Overcoming Slow Decision-Making with Stream Processing
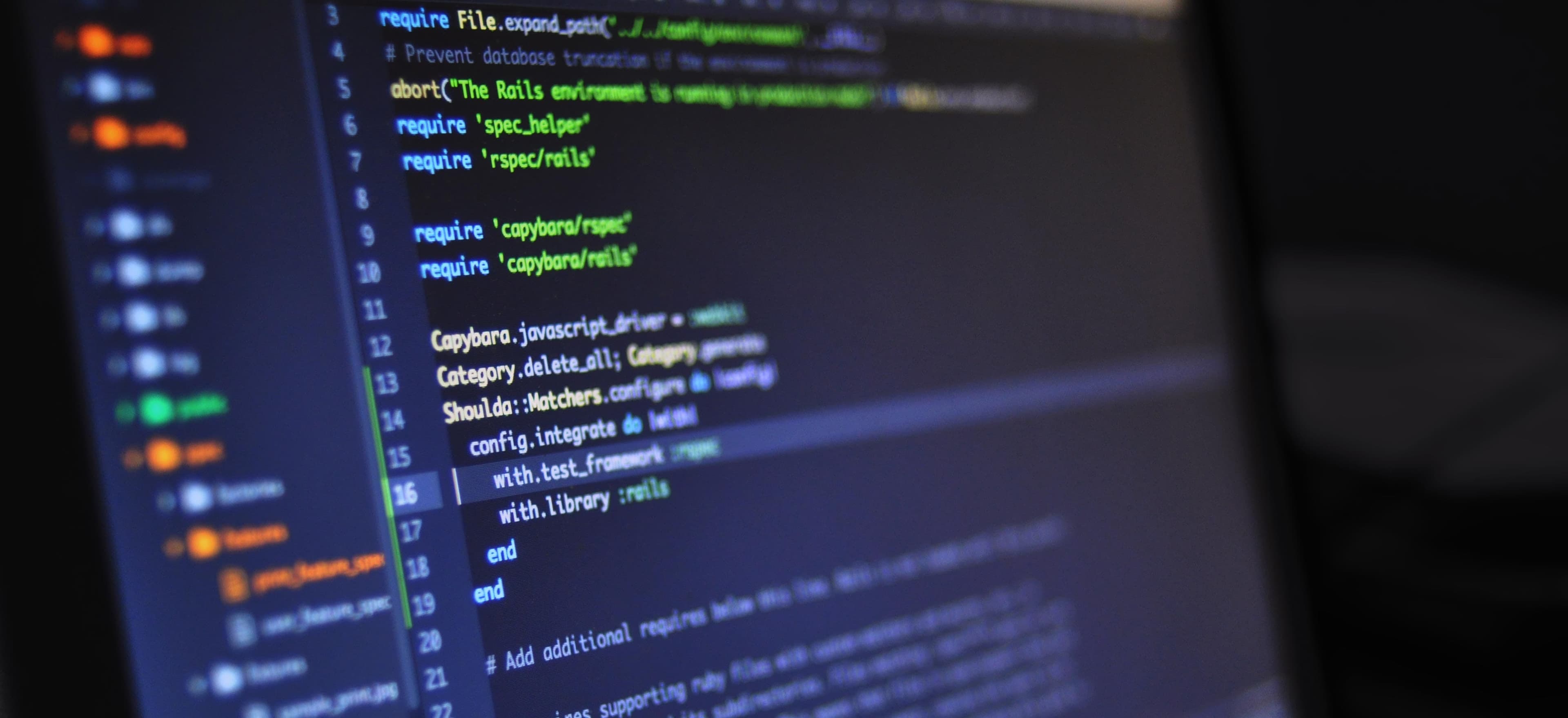
- Published on
Overcoming Slow Decision-Making with Stream Processing
In today's data-driven world, organizations are under increasing pressure to make quick and efficient decisions. Traditional data processing methods, often relying on batch processing, can create significant delays, hinder agility, and ultimately affect a company's competitive edge. Enter stream processing: a paradigm shift that allows organizations to process and analyze real-time data streams efficiently. This blog post will explore stream processing, its benefits, relevant technologies, and Java examples to help you overcome slow decision-making.
What is Stream Processing?
Stream processing is the real-time processing of data streams, allowing organizations to analyze continuous, unbounded sequences of data. Unlike batch processing, which collects data over a period before processing it, stream processing continually processes data as it becomes available. This capability is essential for businesses that must respond quickly to ever-changing data.
Key Benefits of Stream Processing
- Real-time Insights: Continuous streams of data allow organizations to gain insights instantly, enabling smarter and faster decision-making.
- Improved Customer Experience: Brands can personalize services in real-time, improving customer engagement.
- Scalability: Stream processing frameworks are designed to process vast amounts of data across distributed systems seamlessly.
- Event-driven Architecture: Businesses can implement an event-driven approach, ensuring that they react immediately to important changes.
- Reduced Latency: Stream processing reduces the time between data capture and actionable insights.
