Common Pitfalls When Using Lombok in Java Projects
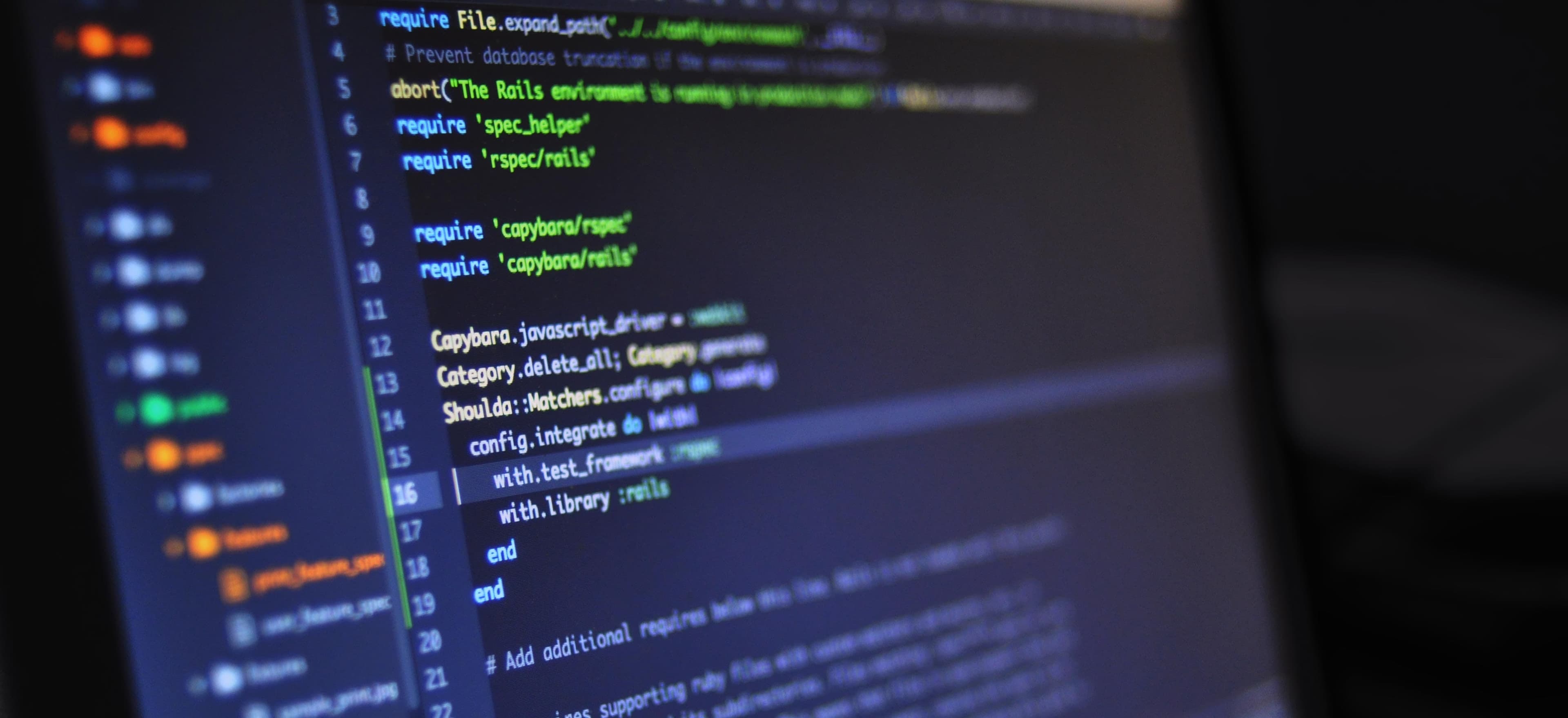
- Published on
Common Pitfalls When Using Lombok in Java Projects
Lombok is a popular Java library that helps reduce boilerplate code in your projects. It allows developers to streamline their Java classes by automatically generating getters, setters, toString methods, and more through annotations. While it can significantly enhance productivity and code clarity, it can also lead to some common pitfalls. This post will explore these pitfalls, provide examples, and offer alternative approaches for effective usage of Lombok.
What is Lombok?
Lombok is a Java library that provides various annotations to help developers minimize boilerplate code. It works at compile time by modifying the Abstract Syntax Tree (AST) of Java classes during the build process. This makes it lightweight, and you do not have to include any runtime overhead. Common Lombok annotations include:
@Getter
and@Setter
: Generate getter and setter methods for class fields.@Data
: Combines@Getter
,@Setter
,@ToString
,@EqualsAndHashCode
, and@RequiredArgsConstructor
.@Builder
: Implements the builder pattern.
import lombok.Data;
@Data
public class User {
private String name;
private int age;
}
In the example above, a class called User
is created with fields name
and age
. The @Data
annotation automatically generates all necessary method implementations.
