain type information at runtime.
For example:
List<String> stringList = new ArrayList<>();
stringList.add("Hello");
// The following will generate a compiler error
// stringList.add(1);
// After type erasure, the runtime works as List<Object>
List rawList = stringList;
rawList.add(1); // This is valid at runtime!
In the example above, even if stringList
is type-safe at compile-time, converting it to a raw type (List
) allows the addition of an integer, which can cause a ClassCastException
at runtime.
2. Casting Issues
While you may usually perform safe casting thanks to generics
, be cautious about what's being cast after type erasure:
Box<String> box = new Box<>();
box.setContent("Java");
Box rawBox = box; // raw type
String content = (String) rawBox.getContent(); // Dangerous casting!
The cast can lead to ClassCastException
if someone else adds a different type to rawBox
. Always make sure to work with the correct types.
3. Restrictions on Generic Types
Certain restrictions exist due to type erasure, limiting what you can do with generic types. One significant limitation is the inability to create generic arrays:
T[] array = new T[10]; // Compilation Error!
You can work around this by creating an array of Object
and casting it:
T[] array = (T[]) new Object[10];
However, this can introduce potential ClassCastException
at runtime.
Practical Example: A Generic Method
Let's take a closer look at a generic method and how type erasure interacts with it. Here is a simple utility method to swap two elements in an array.
public class ArrayUtils {
public static <T> void swap(T[] array, int i, int j) {
T temp = array[i];
array[i] = array[j];
array[j] = temp;
}
public static void main(String[] args) {
String[] strings = {"a", "b", "c"};
swap(strings, 0, 2);
System.out.println(Arrays.toString(strings)); // Output: [c, b, a]
}
}
In this example, we have a generic method swap
that takes an array of type T
and swaps the elements at the specified indices. Due to type erasure, the actual swap operation is performed on an Object[]
at runtime. This retains type safety at compile time, while still leveraging the flexibility of generics.
Wrapping Up
Type erasure is a crucial concept in Java Generics that allows for backward compatibility while enhancing type safety in code. Understanding how type erasure operates can help you write better, more resilient Java code.
To recap:
- Type erasure removes generic type information at compile-time.
- It allows Java's generics to be backward compatible with older Java versions.
- Be aware of casting issues and restrictions that arise from erasure, especially around raw types and arrays.
For further reading, you may want to explore the official Java documentation on Generics.
Thank you for diving deep into Java's type erasure with us! Feel free to leave comments or questions below. Happy coding!
Checkout our other articles