Popular Stream Processing Frameworks
When it comes to stream processing, several frameworks are worth mentioning:
- Apache Kafka: A distributed event streaming platform capable of handling trillions of events a day.
- Apache Flink: A stream processing framework that provides high-throughput, low-latency, and strong consistency guarantees.
- Apache Spark Streaming: Extends Apache Spark for processing real-time data streams with micro-batch processing.
- Spring Cloud Stream: Provides a framework for building event-driven microservices connected to shared messaging systems.
Getting Started with Java and Stream Processing
In this section, we will delve into an example that showcases the power of stream processing using Java. For this demonstration, we will use Apache Kafka because it has gained immense popularity in building real-time data pipelines and streaming applications.
Setting Up a Simple Kafka Producer and Consumer
Before diving into the code, ensure you have Apache Kafka set up on your local machine. You can find installation instructions on the Apache Kafka website.
1. Maven Dependencies
Add the following dependencies to your Maven pom.xml
. This includes the Kafka client library used for producing and consuming messages.
<dependencies>
<dependency>
<groupId>org.apache.kafka</groupId>
<artifactId>kafka-clients</artifactId>
<version>3.0.0</version>
</dependency>
</dependencies>
2. Kafka Producer
Below is an example of a simple Kafka producer. It generates random integers and sends them to a Kafka topic called "numbers."
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerRecord;
import org.apache.kafka.clients.producer.RecordMetadata;
import java.util.Properties;
import java.util.Random;
public class NumberProducer {
private static final String TOPIC_NAME = "numbers";
public static void main(String[] args) {
Properties properties = new Properties();
properties.put("bootstrap.servers", "localhost:9092");
properties.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
properties.put("value.serializer", "org.apache.kafka.common.serialization.IntegerSerializer");
KafkaProducer<String, Integer> producer = new KafkaProducer<>(properties);
Random random = new Random();
for (int i = 0; i < 10; i++) {
Integer number = random.nextInt(100);
ProducerRecord<String, Integer> record = new ProducerRecord<>(TOPIC_NAME, null, number);
producer.send(record, (RecordMetadata metadata, Exception e) -> {
if (e != null) {
System.err.println("Error while producing: " + e.getMessage());
} else {
System.out.println("Sent number: " + number + " to partition: " + metadata.partition());
}
});
}
producer.close();
}
}
Why this code?: The producer generates random numbers and sends them to a Kafka topic called "numbers." This code showcases how to effectively send messages to Kafka asynchronously, improving performance and responsiveness.
3. Kafka Consumer
Next, let's create a simple Kafka consumer that reads messages from the same topic and processes them in real time.
import org.apache.kafka.clients.consumer.ConsumerRecord;
import org.apache.kafka.clients.consumer.KafkaConsumer;
import org.apache.kafka.clients.consumer.ConsumerRecords;
import java.time.Duration;
import java.util.Collections;
import java.util.Properties;
public class NumberConsumer {
private static final String TOPIC_NAME = "numbers";
public static void main(String[] args) {
Properties properties = new Properties();
properties.put("bootstrap.servers", "localhost:9092");
properties.put("group.id", "number-consumer-group");
properties.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
properties.put("value.deserializer", "org.apache.kafka.common.serialization.IntegerDeserializer");
KafkaConsumer<String, Integer> consumer = new KafkaConsumer<>(properties);
consumer.subscribe(Collections.singletonList(TOPIC_NAME));
try {
while (true) {
ConsumerRecords<String, Integer> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, Integer> record : records) {
System.out.printf("Received number: %d from partition: %d%n", record.value(), record.partition());
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
consumer.close();
}
}
}
Why this code?: The consumer connects to the Kafka cluster and listens for incoming messages on the topic "numbers." This example illustrates how organizations can continuously read and process data, leading to quicker decisions.
Handling Real-Time Data Streams: Use Cases
Stream processing can be applied across various industries and real-time scenarios:
-
Financial Services:
- Fraud detection leveraging real-time transaction analysis.
- Stock price updates and alerts based on market changes.
-
Retail:
- Personalized customer recommendations based on user behavior.
- Inventory management and alerting for low-stock items.
-
Telecommunications:
- Network monitoring for jeopardy detection and performance assessments.
- Real-time analytics for customer service interactions to improve support.
-
Internet of Things (IoT):
- Real-time analytics on sensor data from smart devices.
- Predictive maintenance based on device performance metrics.
The Bottom Line
Slow decision-making can cost businesses significantly. Stream processing, coupled with technologies like Apache Kafka, enables fast and effective responses to continuously changing data streams. By embracing this paradigm, organizations can build robust systems that process data in real-time, ensuring they remain competitive in a rapidly evolving landscape.
To further your understanding of stream processing in Java, consider exploring related material. Check out the official Kafka documentation for more comprehensive guides and best practices.
Stream processing is not solely a technical upgrade; it represents a cultural shift in how information is viewed within organizations. Stay ahead of the curve by integrating these powerful solutions today!
Feel free to share your thoughts or experiences with stream processing below. What challenges have you faced, and how did you overcome them? Let’s learn together!
Checkout our other articles