Handling Exceptions in Java Lambdas: A Developer's Guide
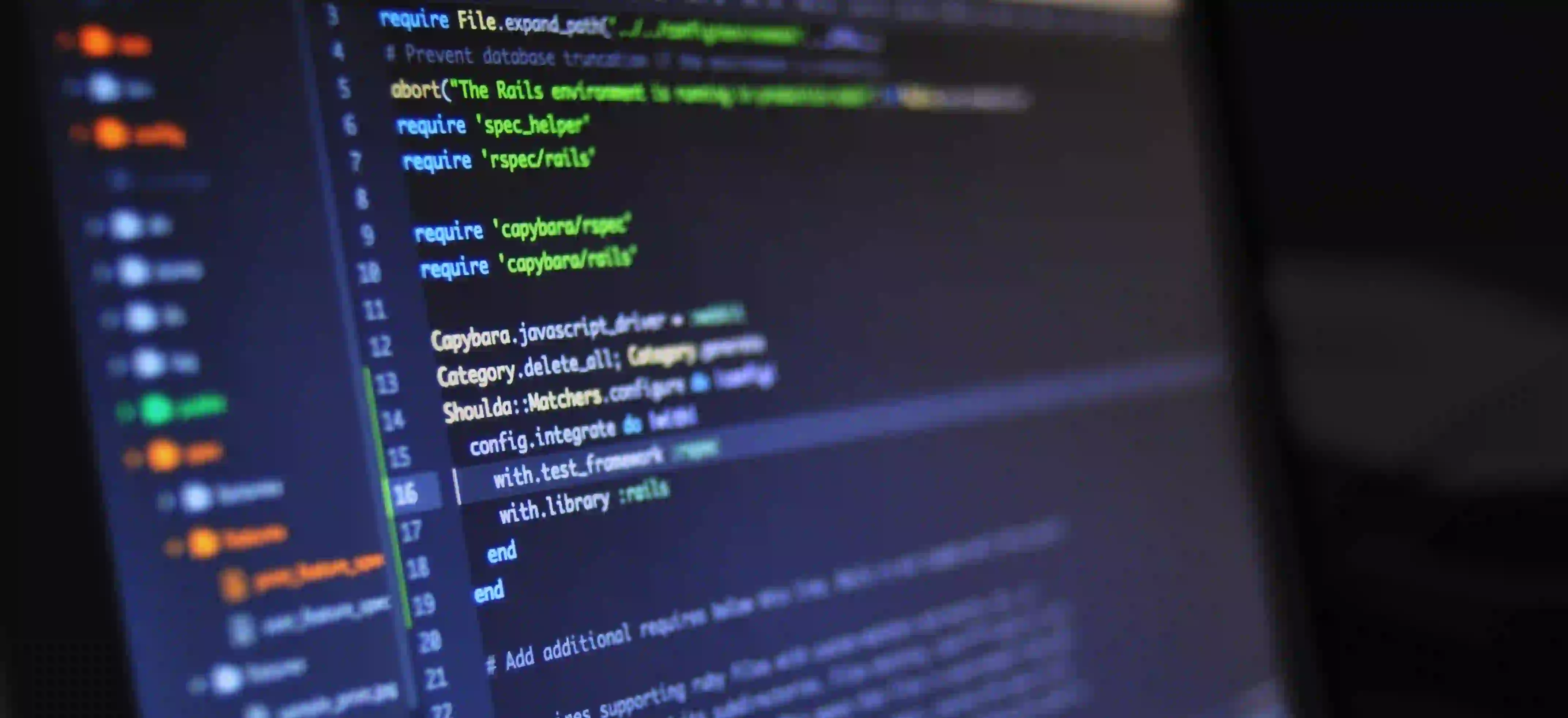
Handling Exceptions in Java Lambdas: A Developer's Guide
Java has evolved significantly over the years, introducing features that improve coding efficiency and readability. One of the standout additions is the lambda expression, which allows for a more functional programming style. However, one issue that developers often encounter when using lambdas is exception handling. In this blog post, we will explore how to effectively manage exceptions in Java lambdas, ensuring robust and maintainable code.
What Are Java Lambdas?
Before diving into exception handling, it's crucial to understand what lambdas are and why they are useful. Introduced in Java 8, lambdas offer a way to implement functional interfaces (interfaces with a single abstract method) in a more concise manner.
