Securing Your Spring Boot Actuator: Key Challenges Unveiled
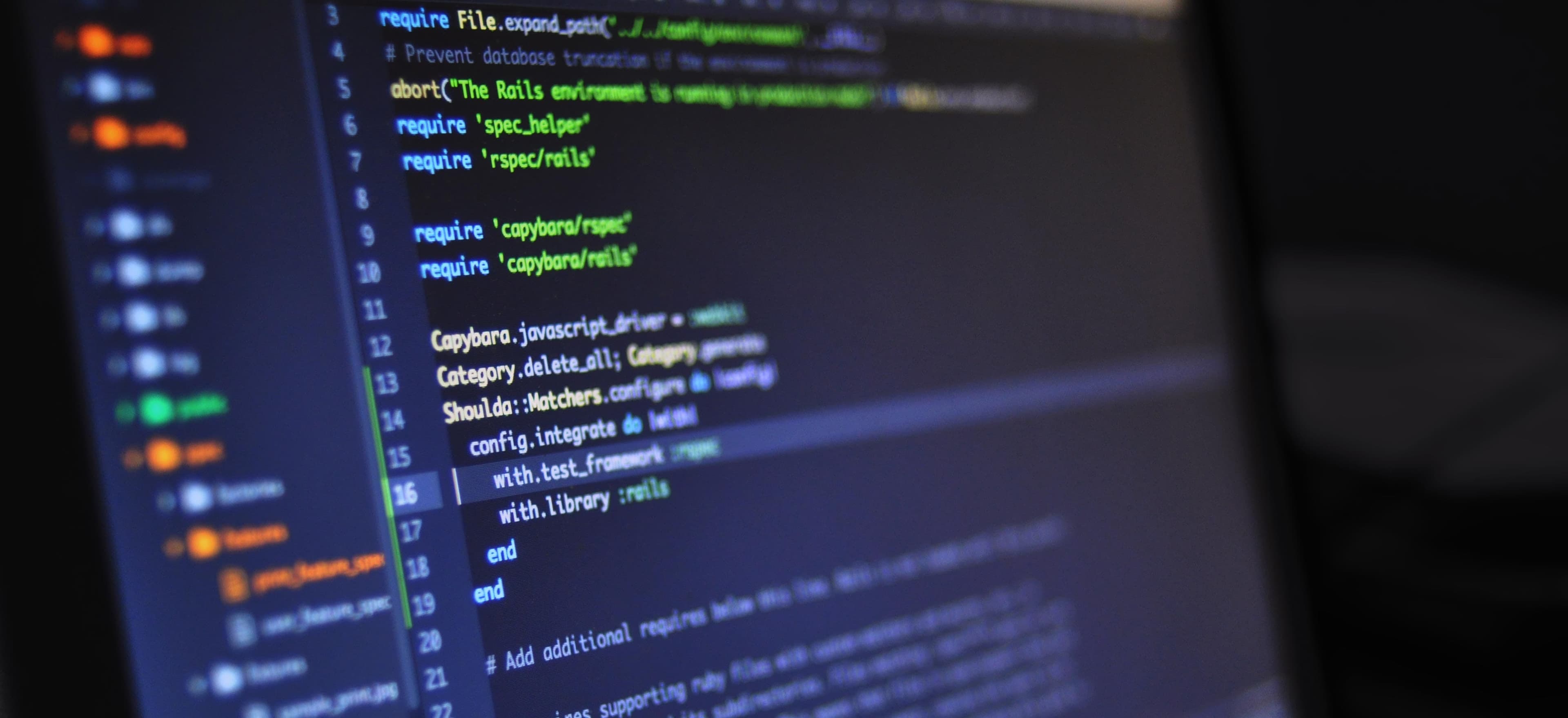
- Published on
Securing Your Spring Boot Actuator: Key Challenges Unveiled
Spring Boot's actuator module is a powerful tool for monitoring and managing Spring applications in production environments. It provides numerous features like health checks, metrics, environment variables, and more. However, these powerful capabilities come with their own set of security challenges. In this blog post, we will explore those challenges and how to effectively secure your Spring Boot actuator endpoints.
What is Spring Boot Actuator?