Pitfall 1: Overusing @Data
One of the most common pitfalls is the overuse of the @Data
annotation. Although it looks convenient for quick setups, it generates a lot of methods that may not be necessary for your domain model.
Why It Matters
Using @Data
indiscriminately can clutter your class with methods that you might not require, such as toString()
, equals()
, and hashCode()
. Additionally, the generated methods may introduce bugs if not implemented with your domain logic in mind.
Alternative Approach
Instead of using @Data
, opt for more specific annotations to generate only what you need:
import lombok.Getter;
import lombok.Setter;
import lombok.EqualsAndHashCode;
@Getter
@Setter
@EqualsAndHashCode
public class User {
private String name;
private int age;
}
In this example, we only generate the necessary getter and setter methods along with an equals
and hashCode
method, keeping our class leaner and more meaningful.
Pitfall 2: Lack of Explicit Constructors
When using Lombok, developers may overlook the benefits of defining explicit constructors. The use of @NoArgsConstructor
, @AllArgsConstructor
, and @RequiredArgsConstructor
can lead to the absence of a meaningful constructor in the class.
Why It Matters
A clear constructor can enhance the readability and maintainability of the code. Indistinct or implicit constructors can make it harder for new developers to understand how to instantiate objects correctly.
Alternative Approach
Explicitly define constructors when necessary:
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.Setter;
@Getter
@Setter
@AllArgsConstructor
public class User {
private String name;
private int age;
}
Here, we use @AllArgsConstructor
, but we can also introduce custom logic in the constructors, enhancing clarity.
Pitfall 3: Confusion with Inheritance
When working with inheritance, Lombok can sometimes lead to confusion, especially with generated methods from parent classes. For example, if a superclass has Lombok's annotations, the behavior of the inherited methods can be unpredictable.
Why It Matters
Inheritance-related issues can arise, leading to unexpected behaviors in overridden and inherited methods. This ambiguity is often overlooked and can cause intricate bugs.
Best Practice
Define your Lombok annotations thoughtfully within the class hierarchy. Be explicit about which methods you want to override and when:
import lombok.Getter;
import lombok.Setter;
@Getter
@Setter
public class Person {
protected String name;
}
@Getter
@Setter
public class User extends Person {
private int age;
@Override
public String toString() {
return "User{name='" + name + "', age=" + age + "}";
}
}
In this code, the toString
method is explicitly defined in the User
class, avoiding confusion from Lombok's implicit behavior.
Pitfall 4: Serialization Issues
Lombok-generated methods can cause issues with serialization. When using libraries like Jackson or Gson to serialize objects, Lombok annotations can conflict with expected behaviors, leading to unplanned serialization outputs.
Why It Matters
Serialization is critical for transferring object states. If the serialized data does not match what you expect, it could lead to significant issues during data transfer or persistence.
Best Practice
Use specific Lombok features to prevent unwanted side effects. Consider employing both Lombok and Jackson annotations appropriately:
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.Data;
@Data
public class User {
@JsonProperty("username")
private String name;
private int age;
}
In this case, we explicitly control how the name
field should appear in JSON output, while still leveraging Lombok for reducing boilerplate.
Pitfall 5: Difficulty in Troubleshooting
Another frequent problem is troubleshooting. While Lombok significantly reduces the amount of code, it can complicate debugging. Stack traces may not provide meaningful insight because the generated code is not visible in the IDE.
Why It Matters
When facing runtime errors, missing methods in your class can result in time-consuming debugging, especially for less experienced developers.
Best Practices
Maintain an understanding of the methods generated by Lombok. Keep your IDE configured to show Lombok-generated methods, and be sure to keep unit tests robust to pinpoint issues rapidly.
My Closing Thoughts on the Matter
Lombok is a powerful library that can enhance productivity, but it's essential to use it thoughtfully. Understanding the common pitfalls—such as overusing @Data
, neglecting constructors, issues with inheritance, serialization conflicts, and troubleshooting challenges—can save time and ensure the success of your Java projects.
Additional Resources
- Lombok Official Documentation
- Baeldung's Guide to Using Lombok
- Maven Lombok Plugin
By considering the information and suggestions highlighted in this article, you can effectively leverage Lombok in your Java projects while avoiding potential pitfalls. Happy coding!
Checkout our other articles