Example of a Lambda Expression
Here's a simple example of a lambda expression:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name));
In this snippet, we use a lambda to print each name from the list. The syntactical simplicity is one reason why lambdas are popular among developers.
The Problem with Exceptions in Lambdas
A lambda expression captures variables from its enclosing context, but any checked exceptions thrown inside a lambda need to be handled properly. Java's mandatory exception handling can create challenges when reworking existing code with lambdas.
Why Is This an Issue?
When you try to throw a checked exception from a lambda, the compiler needs to know how to handle that exception. For example:
Runnable runnable = () -> {
throw new IOException("An IO error occurred");
};
The above code will result in a compilation error, as the IOException
is a checked exception, and lambdas do not have the same exception handling feature that traditional methods do.
Handling Exceptions in Lambda Expressions
To effectively handle exceptions within lambda expressions, there are several strategies you can employ:
- Wrap Exceptions: Create a custom functional interface that allows for exception handling.
- Use Try-Catch Blocks: Handle exceptions directly inside the lambda.
- Wrap the Lambda in Try-Catch: Create methods that can wrap your lambdas in try-catch blocks.
Option 1: Create Custom Functional Interfaces
By creating a custom functional interface that allows checked exceptions, you can maintain clean and concise lambda expressions. Below is a simple implementation.
@FunctionalInterface
interface CheckedConsumer<T> {
void accept(T t) throws Exception;
}
public class ExceptionHandlingExample {
public static <T> Consumer<T> wrap(CheckedConsumer<T> consumer) {
return t -> {
try {
consumer.accept(t);
} catch (Exception e) {
throw new RuntimeException(e);
}
};
}
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(wrap(name -> {
if (name.equals("Bob")) {
throw new IOException("Custom exception for Bob");
}
System.out.println(name);
}));
}
}
Why Use Custom Functional Interfaces?
Using custom functional interfaces like CheckedConsumer
allows you to throw exceptions directly from within your lambda without breaking the functional programming paradigm. Wrapping the exception inside a RuntimeException
keeps the flow of the program intact.
Option 2: Use Try-Catch Blocks Inside Lambdas
For simpler cases, handling exceptions directly within the lambda body can be a quick solution. Here’s an example:
public class ExceptionHandlingDirectly {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> {
try {
if (name.equals("Bob")) {
throw new IOException("Bob encountered an issue.");
}
System.out.println(name);
} catch (IOException e) {
System.err.println("Exception: " + e.getMessage());
}
});
}
}
Why Use Try-Catch Inside Lambdas?
This approach offers simplicity and clarity, especially for smaller applications or scripts. However, note that it can lead to repetitive code if the same exception handling logic is applied across multiple lambdas.
Option 3: Wrapping Lambdas in Try-Catch
You can create higher-order methods that accept lambdas. The alternative is to handle exceptions outside of your lambda, passing the lambda as a parameter to a method designed to catch exceptions.
public class ExceptionHandlingWrapper {
public static <T> void handle(Consumer<T> consumer, T t) {
try {
consumer.accept(t);
} catch (Exception e) {
System.err.println("Exception: " + e.getMessage());
}
}
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> handle(t -> {
if (t.equals("Bob")) {
throw new IOException("Bob cannot be processed.");
}
System.out.println(t);
}, name));
}
}
Why Use a Wrapper Method?
This strategy promotes code reuse and keeps lambdas cleaner. It separate exception handling from the business logic of the lambda, enhancing readability.
Best Practices for Exception Handling in Java Lambdas
Here are some best practices to keep in mind when dealing with exception handling in Java lambdas:
- Use Custom Functional Interfaces: Whenever possible, define your own functional interfaces to better manage checked exceptions.
- Keep Lambdas Clean: Try to limit the logic inside lambda expressions, utilizing methods for complex operations, including exception handling.
- Be Consistent: Maintain a consistent approach across your application or project. This reduces confusion for you and other developers.
- Log Carefully: When catching exceptions, ensure you log them appropriately for debugging purposes.
The Bottom Line
Exception handling in Java lambdas can be challenging but is manageable with the right strategies. By leveraging custom functional interfaces, employing try-catch blocks, or wrapping lambdas in error-handling methods, you can create clean, effective code that remains robust in the face of errors.
These patterns promote cleaner code and enhance application stability. For an in-depth look at Java functional programming, check out Oracle’s Official Documentation.
Feel free to reach out with your thoughts, questions, or experiences regarding exception handling in Java lambdas. Happy coding!