Common Pitfalls When Setting Up Undertow Web Server
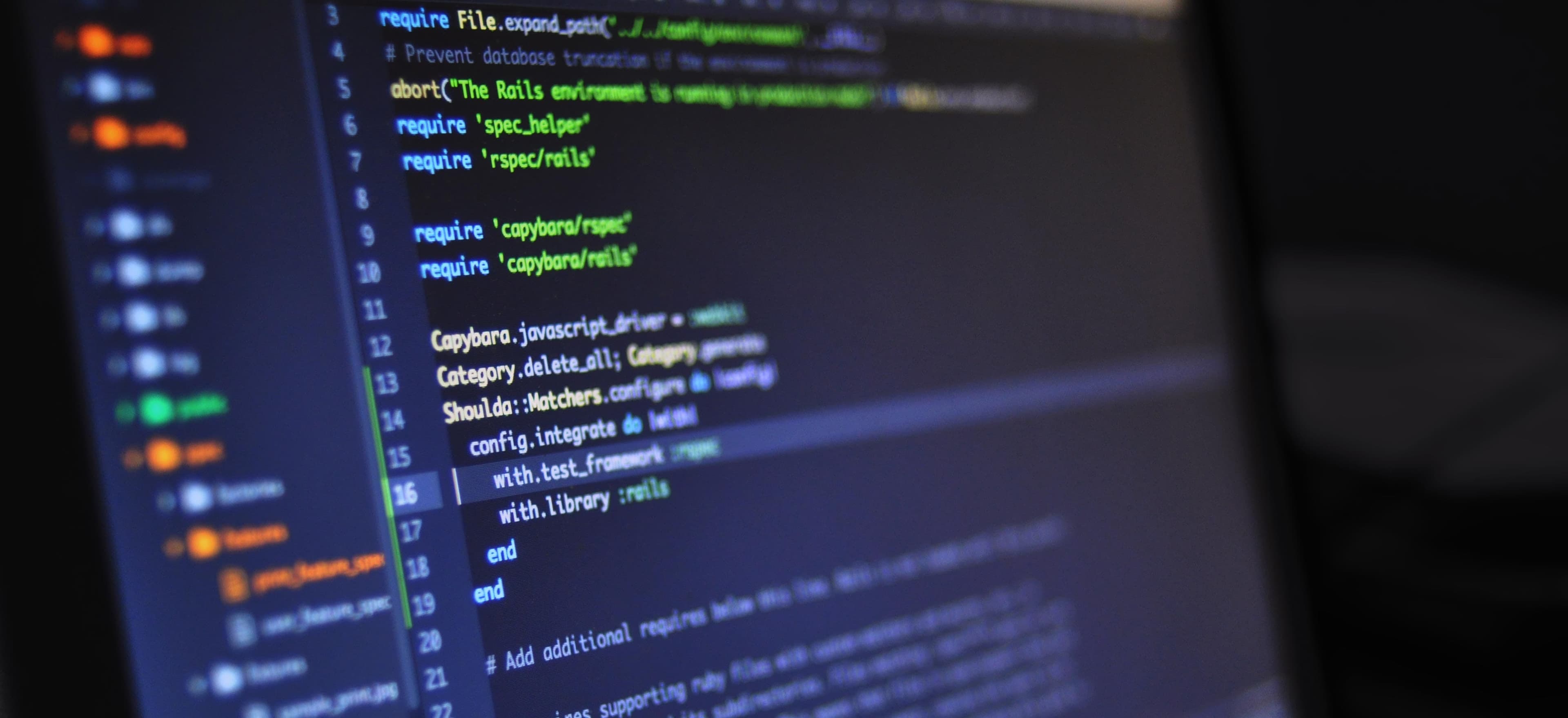
- Published on
Common Pitfalls When Setting Up Undertow Web Server
Undertow is a flexible, performant web server designed for both standalone and embedded usage. Built on the foundations of asynchronous processing, Undertow provides ways to create non-blocking applications with high throughput. However, like any technology, there are common pitfalls that developers might encounter while setting up Undertow. This blog post outlines these pitfalls, guiding you to set up a successful Undertow server while avoiding common missteps.
Understanding Undertow
First, let's clarify what makes Undertow stand out. Unlike traditional servers that use synchronous processing as a default, Undertow harnesses the power of asynchronous processing. This design efficiently handles a large number of concurrent connections, which is crucial for web applications today.
Why Use Undertow?
- Performance: Undertow is lightweight and delivers high performance in handling requests.
- Flexible: It supports HTTP/1.x and HTTP/2, making it a versatile option for modern web applications.
