Common Concurrency Issues in Java Web Applications
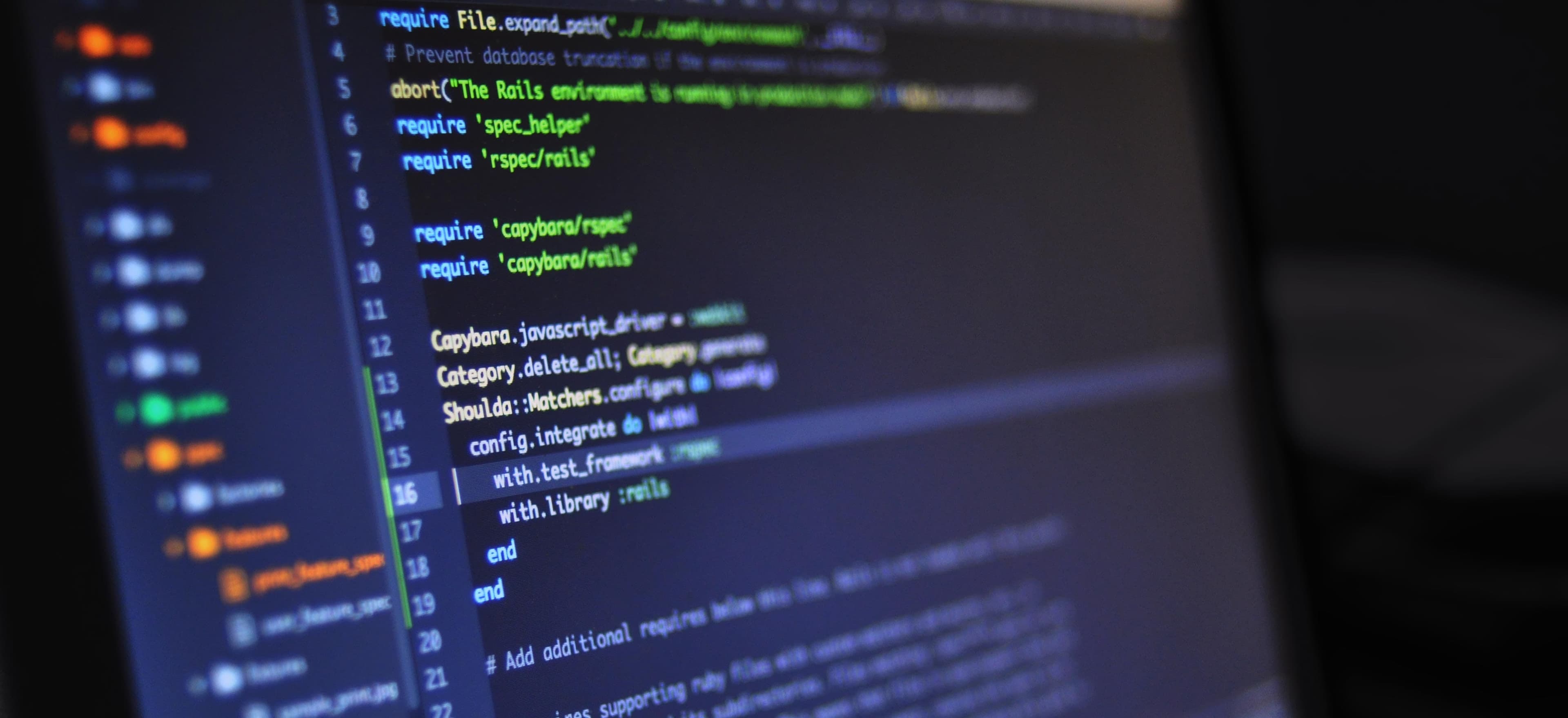
- Published on
Common Concurrency Issues in Java Web Applications
Concurrency in Java is a crucial aspect when developing web applications, particularly in a multi-threaded environment. Understanding the common concurrency issues can greatly enhance the reliability and scalability of your applications. This blog post will discuss essential concepts related to concurrency in Java, common issues developers face, and best practices to mitigate these problems.
Understanding Concurrency in Java
Concurrency refers to the ability of a system to perform multiple tasks simultaneously. In Java, concurrency is handled through multiple threads. A thread is the smallest unit of processing that can be scheduled by the operating system and can be used to perform various tasks in parallel.
Java provides a built-in concurrency framework that includes classes from java.util.concurrent
, which makes it easier to work with threads. However, with concurrent programming comes the risk of various issues that can affect application performance and data integrity. Let's explore some of these common concurrency problems.
1. Race Conditions
A race condition occurs when two or more threads access shared data and try to change it at the same time. If the threads do not synchronize their access, it may result in unexpected behavior or inconsistent data states.
