Common Pitfalls When Developing SOAP Web Services with CXF
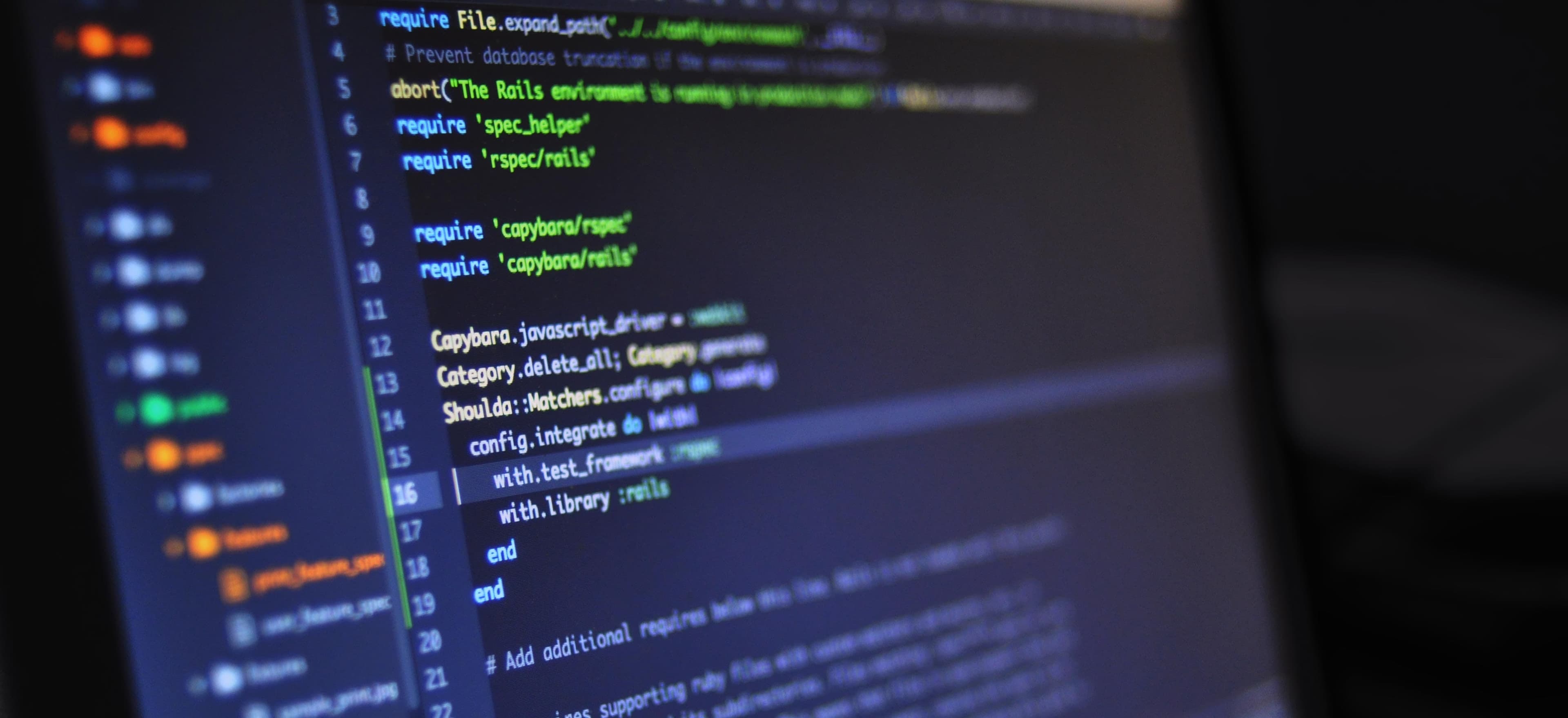
- Published on
Common Pitfalls When Developing SOAP Web Services with CXF
Developing SOAP web services in Java can often feel like a daunting task. When working with the Apache CXF framework, the elegance of design can sometimes be overshadowed by common pitfalls. In this blog post, we will explore the frequent mistakes developers encounter while using CXF for SOAP web services and offer solutions for overcoming these challenges.
What is CXF?
Apache CXF is a robust framework for building web services. It supports both SOAP and REST architectures, making it versatile for various types of applications. CXF provides a rich set of features including WS-Security, WS-Addressing, and seamless integration with Spring.