Spring Boot Actuator integrates seamlessly into your Spring applications and provides built-in endpoints to monitor and manage your application. You can access a wealth of information such as application health, metrics, and environment details through a simple web interface or API calls.
Importance of Security
By exposing actuator endpoints without proper security, you risk exposing sensitive information about your application’s internal configuration, infrastructure, and operational data. This could lead to unauthorized access or even compromise of your application.
Key Security Challenges
- Exposure of Endpoints
- Default Behavior
- Inadequate Authentication
- Sensitive Information Leakage
- Overly Broad Access
Let’s delve deeper into each of these challenges and how to address them effectively.
1. Exposure of Endpoints
Many of the actuator endpoints are designed for application monitoring but can expose a lot of sensitive data, especially in production environments.
Solution: Configure your application to limit and secure the endpoints. You can customize which endpoints are enabled in your application by modifying the application.properties
or application.yml
file.
management:
endpoints:
web:
exposure:
include: health,info
exclude: env,beans,metrics
By only exposing essential endpoints like health
and info
, you can reduce the potential attack surface.
2. Default Behavior
Out of the box, Spring Boot provides a number of actuator endpoints that might be accessible to everyone unless you configure them otherwise.
Solution: Use Spring Security to restrict access. Here's an example of how to secure the actuator endpoints in the application.properties
file.
spring.security.user.name=admin
spring.security.user.password=admin
management.endpoints.web.exposure.include=*
management.endpoint.health.show-details=always
This configuration sets up basic authentication for all actuator endpoints, which is your first line of defense.
3. Inadequate Authentication
Many applications fail to implement robust authentication measures. Relying solely on default username/password combinations is insufficient and insecure.
Solution: Configure Spring Security to enforce stronger authentication mechanisms. Here’s how:
import org.springframework.context.annotation.Bean;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/actuator/**").authenticated()
.and()
.httpBasic();
}
}
This Java configuration ensures that only authenticated users can access actuator endpoints, a critical layer of security.
4. Sensitive Information Leakage
Endpoints like env
or beans
can reveal sensitive data such as API keys or database connection strings that might be embedded within your application properties.
Solution: Always limit the endpoints you expose and ensure that sensitive data isn’t included in the output. Here’s how you might customize the application.yml
:
management:
endpoints:
web:
exposure:
include: health,info
sensitive: "true"
5. Overly Broad Access
If you grant access to all users without any limitations, you are inviting trouble. Hackers thrive on poorly secured applications.
Solution: Limit access at a more granular level. You might want to provide different access levels to different users. Here's an example of using role-based access controls:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/actuator/health").permitAll()
.antMatchers("/actuator/**").hasRole("ADMIN")
.and()
.httpBasic();
}
This configuration allows everyone to access the health endpoint but restricts other actuator endpoints to users with the ADMIN role.
Using Environment-Specific Configuration
An effective practice is to use different security configurations for different environments (development, staging, and production).
You can use profiles in Spring Boot to load different application-{profile}.properties
files based on the active profile. For example, you can have application-prod.properties
that contains stricter access limits than application-dev.properties
.
Monitoring and Logging
Implementing access control is important, but monitoring who has accessed your actuator endpoints is equally critical. You should ensure that your application logs access to these endpoints.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Component;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@Component
public class AccessLogger {
private static final Logger logger = LoggerFactory.getLogger(AccessLogger.class);
public void logAccess(HttpServletRequest request, HttpServletResponse response) {
logger.info("Accessed: {} with status: {}", request.getRequestURI(), response.getStatus());
}
}
A Final Look
Securing your Spring Boot actuator endpoints is not merely a security best practice; it is a necessity in today’s threat landscape.
To recap:
- Limit endpoint exposure: Only expose what is necessary.
- Implement security measures: Use Spring Security for robust authentication.
- Monitor and log accesses: Keeping track of who accesses your data is vital.
- Use different configurations for different environments: Tailor your security to your deployment stage.
By taking these proactive measures, you can significantly enhance the security of your Spring Boot application. For further reading and examples, you might want to explore the official Spring Boot Security Documentation and the Spring Boot Actuator Guide.
Remember, security is not a one-time setup; it's an ongoing process that evolves as your application grows.