Example
Consider a simple bank account class where multiple threads might update the account balance:
public class BankAccount {
private int balance;
public BankAccount(int initialBalance) {
this.balance = initialBalance;
}
public void deposit(int amount) {
balance += amount;
}
public void withdraw(int amount) {
balance -= amount;
}
public int getBalance() {
return balance;
}
}
Here, if two threads call the withdraw
method at the same time, they might read the same balance before either of them has updated it. This can lead to a situation where the balance is incorrect.
Solution
To avoid race conditions, we can use the synchronized
keyword to ensure that only one thread can execute a method at a time.
public synchronized void deposit(int amount) {
balance += amount;
}
By synchronizing the method, we ensure that the critical section of code that modifies the balance is accessed by only one thread at a time. For further reading on concurrency and synchronization, check out the Java Tutorials on Multithreading provided by Oracle.
2. Deadlocks
A deadlock occurs when two or more threads are blocked forever, waiting for each other to release resources. This typically happens when each thread holds a resource and tries to acquire a resource held by another thread.
Example
Consider two threads where each one locks a different resource:
public class Resource {
private final String name;
public Resource(String name) {
this.name = name;
}
public synchronized void methodA(Resource resource) {
System.out.println(Thread.currentThread().getName() + " locked " + this.name);
resource.methodB();
}
public synchronized void methodB() {
System.out.println(Thread.currentThread().getName() + " locked " + this.name);
}
}
If Thread 1 locks Resource A and Thread 2 locks Resource B, and then each thread tries to call a method on the other, a deadlock will occur.
Solution
To avoid deadlocks, ensure that all threads acquire locks in a consistent order. Doing so greatly reduces the chances of deadlock. For more advanced techniques, consider using Lock Objects from java.util.concurrent, which offer more control over locking compared to synchronized methods.
3. Starvation
Starvation occurs when a thread is perpetually denied access to resources. This can happen due to high-priority threads constantly being given preference over lower-priority threads. In Java, this can happen when using locks or synchronized blocks where certain threads are not allowed to execute.
Example
Starvation could occur in this situation:
class HighPriorityTask implements Runnable {
public void run() {
// Task with high priority
}
}
class LowPriorityTask implements Runnable {
public void run() {
// Task with low priority
}
}
If the scheduler continuously executes high-priority tasks, low-priority tasks may never get executed.
Solution
To avoid starvation, be cautious about thread prioritization. Use fair locks from java.util.concurrent.locks
that allow threads to wait in a fair manner.
ReentrantLock lock = new ReentrantLock(true);
Using a fair lock ensures that the longest waiting thread gets the first opportunity to acquire the lock. More details about concurrency utilities can be found in the Java Concurrency in Practice book.
4. Livelocks
Livelocks are similar to deadlocks, but instead of blocking each other, the threads keep changing their state in response to each other, preventing progress.
Example
Consider two threads that continuously keep trying to release resources, but they are unable to:
class LiveLockExample implements Runnable {
private static final Object resource1 = new Object();
private static final Object resource2 = new Object();
public void run() {
synchronized (resource1) {
synchronized (resource2) {
// Perform action
}
}
}
}
If one thread sees a condition and releases its lock to allow the other thread to proceed, it could end up in a loop.
Solution
To avoid livelocks, implement a back-off strategy. When a thread cannot acquire a resource, it waits for a random amount of time before trying again.
5. Visibility Issues
Visibility issues arise when one thread modifies a shared variable, and other threads do not immediately see the updated value. This is particularly common in a multi-threaded environment due to caching and compiler optimization.
Example
In the following case, one thread updates a variable, while another reads it:
public class Visibility {
private static boolean running = true;
public static void main(String[] args) {
Thread t1 = new Thread(() -> {
while (running) {
// Do work
}
});
Thread t2 = new Thread(() -> {
running = false; // Stop the first thread
});
t1.start();
t2.start();
}
}
Thread t1 may never see the updated value of running
, causing it to run indefinitely.
Solution
To ensure visibility, use the volatile
keyword for shared variables. This tells the JVM that the value may be changed by different threads, ensuring proper visibility.
private static volatile boolean running = true;
Using the volatile
keyword guarantees that all threads see the most recent write to the variable.
The Last Word
Concurrency issues in Java web applications can seem daunting, but understanding them is critical for building robust systems. By recognizing common problems like race conditions, deadlocks, starvation, livelocks, and visibility issues, developers can implement effective strategies to mitigate these risks.
Always strive for a solid understanding of the Java concurrency model and leverage best practices, such as using locks, synchronization, and consistent access patterns. For more in-depth insights, resources like Java Concurrency in Practice and the Java Tutorial on Concurrency are invaluable.
Happy coding!