Top 5 Spring Features Every Java Developer Should Master
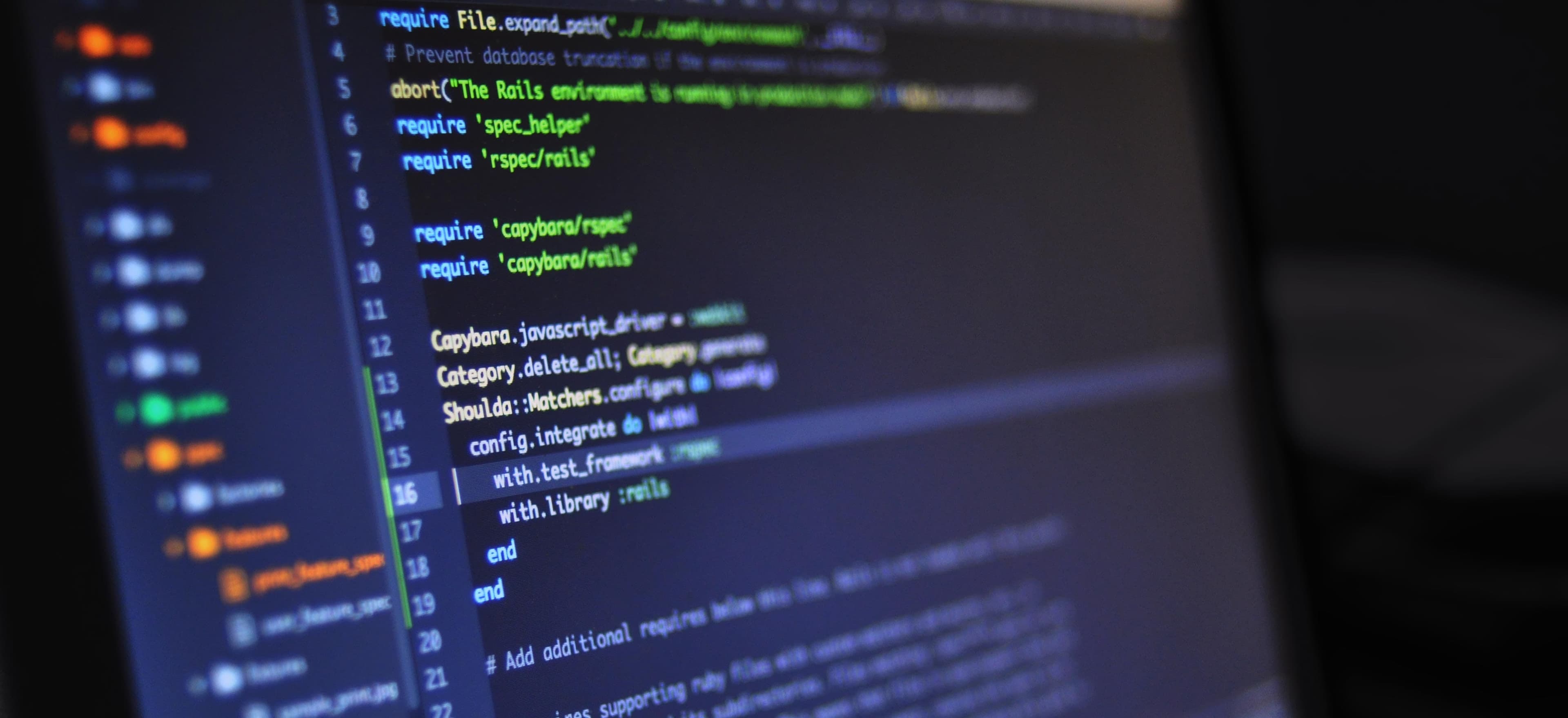
- Published on
Top 5 Spring Features Every Java Developer Should Master
Spring Framework has become synonymous with Java development, powering numerous enterprise applications. Its productivity features help developers build robust, scalable, and maintainable applications. This blog post will explore five essential Spring features that every Java developer should master: Inversion of Control (IoC), Aspect-Oriented Programming (AOP), Spring Data, Spring Security, and Spring Boot.
