Stop Inheriting Problems: Why Avoid Tests with Inheritance
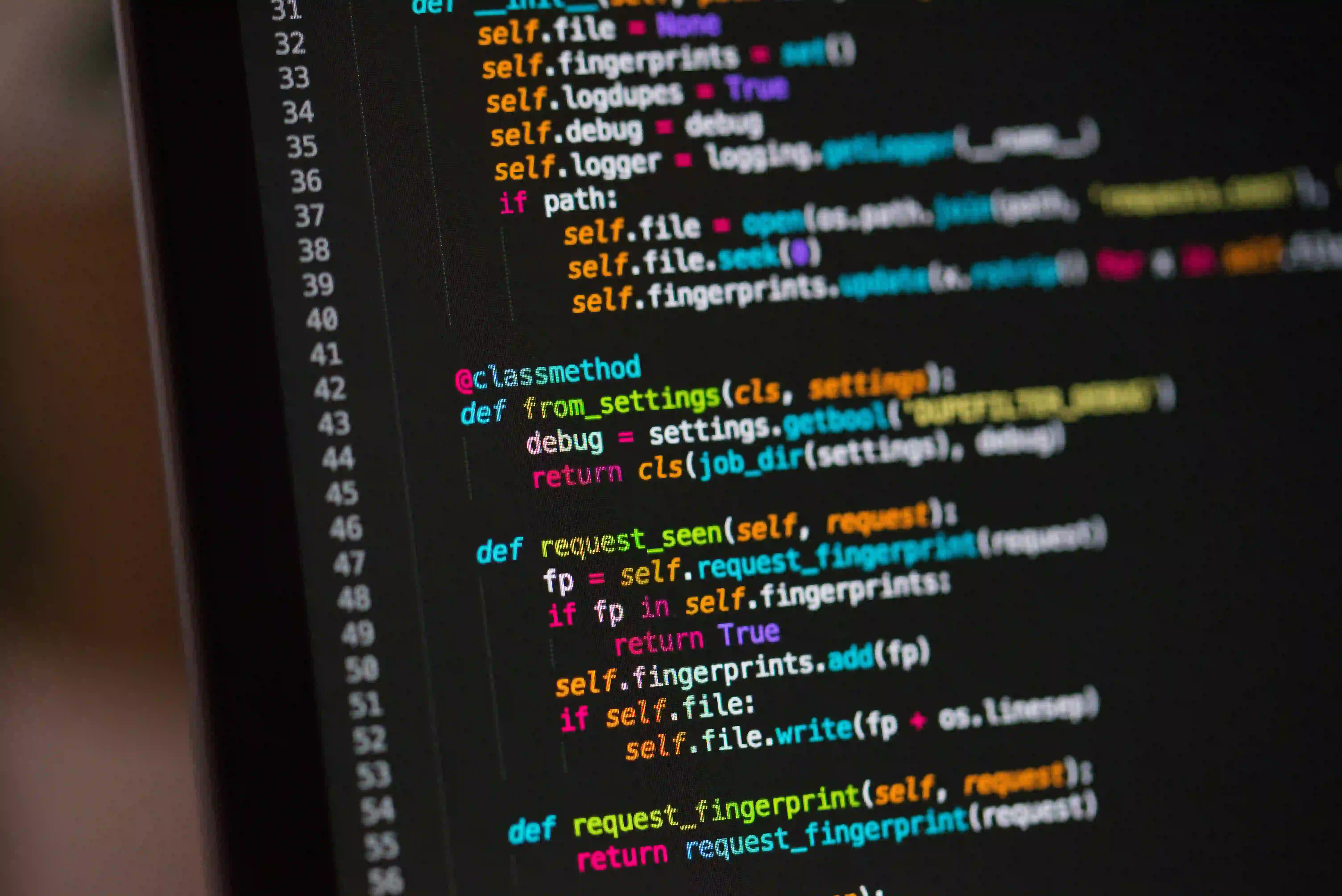
Stop Inheriting Problems: Why Avoid Tests with Inheritance
In modern software development, particularly in Java, inheritance is a cornerstone of object-oriented programming (OOP). It promotes code reusability and allows developers to create a structured hierarchy of classes. However, when it comes to writing unit tests, the story takes a different turn.
In this blog post, we'll dive into the intricacies of inheritance and tests in Java, explore the common pitfalls that arise when relying on inheritance, and equip you with best practices for testing in a way that avoids these problems. By the end, you will have a clearer understanding of why sometimes, it's better not to inherit.