Pitfall 1: Misconfiguration of CXF
The Importance of Configuration
Configuration is foundational when working with CXF. Many developers mistakenly overlook the importance of a correctly configured environment. Inadequate configuration can lead to failing service calls, errors during deployment, or worse, data leakage.
Common Mistakes
-
Incorrect Endpoint Configuration: Ensure your endpoint is correctly specified in both the
@WebService
annotation and the Spring configuration file.import javax.jws.WebService; @WebService(endpointInterface = "com.example.MyService") public class MyServiceImpl implements MyService { // Implementation details }
-
Spring Context Issues: If you use Spring, ensure the Spring context file is correctly set up with
cxf-servlet.xml
.<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"> <import resource="classpath*:cxf-services.xml" /> <bean id="myService" class="com.example.MyServiceImpl" /> </beans>
Resolution Steps
- Double-check your
cxf-servlet.xml
or any relevant XML configuration files for any typos you may have overlooked. - Use logging extensively to capture CXF errors and warnings which can provide useful hints about misconfiguration.
Pitfall 2: Overlooking Security Features
Why Security Matters
Web services deal with sensitive data, and developers must never overlook security implications. Failing to implement adequate security measures could lead to significant vulnerabilities.
Common Security Mistakes
-
Skipping WS-Security: Failing to implement WS-Security may expose your service to unauthorized access.
<cxf:bus> <cxf:security encryptionProperties="client-enc.properties" signatureProperties="client-sign.properties" /> </cxf:bus>
-
Insecure Communication: Always use HTTPS to safeguard communications.
Implementation Tips
- Implement user authentication and authorization checks using WS-Security.
- Regularly audit the security mechanisms in place, adapting them based on new vulnerabilities.
Pitfall 3: Ignoring Exception Handling
The Need for Robust Exception Handling
Web service calls may fail for multiple reasons, from network issues to service overload. Developers must anticipate these failures and handle exceptions gracefully.
Common Errors
-
Generic Exception Handling: Using a catch-all exception will make debugging very challenging.
public MyResponseType mySoapMethod(MyRequestType request) { try { // Business logic here return new MyResponseType(); } catch (SpecificException e) { // Handling specific exceptions return handleSpecificException(e); } catch (Exception e) { // Handle unexpected exceptions log.error("Unexpected Error", e); throw new WebServiceException("An error occurred."); } }
Recommendations
- Capture and log specific exceptions instead of lumping them together.
- Ensure that your service provides meaningful error messages to clients.
Pitfall 4: Inconsistent Versions
Version Control Importance
In the world of APIs, versioning is crucial. Many developers fail to manage changes, leading to inconsistencies that can cripple service consumers.
Common Issues
-
Neglecting Versioning in SOAP Actions: If you change the structure of your payload and do not update the versions, clients may break.
<wsa:Action soap12:mustUnderstand="1">urn:example:MyService#v1.0</wsa:Action>
-
Not Testing with Previous Versions: Always maintain tests for older versions to ensure backward compatibility.
Best Practices
- Implement a clear versioning strategy for both endpoints and messages.
- Consider utilizing tools like Swagger or Postman to document and test API versions efficiently.
Pitfall 5: Excessive Coupling with CXF
Why Decoupling Matters
Close coupling with CXF specifics makes it challenging to migrate to other frameworks in the future. Developers often tie their application logic too tightly to CXF, which can be inconvenient down the line.
Problematic Patterns
-
Directly using CXF objects throughout your application leads to tight coupling.
public class MyBusinessLogic { private MyServiceImpl myService; // This is tightly coupled to CXF directly }
Suggested Solutions
-
Create service interfaces that isolate your business logic from CXF implementations.
public interface MyService { MyResponseType myMethod(MyRequestType request); }
-
Implement Dependency Injection to achieve reduced coupling.
To Wrap Things Up
Developing SOAP web services using CXF presents several pitfalls but is entirely manageable with foresight and best practices. By ensuring proper configuration, security, exception handling, version management, and decoupling, you can significantly increase the stability and reliability of your application.
If you are interested in understanding more about CXF and SOAP web services, I highly recommend the official Apache CXF documentation and related tutorials.
Building SOAP web services does not come without its challenges; however, a strong grasp of these common pitfalls will help you navigate the murky waters of web service development with greater confidence and expertise!
Checkout our other articles