Common Pitfalls When Getting Started with Jedis
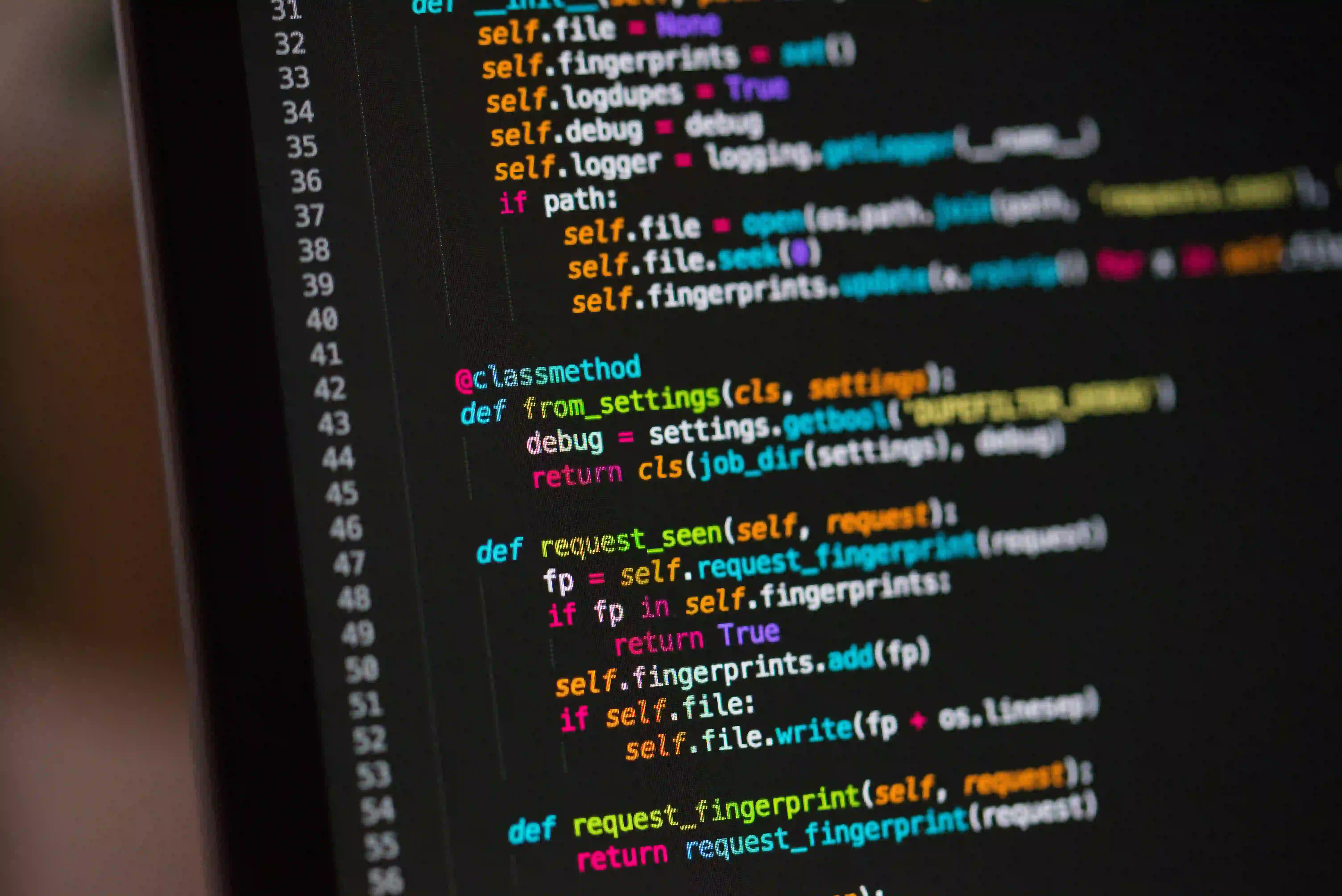
Common Pitfalls When Getting Started with Jedis
Jedis is a popular Java client for Redis, widely used for managing data storage in many applications. While it simplifies interactions with Redis, many beginners encounter various pitfalls that can lead to performance issues or data inconsistencies. In this blog post, we will discuss some of the most common mistakes developers make when starting with Jedis and how to avoid them.
Understanding Jedis
Before diving into the pitfalls, it’s crucial to understand what Jedis is. Redis is an in-memory data structure store, often used as a database or cache. Jedis facilitates communication with Redis in Java, providing a simple API to interact with the database.