The Beauty of Inheritance
Inheritance allows you to create child classes that inherit properties and methods from parent classes. This can lead to elegant designs and reduced code duplication. For instance:
public class Animal {
void makeSound() {
System.out.println("Some generic sound");
}
}
public class Dog extends Animal {
@Override
void makeSound() {
System.out.println("Bark");
}
}
In this example, the Dog
class inherits the method makeSound
from the Animal
class but overrides it to provide a specific implementation. This concept may seem beneficial, but problems arise when writing unit tests for such structures.
Why Avoid Tests with Inheritance?
1. Fragile Base Class Problem
The fragile base class problem refers to the difficulty in maintaining stable inheritance hierarchies. When you modify the parent class, all child classes can potentially break. This makes your tests brittle because changes to a single class can cause cascading failures in tests for multiple classes.
Consider a situation where the Animal
class is updated to have a new method:
public class Animal {
void makeSound() {
System.out.println("Some generic sound");
}
void eat() {
System.out.println("Eating...");
}
}
If you forgot to implement the eat
method in the Dog
class, running tests might expose subtle bugs, leading to an increased maintenance burden.
2. Hidden Dependencies
When using inheritance, the derived class often relies on the behavior of the base class. This can obscure the understanding of what the child class does independently, making it difficult to isolate tests effectively.
If the Dog
class also relies on the state or methods of the Animal
class, any failure in the base class can lead to misleading test results that do not reflect the Dog
class's functionality.
3. Increased Complexity in Unit Tests
Unit tests are meant to validate specific units of code. However, when tests are written for classes employing inheritance, they can become complex and intertwined due to shared behavior.
Using a mocking framework like Mockito may become necessary to mock the base class behavior, leading to more complicated tests.
class DogTest {
private Dog dog;
@Before
public void setUp() {
dog = new Dog();
}
@Test
void testMakeSound() {
dog.makeSound();
// You might need to mock or verify base class behavior here in some scenarios
}
}
4. Tight Coupling
Inheritance creates tight coupling between parent and child classes. Changes in one can force changes in the other. This hampers maintainability and hinders the ability to test components in isolation.
Tightly coupled code can often lead to longer feedback times, as developers will have to run a significant number of tests, even when they may only have changed a single method.
5. Lack of Clarity
Finally, when you rely too heavily on inheritance, it may obscure the relationships and behaviors of your classes. When your tests fail, it is not clear if the failure lies in the derived class, the parent class, or somewhere else entirely.
Clear tests make debugging easier. If you avoid inheritance, your tests can operate independently, providing greater insight into where issues may be occurring.
Best Practices: Prefer Composition Over Inheritance
Rather than using inheritance, consider leveraging composition. Composition allows you to build your classes using other classes, leading to more flexible and maintainable code.
Here’s a simple example employing composition:
public class Dog {
private SoundBehavior soundBehavior;
public Dog(SoundBehavior soundBehavior) {
this.soundBehavior = soundBehavior;
}
void performSound() {
soundBehavior.makeSound();
}
}
interface SoundBehavior {
void makeSound();
}
class Bark implements SoundBehavior {
@Override
public void makeSound() {
System.out.println("Bark");
}
}
In this example, the Dog
class implements behavior via SoundBehavior
, allowing for easier testing. Now, testing Dog
becomes straightforward since the sound behavior can be mocked or stubbed.
Testing With Composition
Testing in this case is also simplified. For example:
class DogTest {
private Dog dog;
@Before
public void setUp() {
dog = new Dog(new Bark());
}
@Test
void testPerformSound() {
// Here, we can assert the expected output directly without worrying about the parent class
assertEquals("Bark", dog.performSound());
}
}
Embrace Interfaces
Interfaces are another powerful tool in Java that allow for polymorphic behavior without the downsides of inheritance. By defining an interface, you can easily define a contract that various classes can implement without being coupled to a single base class.
Closing the Chapter
When writing tests, especially in Java, relying on inheritance can introduce numerous problems: fragile base class issues, hidden dependencies, increased complexity, tight coupling, and diminished clarity. Instead, adopting composition combined with interfaces can lead to more maintainable, flexible, and testable code.
This is not to say inheritance has no place in Java; however, when it comes to testing, it's worth considering alternatives that can simplify your tests and enhance your overall software design.
For more information on unit testing, visit JUnit or Mockito. By staying informed on best practices, you can avoid common pitfalls and build robust, maintainable applications. Happy coding!