Troubleshooting JMS Bridge Issues Between WildFly Servers
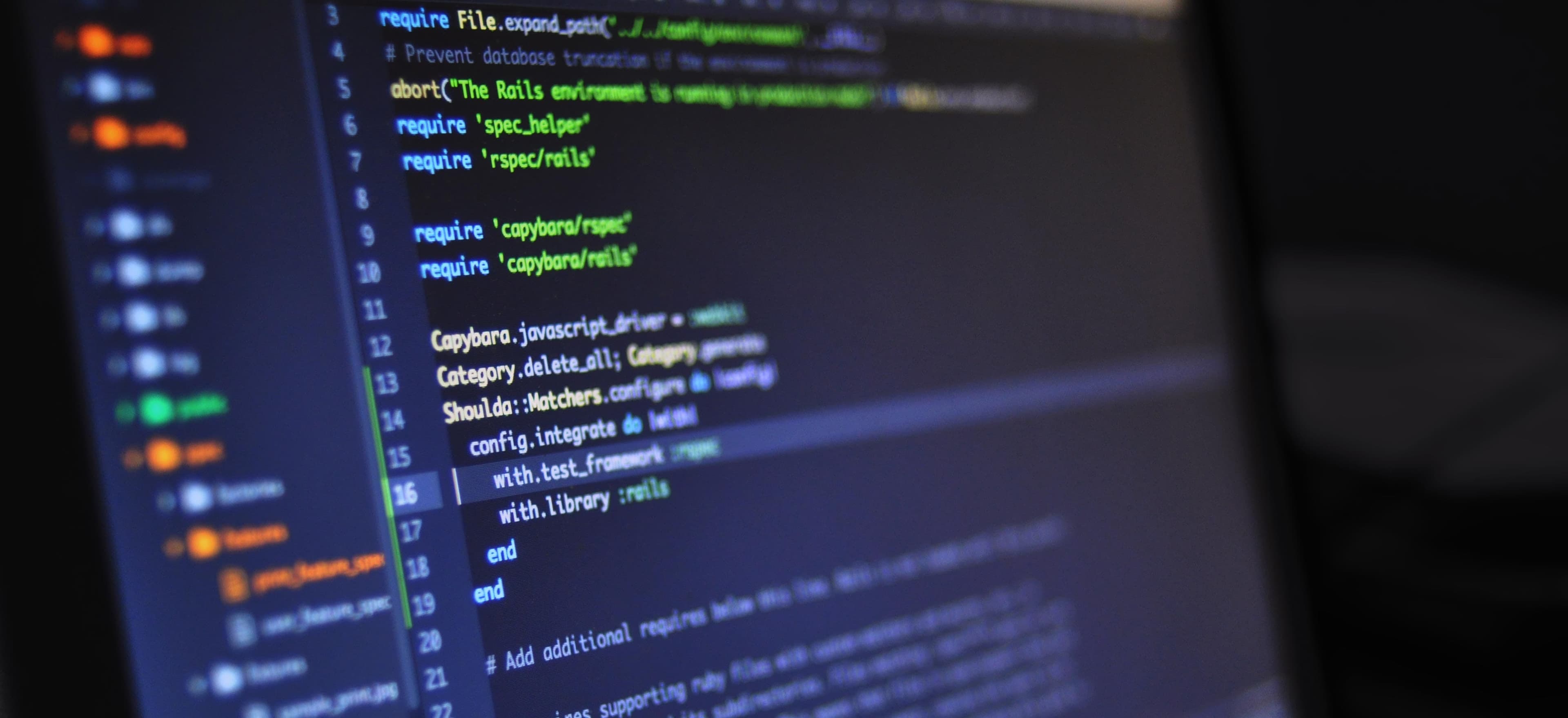
- Published on
Troubleshooting JMS Bridge Issues Between WildFly Servers
Java Message Service (JMS) is a powerful mechanism that allows applications to communicate asynchronously. Many enterprises leverage JMS for robust messaging solutions, and WildFly is a popular application server that provides a comprehensive implementation of JMS. However, setting up a JMS bridge between two WildFly servers can sometimes lead to issues. This blog post will explore common pitfalls and troubleshooting techniques, helping you ensure secure and reliable communications across your architecture.
Understanding JMS Bridge
A JMS bridge facilitates message passing between two different messaging providers, allowing seamless integration. In WildFly, this is typically accomplished through the use of JMS queues and topics. To understand how to troubleshoot your JMS bridge, it’s crucial first to recognize the anatomy of a JMS bridge.
Key Components of a JMS Bridge
- Source Connection Factory: Responsible for creating connections to the source JMS provider.
- Source Destination: The JMS queue or topic from which messages will be consumed.
