Common JSoup Errors When Parsing HTML in Java
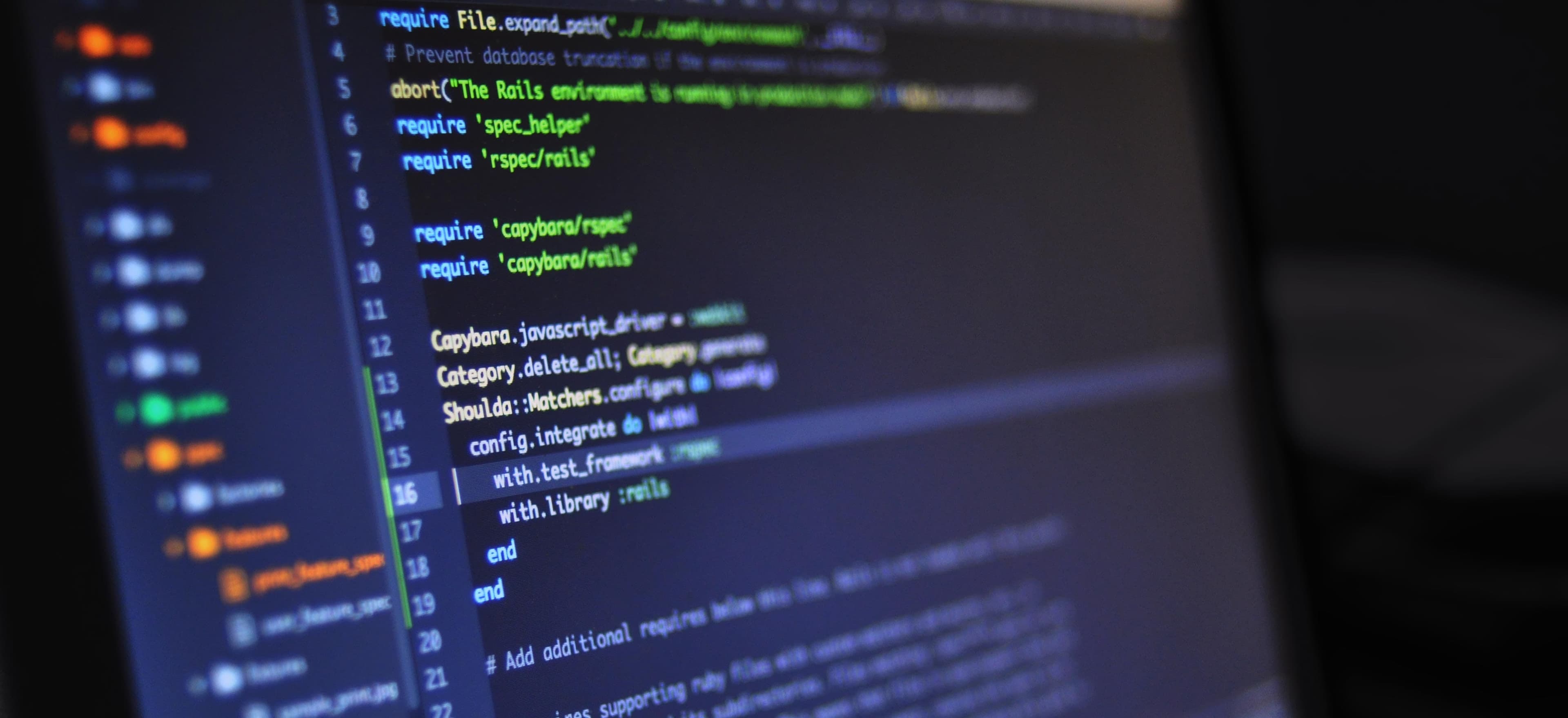
- Published on
Common JSoup Errors When Parsing HTML in Java
In the realm of web scraping and HTML parsing, Java developers often turn to libraries that simplify the process. One such library is JSoup, a powerful tool for working with real-world HTML. However, even the most robust libraries can present challenges. In this blog post, we will cover common JSoup errors that developers encounter when parsing HTML documents, alongside practical solutions and tips to avoid these pitfalls.
What is JSoup?
Before diving into error management, let's briefly explain JSoup. JSoup is a Java library designed for parsing, manipulating, and extracting data from HTML documents. Its syntax is intuitive, modeled after jQuery, making it particularly friendly for those familiar with web technologies.