To get started with Jedis, you can include it in your Maven project with the following dependency:
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>4.3.1</version>
</dependency>
Pitfall 1: Creating Multiple Jedis Instances
Problem
One of the most common mistakes is creating multiple instances of Jedis
unnecessarily. Each instance consumes resources, and creating them frequently can lead to poor performance.
Solution
Instead of creating a new Jedis
instance for every operation, use a pool of Jedis
connections. The JedisPool
class manages a pool of connections and allows you to borrow an instance when needed and return it afterward.
Example Code
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
public class RedisExample {
private static JedisPool jedisPool = new JedisPool("localhost", 6379);
public static void main(String[] args) {
try (Jedis jedis = jedisPool.getResource()) {
jedis.set("key", "value");
String value = jedis.get("key");
System.out.println(value);
} // Automatic resource management
}
}
In the example above, we borrow a Jedis
instance from the pool for our operations and let it go after use, thus optimizing resource usage.
Pitfall 2: Not Handling Exceptions
Problem
Redis operations can fail for various reasons, such as network issues or data inconsistencies. Not handling exceptions can lead to unexpected behavior in your application.
Solution
Always implement proper exception handling to manage errors effectively.
Example Code
try (Jedis jedis = jedisPool.getResource()) {
jedis.set("key", "value");
} catch (Exception e) {
System.err.println("Error while communicating with Redis: " + e.getMessage());
}
This code snippet catches any exceptions thrown while performing Redis operations and logs an error message instead of failing silently.
Pitfall 3: Ignoring Connection Timeout Settings
Problem
By default, Jedis does not configure timeouts, which may lead to hanging connections under certain conditions.
Solution
Always set connection and read timeouts to avoid this problem.
Example Code
JedisPoolConfig poolConfig = new JedisPoolConfig();
poolConfig.setMaxTotal(128);
poolConfig.setMaxIdle(128);
poolConfig.setMinIdle(16);
poolConfig.setTestOnBorrow(true);
JedisPool jedisPool = new JedisPool(poolConfig, "localhost", 6379, 2000);
Here, we have specified a connection timeout of 2000 milliseconds. This ensures your application does not hang indefinitely while trying to connect to Redis.
Pitfall 4: Incorrect Serialization for Complex Objects
Problem
When storing complex objects, using default serialization can lead to issues. Jedis serializes objects as byte arrays, which may not be compatible across different systems or when the object structure changes.
Solution
Use a customizable serializer for your complex objects. You can use libraries like Jackson or Gson for this purpose.
Example Code
import com.fasterxml.jackson.databind.ObjectMapper;
public void saveUser(User user) {
ObjectMapper objectMapper = new ObjectMapper();
try (Jedis jedis = jedisPool.getResource()) {
String userJson = objectMapper.writeValueAsString(user);
jedis.set("user:" + user.getId(), userJson);
} catch (Exception e) {
System.err.println("Error saving user: " + e.getMessage());
}
}
In this example, we're converting the User
object to a JSON string before storing it in Redis, ensuring better compatibility when retrieving the object later.
Pitfall 5: Not Using Data Structures Effectively
Problem
Redis provides various data structures (like Strings, Lists, Sets, Hashes, etc.), but many developers default to using Strings, missing the advantages of more suitable structures.
Solution
Understand and choose the right data structure for your needs. For example, if you want to store user sessions, consider using a Hash:
Example Code
public void saveSession(Session session) {
try (Jedis jedis = jedisPool.getResource()) {
jedis.hset("session:" + session.getId(), "userId", session.getUserId());
jedis.hset("session:" + session.getId(), "created", session.getCreated().toString());
}
}
Using a Hash allows you to store related attributes together, facilitating better management of session data.
Pitfall 6: Not Monitoring Performance
Problem
Without monitoring, you may not realize there are performance bottlenecks in your Redis usage, leading to slowdown in your application.
Solution
Use tools like Redis Monitoring or Redis Slow Log to keep track of operations and identify long-running commands.
Monitoring Example
# Check the Redis slow log
SLOWLOG GET
This command will display the slow log entries so you can analyze performance issues.
My Closing Thoughts on the Matter
Getting started with Jedis offers a gateway to efficient data management with Redis. However, it is essential to be conscious of common pitfalls that can hinder performance and reliability. By using the techniques described in this post—such as utilizing connection pools, handling exceptions, setting timeouts, and selecting the appropriate data structures—you will be well on your way to building robust Java applications that make the most of Redis.
For further reading, consider checking out the official Jedis documentation and the Redis data structure documentation. Happy coding!