Common Mistakes When Using Class Name Locators in Selenium
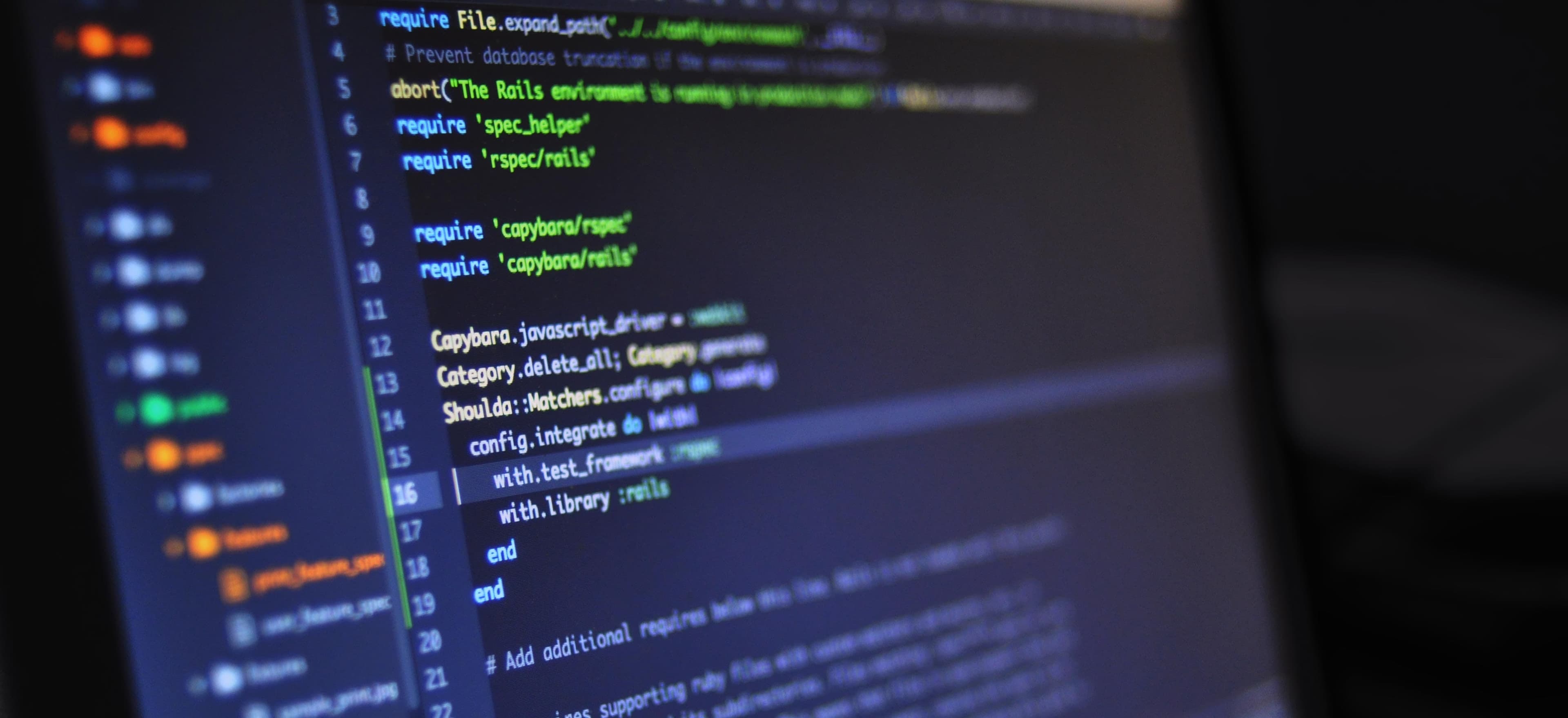
- Published on
Common Mistakes When Using Class Name Locators in Selenium
Selenium is an incredibly powerful tool for automating browser actions, and it has become the de facto standard for web application testing. Among the various locators it provides, the class name locator is often a popular choice among developers and testers. However, improper use of class name locators can lead to frustrating debug sessions and flaky tests. This blog post will discuss common mistakes when using class name locators in Selenium, providing examples and best practices to ensure effective and reliable test automation.
What Are Class Name Locators?
