Optimizing JMS Layer Performance: Common Benchmarking Pitfalls
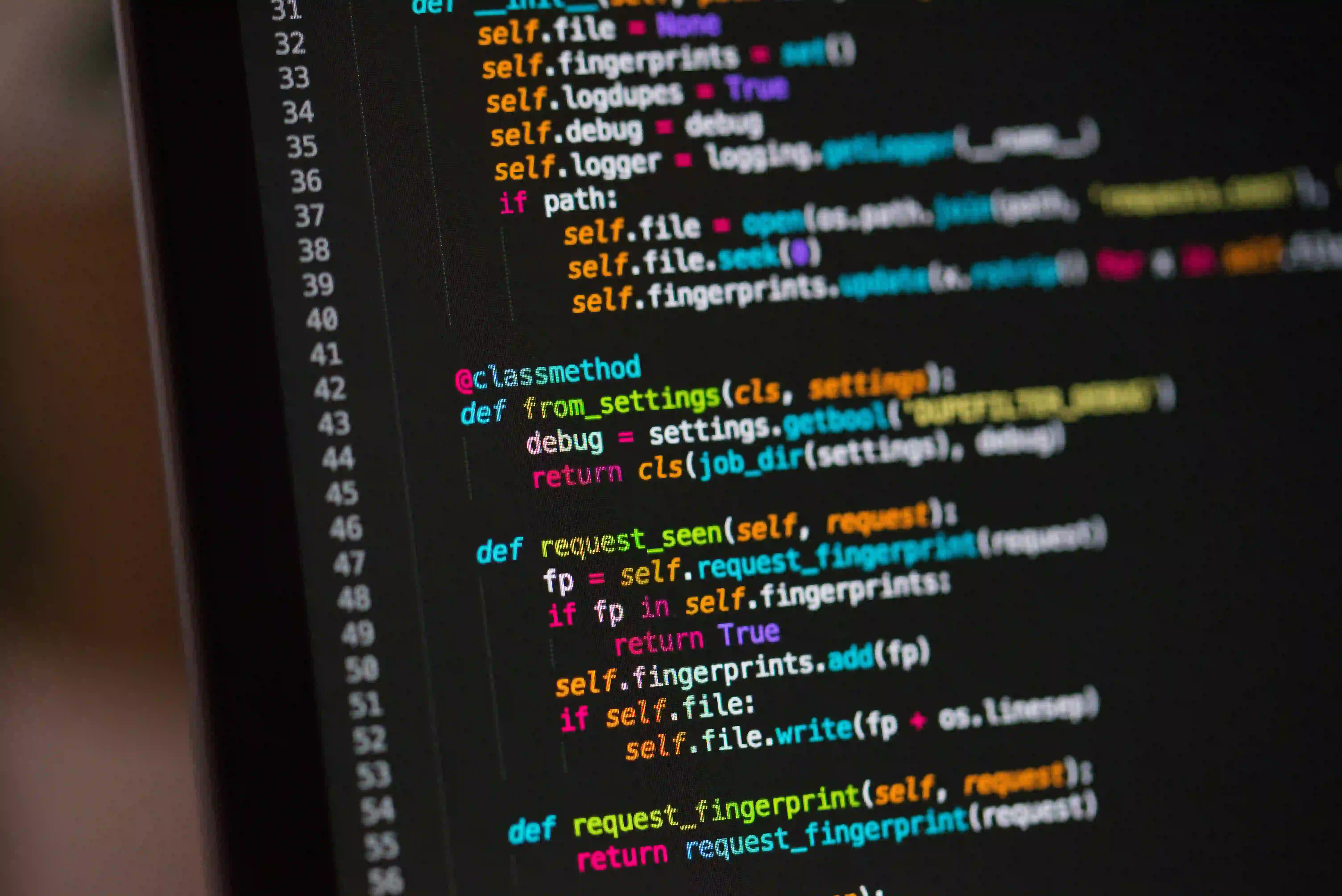
Optimizing JMS Layer Performance: Common Benchmarking Pitfalls
Java Message Service (JMS) is an API that allows Java applications to create, send, receive, and read messages. However, achieving optimal performance is essential for applications that rely heavily on messaging systems. In this blog post, we will explore common pitfalls encountered during JMS benchmarking and provide actionable insights on how to optimize the messaging layer for improved performance.
Understanding JMS Basics
Before diving into performance optimization, let's briefly revisit some key concepts of JMS:
- Message Producer: An application that creates and sends messages.
- Message Consumer: An application that receives and processes messages.
- Message Broker: A middleware component that facilitates communication between producers and consumers.
To grasp how performance works, it's important to examine the flow of messages and how configuration affects it.
Common Benchmarking Pitfalls
While measuring JMS performance, developers can often fall into several traps that may lead to misleading results. Below are the common pitfalls:
1. Ignoring Network Latency
One of the most overlooked factors when benchmarking JMS is network latency. JMS operates over a network, and latency introduces significant variability in performance measurements.
Solution: Use local queues for testing before moving messages to a distributed setup. This eliminates external network delays and allows you to focus on the core performance aspects of the JMS implementation.
