Common Pitfalls in Implementing the Factory Method Pattern
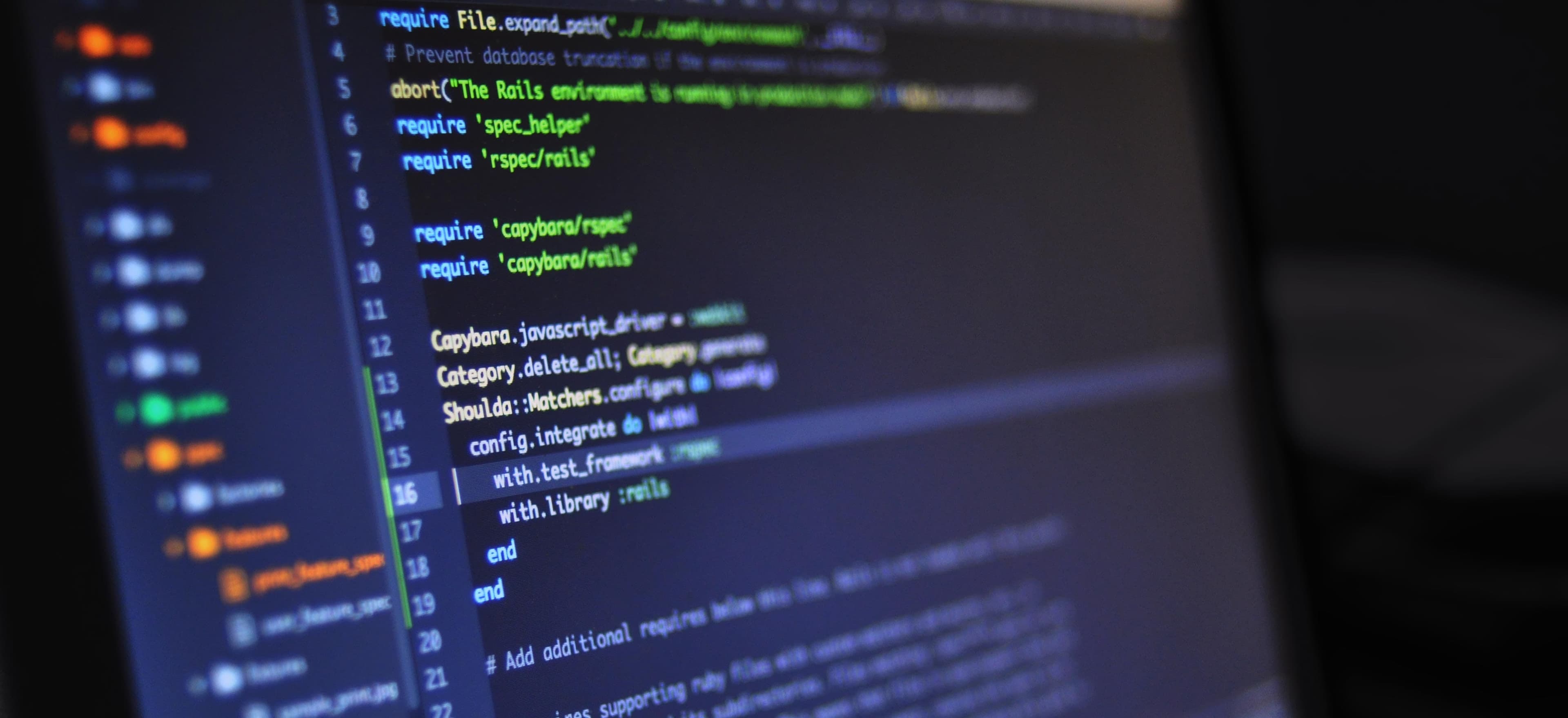
- Published on
Common Pitfalls in Implementing the Factory Method Pattern
The Factory Method pattern is a popular design pattern in object-oriented programming. It enables a class to defer instantiation of an object to subclasses, allowing for greater flexibility when creating objects. However, like any design pattern, it is not free from pitfalls. In this blog post, we’ll explore common pitfalls to watch out for when implementing the Factory Method pattern in Java. This guide is beneficial for both novice and seasoned developers looking to refine their design skills.
Understanding the Factory Method Pattern
Before diving into pitfalls, let's briefly review the Factory Method pattern. As per the Gang of Four (GoF) design patterns, the Factory Method falls under the category of creational patterns. It provides an interface for creating objects in a superclass but allows subclasses to alter the type of objects that will be created.
Key Components
- Product: Defines the interface of objects created by the factory method.
- Concrete Products: Implement the Product interface.
- Creator: Declares the factory method, which returns an object of type Product.
- Concrete Creator: Implements the factory method to return an instance of a Concrete Product.
