Common Java Puzzles That Trip Up OCA Exam Candidates
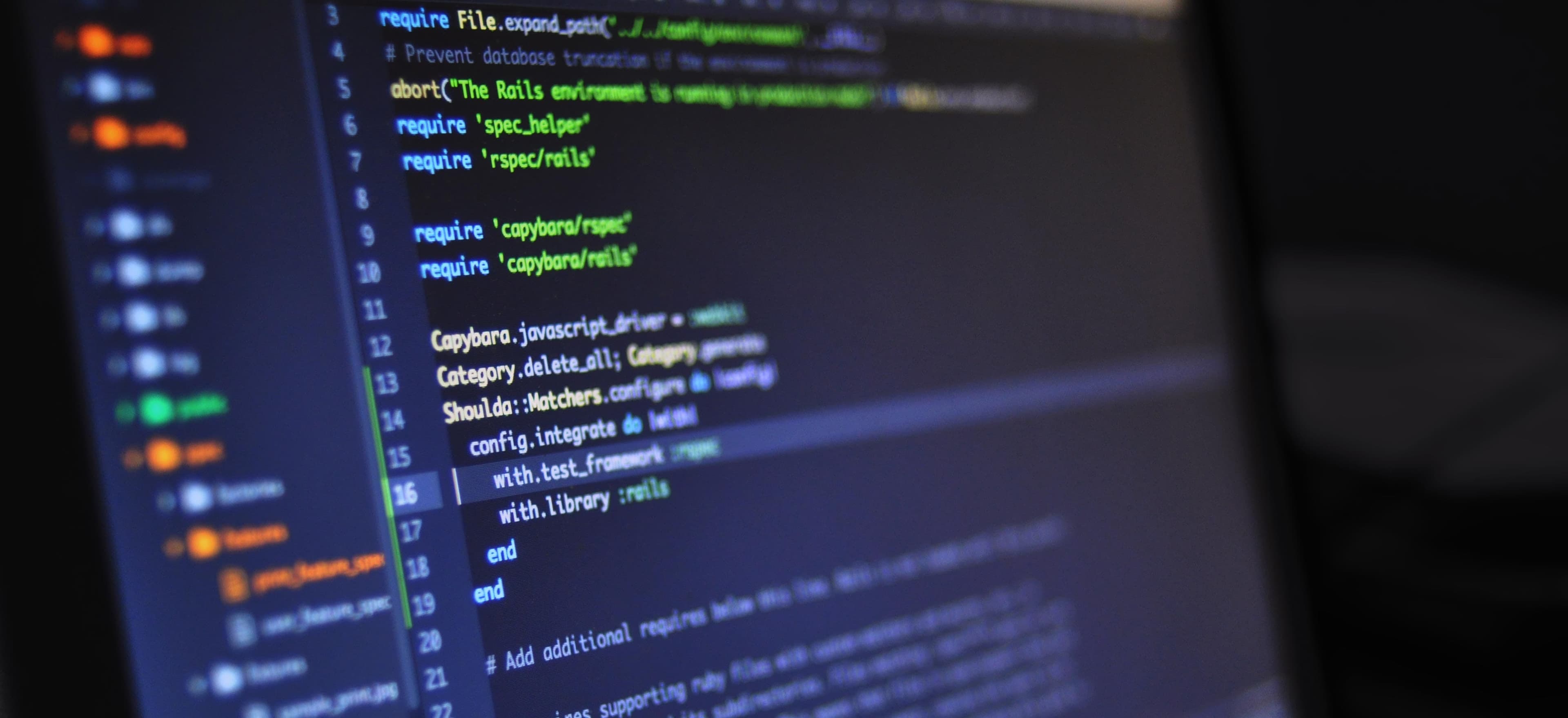
- Published on
Common Java Puzzles That Trip Up OCA Exam Candidates
The Oracle Certified Associate (OCA) exam for Java is a significant milestone for aspiring Java developers. While the exam tests your knowledge of the Java programming language, many candidates often stumble upon common puzzles that can confuse even seasoned programmers. In this blog post, we'll explore these Java puzzles, providing clarity and insight that will help you prepare for the OCA exam.