Class name locators in Selenium allow you to find elements on a web page by their HTML class attribute. When you reference a class name in your code, Selenium can then interact with these elements for clicks, text extraction, and other tasks.
Example:
WebElement element = driver.findElement(By.className("my-class"));
This line of code attempts to locate an element on a web page that has the class name "my-class". However, using class name locators isn't always straightforward, and many mistakes can arise.
Common Mistakes
1. Choosing Non-Unique Class Names
One of the first and most significant mistakes is using non-unique class names. In HTML, it is perfectly valid for multiple elements to share the same class name. If you use a non-unique class name, your test may not interact with the intended element.
Example:
Suppose we have the following HTML structure:
<div class="button">Button 1</div>
<div class="button">Button 2</div>
If your test code is as follows:
WebElement button = driver.findElement(By.className("button"));
button.click();
This code will only click one of the "button" class elements, but it is dependent on the DOM structure at runtime. Sometimes, it could even lead to an ElementNotInteractableException if the wrong button is clicked.
Solution: Always strive for unique class names if possible, or use a combination of locators to improve accuracy.
2. Not Handling Element Visibility
Another common mistake involves locating an element that is present in the DOM but hidden from view. Selenium cannot interact with elements that are not visible, leading to failures in your tests.
Example:
If you have the following HTML:
<div class="hidden" style="display: none;">This is Hidden</div>
Attempting to click on the hidden element might look like this:
WebElement hiddenElement = driver.findElement(By.className("hidden"));
hiddenElement.click(); // This will throw an Exception
Solution: Implement checks to verify that elements are visible before interacting with them.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement visibleElement = wait.until(ExpectedConditions.visibilityOfElementLocated(By.className("hidden")));
visibleElement.click();
3. Ignoring Case Sensitivity
HTML class names are case-sensitive, so using the wrong case can lead to class not found errors. This is particularly a common oversight when dealing with frameworks or libraries that define class attributes inconsistently.
Example:
HTML structure:
<div class="My-Class">Hello</div>
If your code looks like this:
WebElement myClassElement = driver.findElement(By.className("my-class")); // Incorrect case
It will throw a NoSuchElementException because the class name does not match.
Solution: Always double-check the case when referencing class names to avoid this mistake.
4. Hardcoding Class Names
In many scenarios, class names can be dynamically generated or may change with different versions of a web application. Hardcoding class names can thus lead to fragile tests that break with minor updates.
Instead of:
WebElement button = driver.findElement(By.className("btn-primary-1234")); // Dynamically generated
Solution: Use meaningful constants or fetch class names dynamically, if possible.
String buttonClass = getDynamicClassName(); // Assume this method fetches the current class name
WebElement button = driver.findElement(By.className(buttonClass));
5. Not Understanding the Structure of the Page
Before jumping into using class name locators, understanding the DOM structure of the page is crucial. If you select a class based on how it visually looks on the page without understanding where it sits within the hierarchy, you might select the wrong element.
Example:
<div class="card">
<div class="header">Header</div>
<div class="content">Content</div>
</div>
If you mistakenly locate the .header
class for your action thinking it’s a button, you won’t get the expected outcome.
Solution: Inspect the HTML structure carefully before deciding which locator to use.
6. Relying Solely on Class Name Locators
While class name locators are convenient, relying exclusively on them could cause issues down the road, especially if the application undergoes UI redesigns. Class names may change without warning.
Solution: Mix up your locators for robustness. Combine class names with other strategies such as ID, XPath, or CSS selectors. For example:
WebElement button = driver.findElement(By.xpath("//div[@class='card']/div[@class='content']"));
Bringing It All Together
Using class name locators in Selenium can greatly simplify your test scripts, but it’s vital to understand the common pitfalls that can lead to inefficiencies or failures. By avoiding non-unique class names, checking for visibility, being aware of case sensitivity, refraining from hardcoding, understanding the DOM structure, and diversifying locator strategies, you will enhance the reliability and resilience of your automated tests.
For additional insights on Selenium best practices, consider checking out the official Selenium documentation. By applying these lessons and considering the context in which you're using class names, you will build more effective, maintainable, and robust test automation frameworks.
Happy testing! If you have questions or need further clarification on any concepts, feel free to leave a comment below.