The power of JSoup lies in its ability to handle messy HTML, a common occurrence when scraping data from the web. It can manipulate the DOM (Document Object Model), making it an essential choice for developers engaged in web scraping.
Common Errors in JSoup
1. MalformedURLException
One of the most common issues you might face when using JSoup is the MalformedURLException
. This error typically occurs when trying to connect to a URL that is incorrectly formatted.
Example:
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
public class JSoupExample {
public static void main(String[] args) {
try {
Document doc = Jsoup.connect("htp://example.com").get(); // Incorrect URL scheme
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
}
}
Why It Occurs:
In the example above, the URL is incorrectly formatted (using "htp" instead of "http"). This results in a MalformedURLException
.
Solution:
Always ensure the URL starts with http://
or https://
and is correctly formatted. Use tools like URLValidator if needed.
2. IOException
Another common exception is IOException
, which can occur for several reasons, such as network issues or when trying to access a non-existing page.
Example:
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
public class JSoupExample {
public static void main(String[] args) {
try {
Document doc = Jsoup.connect("http://nonexistentwebsite.com").get();
} catch (IOException e) {
System.out.println("IO Exception: " + e.getMessage());
}
}
}
Why It Occurs:
In the above example, trying to connect to a non-existing website results in an IOException
. It could also occur due to timeouts.
Solution:
Be prepared to handle IOException
. Always check if the URL is reachable. You can also implement retry logic to manage temporary connectivity issues.
3. NullPointerException
When you attempt to retrieve elements from a document and they do not exist, you're likely to encounter a NullPointerException
.
Example:
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
public class JSoupExample {
public static void main(String[] args) {
try {
Document doc = Jsoup.connect("http://example.com").get();
Element element = doc.select("div.nonexistent").first(); // Nonexistent selector
System.out.println(element.text()); // This will throw NullPointerException
} catch (NullPointerException e) {
System.out.println("Null Pointer Exception: " + e.getMessage());
} catch (IOException e) {
System.out.println("IO Exception: " + e.getMessage());
}
}
}
Why It Occurs:
In the above code, trying to access a nonexistent <div>
results in a null element, which leads to a NullPointerException
.
Solution:
It's crucial to check if the element is not null
before proceeding to manipulate or extract data from it:
if (element != null) {
System.out.println(element.text());
} else {
System.out.println("Element not found.");
}
4. Parsing HTML Issues
Sometimes, the HTML structure might be malformed or not standard, causing JSoup to parse it incorrectly.
Example:
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
public class JSoupExample {
public static void main(String[] args) {
String html = "<html><head><title>Test</title></head><body><h1>Sample</h1></body>"; // Malformed HTML
Document doc = Jsoup.parse(html);
System.out.println(doc.title()); // This will throw an error
}
}
Why It Occurs:
Malformed HTML, such as missing closing tags, leads to incorrect parsing by JSoup.
Solution:
Before parsing, always validate your HTML. JSoup is fairly resilient, but you can enhance your parsing by using tidyHtml or implementing error-handling strategies.
Document doc = Jsoup.parseBodyFragment(html); // For partial HTML inputs
5. HTTP Errors and Status Codes
Another common error occurs when the server responds with an HTTP status code indicating a failure (like 404 for Not Found or 500 for Server Error).
Example:
import org.jsoup.Connection;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
public class JSoupExample {
public static void main(String[] args) {
try {
Connection.Response response = Jsoup.connect("http://httpstat.us/404").execute();
if (response.statusCode() != 200) {
System.out.println("HTTP Error: " + response.statusCode());
} else {
Document doc = response.parse();
System.out.println(doc.title());
}
} catch (IOException e) {
System.out.println("IO Exception: " + e.getMessage());
}
}
}
Why It Occurs:
Attempting to parse a document from an error response will lead to issues in data extraction.
Solution:
Always check the response status code before proceeding. A status code of 200 means success, while anything else indicates an error.
A Final Look
Mastering HTML parsing with JSoup opens up a plethora of opportunities for web scraping and data extraction in Java. Yet, as we have discussed, it is critical to be aware of common errors such as MalformedURLException
, IOException
, NullPointerException
, issues with parsed HTML, and HTTP errors.
By understanding these challenges and implementing appropriate handling measures, your experience with JSoup and web scraping will become seamless and efficient.
For further reading on JSoup, check the official JSoup documentation which offers detailed guides and examples.
Additional Resources
Using these insights, handle errors effectively and make the most of your JSoup experience! Happy coding!
Checkout our other articles