1. Inversion of Control (IoC)
Perhaps the cornerstone of Spring, Inversion of Control (IoC) allows developers to decouple application components, enhancing flexibility and maintainability.
How IoC Works
In traditional programming, objects control their dependencies. With IoC, however, the control flows in the opposite direction. The Spring IoC container manages object instantiation and wiring. As a result, you can focus on logic rather than the lifecycle and dependencies of objects.
Example:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
class UserService {
public void displayUser() {
System.out.println("Displaying user information");
}
}
@Component
class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
public void showUser() {
userService.displayUser();
}
}
Commentary
In this example, UserController
is dependent on UserService
. By using the @Autowired
annotation, Spring automatically injects the UserService
instance when creating the UserController
. This decouples the two classes, allowing for easier testing and modifications.
For an extensive read on IoC, check out the official Spring Framework Documentation.
2. Aspect-Oriented Programming (AOP)
AOP helps in creating cross-cutting concerns, like logging, authentication, and error handling, that affect multiple layers of an application. It allows developers to separate these concerns from the business logic, leading to cleaner, more maintainable code.
How AOP Works
By leveraging AOP, you can define aspects, pointcuts, and advice. An aspect captures the cross-cutting concern, the pointcut defines where the aspect applies, and advice specifies what action to take.
Example:
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.springframework.stereotype.Component;
@Aspect
@Component
class LoggingAspect {
@Before("execution(* UserService.*(..))")
public void logBefore() {
System.out.println("Before method execution");
}
}
Commentary
In this example, the LoggingAspect
class uses AOP to log a message before any method in the UserService
class executes. The @Before
annotation indicates when the aspect should be applied, in this case, before method execution. This way, you avoid cluttering your business logic with logging details.
For more details on AOP principles in Spring, refer to Spring AOP Documentation.
3. Spring Data
Spring Data simplifies data access by providing a consolidated framework for various persistence data stores. It allows for easier CRUD operations, pagination, and auditing.
How Spring Data Works
Using Spring Data, you can define an interface, and it will automatically provide the implementation. You work with data repositories without the need for boilerplate code.
Example:
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
Commentary
In this example, UserRepository
extends JpaRepository
, allowing you to perform CRUD operations without writing any boilerplate code. The method findByUsername
is automatically implemented by Spring Data based on its naming convention. It streamlines database interactions, making data management a breeze.
For an in-depth look, visit the official Spring Data Reference Guide.
4. Spring Security
In a world where data breaches are common, Spring Security provides a robust framework for authentication and authorization. It allows developers to secure applications with minimal effort.
How Spring Security Works
Spring Security handles user authentication, role-based authorization, and various security vulnerabilities. It integrates seamlessly with existing Spring applications.
Example:
import org.springframework.context.annotation.Bean;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@EnableWebSecurity
class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin();
}
}
Commentary
In this example, the SecurityConfig
class configures HTTP security. It allows unrestricted access to paths matching /public/**
while requiring authentication for all other requests. By incorporating form login, Spring Security takes care of common authentication procedures for you.
To dive deeper into Spring Security, refer to the official Spring Security Documentation.
5. Spring Boot
Spring Boot eliminates boilerplate configuration, making it easier to create stand-alone applications. Its convention over configuration paradigm allows developers to focus on coding rather than setup.
How Spring Boot Works
With Spring Boot, you can create a Spring project with minimal configuration. It comes with embedded servers, such as Tomcat, and integrates various Spring components seamlessly.
Example:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Commentary
In this example, the Application
class is your entry point. By annotating it with @SpringBootApplication
, you enable several configurations, such as component scanning and auto-configuration. This concise setup allows you to focus on the core functionality of your application.
For a comprehensive understanding of Spring Boot, check out the official Spring Boot Documentation.
Closing the Chapter
Mastering these five Spring features—Inversion of Control, Aspect-Oriented Programming, Spring Data, Spring Security, and Spring Boot—will significantly boost your prowess as a Java developer. From decoupled components to streamlined data management, Spring provides the tools necessary to meet contemporary application demands. With each feature complementing the others, learning them will empower you to build robust applications efficiently.
Stay informed, keep practicing, and embrace the world of Spring Framework. Happy coding!
Checkout our other articles