2. Misconfigured Message Broker
The configuration of your message broker plays a crucial role in performance. Misconfigurations can lead to bottlenecks, such as thread starvation or resource exhaustion.
Solution: Always analyze your broker’s configurations. For instance, in ActiveMQ, consider adjusting the following parameters:
<broker xmlns="http://activemq.apache.org/schema/core" brokerName="myBroker" dataDirectory="${activemq.data}">
<systemUsage>
<systemUsage>
<memoryUsage>
<memoryLimit>5mb</memoryLimit>
</memoryUsage>
<storeUsage>
<storeLimit>1gb</storeLimit>
</storeUsage>
<tempUsage>
<tempLimit>1gb</tempLimit>
</tempUsage>
</systemUsage>
</systemUsage>
</broker>
Commentary: By tuning memory and store limits, you ensure that your broker efficiently utilizes resources while preventing overflow that could lead to performance degradation.
3. Lack of Adequate Load Testing
Often, developers test the messaging layer under light load conditions that do not mimic production environments. This can give a false sense of security about performance.
Solution: Conduct load testing using tools like Apache JMeter or Gatling. Simulate real-world scenarios by increasing the number of producers and consumers progressively. Use the following sample code snippet to create a producer in Java:
import javax.jms.*;
import javax.naming.InitialContext;
public class JMSProducer {
public static void main(String[] args) throws Exception {
InitialContext ctx = new InitialContext();
ConnectionFactory factory = (ConnectionFactory) ctx.lookup("ConnectionFactory");
Connection connection = factory.createConnection();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
Queue queue = (Queue) ctx.lookup("dynamicQueues/myQueue");
MessageProducer producer = session.createProducer(queue);
for (int i = 0; i < 1000; i++) {
TextMessage message = session.createTextMessage("Message " + i);
producer.send(message);
}
producer.close();
session.close();
connection.close();
}
}
Commentary: This producer sends 1000 messages to a queue. During load testing, vary the number of messages and observe the performance changes.
4. Not Considering Message Size
The size of the messages being sent can drastically affect throughput and latency. Sending large messages can lead to network congestion and longer processing times.
Solution: Optimize your message sizes. This can often be achieved by compressing the payload:
public TextMessage createCompressedMessage(Session session, String payload) throws JMSException {
TextMessage message = session.createTextMessage();
message.setText(compress(payload)); // assuming compress is a valid method
return message;
}
Commentary: In this snippet, we add compression to the message creation process, potentially reducing the size sent over the network while keeping details intact.
5. Overlooking Acknowledgment Modes
JMS offers different acknowledgment modes (AUTO_ACKNOWLEDGE, CLIENT_ACKNOWLEDGE, DUPS_OK_ACKNOWLEDGE). Misconfiguring these can lead to unnecessary burdens on the system.
Solution: Understand trade-offs between reliability and performance. For instance, if messages can be lost and you are okay with it, using DUPS_OK_ACKNOWLEDGE can enhance throughput. Example:
Session session = connection.createSession(false, Session.DUPS_OK_ACKNOWLEDGE);
Commentary: Using DUPS_OK_ACKNOWLEDGE allows for better throughput at the expense of potential duplicate message delivery.
6. Not Collecting Sufficient Metrics
Proper performance monitoring is essential for identifying areas of improvement. Relying solely on application performance is often inadequate.
Solution: Implement monitoring solutions to collect metrics. Tools like Prometheus and Grafana can help visualize and analyze performance over time. Important metrics include message throughput, latency, and memory usage.
Advanced Optimization Techniques
1. Using Asynchronous Message Processing
Asynchronous message processing can help manage load effectively. By decoupling the message production from consumption, applications can improve throughput and handle spikes efficiently.
Example: Using a message listener:
public class JMSAsyncConsumer implements MessageListener {
@Override
public void onMessage(Message message) {
try {
// Process message asynchronously
processMessage(message);
} catch (JMSException e) {
e.printStackTrace();
}
}
}
Commentary: This implementation allows the application to process messages without blocking the main thread, enhancing scalability.
2. Connection Pooling
Repeatedly creating and closing connections is expensive. Implementing connection pooling can reduce this overhead significantly.
Example: Use a library like C3P0 or Apache Commons Pool to manage a pool of JMS connections efficiently.
3. Message Priority
JMS supports message priority allowing you to control the order of message delivery. By prioritizing critical messages, you can improve performance for high-priority workloads.
// Setting message priority
producer.send(message, DeliveryMode.PERSISTENT, 8, Message.DEFAULT_TIME_TO_LIVE);
Commentary: In this example, a priority level of 8 is set for the message, meaning it will be delivered before standard priority messages.
My Closing Thoughts on the Matter
Optimizing JMS performance requires careful consideration of multiple factors. By recognizing potential pitfalls such as those discussed in this post, developers can make informed decisions to enhance their messaging layers. Always remember that the key to effective messaging is balance—in throughput, latency, reliability, and resource usage.
For further reading, consider the following resources:
- Java Message Service Specification
- ActiveMQ Configuration
- JMS Performance Tuning
By avoiding common pitfalls and implementing best practices, you can ensure that your JMS layer performs optimally, providing a reliable backbone for your messaging system. Happy coding!