Basic Example
// Product interface
public interface Product {
void use();
}
// Concrete Product
public class ConcreteProductA implements Product {
public void use() {
System.out.println("Using ConcreteProductA");
}
}
// Concrete Product
public class ConcreteProductB implements Product {
public void use() {
System.out.println("Using ConcreteProductB");
}
}
// Creator
public abstract class Creator {
public abstract Product factoryMethod();
}
// Concrete Creator
public class ConcreteCreatorA extends Creator {
public Product factoryMethod() {
return new ConcreteProductA();
}
}
// Concrete Creator
public class ConcreteCreatorB extends Creator {
public Product factoryMethod() {
return new ConcreteProductB();
}
}
In this straightforward example, Creator
declares a factory method, allowing subclasses to implement their own logic for creating different types of Product
classes.
Now that we have a foundational understanding, let’s delve into some common pitfalls.
Common Pitfalls in Implementing Factory Method Pattern
1. Overcomplicating the Structure
One common mistake is overcomplicating the class structure. While the Factory Method pattern is meant to promote flexibility, creating too many classes can lead to confusion and make the system harder to maintain.
Why it’s a Pitfall
Alternatively, a complicated hierarchy can obscure the real benefit of using a pattern like Factory Method. Developers may find it hard to understand which classes are instantiating what objects.
Solutions
Consider your domain carefully. Only use the Factory Method pattern when it adds clear benefits in terms of flexibility and maintainability. Overusing patterns can create an unnecessary burden.
2. Violating Open/Closed Principle
The Open/Closed Principle states that software entities should be open for extension but closed for modification. When implementing the Factory Method, it's vital to ensure that new products can be added without altering existing code significantly.
Why it’s a Pitfall
If you frequently modify existing factory methods each time a new product is added, you are violating this principle, which can lead to fragile code that is difficult to scale.
Solutions
Design your factory methods to return a more generic type, using interfaces. This allows you to add new implementations without having to modify the existing code.
Example of Violating Open/Closed Principle
// Poor design: Modifying factory method for new products
public class SimpleCreator {
public Product factoryMethod(String type) {
switch (type) {
case "A":
return new ConcreteProductA();
case "B":
return new ConcreteProductB();
// Adding a new product requires modifying this method
case "C":
return new ConcreteProductC();
default:
throw new IllegalArgumentException("Unknown product type");
}
}
}
Instead, consider using a more extensible approach.
Improved Code Snippet
public interface Creator {
Product factoryMethod();
}
public class ProductFactory {
private final Map<String, Supplier<Product>> registeredProducts = new HashMap<>();
public void registerProduct(String name, Supplier<Product> supplier) {
registeredProducts.put(name, supplier);
}
public Product createProduct(String name) {
Supplier<Product> supplier = registeredProducts.get(name);
if (supplier != null) {
return supplier.get();
}
throw new IllegalArgumentException("Product not registered");
}
}
In this approach, products can be added to the factory through a registration process, mitigating the issue of modifying existing code.
3. Ignoring Dependency Injection
Dependency Injection (DI) is crucial for maintaining loose coupling among components. When implementing the Factory Method pattern, failing to utilize DI can result in tightly coupled code and make testing difficult.
Why it’s a Pitfall
Tightly coupled code makes it more challenging to isolate tests or swap out implementations, leading to potential issues with maintainability.
Solutions
Use frameworks like Spring to handle the instantiation of your factories and products. By leveraging DI, the factory and its products can be injected at runtime.
4. Not Considering Performance Overhead
Another pitfall is not considering the performance overhead associated with object creation. The Factory Method pattern can introduce unnecessary complexity when simpler solutions suffice.
Why it’s a Pitfall
Using this pattern excessively for trivial object creation can lead to performance concerns and increased memory usage.
Solutions
Assess whether the pattern adds value to your specific use case. For quick, lightweight objects that are created frequently, the overhead of a factory might not be justified.
5. Misusing Factory Method for Object Caching
Some developers attempt to use the Factory Method pattern for caching instances of products. While this is not inherently wrong, it leads to confusion about the responsibility of the factory.
Why it’s a Pitfall
Combining these two responsibilities – creation and management of lifecycle (caching) – can lead to increased complexity and decreased clarity, making the code harder to follow.
Solutions
Separate concerns by maintaining a caching mechanism outside of the Factory Method. This ensures your factory remains focused solely on object creation.
Summary
The Factory Method pattern can be a powerful tool, but like all design patterns, it brings potential pitfalls. To recap, here are the key takeaways:
- Avoid Overcomplicating the Structure: Keep your design as simple as possible.
- Adhere to the Open/Closed Principle: Design for extension, not modification.
- Utilize Dependency Injection: Keep your classes loosely coupled.
- Consider Performance: Assess the need for the pattern based on your use case.
- Isolate Responsibilities: Separate object creation from lifecycle management.
By understanding these pitfalls and actively working to avoid them, you'll become adept at utilizing the Factory Method pattern effectively. Happy coding!
Feel free to explore additional resources for mastering design patterns in Java, such as the book "Design Patterns: Elements of Reusable Object-Oriented Software" by Erich Gamma et al., and online articles on platforms like Baeldung or Refactoring Guru. These resources can fortify your understanding and implementation of design patterns.