What are Java Puzzles?
Java puzzles are tricky coding problems or scenarios that reveal potential misunderstandings or false assumptions about how Java operates. They often exploit nuances in the language’s behavior, leading to unexpected results. Understanding these puzzles can deepen your knowledge and increase your chances of success in the OCA exam.
Puzzle 1: Object Equality
Scenario
Consider the following code snippet:
String a = new String("hello");
String b = new String("hello");
System.out.println(a == b);
System.out.println(a.equals(b));
Explanation
- The
==
operator checks if botha
andb
reference the same object in memory. Since we created two separateString
objects, this expression evaluates tofalse
. - On the other hand, the
equals()
method compares the values of the objects, which in this case are both "hello". Thus,a.equals(b)
evaluates totrue
.
Key Takeaway
Always use equals()
to compare string values unless you specifically need to check if two reference variables point to the same object.
Puzzle 2: Integer Caching
Scenario
Consider this code snippet:
Integer x = 100;
Integer y = 100;
System.out.println(x == y);
Explanation
In this case, both x
and y
will output true
. This is because Java caches all Integer objects in the range of -128 to 127. So, x
and y
refer to the same cached object.
However, when you set:
Integer x = 200;
Integer y = 200;
System.out.println(x == y);
The output will be false
, as two distinct objects are created for integers greater than 127.
Key Takeaway
Be cautious about using ==
with Integer
objects. Always use equals()
for value comparison, especially with numbers outside the caching range.
Puzzle 3: Autoboxing and Null
Scenario
Consider the following snippet:
Integer a = null;
int b = a;
Explanation
This code will throw a NullPointerException
. Java attempts to unbox a
into an int
, but since a
is null
, it can't convert it to a primitive type.
Key Takeaway
Understand the difference between primitive types and wrapper classes. Autoboxing and unboxing can lead to exceptions if not handled carefully.
Puzzle 4: Switch Statement with Strings
Scenario
Java allows you to use strings in a switch statement starting from Java 7. But many candidates have misconceptions about it.
String fruit = "apple";
switch (fruit) {
case "apple":
System.out.println("This is an apple.");
break;
case "banana":
System.out.println("This is a banana.");
break;
default:
System.out.println("Unknown fruit.");
}
Explanation
The code above works as expected. However, some candidates worry about performance and whether strings create multiple objects. The underlying mechanism uses the String::hashCode
to create a jump table, which is efficient.
Key Takeaway
Don’t shy away from using strings in switch statements; they’re efficient and can enhance code readability.
Puzzle 5: Final Variables
Scenario
Consider this piece of code:
final int x = 10;
x++;
Explanation
This code will result in a compilation error. The final
modifier prevents the variable x
from being modified after it has been initialized. The moment you try to change its value with x++
, it violates the final
declaration.
Key Takeaway
Be mindful of how the final
keyword works. It is used to make variables, methods, or classes immutable, leading to clarity in your code.
Puzzle 6: The Diamond Problem
Java does not allow multiple inheritance with classes to simplify the language and avoid complexity. However, with interfaces, it allows multiple inheritance but comes with some nuances.
interface A {
default void show() {
System.out.println("Show A");
}
}
interface B {
default void show() {
System.out.println("Show B");
}
}
class C implements A, B {
public void show() {
A.super.show(); // Calls show from A
}
}
Explanation
In this code, Class C
implements both interface A
and B
, each providing a default implementation for the show()
method. If C
does not provide its own show()
method, the compiler will give an error due to the "diamond problem."
Key Takeaway
When working with multiple interfaces that have default methods, always implement the method in your class to avoid ambiguity.
Preparing for the Exam
As you prepare for your OCA exam, keep these Java puzzles in mind. Regular practice and understanding of these concepts will not only make you a better Java programmer but also pave the way for passing the exam. Consider revising from Oracle's official guide to solidify your knowledge.
Closing Remarks
Java puzzles can be tricky but mastering them will prepare you for the challenges ahead, not only in the OCA exam but also in your Java development career. Make sure to practice these concepts and relate them to real-world scenarios. Remember, understanding the 'why' behind code behaviors is crucial.
Happy coding!