- Embedded Deployment: Undertow can be easily embedded into Java applications, enhancing the development process.
Before diving into the setup mistakes developers often make, let us take a look at a simple setup code snippet:
import io.undertow.Undertow;
public class UndertowServer {
public static void main(String[] args) {
Undertow server = Undertow.builder()
.addHttpListener(8080, "0.0.0.0")
.setHandler(exchange -> {
exchange.getResponseHeaders().put(
io.undertow.util.Headers.CONTENT_TYPE, "text/plain");
exchange.getResponseSender().send("Hello, Undertow!");
})
.build();
server.start();
System.out.println("Server started on http://localhost:8080");
}
}
In the above snippet, we have set up a very basic Undertow server. However, as you expand on this, be aware of several pitfalls.
Common Pitfalls When Setting Up Undertow Web Server
1. Improper Port Configuration
When deploying Undertow, you need to carefully configure the server's listener ports. Misconfiguring these can lead to failure in starting the server or conflicts with other applications.
Solution
Ensure the port you choose is not occupied by another service. Use an option like:
.addHttpListener(8080, "0.0.0.0")
This snippet listens on port 8080 for incoming requests. Always check if this port is available to avoid conflicts.
2. Ignoring Asynchronous Processing
One of the standout features of Undertow is its non-blocking nature. However, many developers overlook this and inadvertently write synchronous code.
Why It Matters
Blocking calls can lead to thread exhaustion and performance bottlenecks. Adopt asynchronous processing to maximize Undertow's capabilities.
Example Code
Here's an example of non-blocking I/O:
import io.undertow.server.HttpServerExchange;
public class AsyncHandler implements HandlerWrapper {
@Override
public HttpHandler wrap(HttpHandler handler) {
return exchange -> {
exchange.getResponseSender().send("Processing...");
performAsyncTask(exchange);
};
}
private void performAsyncTask(HttpServerExchange exchange) {
CompletableFuture.runAsync(() -> {
// Simulate a long-running task
try {
Thread.sleep(2000);
exchange.getResponseSender().send("Async task completed");
} catch (InterruptedException e) {
e.printStackTrace();
}
});
}
}
This example showcases how to perform an asynchronous operation, preventing blocking the server’s main thread.
3. Inadequate Exception Handling
Poor exception handling can lead to unhandled errors crashing the application. This is often overlooked during development.
Good Practices
- Use a centralized error handler.
- Log necessary exceptions to identify issues early.
Example Code Snippet
Here's an example of handling errors in Undertow:
.addHttpListener(8080, "0.0.0.0")
.setHandler(exchange -> {
try {
// Your handling logic
} catch (Exception e) {
exchange.setStatusCode(500);
exchange.getResponseSender().send("Internal Server Error");
// Log the exception for debugging
System.err.println("Error: " + e.getMessage());
}
})
By properly managing exceptions, you ensure the server continues running smoothly even under unexpected conditions.
4. Ignoring Dependency Management
Another common error involves overlooking dependency issues. Users may not specify the correct version of Undertow or its dependencies, leading to runtime exceptions.
Dependency Management
Utilize a build tool like Maven or Gradle to manage dependencies effectively.
<dependency>
<groupId>io.undertow</groupId>
<artifactId>undertow-core</artifactId>
<version>2.2.0.Final</version> <!-- Ensure you are using a stable version -->
</dependency>
Using a stable and maintained version helps avoid inconsistencies and bugs.
5. Lack of Configuration for HTTP/2
While Undertow supports HTTP/2, many developers fail to enable it. HTTP/2 significantly improves performance and user experience through reduced latency and improved resource loading.
Enabling HTTP/2
Here's how to enable HTTP/2 in your Undertow configuration:
.addHttpsListener(8443, "0.0.0.0", sslContext) // Enable SSL for HTTP/2
.setHandler(exchange -> {
// Your handler logic
});
Make sure to configure SSL as HTTP/2 requires a secure connection. Learn more about configuring HTTP/2 here.
6. Not Using the Right Middleware Components
Undertow allows for a modular setup via handlers. Developers often fail to utilize the appropriate middleware that can assist in the request processing lifecycle.
Examples of Useful Middleware
- CORS Support: Setup to help manage CORS requests easily.
- Static Content Handling: Efficiently serve static files without writing a custom handler.
In conclusion, to leverage the full capabilities of Undertow, you must avoid these common pitfalls. Always ensure proper configuration, manage dependencies wisely, and utilize asynchronous processing methods. By keeping these points in mind upon setting up Undertow, you will pave the way for a more stable and performant application.
For additional resources and advanced configuration details, refer to the Undertow Documentation. Happy coding!