- Target Connection Factory: Similar to the source, this connects to the target JMS provider.
- Target Destination: The JMS queue or topic to which messages will be sent.
With these components in mind, let’s delve into common JMS bridge issues and how to resolve them.
Common Issues and Resolutions
1. Incorrect Configuration Settings
Misconfiguration is one of the most common issues encountered when dealing with JMS bridges. A small typo can lead to failed connections or dropped messages.
Example:
Ensure you have correctly defined your connection factories and destinations in the standalone.xml
or domain.xml
configuration files.
<subsystem xmlns="urn:jboss:domain:messaging:2.0">
<hornetq-server>
<connection-factory name="InVMConnectionFactory" entries="java:/ConnectionFactory"/>
<jms-queue name="queue/myQueue" entries="java:/queue/myQueue"/>
</hornetq-server>
</subsystem>
Why: Each entry needs to exist correctly and accessible for your JMS bridge to work. Verify that entries match throughout your configuration.
2. Network Issues
Network problems can disrupt the communication between the two WildFly servers. Ensure both servers can reach each other.
Steps to Diagnose:
- Use tools like
ping
to test connectivity. - Check firewalls or security groups that might block the necessary ports.
3. Driver and Library Conflicts
If you are using a custom or third-party driver to interact with JMS resources, ensure its compatibility with WildFly and that no conflicting libraries are in use.
Example:
If you need a specific version of a library, include it in your WildFly module. For instance:
<module xmlns="urn:jboss:module:1.1" name="com.example.jmsdriver">
<resources>
<resource-root path="jms-driver.jar"/>
</resources>
<dependencies>
<module name="javax.api"/>
<module name="javax.jms.api"/>
</dependencies>
</module>
Why: Version conflicts can lead to classloader issues, causing runtime errors. Always use compatible versions.
4. Exception Handling
Sometimes, you may overlook exception handling in your code, which can lead to silent failures. Always log or handle exceptions appropriately.
Example:
Consider this simple producer/consumer in JMS:
try {
Connection connection = connectionFactory.createConnection();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
Queue queue = session.createQueue("queue/myQueue");
MessageProducer producer = session.createProducer(queue);
// Message creation & sending
TextMessage message = session.createTextMessage("Hello JMS!");
producer.send(message);
} catch (JMSException e) {
e.printStackTrace(); // Avoid silent failures
}
Why: By properly handling exceptions, you can more easily identify the root cause of the problem and then resolve it quickly.
5. Logging and Monitoring
Implement logging to monitor your JMS bridge activity. This will help you capture data about message flow and identify any bottlenecks.
- Enable debug logging for JMS in WildFly by adjusting the logging configuration:
<logger category="org.hornetq">
<level name="DEBUG"/>
</logger>
Why: Detailed logs can provide insights into what is happening in real-time, assisting in diagnosing issues rapidly.
Testing Your JMS Bridge
Having an effective JMS bridge tests your system's resilience. You can create a test program to send and receive messages between two WildFly servers.
Test Program Example
import javax.jms.*;
import javax.naming.Context;
import javax.naming.InitialContext;
public class JmsBridgeTest {
public static void main(String[] args) {
try {
Context context = new InitialContext();
ConnectionFactory connectionFactory = (ConnectionFactory) context.lookup("java:/ConnectionFactory");
Queue queue = (Queue) context.lookup("java:/queue/myQueue");
Connection connection = connectionFactory.createConnection();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
MessageProducer producer = session.createProducer(queue);
MessageConsumer consumer = session.createConsumer(queue);
// Sending a test message
TextMessage message = session.createTextMessage("Test Message");
producer.send(message);
System.out.println("Sent message: " + message.getText());
// Receiving a message
connection.start();
TextMessage receivedMessage = (TextMessage) consumer.receive(1000);
System.out.println("Received message: " + receivedMessage.getText());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Run this test on both servers to observe the message flow. This way, you can validate that your JMS bridge is functioning correctly.
References for Further Reading
- WildFly Documentation - A great resource for understanding configurations and setups.
- Java Message Service API - A detailed guide on JMS functionalities and best practices.
Bringing It All Together
Troubleshooting JMS bridge issues can be daunting but is manageable with the right approach. By paying attention to configuration details, diagnosing network issues, and incorporating effective logging, you set yourself up for success. Testing your setup in a controlled environment can also help identify potential issues before they impact your production systems.
By employing these strategies, you can ensure your JMS bridge between WildFly servers runs smoothly, providing a reliable asynchronous communication channel for your applications. A well-configured JMS setup will enhance your application's scalability and reliability, paving the way for future growth and integration possibilities.
Checkout our other articles